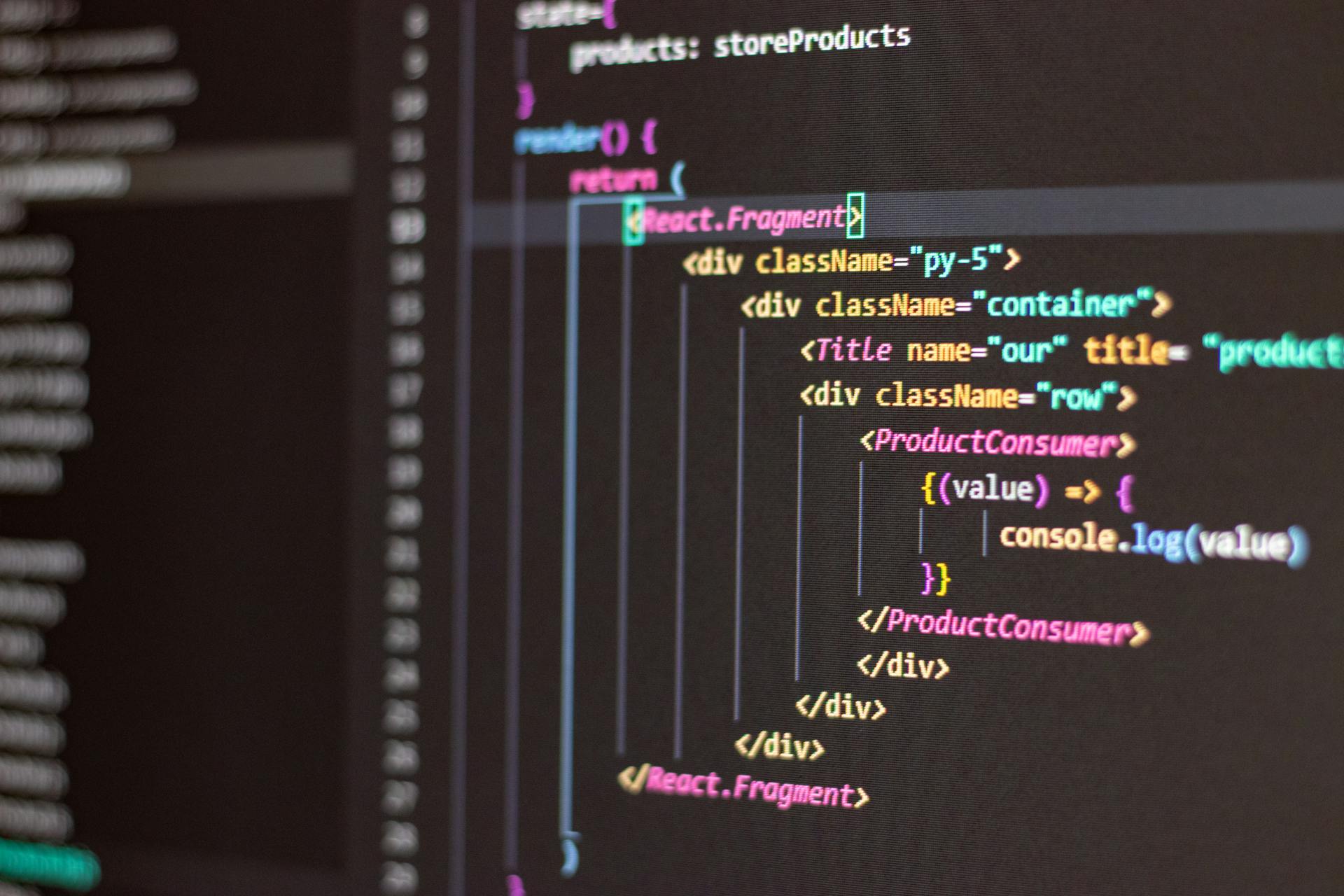
As you build your Next.js app, it's essential to follow best practices for scalable architecture. This means structuring your code in a way that allows for easy maintenance, updates, and growth.
Separate concerns by organizing your code into separate components, such as pages, layouts, and API routes. This keeps your codebase clean and maintainable.
A key aspect of Next.js architecture is its use of server-side rendering (SSR). With SSR, your app's HTML is generated on the server, improving page load times and SEO. This feature is enabled by default in Next.js.
By following these best practices, you'll be well on your way to building a scalable Next.js app that meets your users' needs.
A fresh viewpoint: Next Js Best Practices
Next.js Architecture
You can set up a Next.js project structure by using the create-next-app command, which installs the required dependencies and sets up a basic project structure. This is a great way to get started with a new project.
To maintain a clean project structure, organize your pages and components in a logical manner, taking advantage of the file-based routing system in Next.js. This means each page is represented by a separate file in the pages directory.
Intriguing read: Next Js Folder Structure
You can also set up environment variables in your Next.js project by creating a .env file, which lets you store sensitive information or configuration settings separately from your code.
Here are some key features to consider when choosing Next.js:
Setting Up Your Project
To start a new Next.js project, consider using the create-next-app command, which sets up a basic project structure and installs required dependencies.
This command is a great way to get started quickly, and it's a good idea to use it as a starting point for your project.
You can also set up environment variables in your Next.js project by creating a .env file, which lets you store sensitive information or configuration settings separately from your code.
Here are some initial files and directories you can expect to find in your Next.js project:
- pages directory: This folder is the heart of your Next.js app's routing system, where each .js file or .tsx file inside this directory automatically becomes a route based on its file name.
- public folder: This is where you'll place static assets like images, fonts, and any other files that need to be accessed publicly.
- styles folder: Contains the default CSS files for styling your app.
- .next folder: This is a generated folder that contains the output of the Next.js build process.
- node_modules directory: Houses all the third-party libraries and dependencies for your app.
- package.json file: Keeps track of all the dependencies and scripts for your Next.js project.
- next.config.js file: An optional config file that allows you to customize various aspects of Next.js behavior.
- .gitignore file: A pre-configured file that tells Git which files or folders to ignore in your project.
- README.md file: Provides information about your project, such as setup instructions and documentation.
Why?
Next.js has become a popular choice for developers, and for good reason. It's built on React, which means if you're familiar with React, transitioning to Next.js is seamless.
Intriguing read: Next Js React
One of the key reasons Next.js stands out is its capability for server-side rendering, which enables the rendering of pages directly on the server. This functionality enhances initial page load speed and improves Search Engine Optimization (SEO).
Automatic code splitting is another feature that makes Next.js efficient. It intelligently splits your JavaScript bundles, delivering only the code necessary for the requested page, leading to quicker page loading times and enhanced overall performance.
Routing in Next.js is simplified by adopting a file-system-based approach. Each .js file in the pages directory becomes a route, making navigation straightforward.
With built-in CSS support, developers can choose from styling solutions like CSS Modules, styled components, and Sass, which suits their preferences.
Broaden your view: Next Js Client Side Rendering
Performance Optimization
Next.js provides several features that can help improve the performance of your CMS. One such feature is server-side rendering (SSR), which allows your pages to be rendered on the server before being sent to the client.
Server-side rendering can greatly reduce the initial load time and improve SEO. Next.js also supports static site generation (SSG), where pages are generated at build time and served as static files. This can further improve performance by reducing the need for server-side rendering on every request.
Here are some key Next.js features that can help optimize performance:
- Server-side rendering (SSR)
- Static site generation (SSG)
- Code splitting
- Built-in support for caching and prefetching
Next.js's automatic code splitting and server-side rendering contribute to significantly faster page loads, improving initial load times and ensuring a smoother user experience.
Optimizing Performance
Next.js provides several features that can significantly improve the performance of your application. One such feature is server-side rendering (SSR), which allows your pages to be rendered on the server before being sent to the client, greatly reducing initial load time and improving SEO.
Server-side rendering can be further enhanced by using static site generation (SSG), where pages are generated at build time and served as static files, reducing the need for server-side rendering on every request.
Curious to learn more? Check out: Generating Static Pages Next Js
Automatic code splitting is another feature that can improve performance by breaking down your JavaScript bundles into smaller, more manageable chunks, only loading the necessary code for the requested page.
This approach improves initial load times and ensures a smoother user experience, particularly on slower network connections.
The performance boost achieved through automatic code splitting is crucial, especially in large-scale applications where minimizing initial load times is paramount.
Here are some key features that can help optimize the performance of your Next.js project:
- Server-side rendering (SSR)
- Static site generation (SSG)
- Automatic code splitting
- Built-in support for caching and prefetching
By utilizing these features, you can ensure that your application performs efficiently and provides a seamless user experience.
Optimizing for Scalability
Optimizing for Scalability is crucial as your Next.js app grows. As your application has a large number of pages, organizing the 'pages' directory into subdirectories further can be helpful.
Breaking down features into smaller, reusable modules is a great way to keep the structure scalable and maintainable. This approach is often referred to as modularization.
For your interest: Next Js Pages vs App
A cluttered or confusing folder structure can hinder your app's growth. To avoid deep nesting, keep the folder structure as flat as possible to make it easier to navigate.
Using index.js files within folders to re-export components simplifies imports and makes the structure more scalable. This technique is particularly useful when working with modular components.
Consistency is key when it comes to applying a consistent organizational structure across similar elements. This makes the pattern predictable and easier to maintain.
Here are some additional tips to help you optimize for scalability:
- Organize the 'pages' directory into subdirectories further if you have a large number of pages.
- Modularize features into smaller, reusable modules.
- Avoid deep nesting in the folder structure.
- Use index.js files to re-export components and simplify imports.
- Apply a consistent organizational structure across similar elements.
Env.local and Environment Variables
In Next.js, you can create a .env.local file at the root of your project to define sensitive information.
This file is used to store variables that you don't want to include in your version control system, such as API keys or database passwords.
Next.js loads these variables at build time, making them available in your app through process.env.
You can access these variables in your Next.js app using process.env.API_SECRET and process.env.DATABASE_URL.
This approach helps keep sensitive information secure and organized.
Broaden your view: Nextjs Build Fails Environment Variables
Component Best Practices
In a Next.js project, it's essential to implement best practices for React components. This can be achieved by using functional components instead of class components, as they are simpler and perform better.
Functional components can be leveraged to manage state, side effects, and lifecycle methods using React hooks. Hooks provide a more elegant and concise way of managing component logic, making it easier to maintain and optimize your components.
To promote code reuse and improve maintainability, follow a component-driven development approach by breaking your UI into reusable components. This can be achieved by organizing your components in a directory, such as the components directory, which is a widely adopted convention in Next.js projects.
Here are some best practices to keep in mind when organizing your Next.js project:
- Use functional components instead of class components.
- Leverage React hooks to manage state, side effects, and lifecycle methods.
- Follow a component-driven development approach.
- Implement proper error handling in your components.
- Optimize rendering performance by using memoization techniques.
By following these best practices, you can ensure that your React components are optimized, maintainable, and provide a smooth user experience in your Next.js CMS.
Best Practices for React Components
Implementing best practices for React components is crucial for ensuring the best performance and maintainability of your components in a Next.js project. Functional components are simpler and perform better than class components, so use them whenever possible.
Leverage React hooks to manage state, side effects, and lifecycle methods. Hooks provide a more elegant and concise way of managing component logic.
Follow a component-driven development approach by breaking your UI into reusable components. This promotes code reuse and improves code maintainability.
Implement proper error handling in your components to improve the user experience. Use try-catch blocks or error boundaries to catch and handle errors gracefully.
Optimize rendering performance by using memoization techniques like React.memo or useMemo. This can help prevent unnecessary re-renders and improve overall performance.
Here are some best practices to keep in mind when implementing React components:
By following these best practices, you can ensure that your React components are optimized, maintainable, and provide a smooth user experience in your Next.js CMS.
Custom Layout Setup
Custom Layout Setup is a crucial aspect of building maintainable and scalable applications. Next.js encourages reusable React components, promoting modularity and maintainability.
In Next.js v13 or greater, you already have out-of-the-box support for a layout file, which is similar to the _document.tsx file present in earlier versions. You can put any components that will be shared across the application, such as Navbar, Footer, and others, in this layout.tsx file.
To create a similar layout structure in Next.js v12, create a Layout.tsx file that wraps the children component. This Layout file would behave similarly and import your shared components to all your routes.
The layouts directory can be used to store layout components that wrap around your page content. This is useful for maintaining consistent layouts across different parts of your app, such as headers, footers, and sidebars.
In larger applications, you may have shared layouts that wrap your pages. The ‘layouts’ directory is an excellent place to store these standard layouts, such as ‘MainLayout.js’ and ‘AdminLayout.js’.
If this caught your attention, see: Next Js Src Directory
Improved SEO
Server-side rendering in Next.js is a game-changer for SEO, generating fully rendered HTML pages on the server that search engines can easily index.
This approach provides a complete snapshot of your content, making it easier for search engines to understand and rank your web pages.
Next.js facilitates better indexing and enhances discoverability of your web pages, making search engine visibility a top priority for projects that use this framework.
By leveraging server-side rendering, you can improve your website's search engine optimization and increase its online visibility.
On a similar theme: Nextjs Server Rendering Tailwind
API and Serverless
API endpoints are structured by creating files in the pages/api directory, with the file path determining the URL path. For example, a file named users.js at pages/api/users.js creates an endpoint accessible at /api/users.
The functions you define in the pages/api directory are serverless, running on demand in response to HTTP requests. This approach allows for efficient scaling and is a cost-effective solution for handling backend logic.
Take a look at this: Nextjs Server Actions File Upload
API routes are serverless functions that handle backend logic, created within the 'pages/api' directory. They enable you to build a robust backend directly within your Next.js app.
In Next.js, API routes are invoked when a request is made to a specific endpoint, such as /api/greet. This function responds with a JSON object containing a greeting message.
A unique perspective: Nextjs Backend
Testing and Deployment
Testing and deployment are crucial steps in the development process of a Next.js CMS project. You can ensure your code functions as expected and reduces the risk of bugs by writing unit tests for your components and pages using testing frameworks like Jest or React Testing Library.
Implementing end-to-end (E2E) tests is also essential to simulate user interactions and test the overall functionality of your CMS project. Tools like Cypress or Selenium can be used for E2E testing.
A continuous integration and continuous deployment (CI/CD) pipeline can be set up to automate the testing and deployment process, allowing for faster and more efficient development cycles.
For your interest: Next Js Cms
Consider using a hosting platform like Vercel or Netlify for deploying your Next.js CMS. These platforms provide seamless integration with Next.js and offer features like automatic scaling and SSL certificates.
To automate the deployment process, you can use a CI/CD pipeline. Here are the steps to set up a CI/CD pipeline:
- Set up a Git repository for your project.
- Configure a CI/CD tool like GitHub Actions or CircleCI to automate the testing and deployment process.
- Set up a build command, install command, and root directory in your CI/CD pipeline.
Implementing proper error tracking and monitoring is also crucial to identify and resolve any issues that arise in your deployed CMS project. Tools like Sentry or New Relic can help with error tracking and performance monitoring.
Worth a look: Nextjs Error Boundary
Frequently Asked Questions
What is NextJS built with?
Next.js is built on the latest React features, including Server Components and Actions, providing a robust foundation for modern web applications. Discover how these cutting-edge technologies enhance your development experience.
Is NextJS better than React?
NextJS is a more feature-rich and opinionated alternative to React, particularly well-suited for SEO-focused and pre-rendering applications. Whether it's better for your project depends on your specific needs and goals.
Sources
- https://learn.flexpressai.com/next.js-project-structure
- https://blog.logrocket.com/structure-scalable-next-js-project-architecture/
- https://www.dhiwise.com/post/nextjs-folder-structure-simplified-a-comprehensive-overview
- https://www.saffrontech.net/blog/how-to-structure-a-nextjs-app
- https://www.thetombomb.com/posts/nextjs-app-architecture
Featured Images: pexels.com