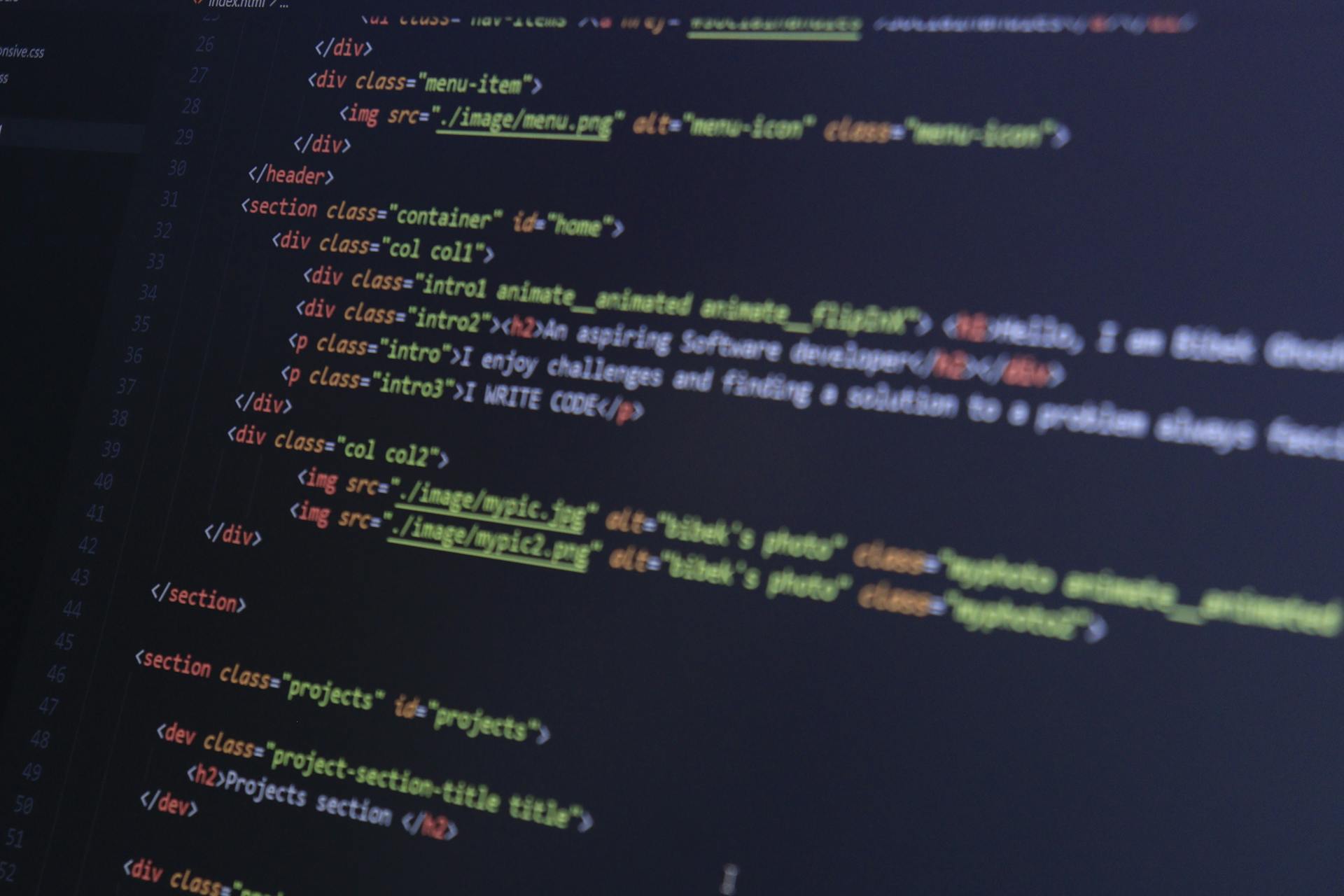
As you start building your Next.js application, it's essential to follow best practices to ensure scalability and maintainability. This means using a modular approach to code organization, separating concerns into different files and directories.
A good rule of thumb is to keep your pages and components as lightweight as possible, avoiding unnecessary complexity. This will make it easier to manage and optimize your application's performance.
For instance, using dynamic imports and lazy loading can significantly reduce the initial bundle size, making your application load faster. This is especially important for applications with a large number of pages or complex components.
By following these best practices, you'll be able to build a scalable and efficient Next.js application that meets the needs of your users.
Related reading: Nextjs Usecontext
Internationalization and Analytics
Internationalization is a breeze with Next.js, requiring less configuration to get set up. This makes it internationally recognized.
To take your analytics to the next level, Next.js provides an analytical dashboard that shows accurate visitor data and page insights out of the box. Quickly build an analytical dashboard and gain valuable insights into your visitors and page insights without extra coding or configuration.
Related reading: Nextjs Dashboard
To track your site's performance, consider using Next.js Speed Insights, which can be enabled by turning on the Web Analytics option in the Vercel Dashboard. This tool allows you to analyze and measure the performance of your application's pages using various metrics.
Some popular APM libraries for Next.js include Sentry, New Relic, and AppSignal. However, if you're looking for a more cost-effective option, consider using Alerty, which uses AI to help you monitor your application performance.
See what others are reading: Using State in Next Js
Internationalization
Internationalization is a crucial aspect of creating an enterprise application that can reach a global audience.
Creating an enterprise application can be easily translated into different languages worldwide.
Internationalization takes less configuration to get set up, making Next.js internationally recognized.
This streamlined setup process allows developers to focus on other important aspects of their application, such as user experience and functionality.
If this caught your attention, see: Creating Shop Page Next Js
Performance Optimization
Performance Optimization is a crucial aspect of Next JS best practices. By following these tips, you can significantly enhance your website's speed, reliability, and performance.
Implementing lazy loading is a great way to improve page load times. It loads images only when a user can see them, reducing the number of network queries and initial load time. This technique can be easily created using Next JS.
To optimize images, use the Next.js Image Component, which automatically optimizes and serves images in modern formats like WebP. This can significantly reduce the size of your images and improve page load times.
Excessive CSS and JavaScript files can slow down the page load, so it's essential to minimize them. Next JS supports automatic CSS and JavaScript minification, but you can take extra steps to minimize file size even further.
Reducing the size of the build bundle is also crucial for performance optimization. You can use dynamic imports via next/dynamic to lazy-load JavaScript resources, deferring loading specific components or libraries until the user performs a particular operation on the page.
To analyze the bundle produced by Next JS and get guidance on how to reduce its size, you can use tools like Webpack Bundle Analyzer, Package Phobia, Bundle Phobia, bundlejs, and Import Cost.
Optimizing page SEO performance is also vital for Next JS sites. You can use Google Lighthouse, an open-source tool built into Chrome, to check your site's SEO performance and get guidance on how to improve it.
You might enjoy: Nextjs Dynamic
Here are some key metrics to focus on:
- Largest Contentful Paint (LCP): Measures the time it takes for the main content of a page to become visible to users.
- First Input Delay (FID): Evaluates the time delay between a user’s first interaction and the browser’s response.
- Cumulative Layout Shift (CLS): Gauges the visual stability of a page by measuring the number of unexpected layout shifts that may annoy users.
By following these performance optimization tips, you can ensure that your Next JS application is fast, reliable, and efficient, providing a seamless user experience and boosting sales.
Project Setup and Structure
To set up your Next.js project, you'll want to start with a solid understanding of the basics. A basic understanding of the DOM, language syntax, and ES6+ features are essential to get started.
To create a new Next.js project, consider using the create-next-app command, which sets up a basic project structure and installs the required dependencies. This will save you time and ensure you're off to a good start.
A well-organized project structure is crucial for smooth development. Next.js uses a file-based routing system, where each page is represented by a separate file in the pages directory. Make sure to organize your pages and components in a logical manner to maintain a clean project structure.
Here are the key points to keep in mind when setting up your Next.js project structure:
Zero Config
Next.js takes the hassle out of project setup with its Zero Config feature. This means you can start building your application right away without worrying about complicated configurations.
With Zero Config, Next.js compiles and builds automatically, allowing you to see the results of your work in real-time with hot refresh. This saves you time and effort that would otherwise be spent on manual configuration.
Next.js automatically scales and optimizes your production application with hot refresh, giving you a seamless development experience.
For your interest: Nextjs Spa
Setting Up Project Structure
Setting up a Next.js project structure is crucial for smooth development. Consider using the create-next-app command to create a new Next.js project, which sets up a basic project structure and installs the required dependencies.
To maintain a clean project structure, organize your pages and components in a logical manner. This includes separating each page into a separate file in the pages directory, making it easy to find and update specific pages.
Curious to learn more? Check out: Next Js App vs Pages
A file-based routing system is used in Next.js, which simplifies page navigation. To set up environment variables, create a .env file to store sensitive information or configuration settings separately from your code.
Here are some key considerations to keep in mind when setting up your project structure:
Testing and Deployment
Testing and deployment are crucial steps in the development process of a Next.js CMS project. Writing unit tests for your components and pages using testing frameworks like Jest or React Testing Library ensures that your code functions as expected and reduces the risk of bugs.
Implementing end-to-end (E2E) tests simulates user interactions and tests the overall functionality of your CMS project. Tools like Cypress or Selenium can be used for E2E testing.
Setting up a continuous integration and continuous deployment (CI/CD) pipeline automates the testing and deployment process, allowing for faster and more efficient development cycles.
Here are some popular hosting platforms for deploying your Next.js CMS: Vercel, Netlify, and others that provide seamless integration with Next.js and offer features like automatic scaling and SSL certificates.
Implementing proper error tracking and monitoring helps identify and resolve any issues that arise in your deployed CMS project. Tools like Sentry or New Relic can help with error tracking and performance monitoring.
A unique perspective: Next Js Development Company
Best Practices
To ensure your Next.js project runs smoothly, consider the following best practices. Implementing them can make a big difference in performance and maintainability.
Use functional components instead of class components, as they are simpler and perform better. Functional components are the way to go, especially in Next.js projects.
To optimize rendering performance, use memoization techniques like React.memo or useMemo. This can help prevent unnecessary re-renders and improve overall performance. By doing so, you'll notice a significant improvement in your project's speed.
Here are some key takeaways to keep in mind:
Proper error handling is also crucial. Use try-catch blocks or error boundaries to catch and handle errors gracefully. This will improve the user experience and make your project more reliable.
Readers also liked: Nextjs Error Boundary
15 Practices for Clean and Responsive Applications
Optimizing CSS is crucial for faster page loads, and you can achieve this by reducing HTTP requests, deleting unneeded styles, and optimizing selectors. This can be done using tools like PurifyCSS.
Minimizing CSS and JavaScript files is also essential, as excessive files can slow down the page load. Next.js supports automatic CSS and JavaScript minification, but you can take extra steps to minimize file size using CSS Modules or Styled Components for scoped styling.
To reduce JavaScript main thread work, use concurrent rendering to optimize JavaScript execution. This can be achieved using Next.js, which enables concurrent rendering.
Reducing the size of the build bundle is also vital, and you can achieve this by using dynamic imports to lazy-load JavaScript resources. This can be done using the next/dynamic feature in Next.js.
To analyze the bundle produced by Next.js, you can use tools like Webpack Bundle Analyzer, Package Phobia, Bundle Phobia, bundlejs, and Import Cost.
Optimizing page SEO performance is crucial, and you can achieve this by using Google Lighthouse to check your site's SEO performance. This tool can provide guidance and best practices for improving site performance, accessibility, and SEO.
Minimizing third-party dependencies is also essential, as they can increase the bundle size and affect performance. Opt for lightweight alternatives or write custom solutions to reduce dependencies.
Lazy loading can enhance website performance by lowering page load times and improving user experience. This can be achieved using Next JS, which allows you to create lazy-loaded components.
Broaden your view: Nextjs Seo
Properly organizing code within a component is essential for readability and maintainability. A well-structured component typically follows a consistent order: imports, type definitions, component definition, hooks, event handlers, and render logic.
Consistent naming conventions are crucial for enhancing code readability and maintainability. For component names, use PascalCase, and for props and methods, use camelCase. For custom style classes, use snake_case.
Here are some tools you can use to remove unused dependencies:
- Datadog NextJS
- Posthog NextJS
- NextJS Prometheus
- Newrelic NextJS
- Front End Monitoring Tools
Optimize Web Fonts
Fonts can significantly affect load times, especially if you're using multiple font families or weights.
Preloading and optimizing fonts reduces the delay in text appearing on the page, resulting in faster font rendering.
Properly loading fonts prevents text from shifting after rendering, reducing layout shifts.
To optimize Google Fonts in your Next.js project, use the next/font feature.
next/font optimizes local fonts, eliminating external network requests for enhanced privacy and performance.
Starting from Next.js 13, it supports self-hosting any Google Font to avoid fetching font files from Google.
You can also use lazy loading for images to enhance website performance, but that's a topic for another section.
Curious to learn more? Check out: Next Js Loader
Setting Up a Debugger
Setting up a debugger is crucial to track down bugs in your Next.js applications on Vercel. You can use the Next.js debugger to debug your applications.
To set up the Next.js debugger, you need to use Vercel. Vercel is a platform that allows you to deploy and manage your Next.js applications.
The Next.js debugger can be set up to track down bugs in your Next.js applications on Vercel. This is especially useful if you're struggling to find bugs in your applications.
You can find more information on how to set up the Next.js debugger to debug your applications on Vercel in the article "How To Set Up Nextjs Debugger To Debug Your Applications".
Broaden your view: How to Debug Next Js in Vscode
Analyzing Reddit Thread: CMS Recommendation
The user in the Reddit thread is looking for a Content Management System (CMS) that integrates well with NEXT.js and offers GraphQL functionality.
They have experience with simpler projects using React, NEXT.js, and Firebase, but are now seeking a more robust solution for complex content management needs.
Related reading: Next Js State Management
A headless CMS approach might be a good fit, where the CMS focuses on content management and interacts with the frontend (NEXT.js) via an API, with GraphQL as the chosen protocol.
Firebase, while offering some CMS functionalities, might not be the most suitable option for this scenario.
Here are some key points to consider when evaluating a CMS for your NEXT.js project:
In this scenario, a CMS that offers a headless approach and GraphQL support would be a good fit, allowing for efficient data management and integration with NEXT.js.
Sources
- https://alerty.ai/blog/next-js-best-practices
- https://learn.flexpressai.com/next.js-project-structure
- https://reacttonext.hashnode.dev/optimizing-nextjs-applications-for-performance-tips-and-best-practices
- https://www.getfishtank.com/insights/best-practices-for-nextjs-and-typescript-component-organization
- https://www.codemotion.com/magazine/frontend/optimize-next-js-for-production/
Featured Images: pexels.com