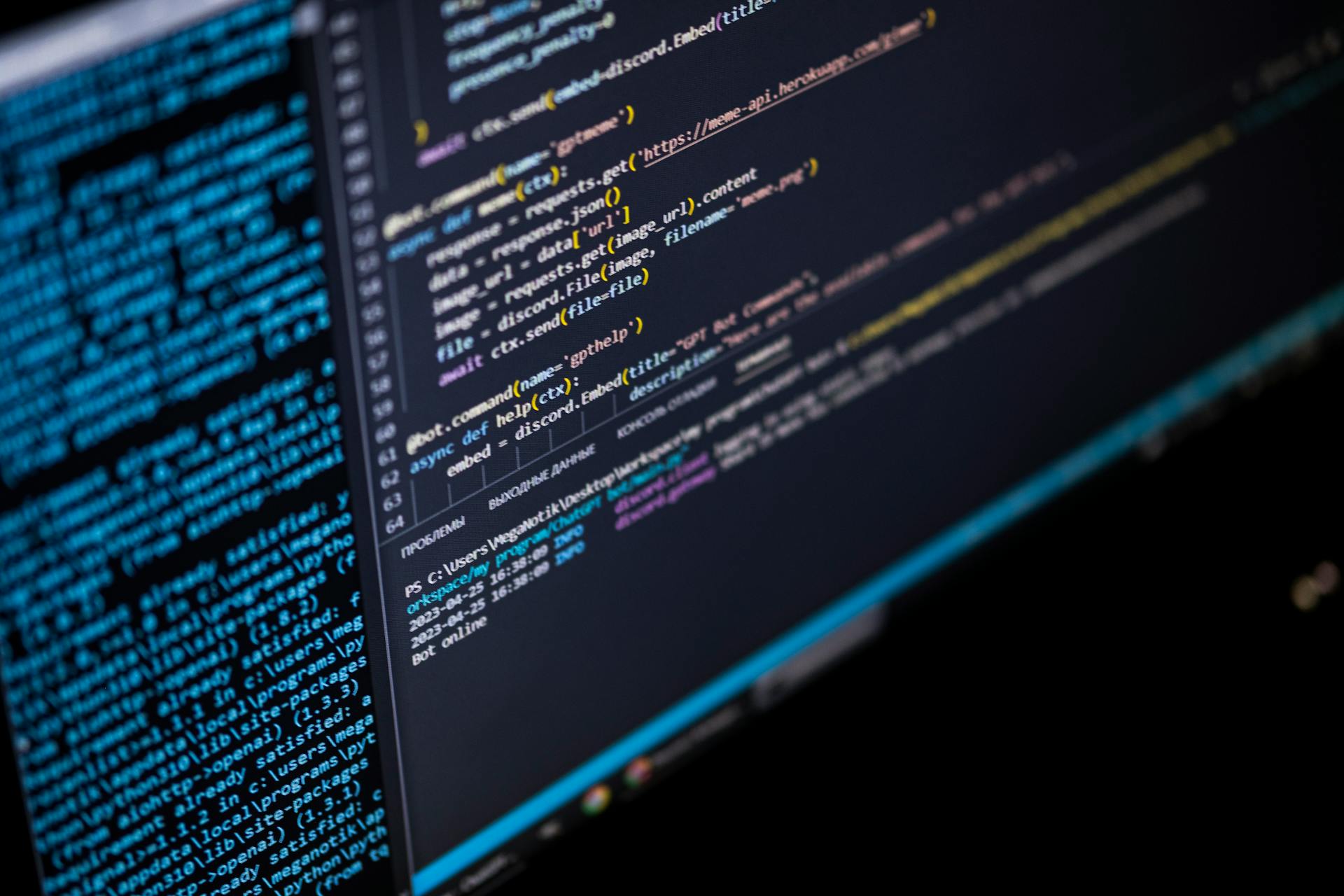
Converting Next.js to TypeScript can seem daunting, but it's a great way to add type safety and maintainability to your project.
To start, you'll need to have Node.js and npm installed on your machine, as well as a basic understanding of Next.js.
Next.js is built on top of React, so if you're already familiar with React, you'll have a head start in understanding the basics of Next.js.
You can start by creating a new Next.js project using the `create-next-app` command, which will give you a basic project structure to work from.
What is TypeScript?
TypeScript is a superset of JavaScript that adds optional static typing and other features to improve the development experience.
TypeScript was created by Anders Hejlsberg, the lead designer of C# and Visual Basic .NET, who aimed to address the limitations of JavaScript.
TypeScript is designed to work seamlessly with existing JavaScript code and libraries, allowing developers to incrementally adopt static typing and other features.
TypeScript is developed by Microsoft and is open-source, which means it's free to use and distribute.
TypeScript's static typing helps catch errors early in the development process, reducing the likelihood of runtime errors.
TypeScript's type checking can also help improve code maintainability and readability by providing clear and concise type information.
TypeScript's optional static typing means that developers can choose to use it or not, depending on their project's needs.
TypeScript's type system is based on a concept called "type inference", which allows TypeScript to automatically infer the types of variables and expressions.
TypeScript's type inference is based on the concept of "type annotations", which are used to explicitly specify the types of variables and expressions.
TypeScript's type annotations can be used to specify the types of function parameters, return types, and variable types.
TypeScript's type annotations can also be used to specify the types of object properties and array elements.
TypeScript's type annotations can help improve code maintainability and readability by providing clear and concise type information.
TypeScript's type annotations can also help catch errors early in the development process, reducing the likelihood of runtime errors.
TypeScript's type annotations are optional, which means that developers can choose to use them or not, depending on their project's needs.
Setting Up and Migration
Setting up and migration to TypeScript in Next.js is a straightforward process. You can start by renaming your JavaScript files to TypeScript files, for example, renaming index.js to index.ts.
To migrate an existing Next.js project to TypeScript, you can follow these steps: create a tsconfig.json file, install the necessary dependencies, populate the tsconfig.json file, and rename the extensions of your JavaScript files from .js to .ts.
You can also add TypeScript to an existing project by creating a tsconfig.json file, installing the necessary dependencies, and populating the tsconfig.json file. Then, rename your JavaScript files to TypeScript files by changing their extensions to .ts or .tsx.
Here's a summary of the steps:
- Create a tsconfig.json file
- Install the necessary dependencies
- Populate the tsconfig.json file
- Rename JavaScript files to TypeScript files
By following these steps, you can successfully set up and migrate your Next.js project to TypeScript.
Creating and Configuring
To create and configure a Next.js project for TypeScript, you need to install the necessary dependencies. This can be done by running the command in the terminal.
Next, you need to create a tsconfig.json file in the root of your project. This file contains all the configurations of the project. You can create it by running a command in the terminal, and by default, the file will be empty.
The tsconfig.json file needs to be populated with some basic configurations. This can be done by running a command in the terminal. It's also recommended to add the moduleResolution rule if it's not present in the tsconfig file.
Here are the basic steps to create and configure a Next.js project for TypeScript:
Once you've completed these steps, you can start using TypeScript in your Next.js project by renaming your JavaScript files to TypeScript files.
Benefits of
Using TypeScript in a Next.js project offers several benefits.
Type Safety is a significant advantage, allowing you to catch type-related errors at compile-time rather than runtime, which helps in reducing bugs and increasing the overall reliability of your code.
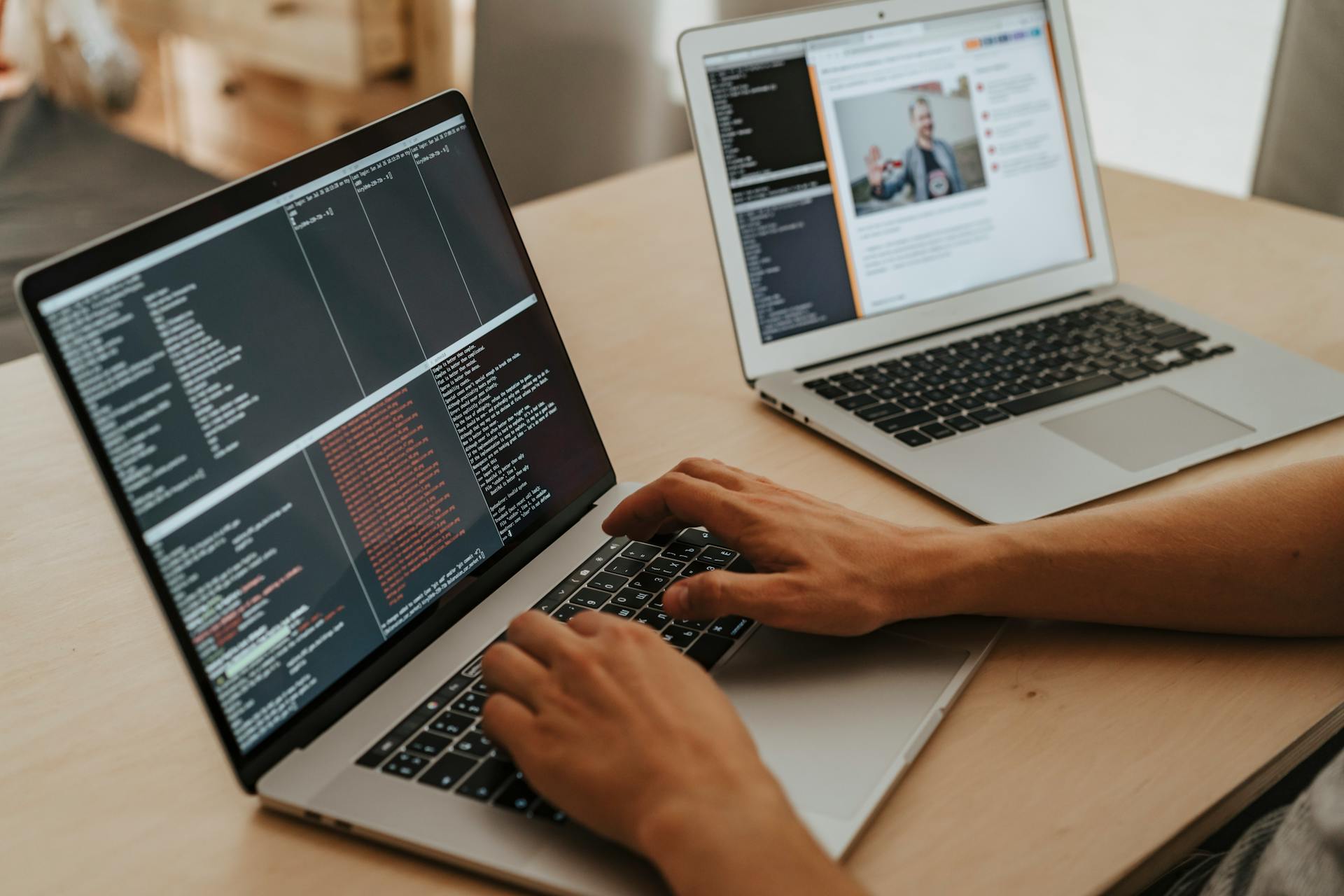
Better Tooling support is another benefit, including autocompletion, type inference, and refactoring capabilities, which improve developer productivity and make code maintenance easier.
TypeScript's type annotations act as documentation for your code, making it easier for other developers to understand and collaborate on your Next.js project.
TypeScript encourages writing clean and maintainable code by enforcing strict type checks, leading to better code quality and reducing the likelihood of introducing common programming errors.
With TypeScript, refactoring becomes easier and safer, as the compiler provides feedback on potential issues during refactoring, allowing you to make changes with confidence.
Project Configuration
To configure a Next.js project for TypeScript, you need to create a tsconfig.json file in the root of your project. This file contains all the configurations for your project.
The tsconfig.json file is created by running a command in the terminal. Once installed, you can populate the file with some basic configurations by running another command. You can then customize these configurations as needed.
Next, you need to install the necessary dependencies for TypeScript. This can be done by running a command in the terminal. The dependencies will be installed, and you'll be ready to start using TypeScript in your project.
Here are the steps to create a tsconfig.json file:
1. Create a tsconfig.json file by running the command `npx tsc --init` in the terminal.
2. Install the dependencies by running the command `npm install --save-dev typescript @types/node @types/react @types/next` in the terminal.
3. Populate the tsconfig.json file by running the command `npx tsc` in the terminal.
4. Add the moduleResolution rule to the tsconfig.json file if it's not already present.
After completing these steps, you can start using TypeScript in your Next.js project by renaming your JavaScript files to TypeScript files.
Creating Components
Creating components in Next.js is a straightforward process. You can create your components in the Next.js TypeScript app, and in the example given, a counter component is created.
To create a new component, you can create a new folder named components inside the pages folder. In this folder, you can create a new file with a .tsx extension, such as IncDecButton.tsx. The type of state counter is specified as a number, which is useful for declaring the type of variables.
The Next.js app is ready to be tested on the browser window after performing all the steps. To test the app, you can locate the root of the project and run the command. If everything works as expected, you can see your Next.js app with TypeScript at http://localhost:3000/.
Refactoring
Refactoring is a crucial step in the process of creating and configuring a project. You'll notice your project still runs fine, and this is because all of your .js or .jsx files are still JavaScript.
To switch them to TypeScript, simply change the file extension to .ts or .tsx. This small change can make a big difference in the functionality and efficiency of your project.
By making this switch, you'll be able to take advantage of the benefits of TypeScript, including improved code quality and maintainability. Your project will still run without any issues, thanks to the seamless transition from JavaScript to TypeScript.
Improving Performance
Enabling strict mode in TypeScript can help catch potential errors at compile-time and promote better code quality by setting "strict": true in your tsconfig.json file.
Writing efficient and performant TypeScript code involves following best practices such as minimizing unnecessary type checks.
Incremental compilation in Next.js uses TypeScript's incremental compilation feature to speed up subsequent builds by only recompiling modified files.
Leverage Next.js's built-in optimizations like Babel macros, tree shaking, and code splitting to reduce bundle sizes and improve performance.
Using performance profiling tools like the Chrome DevTools Performance tab can help identify and optimize performance bottlenecks in your Next.js application.
Static optimization in Next.js, combined with TypeScript, allows for efficient pre-rendering of static pages, improving performance and reducing the time to first meaningful paint (TTMP).
Code splitting in Next.js, aided by TypeScript's type system, enables efficient splitting and lazy loading of code into smaller chunks.
Optimizing algorithms and minimizing object creation can also contribute to writing efficient and performant TypeScript code.
Testing and Debugging
Testing and Debugging is a crucial part of the conversion process to TypeScript in Next.js. You can follow the same testing strategies as regular TypeScript projects, including unit testing with Jest and integration testing with Jest, Testing Library, or Cypress.
Unit testing is a great way to ensure individual functions, components, and modules work correctly, and TypeScript's type system helps provide type-safe mocks and assertions. Integration testing, on the other hand, ensures different parts of your Next.js application work together correctly.
For debugging, you can use breakpoints to pause execution at specific lines and inspect variables, or utilize console.log statements to log values and debug information. TypeScript's type system helps ensure you log the correct types, reducing the likelihood of type-related errors.
Testing Strategies
Testing Strategies are crucial for ensuring the quality of your Next.js application. Unit Testing is a great place to start, where you write tests for individual functions, components, and modules using Jest.
You can utilize TypeScript's type system to provide type-safe mocks and assertions. This helps catch bugs early on and ensures your code is stable.
Integration Testing is another important strategy, where you test how different parts of your application work together. You can use tools like Jest, Testing Library, or Cypress to write integration tests that cover multiple components, API routes, or pages.
Snapshot Testing is a useful feature in Jest that allows you to capture the output of a component or data structure and compare it against a stored snapshot. This helps catch unexpected changes in the output and ensures consistent behavior.
End-to-End Testing simulates user interactions and tests the entire application flow. Tools like Cypress or Selenium can be used to write end-to-end tests for your Next.js application.
Mocking APIs is essential when testing components or modules that interact with external APIs. You can use mocking libraries like msw or nock to mock API responses, and TypeScript's type system can help ensure that the mocked responses match the expected types.
Debugging
Debugging is a crucial part of the testing process, and there are several techniques you can use to identify and fix issues in your code.
Use breakpoints to pause the execution of your code at specific lines, allowing you to inspect variables and step through the code to identify problems.
Inserting console.log statements in your code can help you log values and debug information, and TypeScript's type system ensures that you log the correct types, reducing the likelihood of type-related errors.
TypeScript's type inference can help you identify potential issues during debugging, so pay attention to the inferred types and check if they match your expectations.
Inspecting TypeScript compiler errors can provide valuable insights into the root causes of issues, and you can use your IDE's error panel or the command line output to understand the error messages.
State
State is crucial for storing data in a React application. You can add types to a useState() hook by providing a type parameter to the function.
TypeScript will do its best to infer the type from your state's initial value, but for more complex examples, you have to include the type parameter. This is especially true when working with arrays of data, like an array of Facts.
If you're storing an array of data in state, make sure to include the type parameter. TypeScript will use this information to help catch any type-related errors.
Deploying and Tooling
Deploying and tooling is a crucial step in the process of converting your Next.js app to TypeScript. To deploy a Next.js app with TypeScript, you'll need to use the following command to build your app for production.
You can then start the server in production mode by running the server in production mode. This will prepare your app for deployment to a hosting platform or service.
Some popular options for deploying your Next.js app with TypeScript include Vercel, AWS Amplify, Heroku, and Netlify. Vercel, in particular, is a great choice because it has built-in support for TypeScript and offers seamless deployment workflows.
Deploying a App
Deploying a Next.js app is similar to deploying a regular Next.js app, and it can be done using various hosting platforms or services.
To start, you'll need to build your Next.js app for production using the command `next build`. This step is crucial in preparing your app for deployment.
You can then start the server in production mode using the command `next start`. This will make your app available for users to access.
Some popular options for deploying your Next.js app include Vercel, AWS Amplify, Heroku, and Netlify. Vercel, in particular, is a great choice since it's the creator of Next.js and has built-in support for TypeScript.
AWS Amplify is another cloud-based service that simplifies the deployment of web applications, including Next.js apps with TypeScript. Heroku is a platform as a service (PaaS) that supports Next.js apps and offers a straightforward deployment process.
Netlify is a popular hosting platform for static websites and JAMstack applications, including Next.js apps with TypeScript. It provides easy deployment and continuous integration workflows.
Tooling and Resources
To get the most out of your Next.js project, you'll want to leverage the right tooling and resources. TypeScript is the primary tool for working with TypeScript in Next.js, providing static typing and other language features that improve code quality and maintainability.
TypeScript ESLint is a must-have for linting your TypeScript code and enforcing coding standards. It provides rules specific to TypeScript and integrates well with Next.js projects.
Prettier is a code formatter that helps maintain consistent code style, and using it in combination with TypeScript can format your code automatically.
DefinitelyTyped is a repository of TypeScript type definitions for popular JavaScript libraries, providing type definitions for many Next.js dependencies, allowing you to use them with TypeScript.
The TypeScript Handbook is an official guide that covers all aspects of TypeScript, providing detailed explanations, examples, and best practices for using TypeScript effectively.
Sources
- https://nextjs.org/docs/app/api-reference/config/typescript
- https://www.scaler.com/topics/typescript/next-js-typescript/
- https://nono.ma/build-a-next-js-app-with-react-typescript
- https://www.squash.io/how-to-use-typescript-with-nextjs/
- https://upmostly.com/next-js/how-to-convert-your-next-js-app-to-typescript
Featured Images: pexels.com