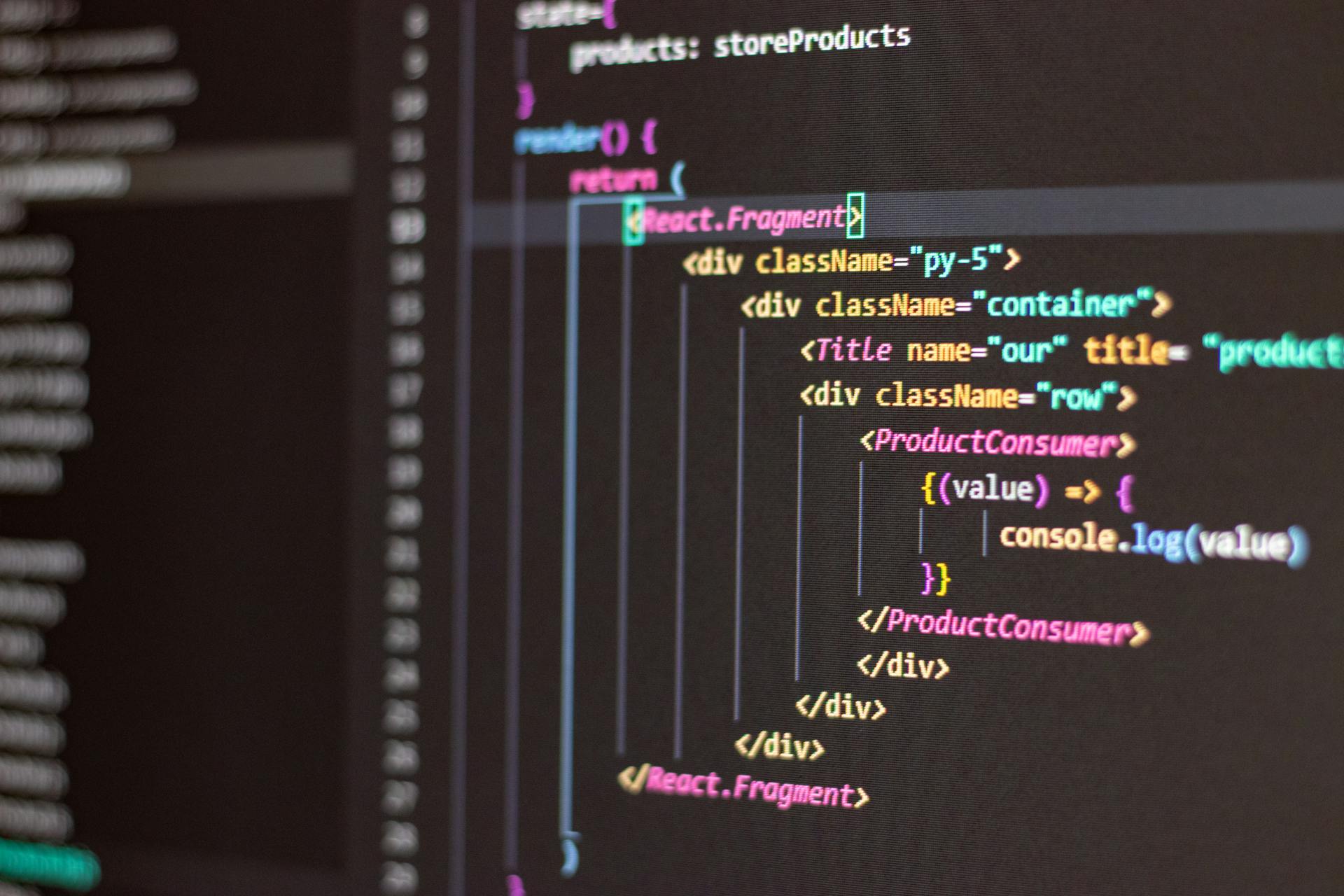
Optimizing image search performance in Next.js is crucial for a seamless user experience. By implementing image search techniques, you can significantly improve load times and user engagement.
One key technique is lazy loading images, which loads images only when they come into view. This is particularly useful for large image collections, where loading all images at once can be slow and resource-intensive.
Lazy loading images can be achieved through the use of libraries like Next-Image or react-lazy-load. These libraries provide a simple and efficient way to implement lazy loading, making it easier to optimize image search performance.
Proper image compression is also essential for better performance. By compressing images without sacrificing quality, you can reduce the file size and improve load times.
A different take: Nextjs Performance
Next.js Techniques
Next.js provides enhanced image optimization, which means images will load faster, even with poor internet connections. This is especially important for image search, where speed and accessibility are crucial.
The alt attribute is now required in Next.js, enforcing accessibility best practices for images. This is a great step forward for image search, as it ensures that images are properly described and can be understood by users with visual impairments.
Next.js ships less client-side JavaScript, which helps reduce layout shifts and provides faster loading. This results in a better user experience, especially for image-heavy pages like those found in image search.
Next.js 14 Improvements
Next.js 14 has made significant improvements that will enhance your development experience. It provides enhanced image optimization, meaning images will load faster, even with poor internet connections.
This is a game-changer for users with slow internet, and it's something I've personally noticed when working with Next.js. The alt attribute is now required, which enforces accessibility best practices for images.
This means you'll need to include an alt attribute in your image tags, but it's a small price to pay for better accessibility. Next.js 14 ships less client-side JavaScript, which helps reduce layout shifts and provides faster loading.
This is a key improvement, as it can significantly impact the user experience. Next.js 14 supports native browser lazy loading, which means the component is much faster because native lazy loading doesn’t require hydration, leading to better performance.
This is a major advantage over traditional lazy loading methods, and it's something you can take advantage of in your Next.js 14 projects.
Priority
The priority prop is a game-changer for images visible above the fold. This is because it tells the browser to preload the image as a high priority, giving you a performance boost.
Images on landing pages, for example, should use the priority prop to ensure they load quickly. Lazy loading is automatically disabled for images using priority.
To use the priority prop, you simply need to add a Boolean value, which defaults to false.
Image Optimization
Image optimization is crucial for next js image search as it directly impacts the user experience and website performance. Images account for almost 75% of a webpage's weight, making them a major culprit when it comes to size.
Data from Web Almanac 2019 shows that images are the number one contributor to a page's weight, whether on desktop or mobile. To eliminate the negative impact, we must ensure our images are properly optimized for peak web performance.
The image optimization process involves compressing and reducing the size of image files, resizing images to the appropriate dimensions, and choosing next-gen image formats that provide optimal performance, such as WebP and AVIF. WebP is a modern image format that provides superior lossy and lossless image compression for web images without compromising quality.
Using modern formats like WebP and AVIF preserves fidelity and quality while remaining performant. Next.js automatically serves images in these formats, ensuring only the most optimized and lightweight version reaches the client.
To prepare images for optimization, consider using a Content Delivery Network (CDN) such as Cloudinary to host your images. CDNs provide automatic caching, file compression, and image resizing on the fly, which can greatly benefit image performance.
Here are some key considerations for image optimization:
- Choose the right image format: WebP is highly recommended due to its many advantages and performance benefits.
- Compress images: Keep your images below 1 Mb and use tools like TinyPNG or Compressor.io for image compression.
- Serve the right images for the right device sizes: Use responsive sizing and the Responsive Breakpoints Generator tool to generate multiple image file sizes for different screen sizes.
Optimized images lead to fast-loading websites, meaning users get the best experience possible. Next.js provides enhanced image optimization, which means images will load faster, even with poor internet connections.
Image Loading
Next.js makes image loading a breeze, and it's optimized for performance. It automatically serves images in modern formats like WebP and AVIF, ensuring only the most optimized and lightweight version reaches the client.
This approach preserves fidelity and quality while keeping things performant. In fact, Next.js can even convert images from JPG to WebP, reducing size and improving loading times.
For more control over image loading, Next.js provides a built-in Image Optimization API. If needed, you can create a custom loader function to serve images from your preferred CDN, like Cloudinary, Imgix, or Cloudflare.
To set up a custom loader, configure next.config.js with the necessary settings. This will allow you to dynamically transform and optimize images using Cloudinary's powerful features.
With Next Cloudinary, you can optimize images by delivering the most modern format for the browser. This is especially useful when fetching and displaying raw images from Cloudinary, which might be too large.
Properties of
The next/image component has sixteen properties, but let's focus on the most important ones for image search in Next.js.
One property is the ability to dynamically transform and optimize images with Cloudinary, which can be used to deliver the most modern format for the browser.
Setting the width and height attributes for remote images ensures optimal performance and prevents layout shifts as the images load, allowing Next.js to determine the appropriate sizes for the image.
This is particularly useful when using the CldImage component from Next-Cloudinary, which automatically optimizes images and can even add responsive sizing by adding the sizes attribute.
Adding the sizes attribute will build a srcset attribute, so you only render the size you need for the viewport, giving you much better performance and avoiding sending way bigger image files than you need.
Check this out: Next Js Performance Analyzer
Cloudinary Integration
Integrating Cloudinary into your Next.js app can take your image search capabilities to the next level. You can use the Cloudinary Node.js SDK to fetch images from Cloudinary using the search method.
To get started, you'll need to make your home component asynchronous to use the async/await syntax. This will allow you to fetch image resources and display them in your gallery.
You can use the CldImage component from Next-Cloudinary to dynamically transform and optimize your images. This will automatically optimize the images by delivering the most modern format for the browser.
To further optimize your images, consider using a Content Delivery Network (CDN) such as Cloudinary to host your images. This can provide many benefits, including automatic caching, file compression, and image resizing on the fly.
The three most popular image formats on the web are JPEG, PNG, and WebP. WebP is highly recommended due to its many advantages and performance benefits, including superior lossy and lossless image compression and faster load times.
Here are some tools you can use to optimize your images:
- WebP-Converter: a good tool for converting your images to WebP.
- Responsive Breakpoints Generator tool by Cloudinary: a good tool for generating multiple image file sizes for different screen sizes.
- TinyPNG, Compressor.io: great tools for image compression.
Getting Started
To start a Next.js image search project, you need to create a skeleton that includes a search form and a grid to display images.
You can find the project structure in the page.tsx file within the app directory. Make sure you have some images uploaded to your Cloudinary account.
The project uses Tailwind Elements to design the search form and grid. You'll need to have some images uploaded to Cloudinary to display in your gallery.
Sources
- https://uploadcare.com/blog/next-js-image-optimization/
- https://nextjs.org/docs/pages/api-reference/components/image-legacy
- https://prismic.io/blog/nextjs-image-component-optimization
- https://spacejelly.dev/posts/image-gallery-with-search-in-next-js-with-cloudinary-node-sdk
- https://refine.dev/blog/using-next-image/
Featured Images: pexels.com