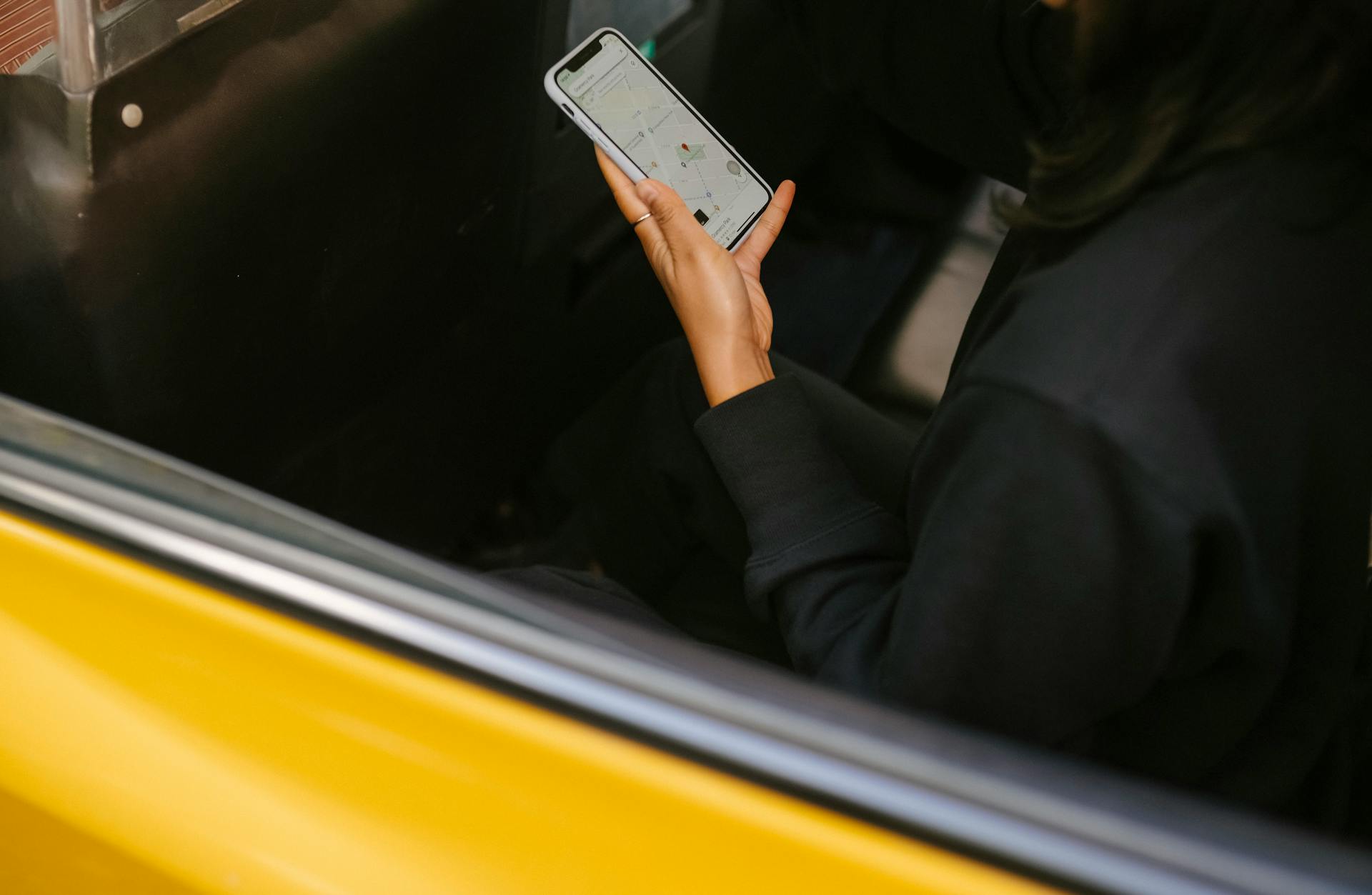
Building a high-performance SwiperJS slider with TypeScript and NextJS requires a solid understanding of how to integrate these technologies effectively.
To start, you'll need to install the necessary packages, including SwiperJS and the TypeScript definitions.
In the article, we'll explore how to properly set up SwiperJS with NextJS, including importing the necessary modules and configuring the slider.
With the basics covered, we can dive into the specifics of building a high-performance slider, including optimizing images and using lazy loading to improve performance.
Intriguing read: Nextjs Performance
Slider Configuration
Slider Configuration is crucial to create a visually appealing and user-friendly interface. To achieve this, you can customize the slider's width and height.
By default, Swiper's width is set to 100%, but you can change it to a fixed value, for example, 800px, to create a responsive slider. The height can also be customized to match your design.
In the example from the article, the width is set to 100%, and the height is set to auto, allowing the slider to adapt to its content. This is a great way to create a flexible and scalable slider.
On a similar theme: Nextjs Slider
Configuring in React
Configuring in React is a crucial step in creating a smooth user experience. You can add custom styles to your Swiper component by creating a CSS file, such as src/components/Swiper.css.
To create a Swiper component in React, you need to add some basic configuration. This includes defining the slides, navigation, and other essential features.
You can customize the appearance of your Swiper component by adding custom styles to the CSS file. For example, you can add styles for the Swiper component by following the code in the Swiper styles section.
In React, you can configure the Swiper component by using props to pass configuration options. This allows you to customize the behavior and appearance of the Swiper component.
By following these configuration steps, you can create a responsive and user-friendly Swiper component in your React application.
On a similar theme: Next Js File Upload
Controller
The Controller is a crucial part of setting up your Swiper instances. It requires passing one Swiper instance to another.
For your interest: Swiper in Nextjs
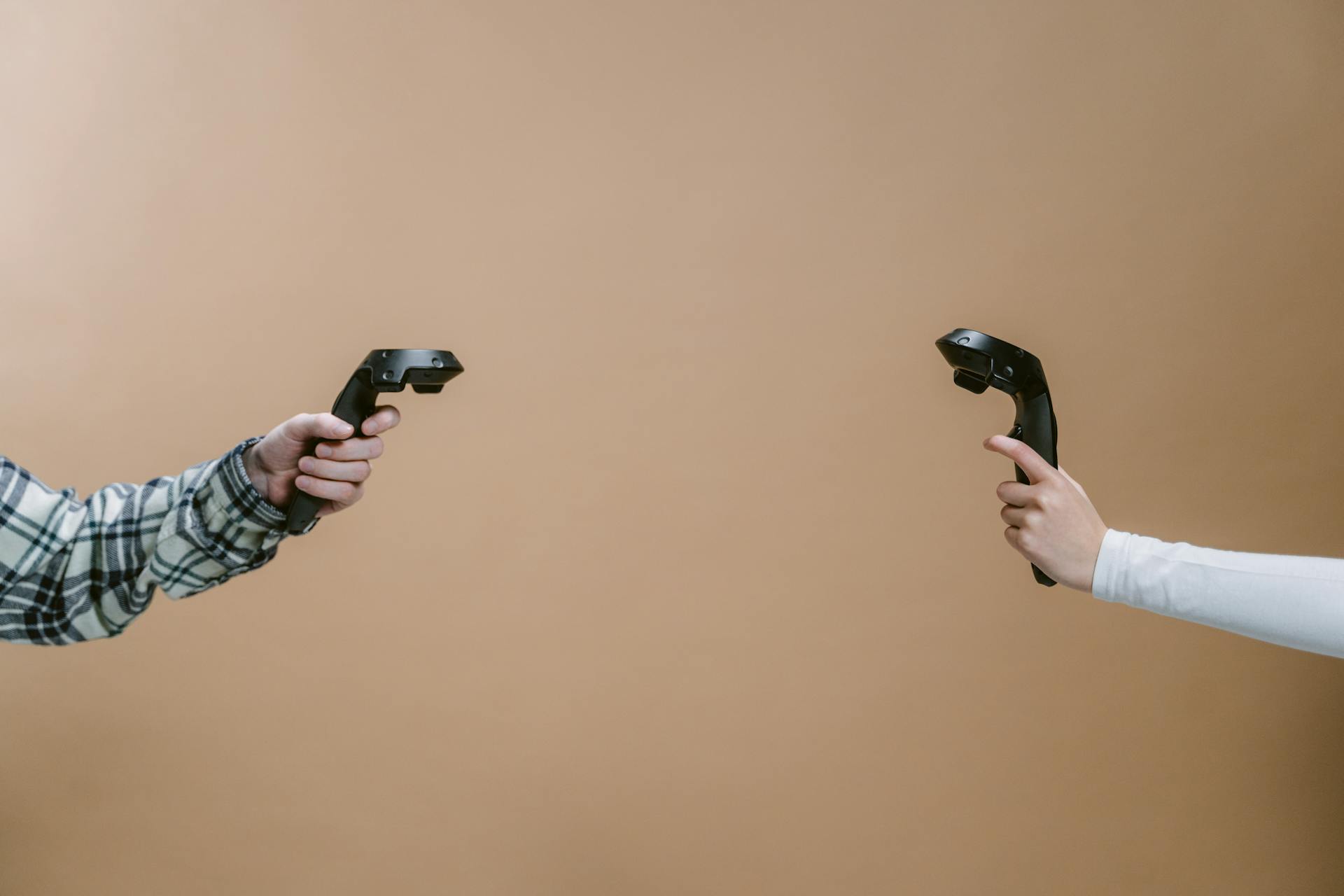
For two-way control, you'll need to set it up like this: pass one Swiper instance to another. This setup allows both Swipers to control each other.
The Controller's job is to facilitate communication between Swiper instances. It's essential to get this right to avoid any issues with your slider configuration.
To achieve two-way control, you'll need to pass the Swiper instance to the Controller. This will enable both Swipers to control each other seamlessly.
Slider Logic
Slider Logic is all about creating a seamless user experience. In a typical Next.js application, SwiperJS can be used to create a slider that automatically switches between slides after a certain interval.
To set up automatic switching, you can use the `autoplay` property in the SwiperJS configuration. This property is set to `true` by default, but you can change it to `false` if you prefer to control the slider manually.
The interval at which the slider switches between slides can be set using the `loop` property, which is set to `true` by default. This means the slider will automatically switch to the next slide after a certain interval, even if it's the last slide in the list.
Prevent Swiping
To prevent swiping on both ends, we need to understand the problem. We already know that we use offsetX to move the container of the image, and that it shouldn't move beyond the images. The far most left offsetX value is 0, since we start with 0 for offsetX to display the first image.
The far most right offsetX value is the width of the container, which we'll call minOffsetX. We can get the width of the container using the useRef for the container element.
We need to deduct the display size of the container from the scrollWidth to get the correct value, since we start from 0 to display the first image. This will give us a negative value to move the images from right to the left.
To prevent swiping from either end, we can add if conditions to check if the offsetX value is less than 0 or greater than the container's width. We can use the maxOffsetX and minOffsetX values we calculated earlier to make these checks.
Here's an interesting read: Nextjs Useeffect
Touch/Drag Logic
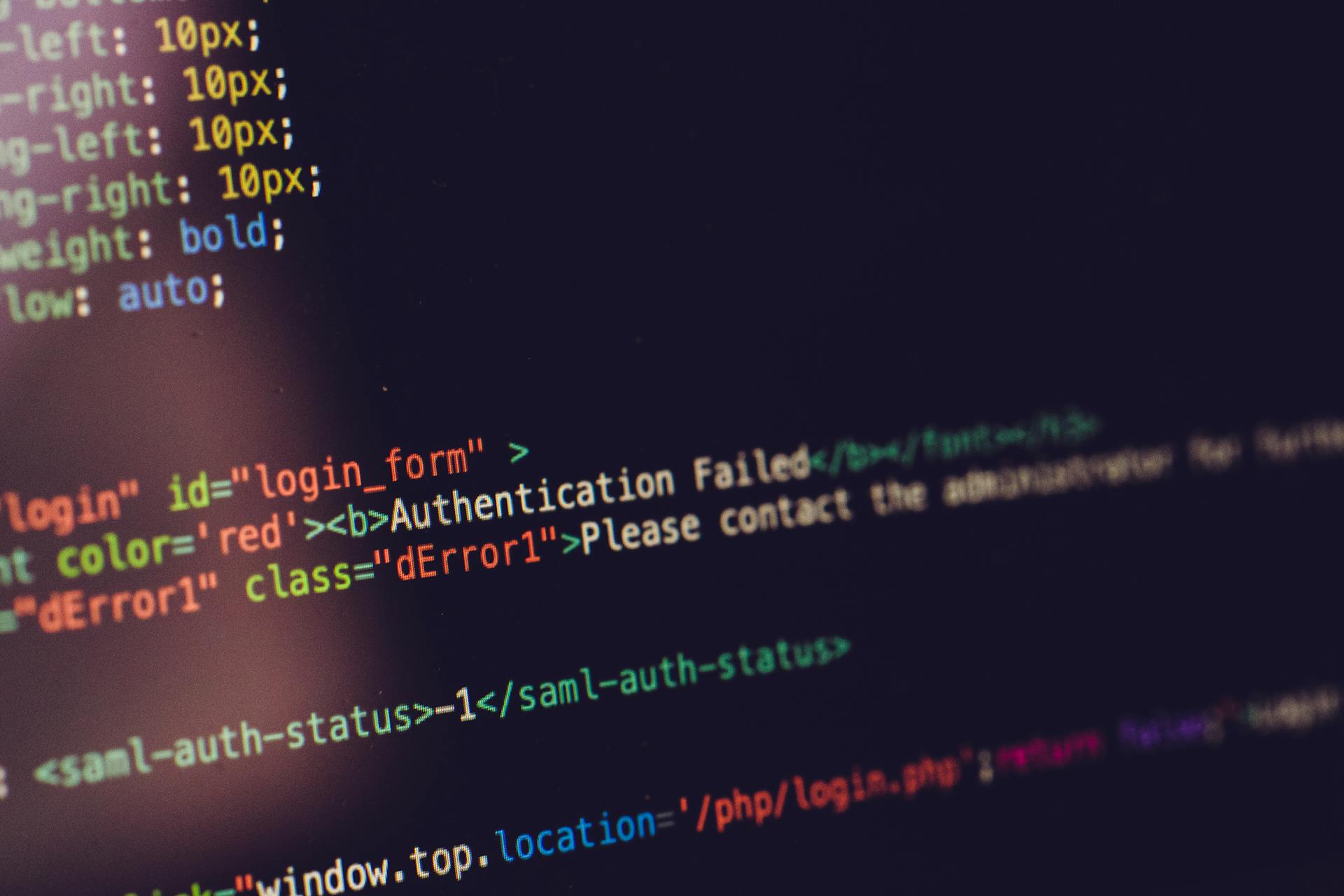
Touch/Drag Logic is a crucial aspect of Slider Logic. It determines how the slider responds to user input.
A slider's touch/drag logic can be set to either "touch" or "drag" mode. In "touch" mode, the slider snaps to the nearest value when the user touches it. This is useful for applications where precise control is not necessary.
In "drag" mode, the slider moves smoothly as the user drags their finger across the screen. This is ideal for applications where precise control is required, such as audio levels or font sizes.
The slider's touch/drag logic can also be customized to respond to different types of user input, such as tap, swipe, or pinch gestures. This allows developers to create unique and engaging user experiences.
A good example of touch/drag logic in action is a music player app, where the user can adjust the volume by dragging their finger up or down on the screen.
Slide Render Function
The SwiperSlide component has a render function that receives an object with several properties. This function is a powerful tool for customizing your slides.
The object passed to the render function contains properties like isActive, isPrev, isNext, isVisible, and isDuplicate. isActive is true when the current slide is active.
These properties can be used to conditionally render elements or apply different styles to your slides. For example, you can show a different image or text when the slide is active.
Here are the properties you can expect to receive in the object:
- isActive - true when the current slide is active
- isPrev - true when the current slide is the previous from active
- isNext - true when the current slide is the next from active
- isVisible - true when the current slide is visible (watchSlidesProgress Swiper parameter must be enabled)
- isDuplicate - true when the current slide is a duplicate slide (when loop mode enabled)
By using the render function and these properties, you can create a dynamic and engaging slider experience for your users.
Slider Props and Functions
The Swiper React component receives all Swiper parameters as component props, plus some extra props.
The extra props include tag, wrapperTag, and onSwiper, which allow you to customize the HTML element tags and receive the Swiper instance.
Additional reading: Next Js Typescript Example Props
You can customize the main Swiper container HTML element tag with the tag prop, which defaults to 'div'.
The wrapperTag prop allows you to customize the Swiper wrapper HTML element tag, which also defaults to 'div'.
The onSwiper prop is a callback that receives the Swiper instance.
Here's a list of the extra props:
- tag: string, defaults to 'div'
- wrapperTag: string, defaults to 'div'
- onSwiper: (swiper) => void
The useSwiperSlide hook is another useful function for components inside of Swiper slides, which allows you to get the slide data.
Props
Props are a fundamental part of building reusable components in React. They allow you to pass data from a parent component to a child component, making it easy to customize and reuse components across your application.
In the context of the Swiper component, props are used to pass an array of Swiper items. This is done by wrapping the SwiperItemType type in an Array, like this: `SwiperItemType[]`. This tells TypeScript that the field accepts an array of Swiper items.
For your interest: Next Js Components Folder
The Swiper component also accepts other props, such as the tag, wrapperTag, and onSwiper props. These props are used to customize the appearance and behavior of the Swiper component.
Here's a summary of the props accepted by the Swiper component:
The SwiperItem component also accepts props, specifically the source URL of the image and an alternative text for each item. These props are defined as required string fields, ensuring that they are always passed to the component.
By using props, you can make your components more reusable and customizable, which is essential for building robust and maintainable React applications.
If this caught your attention, see: Nextjs Component Library
Indicators
Indicators are a crucial feature to add to our swiper, allowing users to know how many images are in the swiper and which one is currently displayed.
We can simply loop the images again to display the indicators, using Array.map() to loop the array and get their index. This index will be stored in a state variable called currentIdx.
The number of indicators we need is equal to the number of images we have. We can use this information to create the indicators and make them clickable to move around the images.
To set the new currentIdx after the user does a swipe or drag, we can divide the offsetX by the containerWidth, making sure to use Math.abs() to handle negative values.
To move the images when we click on an indicator, we can multiply the index and the containerWidth, making it negative to move the images to the left.
This approach will allow us to create a smooth and intuitive user experience, making it easy for users to navigate through the images in our swiper.
Worth a look: How to Use Reducer Api in Next Js 14
Sources
- https://dominicarrojado.com/posts/how-to-create-your-own-swiper-in-react-and-typescript-with-tests-part-1/
- https://swiperjs.com/react
- https://stackoverflow.com/questions/77703570/getting-error-in-swiper-slider-when-using-it-with-next-js-and-typescript
- https://medium.com/@mehdi.jabbour/how-to-install-and-use-swiper-js-in-reactjs-nextjs-5fba193596a
- https://dev.to/ivadyhabimana/customizing-swiperjs-prevnext-arrow-buttons-and-pagination-bullets-in-react-3gkh
Featured Images: pexels.com