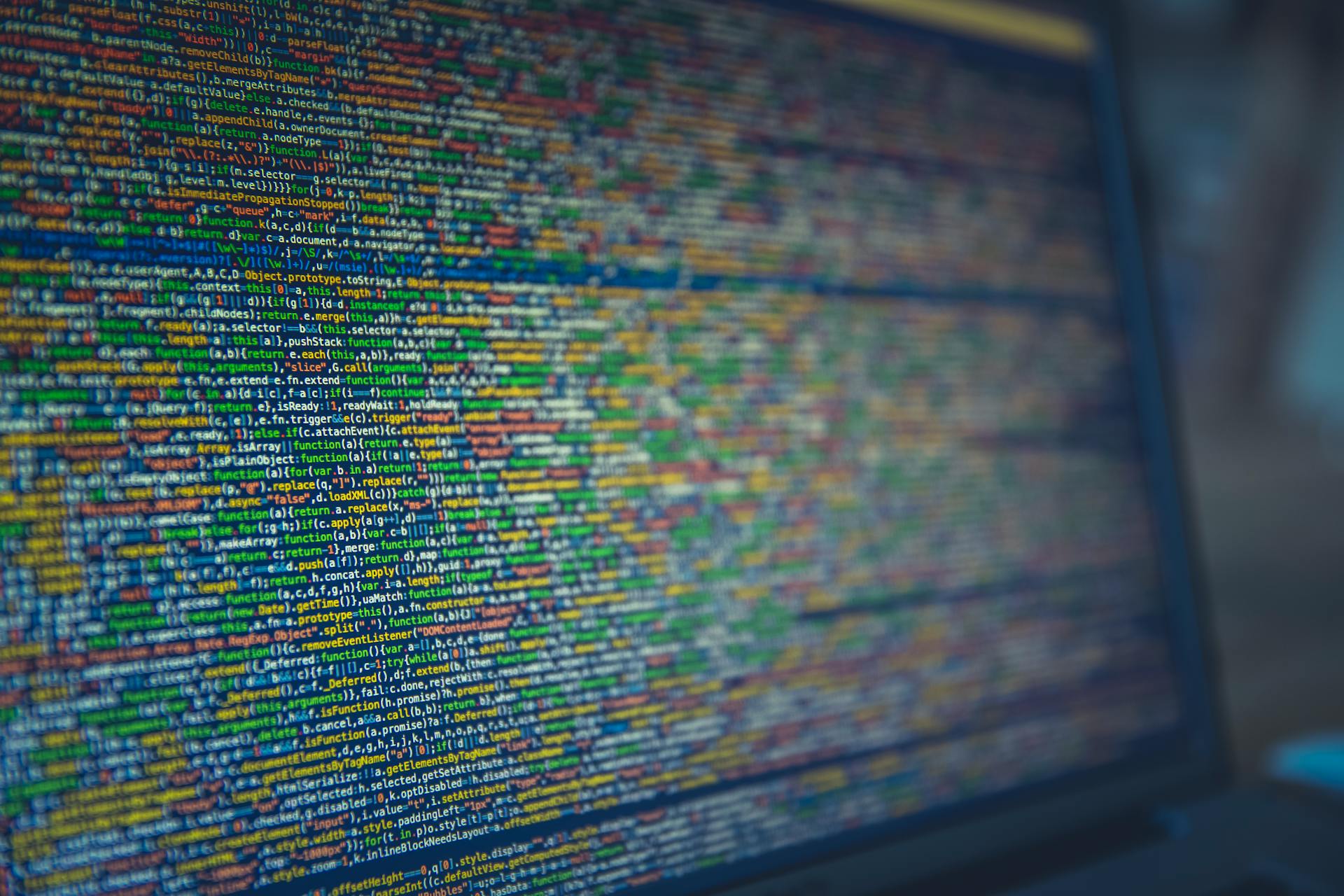
Building high-quality apps with Apollo Client and Next.js requires a solid understanding of how they work together. Apollo Client is a popular state management library that helps you fetch and manage data from APIs, while Next.js is a React-based framework for building server-rendered and statically generated applications.
To get started with Apollo Client and Next.js, you need to install the necessary packages, including @apollo/client and next-apollo-client. This will allow you to use Apollo Client's features, such as caching and pagination, within your Next.js application.
With Apollo Client, you can easily manage data fetching and caching, making it easier to build fast and scalable applications. By integrating Apollo Client with Next.js, you can take advantage of Next.js's server-rendering and static site generation features to create highly optimized applications.
Here's an interesting read: Next Js Client Side Rendering
Getting Started
Getting Started with Apollo Client in NEXT.js is a breeze. To integrate Apollo Client into a NEXT.js project, you'll need to import the necessary components and hooks in a component.
Check this out: Next Js Client Portal
First, you'll need to import the necessary components and hooks. This is done by importing the components and hooks in a component.
Apollo Client is a powerful and flexible GraphQL client that simplifies working with GraphQL in JavaScript applications. It's a must-have for any NEXT.js project that involves GraphQL.
To get started, you'll need to follow a few simple steps. Import the necessary components and hooks in a component, and you're on your way to leveraging the full power of Apollo Client.
Consider reading: Next Js Components
Setting Up in an App
To set up Apollo Client in your Next.js app, start by installing the necessary packages using npm install @apollo/client @apollo/experimental-nextjs-app-support. This will give you access to the Apollo Client and GraphQL dependencies required for making GraphQL requests and managing data.
Create a new file called apollo-client.js in the root of your project and add the following code to set up the client. You can also create a custom Apollo Client setup using the experimental support, which includes features like SSR and caching mechanisms.
Expand your knowledge: Using State in Next Js
To make the Apollo Client instance available to all components in your Next.js application, wrap your root component with the ApolloProvider component from @apollo/client. This sets up the Apollo Client and makes it available to all components in your NEXT.js application.
Here's a step-by-step guide to setting up Apollo Client in your Next.js app:
1. Create a new file called apollo-client.js in the root of your project.
2. Install the necessary packages using npm install @apollo/client @apollo/experimental-nextjs-app-support.
3. Create a custom Apollo Client setup using the experimental support.
4. Wrap your root component with the ApolloProvider component from @apollo/client.
By following these steps, you'll be able to set up Apollo Client in your Next.js app and start making GraphQL requests and managing data.
Consider reading: Install Next Js
Fetching and Caching
Fetching and caching are crucial aspects of building a Next.js application with Apollo Client. You can use Apollo Client to fetch data from a GraphQL server, and Next.js patches all fetch requests, including those made with Apollo Client.
If this caught your attention, see: Next Js Fetch Data save in Context and Next Route
In Server Components, you can use the fetch API to fetch data, but it's a bit verbose. You can replace the fetch call with Apollo Client, which makes it easier to use the client directly in your components.
One thing to note is that in Server Components, you're not allowed to use any hook, including ApolloProvider and useQuery. However, you can still use the client directly in your components by installing the experimental library for Apollo Client.
This library makes it easier to use Apollo Client in Server Components and Client Components when using Next.js 13. You can use the library to get a function that allows you to get the Apollo Client every time you need it.
To configure the Apollo cache, you can customize it to suit your needs. For example, you can define type policies and custom merge functions to handle specific fields differently.
Here are some strategies to prevent duplicate requests and reduce response latency:
- Use the cache-and-network fetch policy, which allows the application to use cached data while simultaneously fetching fresh data from the network.
- Enable data normalization, define custom cache resolvers, or specify cache eviction policies.
By using Apollo Client's caching mechanism, you can improve the performance of your application. Here are some ways to optimize caching in your Next.js application:
Server Side Rendering
Server Side Rendering is a powerful feature in Next.js that allows you to fetch data on the server before sending the HTML to the client. This can improve the initial load time and is beneficial for SEO.
To implement Server Side Rendering with Apollo Client, you can use the getServerSideProps method provided by Next.js. This function will be used during each page request to get any data passed into the page component as props.
You can use Apollo Client to handle SSR by creating an instance of the client and configuring it to handle SSR. This can be done by setting the ssrMode option to true when creating the client.
Here's an example of how to create an Apollo Client instance for SSR:
```javascript
import { ApolloClient, InMemoryCache } from '@apollo/client';
const client = new ApolloClient({
ssrMode: true,
uri: 'https://your-graphql-api.com/graphql',
cache: new InMemoryCache(),
});
```
By using getServerSideProps and Apollo Client, you can fetch data on the server and pass it to the client to hydrate the Apollo Client cache.
A unique perspective: Getserversideprops Nextjs
Here's a comparison of the different data fetching methods in Next.js:
Note that getServerSideProps is the recommended method for data fetching in Next.js, especially when using Apollo Client.
Advanced Usage
In Apollo Client Next.js, you can use the `useQuery` hook to fetch data from a GraphQL API. This hook is a powerful tool for fetching data in a Next.js application.
To use `useQuery` effectively, you need to understand how to create a query, which involves defining the query string and the variables it needs. For example, in the article, we saw how to create a query for fetching a list of users using the `query` function.
By using the `useQuery` hook, you can fetch data in a flexible and efficient way, allowing you to handle errors and loading states with ease.
Consider reading: How to Use Context Api in Next Js 14
Advanced Usage
To get the most out of advanced usage, you need to master the art of combining different features.
One of the most powerful features is the ability to nest functions, allowing you to create complex expressions that can be used in a variety of contexts.
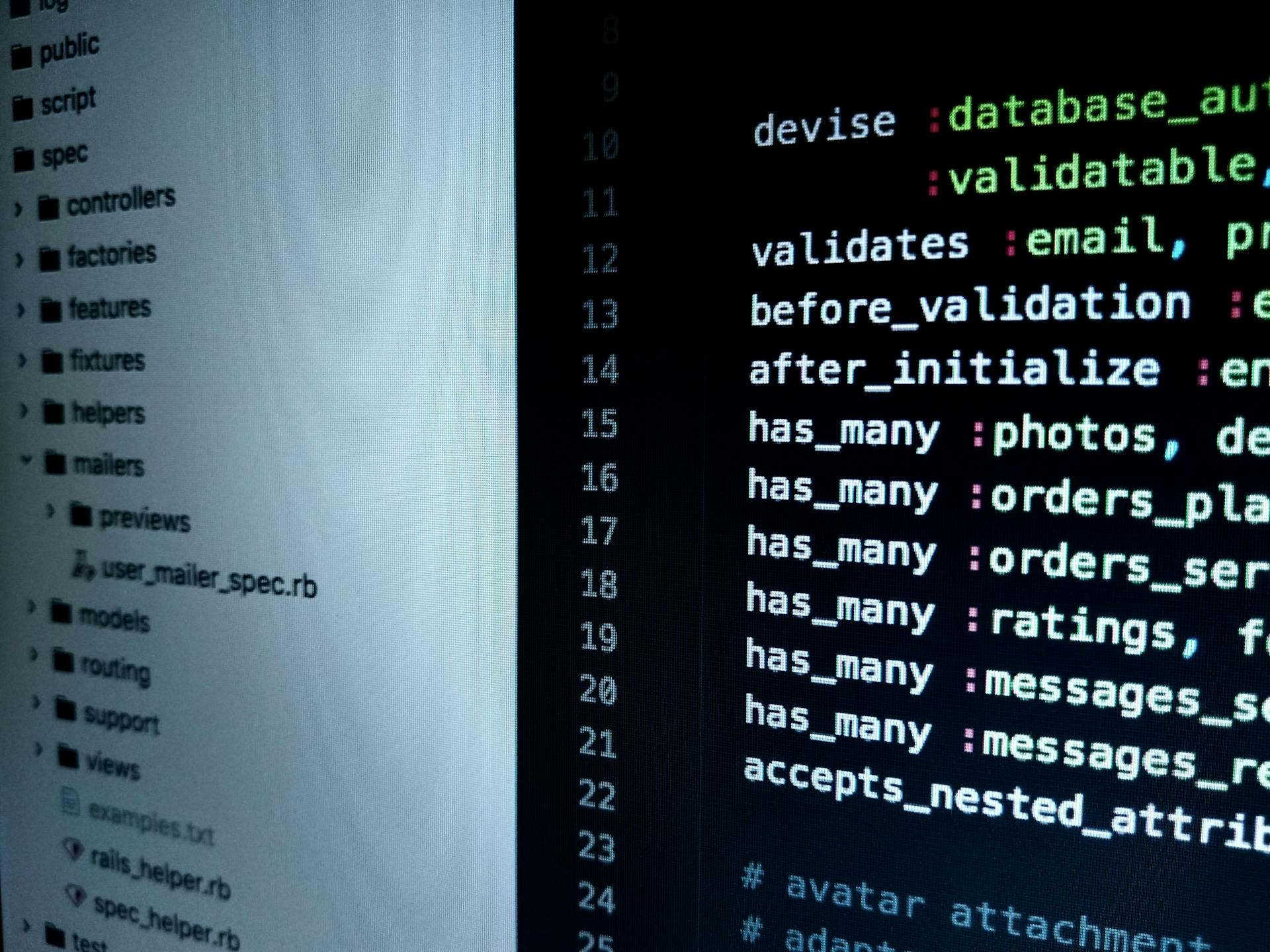
As we saw in the section on "Mastering Functions", this can be incredibly useful for tasks like data analysis and scientific modeling.
By combining functions with conditional statements, you can create robust and efficient code that can handle a wide range of inputs.
For example, using the "if-else" statement, you can create a function that returns a different value based on the input.
This can be especially useful when working with data that has different formats or structures.
In the section on "Working with Data", we saw how to use conditional statements to filter and manipulate data.
By combining these techniques, you can create advanced data analysis tools that can help you gain valuable insights from your data.
To take your advanced usage to the next level, be sure to experiment with different combinations of features and techniques.
This will help you develop a deeper understanding of how they work together and how to use them to solve complex problems.
On a similar theme: Tutorial Building Useful Nextjs Tool
Experimental App Support
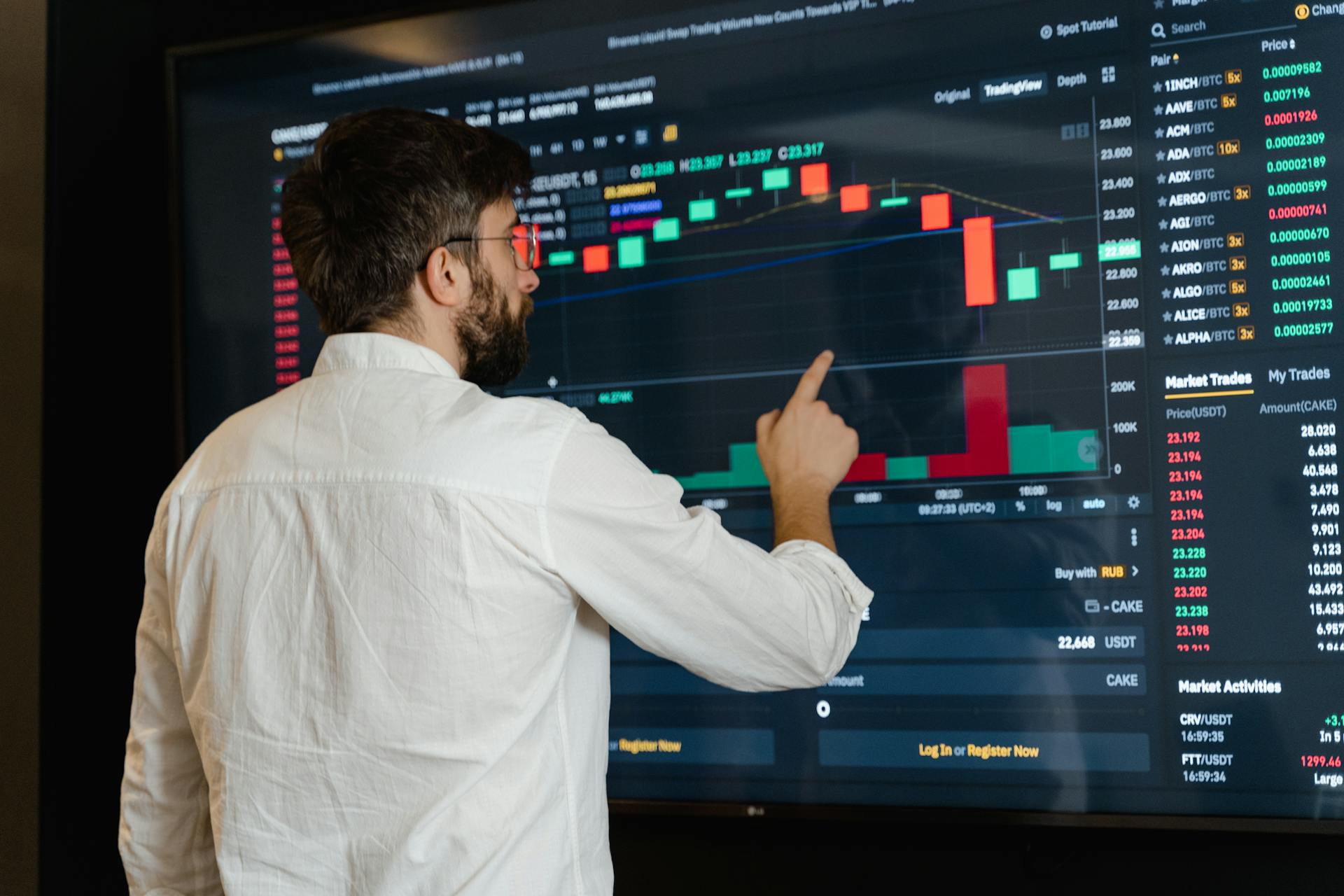
Experimental App Support is a game-changer for Next.js developers. It introduces powerful features that integrate seamlessly with Next.js' advanced rendering capabilities.
To get started, you'll need to install the @apollo/experimental-nextjs-app-support package. This package provides tools and hooks specifically designed for Next.js applications.
This setup ensures that Apollo Client integrates smoothly with Next.js, leveraging SSR and caching mechanisms. The result is a developer experience and performance that's hard to beat.
Here's a quick rundown of the installation process:
- Installation: Install the @apollo/experimental-nextjs-app-support package.
With experimental support in place, you can create a custom Apollo Client setup that's tailored to your Next.js application. This is where the magic happens, and you can start leveraging the full potential of Apollo Client and Next.js.
Custom Components and Fetching
Custom components in Next.js can leverage Apollo Client for sophisticated client-side data fetching techniques. One approach is to create reusable custom hooks for fetching data, which can be used in various components throughout your application.
To create a custom hook, you can use the `useQuery` hook from Apollo Client. This hook allows you to fetch data from your API and handle loading and error states.
Here are some best practices for custom components and client-side data fetching techniques:
- Use custom hooks to fetch data in a reusable way.
- Implement optimistic UI updates to improve user experience by updating the UI before the server confirms the change.
By following these best practices, you can create efficient and effective custom components that leverage Apollo Client for client-side data fetching.
Handling Authentication
Handling authentication is crucial for any NEXT.js application, and Apollo Client provides the necessary tools to integrate with your chosen authentication mechanism.
You can use techniques such as setting authentication headers, persisting tokens in local storage, or using authentication libraries like next-auth to handle authentication.
Implementing authentication is specific to your application, but Apollo Client gives you the flexibility to choose the method that best suits your needs.
For instance, you can use next-auth to simplify the authentication process and focus on other aspects of your application.
Remember, Apollo Client provides the necessary tools to integrate with your chosen authentication mechanism, so you can focus on building a robust and secure application.
Error Handling and States
Error handling and states are crucial in Apollo Client Next.js, and thankfully, it handles loading and error states for you. This means you can focus on building your application without worrying about the underlying mechanics.
The useQuery and useMutation hooks return loading and error states that you can use to display loading spinners or error messages in your components.
Benefits and Optimization
Integrating Apollo Client with Next.js offers several benefits, including enhanced performance by leveraging Next.js's SSR and SSG capabilities.
By using client-side components in Next.js, you can create a responsive and dynamic user experience with Apollo Client's powerful tools for managing client-side data, such as caching, optimistic UI updates, and error handling.
Apollo Client's integration with Next.js streamlines the development process, making it easier to debug and optimize GraphQL queries with features like the Apollo DevTools.
Here are some ways to optimize caching in your NEXT.js application:
- Caching and performance optimization are key benefits of using Apollo Client.
- Apollo Client provides a built-in caching mechanism that improves application performance.
- You can customize the Apollo cache to suit your needs by defining type policies and custom merge functions.
This approach allows for quick retrieval and updating of data, enhancing performance and responsiveness in your application.
Benefits of Integrating
Integrating Apollo Client with Next.js offers several benefits, but one of the most significant advantages is enhanced performance. By leveraging Next.js’s SSR and SSG capabilities, you can fetch data on the server side and deliver fully rendered HTML to the client, reducing the time to first meaningful paint.
This results in a better overall performance of your application. You can see this in action by using Next.js’s built-in features, such as server-side rendering and static site generation.
SEO optimization is another key benefit of integrating Apollo Client with Next.js. Server-side rendering and static site generation ensure that search engines can easily crawl and index your pages, leading to better SEO performance.
Apollo Client helps you manage and query data efficiently, ensuring your content is always up-to-date and optimized for search engines. This is especially important for websites that rely heavily on search engine traffic.
Apollo Client provides powerful tools for managing client-side data, such as caching, optimistic UI updates, and error handling. By using client-side components in Next.js, you can create a responsive and dynamic user experience.
Consider reading: Next Js Seo
Here are some of the key features of Apollo Client that make it ideal for client-side data management:
- Caching: Apollo Client can cache data on the client-side, reducing the number of requests made to the server.
- Optimistic UI updates: Apollo Client can update the UI immediately, without waiting for the server to respond.
- Error handling: Apollo Client can handle errors on the client-side, providing a better user experience.
Finally, integrating Apollo Client with Next.js streamlines the development process. With features like the Apollo DevTools, you can debug and optimize your GraphQL queries with ease, leading to a more efficient development workflow.
Caching and Optimization
Integrating Apollo Client with Next.js offers enhanced performance by leveraging Next.js's SSR and SSG capabilities, which reduces the time to first meaningful paint and improves overall performance.
Apollo Client provides a built-in caching mechanism that improves performance by reducing redundant network requests. By default, Apollo Client uses an in-memory cache to store fetched data.
To configure the Apollo cache, you can customize it to suit your needs, such as defining type policies and custom merge functions to handle specific fields differently. This ensures data-fetching operations are optimized and managed effectively.
Apollo Client's caching mechanism plays a crucial role in managing data efficiently. The InMemoryCache is the default cache implementation for Apollo Client, which stores your GraphQL data in a normalized, in-memory, JSON object.
Here are some ways to optimize caching in your NEXT.js application:
- Customize the cache configuration to suit your application's needs, such as enabling data normalization or defining custom cache resolvers.
- Use the Apollo DevTools to debug and optimize your GraphQL queries with ease.
- Update your client to avoid any caching on the server side, especially if you're using Vercel.
By optimizing caching and using Apollo Client's powerful tools for managing client-side data, you can create a responsive and dynamic user experience that improves performance and scalability.
Sources
- https://www.apollographql.com/blog/next-js-getting-started
- https://www.apollographql.com/blog/how-to-use-apollo-client-with-next-js-13
- https://www.dhiwise.com/post/a-comprehensive-guide-to-nextjs-apollo-client-integration
- https://dev.to/andisiambuku/how-to-integrate-graphql-in-next-js-using-apollo-client-240p
- https://clouddevs.com/next/graphql/
Featured Images: pexels.com