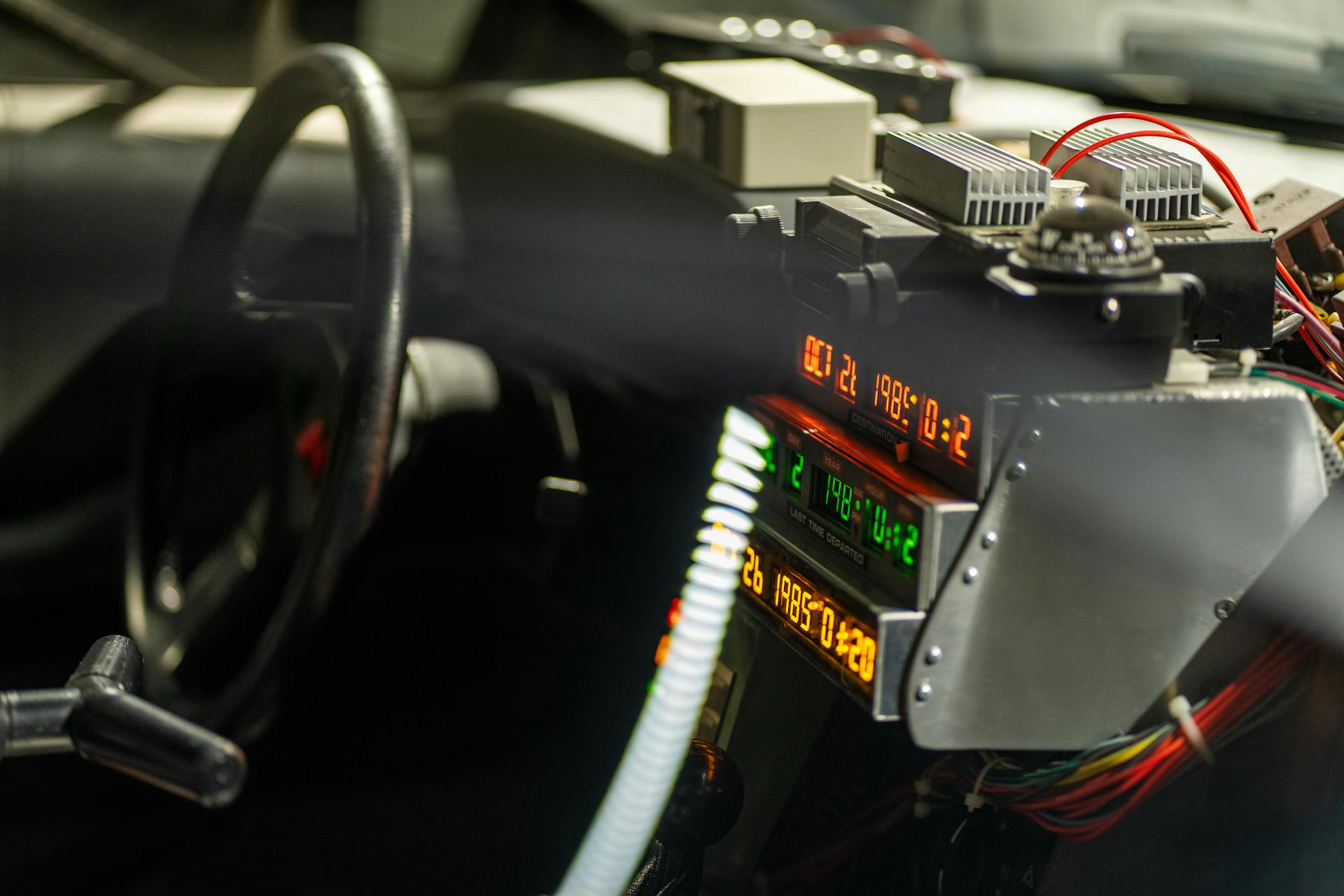
Building scalable apps with Firebase Firestore Next.js requires a solid understanding of how to structure your data and integrate it with Next.js.
With Firebase Firestore, you can store and manage your data in a scalable and flexible way.
By using Next.js, you can create fast and efficient server-side rendered applications.
Next.js and Firebase Firestore can be integrated seamlessly to create a powerful and scalable application.
By combining these two technologies, you can build a data-driven application that can handle large amounts of data and traffic.
This integration allows for real-time data synchronization and automatic data backup.
Firebase Firestore's scalability and Next.js's server-side rendering capabilities make for a winning combination.
A different take: Nextjs App Route Get Ssr Data
Setting Up
Setting up your development environment is the first step in building your Firebase Firestore Next.js app. Start by setting up your development environment and GitHub repository, which can be found at https://github.com/firebase/friendlyeats-web.
The repository contains sample projects for multiple platforms, but you'll only need the nextjs-start directory. Copy this folder into your own repository.
Related reading: Next Js Software Staging Environment Aws Amplify Gen 2
To set up Cloud Firestore, navigate to the Firebase console and expand the Build section. Select Firestore Database and click Create database. Choose a location for your database that's close to your users and click Next.
You'll want to create the database in test mode, which allows you to make requests to the database for a longer period of time. This is a crucial step, as it enables you to test your app without exposing it to potential security risks.
Here's a step-by-step guide to setting up Cloud Firestore:
- In the Firebase console, expand Build and select Firestore database.
- Click Create database.
- Leave the Database ID set to (default).
- Select a location for your database and click Next.
- Click Start in test mode.
- Click Create.
After creating your database, select Usage from the top menu bar and edit the rules. Publish the changes to enable requests to the database for a longer period of time. Remember to switch to production mode when your app is live to prevent cyber attacks.
Finally, create three database collections for the categories, products, and sales data.
You might enjoy: Next Js Database
Folder and File Structure
The folder and file structure of the app is divided into several key areas. Each area contains specific types of code and assets.
The src/components folder holds React components for filters, headers, restaurant details, and reviews. These components are crucial for building the user interface of the app.
The src/lib folder is where you'll find utility functions that aren't necessarily bound to React or Next.js. These functions can be used throughout the app to perform various tasks.
Firebase-specific code and Firebase configuration can be found in the src/lib/firebase folder. This includes settings for authentication, storage, and other Firebase services.
Static assets like icons are stored in the public folder. These assets are used to enhance the visual appeal of the app.
The src/app folder is dedicated to routing with the Next.js App Router. This is where you'll configure the app's navigation and routing behavior.
An API route handler is located in the src/app/restaurant folder. This handler is responsible for processing requests to the app's restaurant API.
The project dependencies are listed in package.json and package-lock.json files. These files are maintained by npm and ensure that the app's dependencies are correctly installed and updated.
Next.js-specific configuration is stored in the next.config.js file. This file enables server actions, which allow the app to perform server-side rendering and other advanced features.
The JavaScript language-service configuration is defined in the jsconfig.json file. This file ensures that the app's JavaScript code is properly formatted and linted.
Explore further: Next Js React Fundamentals
Backend and Database
To set up the backend, navigate to the App Hosting page in the Firebase console and click "Get started" to start the creation flow. Configure your backend by connecting your GitHub repository and setting deployment settings, including naming your backend "friendlyeats-codelab" and associating it with your existing Firebase web app.
You can monitor the status of your new App Hosting backend in the Firebase console, and once the rollout completes, click your free domain under "domains" to see your site in action. Every time you push a new commit to the main branch of your GitHub repository, a new build and rollout will begin, and your site will automatically update once the rollout completes.
Here's a quick rundown of the steps to set up your backend and database:
- Create a new backend in the Firebase console and configure it to connect to your GitHub repository.
- Set up Cloud Firestore by creating a new database in the Firebase console and selecting a location for your database.
- Use Cloud Firestore snapshot listeners to listen for updates to your database in real-time.
By following these steps, you'll have a fully functional backend and database set up for your Firebase Firestore Next.js project.
Cloud Storage Setup
In the Firebase console, expand Build and then select Storage to get started with Cloud Storage.
To set up Cloud Storage, click Get started and select a location for your default Storage bucket. Buckets in US-WEST1, US-CENTRAL1, and US-EAST1 can take advantage of the "Always Free" tier for Google Cloud Storage.
You'll need to click Start in test mode and read the disclaimer about security rules. Don't distribute or expose an app publicly without adding Security Rules for your Storage bucket.
To proceed, click Create and your Storage bucket will be set up.
Create a Backend
To create a backend, start by navigating to the App Hosting page in the Firebase console. Click "Get started" to begin the backend creation flow.
Configure your backend by connecting your GitHub repository, which you created earlier. Set deployment settings and name your backend "friendlyeats-codelab".
You'll need to select an existing Firebase web app from the dropdown menu. Click "Finish and deploy" to initiate the rollout process. Once complete, click your free domain under "domains" to view the status of your new App Hosting backend.
Broaden your view: Nextjs Firebase
Note that you can monitor the underlying Google Cloud services for your App Hosting backend by following links from the console. This is especially useful for troubleshooting any issues that may arise.
Here are the steps to create a backend in a concise list:
- Navigate to the App Hosting page in the Firebase console
- Click "Get started" to start the backend creation flow
- Configure your backend by connecting your GitHub repository
- Set deployment settings and name your backend "friendlyeats-codelab"
- Select an existing Firebase web app from the dropdown menu
- Click "Finish and deploy" to initiate the rollout process
- Click your free domain under "domains" to view the status of your new App Hosting backend
Send State to Server
To send state to the server, you'll need to use a service worker.
We've seen how to use a service worker to pass authentication state to the server, specifically by replacing the fetchWithFirebaseHeaders and getAuthIdToken functions.
A service worker is a script that runs in the background, allowing you to manage network requests and cache data.
This is particularly useful when working with authentication state, as it enables you to send the state to the server in a secure and reliable manner.
To implement this, you'll need to replace the existing code with the new service worker code.
This involves updating the fetchWithFirebaseHeaders and getAuthIdToken functions to use the service worker.
Using a service worker to send authentication state to the server provides several benefits, including improved security and reduced latency.
By leveraging the service worker, you can ensure that sensitive data is transmitted securely and efficiently.
For your interest: Using State in Next Js
Cloud Firestore
Choosing a location that's close to your users is important because it will improve the performance of your app. For a real app, you want to choose a location that's close to your users. You can click Next to proceed.
Click Start in test mode and read the disclaimer about the security rules. You'll add Security Rules later in this process to secure your data. Do not distribute or expose an app publicly without adding Security Rules for your database. Click Create to set up your database.
To create a new database, you'll need to go through these steps:
- In the left-panel of the Firebase console, expand Build and then select Firestore database.
- Click Create database.
- Leave the Database ID set to (default).
- Select a location for your database, then click Next.
- Click Start in test mode. Read the disclaimer about the security rules.
- Click Create.
You can create a new document (category) on Firebase when the user adds a new category. The code snippet accepts the category name from the input field and creates a new document (category) on Firebase.
Suggestion: New Nextjs Project Typescript
Real-Time Features
Real-time collaboration is crucial in the fast-paced world of collaborative editing, where Next.js and Firebase emerge as a powerful solution.
By combining Next.js and Firebase, you can revolutionize collaborative editing experiences and fetch real-time data from Firestore in a Next.js component. This synergy enables seamless updates and synchronization.
To fetch and display real-time data from Firestore in a Next.js component, you can use the useEffect hook to fetch the data when the component mounts. This involves importing the necessary modules and the firebase object from your firebase.js file, creating a state variable to store the fetched data, and mapping over the retrieved documents to render individual paragraphs for each item.
Here are the steps to fetch and display real-time data from Firestore in a Next.js component:
- Import the necessary modules and the firebase object from your firebase.js file.
- Create a state variable to store the fetched data.
- Use the useEffect hook to fetch the data when the component mounts.
- Inside the useEffect hook, use the firebase.firestore() method to access Firestore and fetch the data from a specific collection.
- Map over the retrieved documents and store them in the state variable.
Restaurant Updates
To implement real-time updates for your restaurant data, you can use Cloud Firestore snapshot listeners. This allows you to receive notifications whenever a change is made to the restaurant's collection.
You'll need to replace the getRestaurantsSnapshot() function in the src/lib/firebase/firestore.js file with the provided code. This will enable the callback mechanism that invokes every time a change is made to the restaurant's collection.
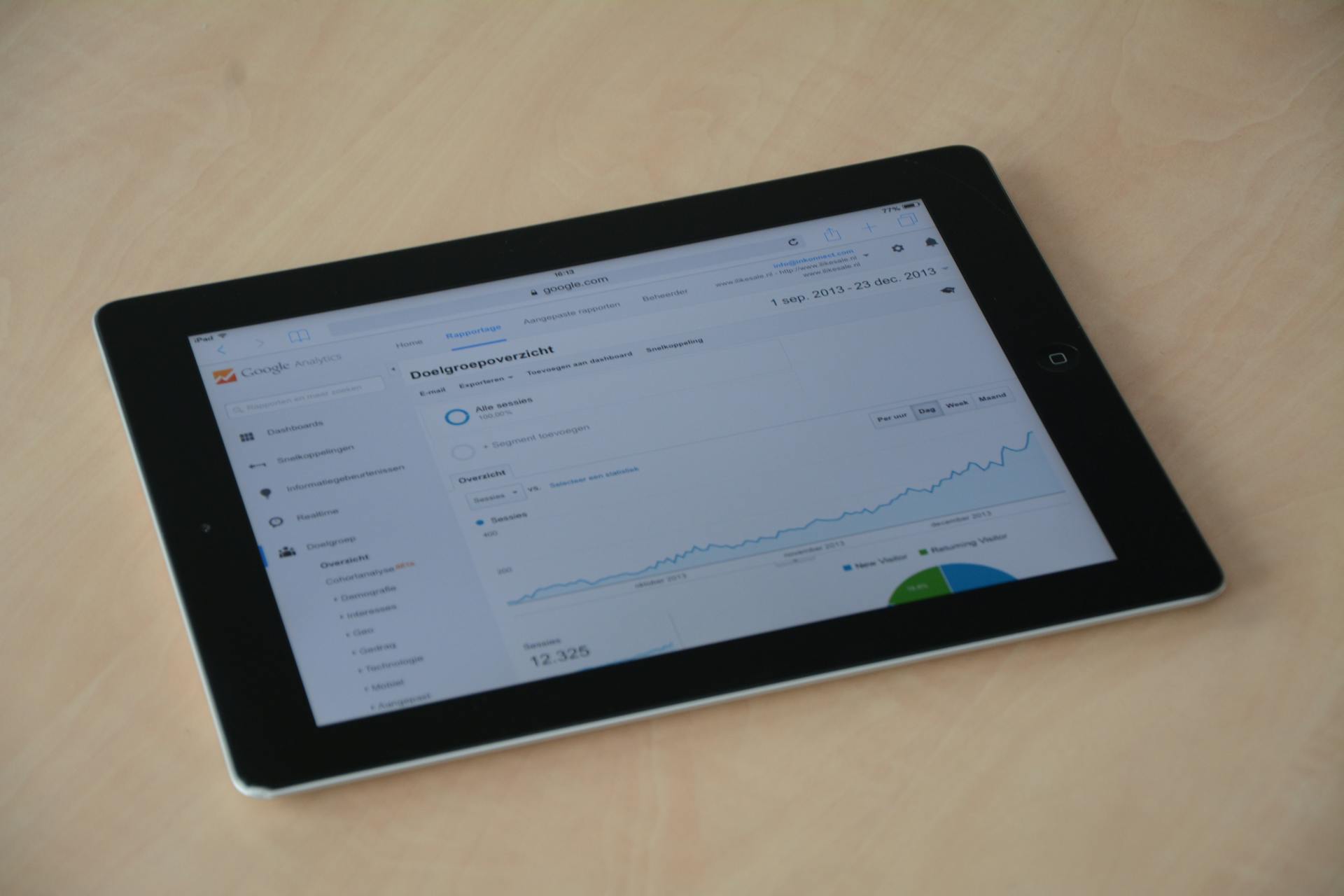
Changes made through the Firestore Database page will now reflect in your web app in real time. You can test this by selecting > Add sample restaurants in your web app. If your snapshot function is implemented correctly, the restaurants should appear in real-time without a page refresh.
To deploy your changes, create a commit with a message like "Listen for real-time restaurant updates" and push it to your GitHub repository. Then, open the App Hosting page in the Firebase console and wait for your new rollout to complete.
Real-Time Communication with CRUD Operation
Real-time communication is a crucial aspect of real-time features, and Firebase provides an efficient way to achieve this through its Real-time Database. In the context of CRUD (Create, Read, Update, Delete) operations, Firebase's Real-time Database allows for seamless data synchronization across all connected devices.
To delete a category and its associated products, you can use the deleteCategory function, which accepts the ID and name of the selected category. This function deletes the category from the list and also deletes the products under the selected category.
A unique perspective: Nextjs Middleware Firebase Auth
The deleteCategory function is a great example of how Firebase's Real-time Database can be used to implement CRUD operations in a real-time application.
Here's a step-by-step guide to implementing CRUD operations with Firebase's Real-time Database:
- Create a new category and add it to the list
- Read the list of categories and retrieve the category details
- Update an existing category and save the changes
- Delete a category and its associated products
By following these steps, you can create a robust and scalable real-time application with Firebase's Real-time Database.
In a real-time application, data is constantly being updated and synchronized across all connected devices. To achieve this, you can use Firebase's Real-time Database and its associated functions, such as deleteCategory.
Intriguing read: Next Js Single Page Application
Security and Authentication
Firebase provides a complete authentication system that allows you to easily manage user authentication in your application. With Firebase Authentication, you can authenticate users using email/password, phone numbers, Google, Facebook, and more.
You can implement user sign-up, sign-in, and access control features with ease using Firebase Authentication. To implement Firebase Authentication in a protected Next.js component, you need to import the necessary modules and the firebase object from your firebase.js file.
Discover more: Nextjs Usecontext
Firebase Authentication provides a secure and easy-to-use authentication system for your web applications. It offers a complete authentication system that allows you to easily manage user authentication in your application. With Firebase Authentication, you can authenticate users using email/password, phone numbers, Google, Facebook, and more.
Here are some key features of Firebase Authentication compared to traditional approaches:
Firebase Authentication also provides a secure way to protect pages containing confidential data from unauthenticated users. You can create a new user on your Firebase console and execute the function below when the sign-in form is submitted.
Access Control
Access Control is a crucial aspect of security and authentication. Firebase Authentication provides a secure and easy-to-use authentication system for web applications, allowing you to implement user sign-up, sign-in, and access control features with ease.
To implement access control in a protected Next.js component, you need to create a state variable to store the authenticated Firebase user. This can be done by importing the necessary modules and the firebase object from your firebase.js file, and then using the useEffect hook to listen for changes in the user's authentication state.
For another approach, see: State Management in Nextjs 14
The AuthProtectedComponent listens for changes in the user's auth status using the onAuthStateChanged method. If a user is authenticated, their display name is rendered in a welcome message. Otherwise, for an unauthenticated user a message asking them to sign in is displayed.
Here are some key features of Firebase Authentication that enable access control:
• Secure authentication system for web applications
• Easy-to-use sign-up, sign-in, and access control features
• Supports multiple authentication providers, including email/password, phone numbers, Google, Facebook, and more
By implementing Firebase Authentication in your Next.js app, you can secure your application and provide personalized experiences for your users.
Adding Comments
Adding comments to a post is a straightforward process. You'll need to update the postComment function with the Comments.tsx file to fetch the particular post.
To do this, you'll need to fetch the post and add the newly created comment to the comments array attribute. This is a crucial step in enabling users to engage with each other's content.
By following these simple steps, you'll be able to add comments to your posts and enhance the user experience.
A different take: Add Image to Next Js
Comparing Methods
Traditional authentication methods can be a hassle to set up, requiring server-side logic and manual user session management.
Ease of integration is one of the key advantages of Firebase authentication, which offers simple integration with its pre-built methods.
Implementing social media authentication with traditional approaches can be complex, involving individual OAuth providers.
Firebase, on the other hand, provides out-of-the-box support for various OAuth providers like Google, Facebook, Twitter, and more.
Setting up passwordless authentication with traditional methods may require additional libraries, but Firebase offers built-in support.
Implementing multi-factor authentication (MFA) with traditional approaches may require additional third-party tools, but Firebase provides straightforward MFA integration.
Real-time user management is another area where Firebase shines, automatically synchronizing user data across devices in real-time.
Customization and extensibility are also key benefits of Firebase authentication, with options to extend through Cloud Functions.
Frequently Asked Questions
Is NextJS good with Firebase?
Yes, Next.js integrates well with Firebase, enabling robust full-stack application development. This powerful combination simplifies authentication, data management, and server-side rendering for high-performance web applications.
How to use Firebase database in NextJS?
To use Firebase database in NextJS, first set up your Firebase project and add a web app to it, then follow the steps to set up Firebase services in the console. This will enable you to integrate Firebase database with your NextJS application.
Sources
- https://firebase.google.com/codelabs/firebase-nextjs
- https://dev.to/arshadayvid/how-i-built-a-sales-management-app-with-nextjs-13-typescript-and-firebase-16cb
- https://theankurtyagi.com/how-to-create-blog-with-nextjs-and-firebase/
- https://www.freecodecamp.org/news/create-full-stack-app-with-nextjs13-and-firebase/
- https://blogs.purecode.ai/blogs/nextjs-firebase
Featured Images: pexels.com