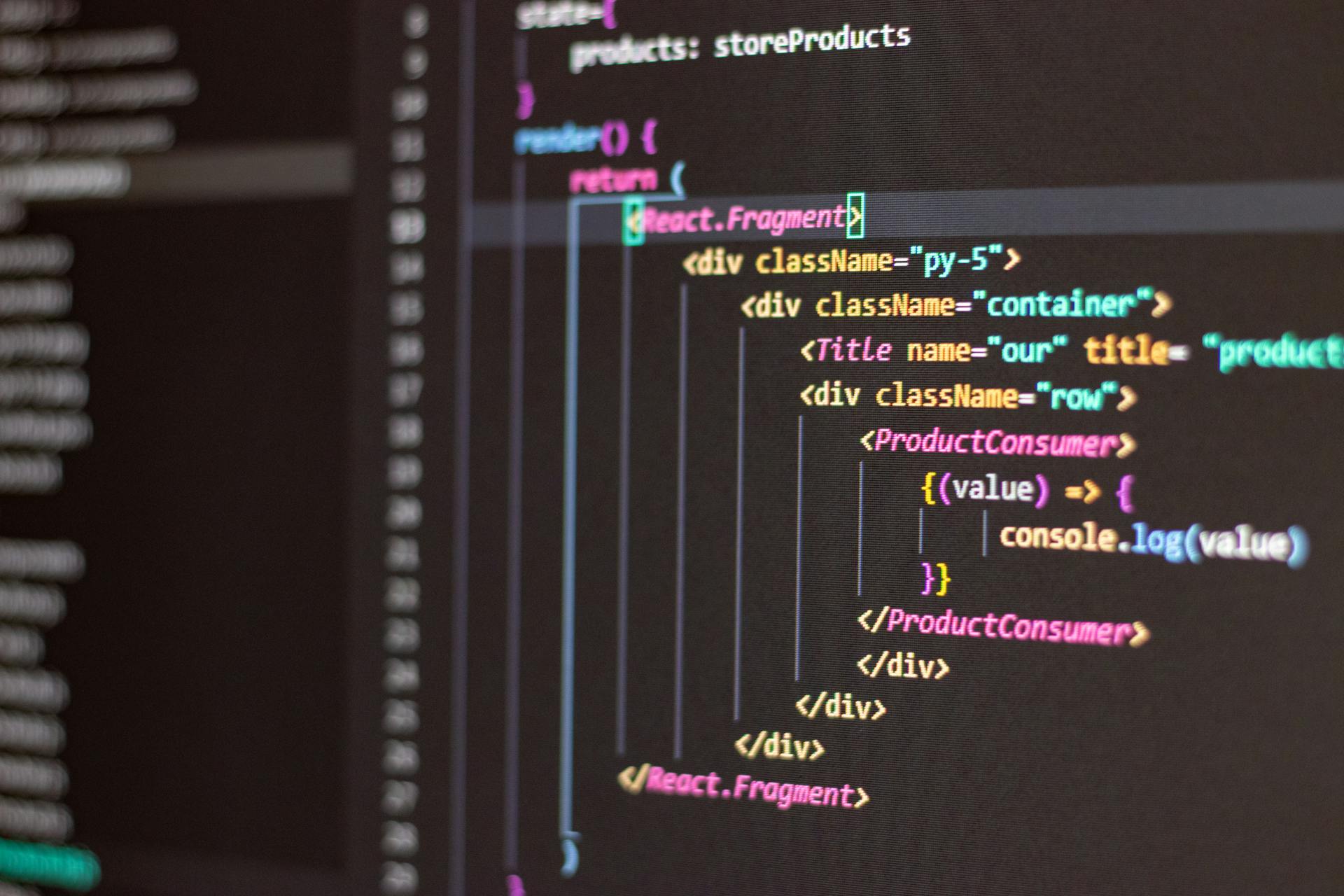
Next.js uses Webpack under the hood, and understanding its configuration is crucial for optimizing your application's performance. Webpack is a module bundler that takes your code and packages it into a single file, which is then served to the client.
In Next.js, you can configure Webpack through the next.config.js file. This file allows you to customize various Webpack settings, such as the module resolution and the output file path. For example, you can specify the output file path by setting the `target` option to `'web'`.
Code splitting is a technique used to break down large codebases into smaller, more manageable chunks. In Next.js, code splitting is achieved through the use of dynamic imports and the `getStaticProps` method. By using these methods, you can split your code into smaller modules that are loaded only when needed, resulting in faster page loads and improved performance.
Discover more: Next Js Project Structure
Configuring and Customizing
To enable the bundle analyzer in a Next.js app, add the following code to your next.config.js file.
You can customize your webpack configurations in Next.js by adding the webpack property to your next.config.js file and passing your configurations on to it.
To start customizing the Webpack configuration in your Next.js project, navigate to your project's root directory and edit or create a webpack.config.js file.
The webpack property in the exported object from this file accepts two arguments: config, representing the current Webpack configuration, and options, which includes various settings and flags provided by Next.js.
You can modify the config object to add loaders, plugins, or other adjustments to meet your project's needs.
For more complex scenarios, you might consider using custom plugins to extend Webpack's functionality within your Next.js project, such as the dotenv-webpack plugin which can load environment variables directly into your application.
Next.js lets you alter its default Webpack setup by tweaking the webpack property in the exported object from the webpack.config.js file, allowing you to add loaders, plugins, or other adjustments to meet your project's needs.
Curious to learn more? Check out: File Upload Next Js Supabase
Analyzing and Optimizing
Analyzing your Next.js app bundles is crucial for optimizing performance. To run an analysis, start your app in development mode with the ANALYZE environment variable set to true.
The bundle analyzer will automatically create an analyze folder in your .next folder, containing three files: client.html, nodejs.html, and edge.html. These files represent the client side, server side, and edge APIs of your app, respectively.
You can inspect your bundles by opening the client.html and nodejs.html files in your browser. The bundle analyzer will provide bundle analysis information in your terminal.
By analyzing your bundles, you can identify potential bottlenecks and areas for improvement. Consider the following tips:
- Spot large dependencies by inspecting modules with large sizes.
- Detect duplicate code by looking for library or module appearances multiple times in the bundle.
- Analyze bundle size metrics to optimize modules with large stat sizes.
- Identify unused code by inspecting the analysis report and excluding unused code by modifying the webpack config.
Here are some common issues to look out for:
To optimize your app's performance, consider compressing or resizing images with large file sizes, removing duplicate npm packages, removing unused npm packages, and using minification, tree shaking, and code splitting to optimize JavaScript files.
Broaden your view: Next Js Npm Install
Analyzing the
To analyze your Next.js app bundles, you'll need to start your app in development mode with the ANALYZE environment variable set to true. This will automatically create an analyze folder in your .next folder, containing three files: client.html, nodejs.html, and edge.html.
Related reading: Html to Json Parser Nextjs
The client.html file represents the client-side, while the nodejs.html file represents the server-side. The edge.html file, however, is still under development and doesn't currently provide any data.
After running the analysis, you can inspect your bundles and start identifying potential issues. You can also select an entry point for initial chunks, such as any of your app's pages.
To get a better understanding of the output, let's break down the key components:
- Rectangles: Each rectangle represents a module or package, with the size showing how large or tiny it is compared to others in the bundle.
- Color grouping: Files or modules that are related are grouped by colors.
- Hovering: Hovering over any rectangle shows you the stats related to that module.
- Clicking: Clicking on a module zooms into it, allowing you to have a more detailed view, especially if it has many nested modules/files inside of it.
Here are the key metrics to focus on:
- Stat size: The size of the JavaScript bundle when served to the client.
- Parsed size: The size of the bundle after the web browser has parsed it.
- Gzipped size: The size of the JavaScript bundle when compressed using the Gzip algorithm.
By analyzing these metrics, you can identify which bundles are taking up the most space and start optimizing your app's performance.
Optimizing Load Times and User Experience
Optimizing Load Times and User Experience is crucial for a Next.js app. A large JS bundle directly affects load times, leading to a poor user experience, especially on mobile devices or slow network connections.
The size of your JS bundle is a major contributor to load times. Large bundles take longer to download and parse, which can lead to a snappier, more responsive app.
Analyzing and optimizing your bundles can improve load times. This is achieved by identifying and addressing issues such as large image file sizes, duplicate npm packages, unused npm packages, and heavy JavaScript files.
Here are some possible ways to address these issues:
- Compress or resize images with large file sizes
- Remove duplicate npm packages
- Remove unused npm packages
- Use minification, tree shaking, and code splitting to optimize JavaScript files
By implementing these techniques, you can significantly improve your app's load times and user experience.
Code Splitting for Faster Load Times
Code splitting is a technique that can significantly reduce the initial page load of your pages, especially in large applications. It involves splitting your code into small chunks, which can then be loaded when needed.
By using dynamic imports, you can manually optimize your pages to only load the necessary code. For example, a component like DynamicComponent will only be loaded when needed, so it doesn't increase your page's load time.
Next.js already does code splitting for you, but it's worth understanding how it works and how you can optimize it further.
You might like: Next Js Pages
Here are some key benefits of code splitting:
- Reduces initial page load time
- Improves user experience
- Enhances SEO by favoring fast-loading sites
To get started with code splitting, you can use dynamic imports in your Next.js app. This will allow you to load only the necessary code, reducing the initial page load time and improving overall performance.
Remember, code splitting is an essential technique for optimizing app performance, especially in large applications. By using it effectively, you can create a snappier, more responsive app that delights users and contributes positively to SEO.
Worth a look: Nextjs Code Block
Troubleshooting and Best Practices
To troubleshoot issues with customizing Webpack in Next.js, carefully review the console output for error messages. This can provide valuable insights into any configuration problems.
Running your project with the npm run dev command can help identify issues in real-time, as it compiles your application. This can be a game-changer for spotting problems before they affect the user experience.
Following best practices such as effective code splitting, asset optimization, and careful loader configuration can greatly enhance the performance and maintainability of your Next.js application.
Check this out: Nextjs Spa
Troubleshooting and Best Practices
Customizing Webpack in Next.js can occasionally lead to unexpected issues. Running your project with the npm run dev command can provide insights into any configuration problems, as it compiles your application in real-time.
Carefully review the console output for error messages to troubleshoot issues. This will help you identify and fix problems quickly.
Effective code splitting is a best practice in Next.js that can greatly enhance the performance and maintainability of your application.
Asset optimization is another crucial aspect of Next.js development, and it can have a significant impact on user experience.
Thoroughly testing your custom Webpack configuration in both development and production environments is essential to prevent any negative impacts on the user experience.
Readers also liked: Nextjs Development Company
Identifying Potential Issues
Carefully review your console output for error messages, as they can provide valuable insights into configuration problems.
To identify potential issues, start by looking for unexpectedly large modules. If a module is larger than expected, it might be including unnecessary code or dependencies, so investigate whether you can import only the parts you need or replace them with a lighter alternative.
Check your bundles for duplicates, as repeated modules can indicate an issue with your code-splitting strategy. Consider refactoring to ensure that common dependencies are shared effectively.
Third-party packages can sometimes dominate your treemap, so analyze them closely. Research if smaller alternatives exist to reduce your bundle size.
Review your dynamic imports to ensure they're being used effectively to split your code into manageable chunks. If you're not using them correctly, it can lead to unnecessary code being loaded.
Regularly run the next bundle analyzer as part of your development process to monitor changes in bundle size and catch any regressions.
Frequently Asked Questions
What replaced webpack in Nextjs?
Turbopack has replaced Webpack in Next.js, requiring a new configuration approach. Check the documentation for setup instructions and supported loader compatibility
Is webpack still being used?
Yes, Webpack is still widely used and actively maintained, with a large community and ecosystem supporting its continued development and adoption. Its long history and widespread use make it a reliable choice for many developers.
Does NextJS use webpack or SWC?
Next.js uses SWC for code transformation and minification, not Webpack. This allows for faster build times and improved performance in production environments.
Where is the Next.js config?
The Next.js config is located in a file named `next.config.js` at the root of your project directory. Find it alongside your `package.json` file to start customizing your Next.js setup.
Sources
- https://blog.logrocket.com/how-to-analyze-next-js-app-bundles/
- https://www.dhiwise.com/post/next-bundle-analyzer-your-key-to-optimizing-next-js-app
- https://www.dhiwise.com/post/how-to-customize-the-next-js-webpack-config-a-developer-guide
- https://flowbite.com/docs/getting-started/quickstart/
- https://nextjs.org/docs/pages/api-reference/next-config-js/output
Featured Images: pexels.com