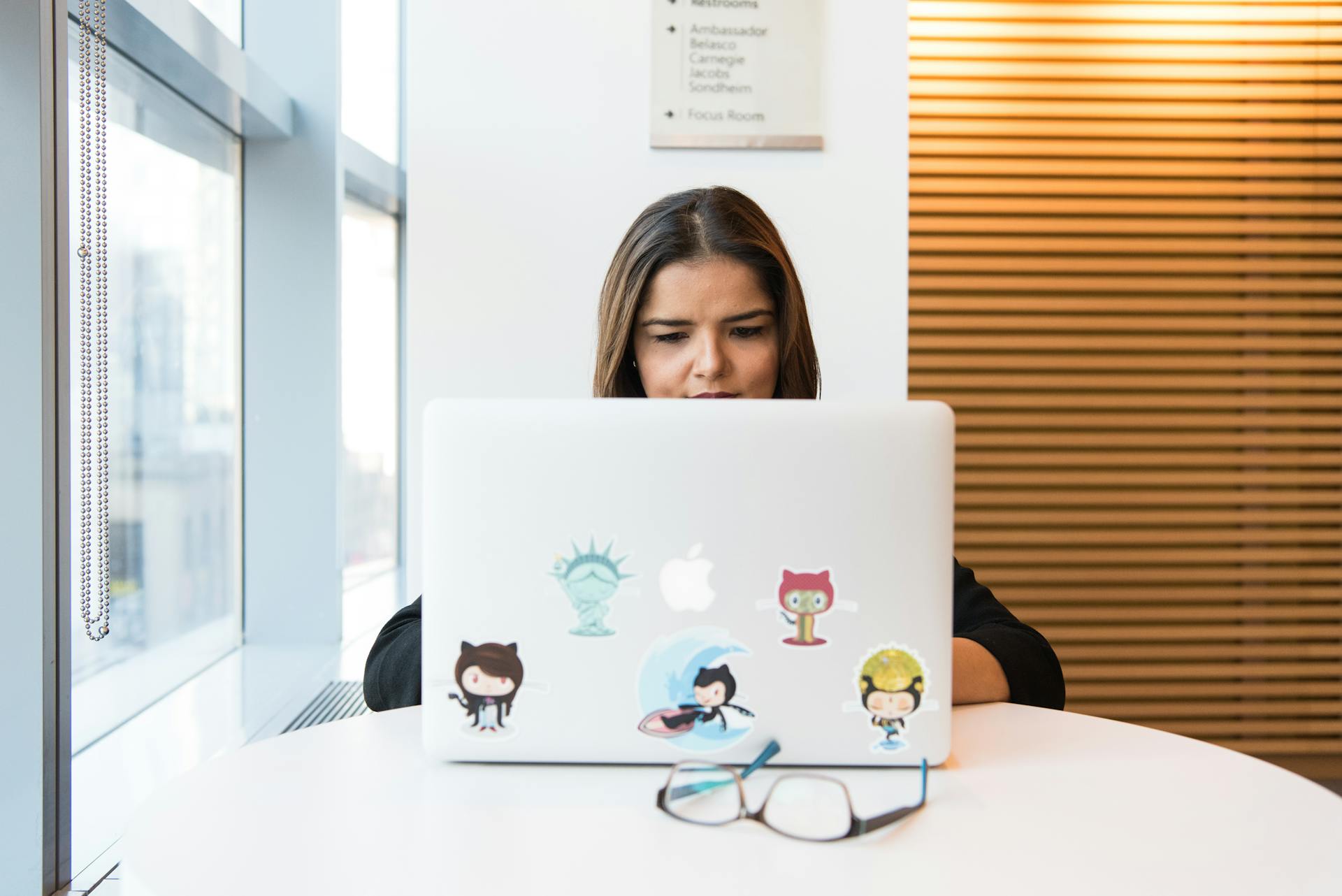
Implementing Swiper in Next.js can seem daunting, but it's actually quite straightforward. You can install Swiper using npm or yarn, and then import it into your Next.js project.
First, make sure you have a basic understanding of Next.js and its file structure. Next.js projects typically have a pages directory where all the pages are stored. Swiper will be used to create a carousel component that can be used across multiple pages.
To get started, create a new component for your carousel, and import Swiper from the Swiper library. You can then initialize Swiper in your component, and use its methods to control the carousel.
Explore further: Next Js Draggable
Creating a Slider Component
To create a slider component in Next.js, you'll need to create a "components" folder in your app's root directory. Inside this folder, create two new files: "Slide" and "Slider".
The "Slide" component should be a .jsx/.tsx file that defines a new component called "Slide" with a single prop called "content". This prop will be used to display the content added to your slides in Contentful.
Check this out: Nextjs Slider
The "Slider" component should be another .jsx/.tsx file that defines a new component called "Slider" with a prop called "slides". This prop will be an array of all your slide objects retrieved from Contentful.
You'll need to import Swiper and SwiperSlide from swiperjs, as well as your Slide component, into your Slider component.
Here are the import statements you'll need:
- Swiper and SwiperSlide from swiperjs
- Our Slide component
- Swiper’s css file
In your return statement, you'll want to return a Swiper component and map through all the slides you got passed to render a Slide component inside a SwiperSlide component for each one.
You'll also need to pass some parameters to the Swiper component, such as spaceBetween, slidesPerView, and centeredSlides, to customize the slider.
Contentful and GSAP Integration
Contentful is a headless CMS that allows you to manage your content in a flexible and scalable way. It's a great tool to use with Next.js and GSAP for creating interactive and dynamic web applications.
One of the key benefits of using Contentful with GSAP is that it enables you to separate your presentation layer from your content. This makes it easier to manage and update your content without having to worry about the underlying code.
With Contentful, you can create a content model that defines the structure of your content, and then use GSAP to animate and interact with that content in a visually appealing way. This is especially useful for creating complex animations and interactions that require a high degree of customization.
GSAP's powerful animation engine can be used to bring your content to life, and Contentful's flexible content model makes it easy to manage and update that content over time. This combination of tools makes it easy to create high-quality, interactive web applications that engage and delight your users.
In our example, we used Contentful to manage the content for our Swiper component, and GSAP to animate and interact with that content in a visually appealing way. This allowed us to create a highly customizable and dynamic web application that met the needs of our users.
Handling Events and Interactions
Handling events and interactions with Swiper in Next.js is crucial for creating a seamless user experience. To listen for dispatched events, we need to create a new component called AnimationEventListener.
We'll put this component inside our Swiper component in the Slider file, where it won't return any JSX, but will have a useEffect that adds event listeners for our custom events. The useSwiper hook is essential here, as it lets us programmatically update our Swiper Slider.
We'll create a swiper variable and assign it to this useSwiper hook. To handle adding and removing the event listeners, we'll make two functions: addListeners and removeListeners.
Inside our addListeners function, we'll add event listeners for our events, and define a function called slide to update the slide based on the nextIndex property passed with the event. We'll use the slideTo function from useSwiper to update the slide.
Before sliding to the next slide, we'll check that swiper.params are not undefined, to ensure we're getting the correct data about our Slider. We'll also check that swiper.destroyed is not equal to true, to avoid adding event listeners when we don't have the correct data.
If swiper.destroyed is false, we'll add the event listeners. Finally, we'll return a cleanup function in our useEffect that calls the removeListeners function.
Thumbnail Slider
To build a thumbnail slider in Next.js, you'll need to create a new directory in your project called "components". Inside this directory, create a new file named ThumbnailSlider.tsx or .jsx, depending on your JavaScript preferences.
In this file, you'll want to add the necessary imports for Swiper. You can do this by copying the following code: [list of imports]. This will allow you to use Swiper in your ThumbnailSlider component.
To make your ThumbnailSlider component functional, you'll need to map through an array of slide objects and render a SwiperSlide component for each one. This will create the thumbnail slider that users can click through.
Here's a breakdown of the necessary parameters to pass into the Swiper component: [table]
By following these steps and using the necessary parameters, you'll be able to create a functional thumbnail slider in Next.js using Swiper.
Sources
- https://flowbite.com/docs/components/carousel/
- https://sitecore.stackexchange.com/questions/38503/how-to-use-js-in-carousel-code-into-a-functional-next-js-component-for-scrolling
- https://medium.com/@mehdi.jabbour/how-to-install-and-use-swiper-js-in-reactjs-nextjs-5fba193596a
- https://www.xtivia.com/blog/contentful-swiperjs-and-gsap-integration-for-animations/
- https://blog.stackademic.com/creating-a-thumbnail-slider-in-next-js-with-tailwind-and-swiper-88cd3fd67709
Featured Images: pexels.com