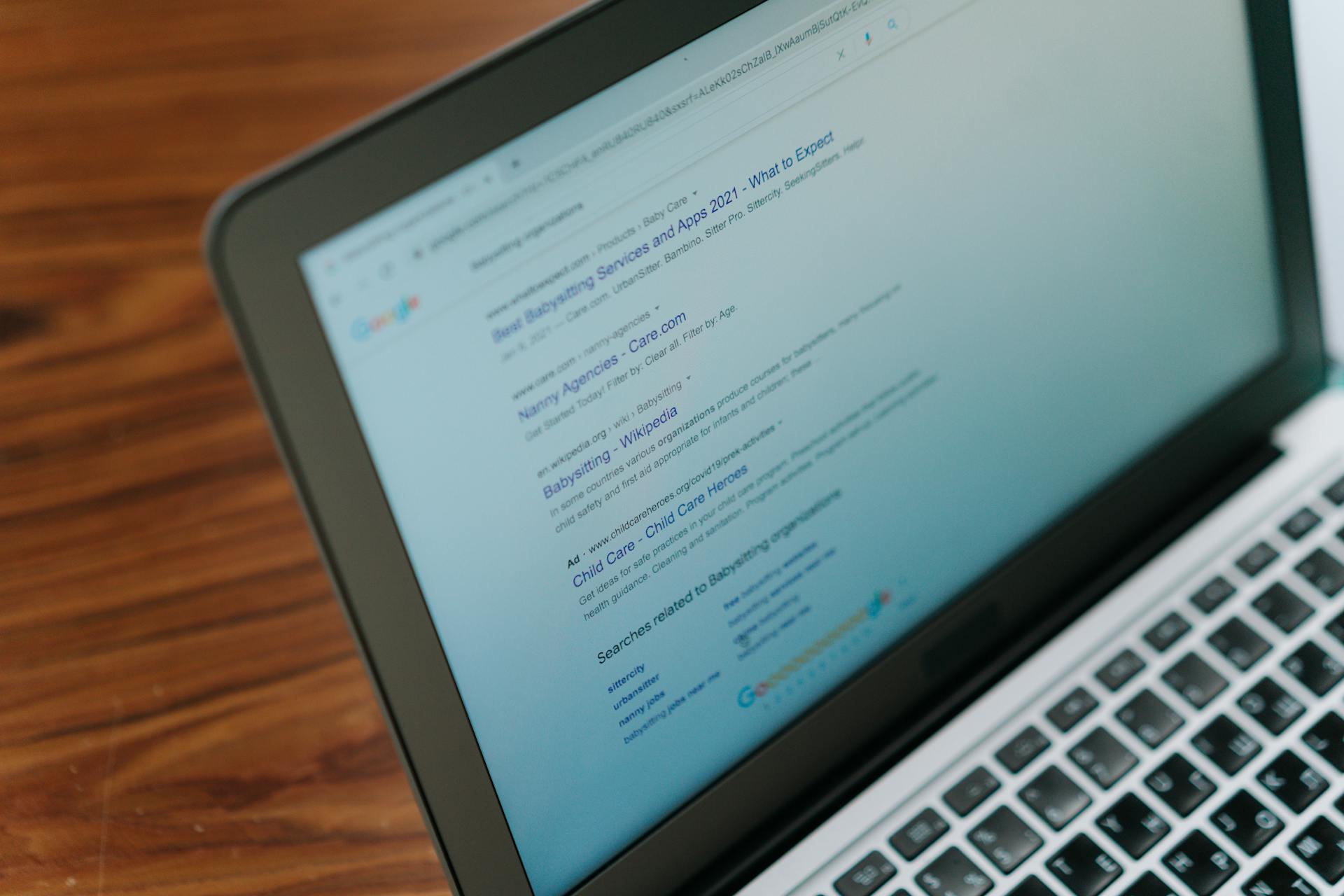
Enabling client-side caching in Next.js is a straightforward process that can significantly improve page load times. By default, Next.js uses a caching mechanism called "Automatic Static Optimization" (ASO) to cache pages.
To enable client-side caching, you need to configure the Next.js cache using the `next/config` API. This involves setting up a cache store, such as Redis or Memcached, and configuring caching rules for specific pages or routes.
Client-side caching can be configured to cache pages based on their route, query parameters, or even user-specific data. This allows you to fine-tune caching to suit your application's needs.
By configuring client-side caching effectively, you can improve the performance and scalability of your Next.js application.
See what others are reading: Speed up a Next Js Application Fetching Data
What is Client-Side Caching?
Client-side caching is a technique used to store frequently accessed data in a user's browser, reducing the need for repeated requests to a server. This can improve page load times and provide a better user experience.
Service workers provide more control over how you cache, allowing you to cache entire pages' assets at once using the clone() method from the service worker's fetch event handler.
Local storage, on the other hand, stores key-value pairs of data in the user's browser using the web storage API localStorage, which remains even when the browser window is closed. This can be used to store user preferences, partially completed forms, and authentication tokens.
Here are some ways to implement client-side caching in Next.js:
- Service workers for granular caching
- Local storage for smaller cached data
How it Works
Client-side caching is a technique that stores frequently accessed data or assets locally on a user's device. This is done to reduce the amount of time it takes to load a webpage or application.
By storing data locally, the user's device can quickly retrieve it from the cache instead of having to request it from the server. This results in faster page loads and a better user experience.
The cache is typically stored in the browser's memory, which is known as the browser cache. This cache is separate from the server-side cache, which is managed by the web server.
The browser cache can store a variety of data types, including images, stylesheets, scripts, and even entire web pages. This allows the browser to quickly retrieve these assets and render them on the page.
Client-side caching can be enabled or disabled by the user, depending on their browser settings. This means that users have control over how their device stores and retrieves data.
Benefits and Use Cases
Client-side caching is a game-changer for improving website performance. By storing frequently-used resources locally on a user's device, it reduces the need for repeated requests to the server, resulting in faster page loads and a better user experience.
Caching can help reduce server load, which is especially important for e-commerce websites with high traffic during peak shopping seasons. This can lead to significant cost savings on infrastructure and maintenance.
A well-implemented caching strategy can also improve search engine rankings by reducing page load times and increasing user engagement. This is because search engines like Google prioritize websites that provide a fast and seamless user experience.
Discover more: Next Js Image Search
Client-side caching is particularly useful for websites with dynamic content, such as news articles or social media feeds, where the content is updated frequently. By caching the latest updates, users can access the most up-to-date information without having to reload the entire page.
For example, a news website can cache the latest headlines and articles, allowing users to quickly access the most recent information without having to wait for the entire page to load. This can be a huge advantage for users who are interested in staying up-to-date with the latest news and developments.
Enabling Client-Side Caching in Next.js
To enable client-side caching in Next.js, you have two primary approaches: Service Workers for Granular Caching and Local Storage for Smaller Cached Data. Service workers provide more control over how you cache, allowing you to cache specific resources.
You can register a service worker in _app.js to enable application-wide caching. This ensures the service worker has access to all resources in your Next.js project, including pages and assets. To do this, add the registration code in the function.
To accomplish efficient client-side caching for the current user's page, you use the clone() method from the service worker's fetch event handler. This caches the entire page's assets at once.
Here's a comparison of the two approaches:
Configuration Options
Client-side caching in Next.js can be configured in various ways to suit your needs.
You can enable client-side caching by setting the `revalidate` option to `false` in the `use ` and `getStaticProps` functions. This will allow the cache to be updated on the client-side.
The `revalidate` option is used to determine how often the cache should be updated. If set to `false`, the cache will only be updated when the page is reloaded.
To configure the cache to update on every request, you can set the `revalidate` option to `true`. This is useful for pages that frequently change.
The `cache` option can be used to specify a custom cache implementation. This allows you to use a different caching strategy than the default one provided by Next.js.
A unique perspective: Next Js Revalidate
By using a custom cache implementation, you can optimize the caching behavior for your specific use case.
The `cache` option can be used in combination with other caching options, such as `revalidate` and `fallback`, to create a custom caching strategy.
The `fallback` option can be used to specify a fallback behavior when the cache is not available. This can be useful when dealing with pages that are not cached or when the cache is outdated.
By setting the `fallback` option to `true`, you can specify a fallback behavior that will be used when the cache is not available.
Best Practices
To enable client-side caching in Next.js, you should use the `getStaticProps` method to generate static HTML files for your pages. This method allows you to pre-render pages at build time, which can then be cached by the browser.
Make sure to use the `revalidate` option to specify how often the page should be revalidated. For example, if you set `revalidate` to 10, the page will be revalidated every 10 minutes.
Using `getStaticProps` can improve performance by reducing the number of requests made to your server. In fact, Next.js recommends using this method for pages that don't require server-side rendering.
Take a look at this: Why Next Js
Clearing the Cache in Next.js
To clear the cache in Next.js, you can use the `next build` command followed by the `--clean-cache` flag.
This will delete the cache files generated by Next.js.
You can also use the `next clean` command to clear the cache.
If this caught your attention, see: Clear Onedrive Cache
Manual Clearing
Manual clearing is a more traditional approach to clearing the cache in Next.js, but it's still a viable option.
You can use the `next build` command to clear the cache manually, but be aware that this will delete all cached files.
This method is useful when you need to delete the cache for a specific page or component, as it allows you to target specific files.
For example, you can use `next build public/my-page.js` to delete the cache for the `my-page.js` file.
However, be aware that this method can be time-consuming if you have a large project, as you'll need to manually clear the cache for each file.
It's also worth noting that manual clearing can be less efficient than other methods, as it requires more manual effort and can be prone to human error.
You might enjoy: Next Js Clear Image Cache
Automated Clearing
Automated Clearing helps Next.js applications by automatically clearing the cache when the app's code or data changes. This feature is enabled by default in Next.js 13.
The cache is cleared when the app's code changes, which can be triggered by updating the `pages` directory. This ensures that the app is always serving the latest version of the code.
Automated Clearing uses a mechanism called "route revalidation" to check if the cache is stale. If the cache is stale, it is automatically cleared and rebuilt. This process happens in the background, without requiring any manual intervention.
Route revalidation is triggered by changes to the `getStaticProps` or `getServerSideProps` functions in the `pages` directory. These functions are responsible for generating static props for pages, and changes to them can cause the cache to become stale.
Take a look at this: Nextjs Route
Sources
- https://awstip.com/nextjs-aws-amplify-cloudfront-cache-it-real-good-1211466cecfd
- https://supabase.com/blog/fetching-and-caching-supabase-data-in-next-js-server-components
- https://nextjs.org/docs/app/building-your-application/data-fetching/incremental-static-regeneration
- https://swr.vercel.app/docs/mutation
- https://semaphoreci.com/blog/cache-optimization-nextjs
Featured Images: pexels.com