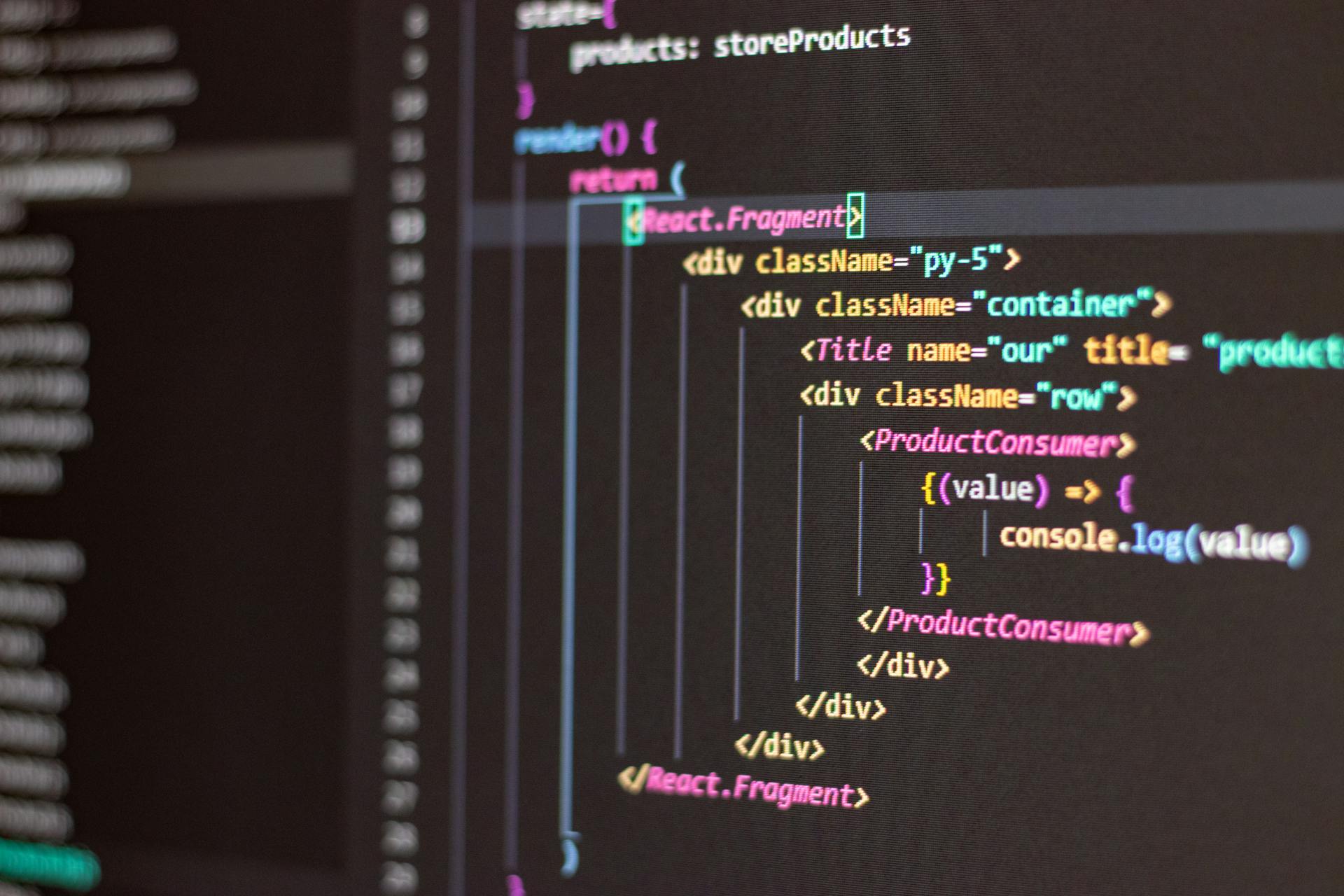
Building a Draggable Todo List with Next Js is an exciting project that can be achieved with the right tools and techniques. To get started, you'll need to set up a new Next Js project using the command `npx create-next-app my-todo-app`.
You can then create a new component for your todo list item, which will contain the draggable functionality. This is done by using the `draggable` attribute on the todo item element, as shown in the code snippet: `...`.
To make the todo list items actually draggable, you'll need to define the `handleDragStart` function, which will be called when the user starts dragging an item. This function will return the data transfer object, which contains information about the dragged item.
The `handleDragOver` function is also crucial in making the todo list items draggable, as it will be called when the user hovers over a list item with a dragged item. This function will prevent the default behavior of dropping the item, allowing the user to drag it to a new location.
Discover more: Using State in Next Js
Serverless Todo List
In a serverless architecture, the Todo List app is a great example of how to handle state management efficiently.
The app uses a combination of React Context API and the useReducer hook to manage state.
This approach allows for easy sharing of state between components and efficient updates.
The Todo List app also uses a separate API endpoint to store and retrieve data.
This decouples the app's logic from the data storage and retrieval process.
The API endpoint is implemented using a serverless function, which handles requests and returns responses.
This approach eliminates the need for a dedicated server and reduces costs.
The Todo List app's API endpoint is also secured using authentication and authorization mechanisms.
This ensures that only authorized users can access and modify the Todo List data.
The serverless architecture of the Todo List app makes it highly scalable and flexible.
It can handle a large number of users and requests without requiring significant infrastructure changes.
This makes it an ideal solution for applications with variable or unpredictable traffic patterns.
Discover more: Next Js Architecture
Creating Task Rows
Creating task rows is a crucial step in building a Next.js draggable application. We start by checking if tasks is not null, because Next.js does server-side rendering.
To create task inputs, we add the following code below the last div row. The tasks state is added after the component is mounted, which means we can't rely on it being available immediately.
We create an input element for each task with a value equal to the task name. This allows users to see the task name as they type it.
Each input element also has a delete button for deleting the element. We'll use the data attributes to update the tasks state when a task is edited or deleted.
Drag and Drop Functionality
To implement drag and drop functionality in your Next.js project, you'll need to integrate Swapy, a library that makes it easy to add drag and drop capabilities to your application.
Now that Swapy is installed, you can follow a step-by-step process to set up drag and drop functionality.
The key to making drag and drop work is to set the draggable property of the task duration element to true, which creates a "ghost" image of the task duration element attached to the mouse cursor as it's dragged.
with Drag and Drop
Implementing drag and drop functionality in your project can be a game-changer for user experience. To get started, you'll need to install Swapy, a library specifically designed for this purpose.
Swapy is a powerful tool that makes it easy to add drag and drop functionality to your Next.js project. Installing it is a straightforward process that will have you up and running in no time.
To integrate Swapy into your project, you'll need to follow a step-by-step process that will guide you through the setup. This process is designed to be easy to follow and will have you implementing drag and drop functionality in no time.
With Swapy installed and set up, you'll be able to create a seamless drag and drop experience for your users. This will enhance their overall experience and make your project stand out from the crowd.
Creating the Gantt Chart Grid
Creating the Gantt Chart Grid is a crucial step in building a functional Gantt chart. We'll start by creating the grid in the TimeTable component.
The grid is created by looping through the number of months in the time period range, which is currently set to two. We'll be able to change this value later when we complete the TimeRange component's code.
We use JavaScript Date methods, as well as the getDaysInMonth and getDayOfWeek functions from our dateFunctions file, to create the day and week rows. The value for numMonths is two, and we use the month variable and the setMonth method to increment the month value on each loop.
Each row is created using a nested loop, which makes it easy to add the correct number of cells for each day and week. The data-attributes data-task and data-date are added to each cell, which we'll use later when we add drag-and-drop functionality to the task durations.
We also use the months array from our constants file to add styled React elements with the correct month name to the monthRows array. This ensures that the month names are displayed correctly in the grid.
The grid is now fully created, and we can see the year and month, day of the month, and day of the week rows. The next step is to create the task row cells and add the task durations.
For your interest: Next Js Fetch Data save in Context and Next Route
Integration and Existing App
We've made it easy to get started with Next.js draggable components. To begin, you can use the Builder Devtools for Next.js to simplify the integration process.
If you want to start from an existing GitHub repo, use the command provided in the documentation.
You can also add drag-and-drop components to an existing Next.js app, no problem! Just use the command mentioned in the article.
Consider reading: Use Theme in Server Components Nextjs
Sources
- https://wagnercaetano.dev/post/project/Serverless-Todo-List-with-Drag-and-Drop-Functionality-using-Next.js-fb7e52b
- https://developer.mozilla.org/en-US/docs/Web/API/HTML_Drag_and_Drop_API/Recommended_drag_types
- https://bryntum.com/blog/creating-a-gantt-chart-with-react-using-next-js/
- https://medium.com/@lupucl/effortlessly-implement-drag-and-drop-in-next-js-with-swapy-a-step-by-step-guide-bfa3b2792d4b
- https://www.builder.io/blog/nextjs-drag-and-drop
Featured Images: pexels.com