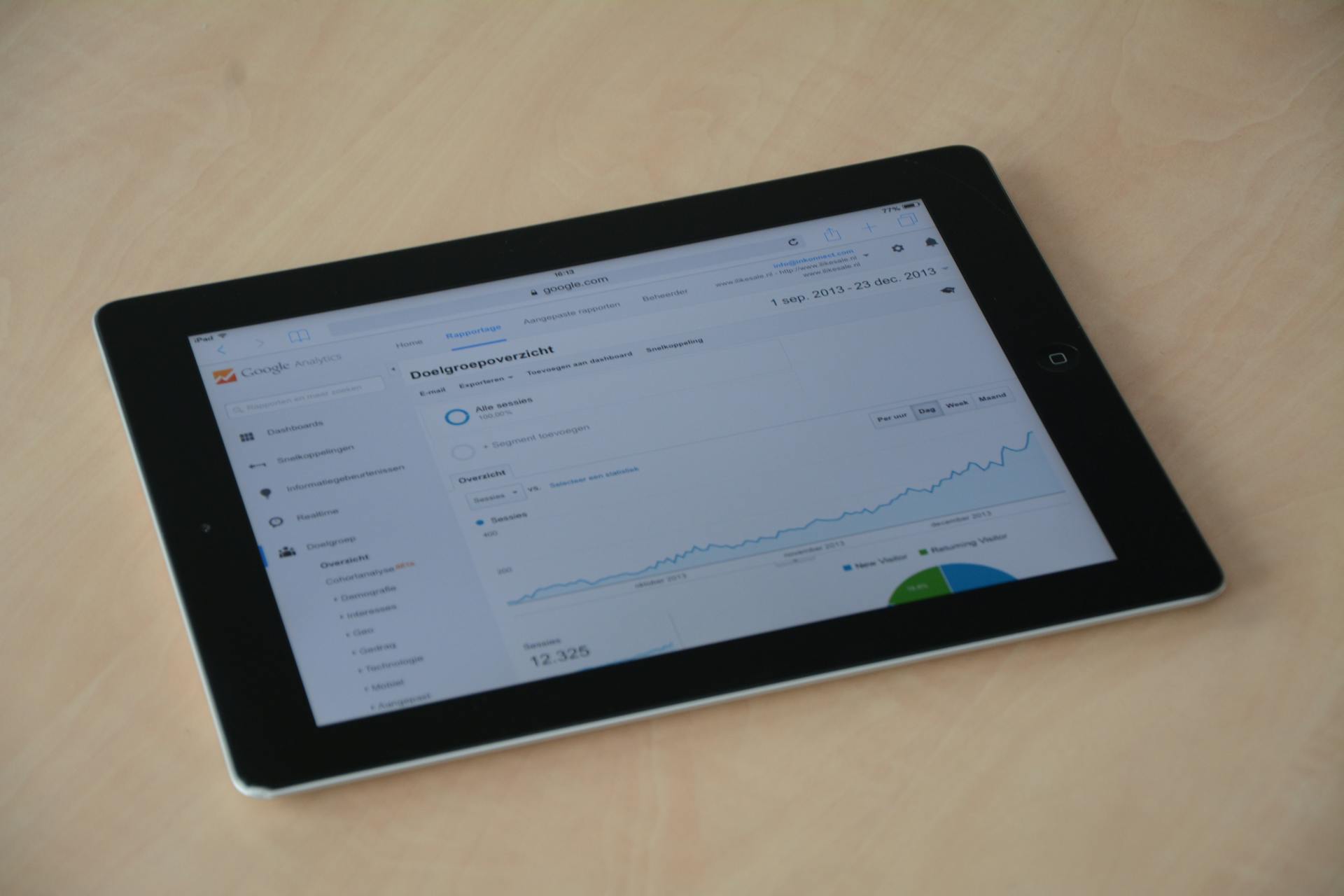
Setting up Firebase with Next.js is a straightforward process that can be completed in just a few steps. First, you'll need to create a new Firebase project in the Firebase console.
Next, you'll need to install the Firebase SDK in your Next.js project using npm or yarn. This can be done by running the command `npm install firebase` or `yarn add firebase` in your terminal.
Once the SDK is installed, you can initialize Firebase in your Next.js project by creating a new instance of the Firebase app and passing in your project's configuration. This can be done in a file like `firebase.js` or `firebase.config.js`.
With Firebase set up and configured, you can start using its features in your Next.js project. For example, you can use Firebase Authentication to handle user authentication, or Firebase Firestore to store and manage data.
You might like: New Relic Lambda Layer
Development Environment
To set up your development environment for Firebase Next.js, start by creating a GitHub repository and cloning the codelab source from https://github.com/firebase/friendlyeats-web. This repository contains sample projects for multiple platforms, but you'll only need the nextjs-start directory.
Here's an interesting read: Nextjs Middleware Firebase Auth
The nextjs-start folder can be copied into your own repository. This will give you a solid starting point for your project.
Next.js has built-in dotenv support, which allows you to securely store and read your Firebase configuration. You can use environment variables prefixed with NEXT_PUBLIC_ to make your Firebase configuration accessible in the client-side bundle.
You'll need to set up environment variables like NEXT_PUBLIC_FIREBASE_PROJECT_ID, FIREBASE_ADMIN_CLIENT_EMAIL, and FIREBASE_ADMIN_PRIVATE_KEY, which can be retrieved from a .json file downloaded after generating service account credentials.
Project Setup
To set up a Firebase project, start by navigating to your Project overview in your Firebase project and click Web. If you already have apps registered, click Add app to see the Web icon.
You'll need to enter a memorable app nickname, such as My Next.js app, in the App nickname text box.
Keep the Also set up Firebase Hosting for this app checkbox unchecked.
To add a web app to your Firebase project, follow these steps:
- Navigate to your Project overview
- Click Web
- In the App nickname text box, enter a memorable app nickname
- Keep the Also set up Firebase Hosting for this app checkbox unchecked
- Click Register app > Next > Next > Continue to console
Once you've set up your Firebase project, you can install the Firebase SDK in your Next.js project by running a code snippet. This will allow you to access Firebase features within your application.
Firebase Setup
To set up Firebase, start by running npm install firebase at your project's root directory. This will get the Firebase Client SDK installed and ready to go.
Next, create a new file called firebase.ts in your project root directory and paste the initialization code from the Firebase Client SDK documentation. This will expose the app object for use in your client components.
Console Up Services
Console Up Services are a great way to get your Firebase setup up and running. With Realtime Database, you can easily store and sync data across all your app's clients in real-time, making it perfect for apps that require real-time updates, such as live chat or multiplayer games.
Realtime Database allows for easy data synchronization across all connected clients. This means that any changes made to the data will be reflected instantly across all connected devices, making it ideal for apps that require real-time updates.
Cloud Firestore is another great option for storing and syncing data, offering more features and scalability than Realtime Database. It's perfect for apps that require more complex data structures and scalability, such as social media platforms or e-commerce websites.
For another approach, see: Nextjs Database
Cloud Firestore offers offline support, allowing users to continue using your app even without an internet connection. This feature is especially useful for apps that require users to access data even when they're offline, such as navigation apps or productivity tools.
Hosted Firebase Functions provide a scalable and secure way to run backend code, allowing you to build custom APIs and microservices. With Functions, you can create server-side logic that runs in response to events triggered by Firebase features, such as Realtime Database or Cloud Storage.
Hosted Firebase Functions support a wide range of languages, including Node.js, Python, and Go. This means you can choose the language you're most comfortable with and use it to build your backend logic, making it easier to integrate with your existing codebase.
Intriguing read: Next Js Serverless Functions
Installing Client SDK
To install the Firebase Client SDK, run npm install firebase at the project's root directory. This will get the necessary library installed.
You can then create a firebase.ts file in the project root directory to initialise the Firebase Client SDK. Paste the following code into this file.
This will expose the app object for client components, making it available for use in your application.
Broaden your view: Serverless Aws Upload File to S3 Api Gateway Typescript
Server and Client
In a Firebase Next.js app, you can have both server and client components. The src/app/page.js file is a server component responsible for the main page, while the src/components/RestaurantListings.jsx file is a client component denoted by the "use client" directive.
The server component is rendered on the server, including the Firebase data-fetching code. This means that data can be loaded at server runtime, as seen in the initial set of restaurants loaded from the src/app/page.js file.
To verify that restaurant listings load at server runtime, you need to configure the src/components/RestaurantListings.jsx file to hydrate server-rendered markup. This involves adding a specific directive to the file.
You can create a new file called firebase.js in your app, which will hold your Firebase configuration code. This file is crucial for connecting your app to Firebase.
A Next.js Server Action provides a convenient API to access form data, such as data.get("text") to get the text value from the form submission payload. You can use this action to process the review form submission in your app.
A unique perspective: Why Next Js
The _app.js file is the root of the app and should get run for every page a user might visit. You can add an import statement for Firebase in this file to make Firebase available globally.
Here's a summary of the different types of components in a Firebase Next.js app:
Authenticating User Access
You can protect certain pages from unauthenticated users by determining whether the current user is signed in or not. This can be helpful when you need to restrict access to sensitive information or features.
To achieve this, you need to add code that listens to the authentication state of the signed-in user and updates the current view of the application based on the authentication status. This is done by adding a code snippet to the posts/create/page.tsx file.
Firebase allows you to easily manage user authentication in your application with its complete authentication system. You can authenticate users using email/password, phone numbers, Google, Facebook, and more.
To implement the sign-in and sign-out functions, you need to replace the onAuthStateChanged, signInWithGoogle, and signOut functions in the src/lib/firebase/auth.js file with the following code:
- Firebase API
- Description
- GoogleAuthProvider
- Creates a Google authentication provider instance.
- signInWithPopup
- Starts a dialog-based authentication flow.
- auth.signOut
- Signs out the user.
The src/components/Header.jsx file already invokes the signInWithGoogle and signOut functions, making it easy to integrate with your application.
Data Management
To manage your data effectively, create a new Firebase > Firestore directory to keep all your Firestore-related code organized.
This will help you keep your project tidy and make it easier to find the code you need.
In this directory, you'll add all your Firestore-related code, making it a centralized location for managing your data.
Think of it like a filing cabinet for your project, where you can store and access your data with ease.
By doing this, you'll be able to focus on building your application without getting bogged down in data management details.
To access your Firestore data, you can use the Firebase console or write custom code to interact with your database.
Remember, a well-organized data management system is key to a successful Firebase Next.js project.
File Management
In Firebase Next.js, you can save user-uploaded files from the web app by replacing the image associated with a restaurant when you're logged in. This involves uploading the image to Firebase Storage and updating the image URL in the Cloud Firestore document that represents the restaurant.
To achieve this, you'll need to update the updateRestaurantImage() and uploadImage() functions in the src/lib/firebase/storage.js file with the provided code. The updateRestaurantImageReference() function is already implemented for you, which updates an existing restaurant document in Cloud Firestore with an updated image URL.
You can also set up Cloud Storage for Firebase by following these steps: In the Firebase console, expand Build and then select Storage.Click Get started.Select a location for your default Storage bucket.Click Start in test mode and read the disclaimer about the security rules.Click Create.
See what others are reading: Next Js Serverless
Cloud Storage
Cloud Storage is a powerful tool for managing files in your web app. You can save user-uploaded files from the web app by uploading the image to Firebase Storage and updating the image URL in the Cloud Firestore document that represents the restaurant.
A unique perspective: Azure Linux Function App
To set up Cloud Storage for Firebase, you need to follow these steps: expand Build and then select Storage in the left-panel of the Firebase console, click Get started, select a location for your default Storage bucket, and click Start in test mode.
You can verify that the image-upload functionality works as expected by creating a commit with a specific commit message, pushing it to your GitHub repository, and waiting for your new rollout to complete. Then, you can open the App Hosting page in the Firebase console, log in to the web app, select a restaurant, click to upload an image, and navigate to Cloud Storage for Firebase to check if the image exists in the folder that represents the restaurant.
You can also use Cloud Storage to upload post cover images, just like you do with restaurant images. To do this, you need to update the `handleCreatePost` function within the posts/create/page.tsx file to save the post's details to Firebase Firestore, upload the post's cover image to Firebase Cloud Storage, and update the posts collection with a new attribute containing the URL of the uploaded cover image.
Here are the steps to set up Cloud Storage for Firebase:
- Expand Build and then select Storage in the left-panel of the Firebase console.
- Click Get started.
- Select a location for your default Storage bucket.
- Click Start in test mode.
- Click Create.
Create a File
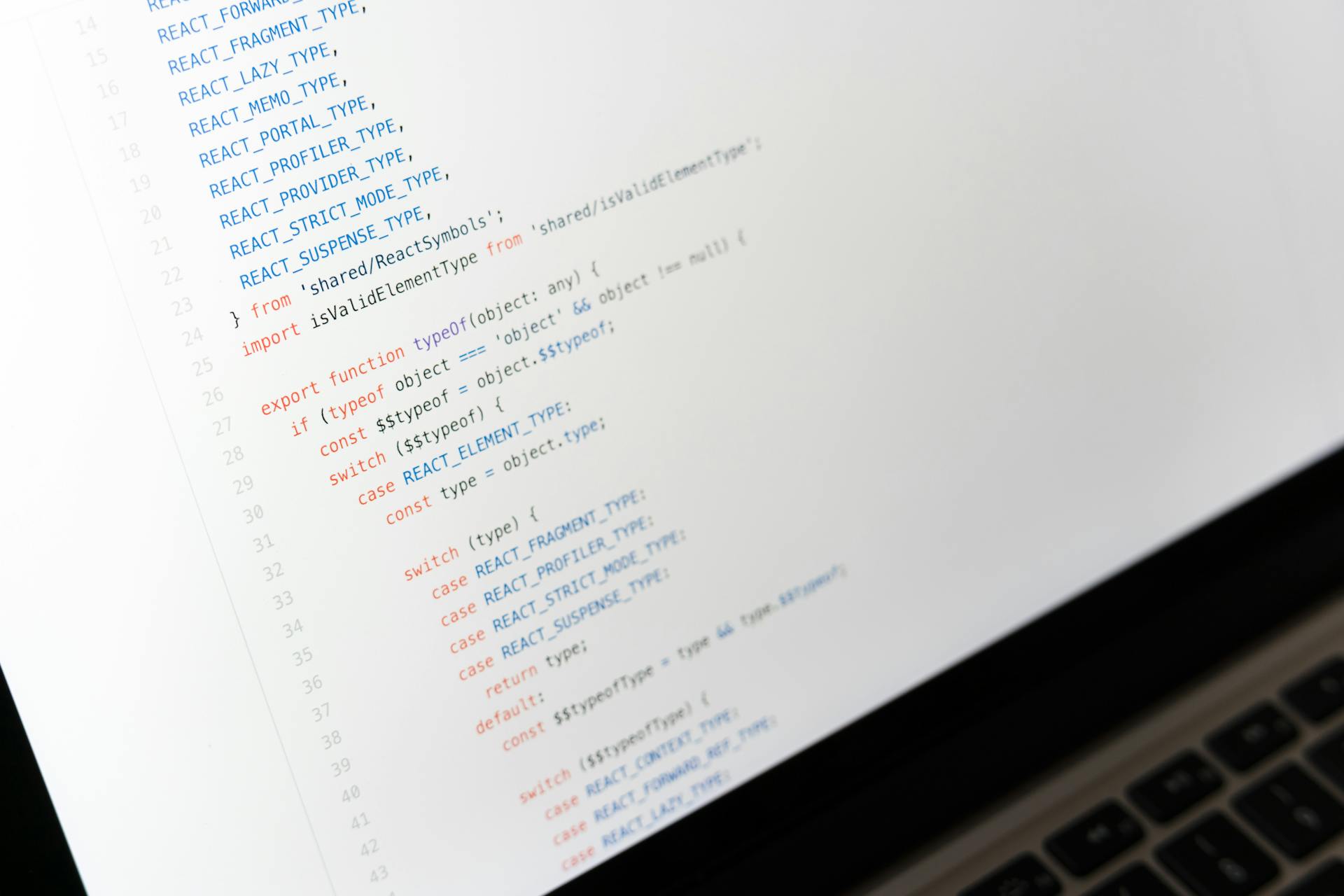
Creating a new file in your app is a straightforward process.
You can pick a sensible location for the file, such as a components folder or src folder, to keep it organized.
To create a new file, simply go to your app and click on the "Create new file" option.
Give the file a name that makes sense, like "firebase.js" as seen in the example.
Paste in your config code into the new file.
Remember, it's essential to add a specific line to the file as requested by Firebase, which can sometimes get you stuck.
Next.js Features
Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized web applications. It provides a lot of built-in features that make development easier.
One of the key features of Next.js is its ability to automatically optimize images. This means you don't have to worry about resizing or compressing images, Next.js will take care of it for you.
You might enjoy: Next Js on Aws
Next.js also has built-in support for internationalization (i18n) and localization (L10n). This allows you to easily translate your application into different languages and adapt it to different regions.
With Next.js, you can use API routes to create server-side API endpoints. This makes it easy to handle API requests and return data to the client.
Next.js also allows you to use getStaticProps to pre-render pages at build time. This improves performance and reduces the load on your server.
Next.js has a built-in CSS-in-JS solution called styled-components. This allows you to write CSS in your JavaScript files and easily manage styles.
Next.js also supports automatic code splitting, which means that only the code that's needed by the user is loaded. This improves performance and reduces the load on the server.
Consider reading: Next Js App Router Api
Database and Firestore
To set up a database and Firestore in your Next.js project, you'll need to create a new Firebase project and install the Firebase SDK. This can be done by running a code snippet in your terminal.
You can use a file called `firebase.ts` to store your Firebase configuration code. This file should be located at the root of your Next.js project.
To communicate with your database, create a new directory called `firestore` inside your Firebase directory. This is where you'll store all your Firestore-related code.
Here are some key steps to keep in mind:
- Create a new file called `addData.js` inside the `firestore` directory and add code to add data to your Firestore database.
- Create a new file called `getData.js` inside the `firestore` directory and add code to retrieve data from your Firestore database.
- Use a snapshot listener to listen for updates in your Firestore database. This can be done by replacing the `getRestaurants()` function with a `getRestaurantsSnapshot()` function, which provides a callback mechanism to invoke every time a change is made to the restaurant's collection.
By following these steps, you'll be able to set up a database and Firestore in your Next.js project and start communicating with your database in real-time.
Cloud Firestore Snapshot Listeners for Restaurant Updates
To listen for restaurant updates in real-time, you can use Cloud Firestore snapshot listeners. This allows your web app to reflect changes made to the restaurant's collection instantly.
You'll need to replace the getRestaurantsSnapshot() function with a callback mechanism that invokes a function every time a change is made to the collection. This can be done by adding a new file in the src/lib/firebase/firestore.js directory.
Recommended read: Firebase Firestore Nextjs
In this new file, add the following code to implement the getRestaurantsSnapshot() function.
- Replace the getRestaurantsSnapshot() function with the following code:
This will enable real-time updates in your web app. To test it, add sample restaurants and observe how they appear instantly without a page refresh.
To set up the snapshot listener, you'll need to create a commit with a commit message "Listen for realtime restaurant updates" and push it to your GitHub repository. Then, open the App Hosting page in the Firebase console and wait for your new rollout to complete.
Here's a step-by-step guide to implementing the snapshot listener:
- Create a commit with commit message "Listen for realtime restaurant updates" and push it to your GitHub repository.
- Open the App Hosting page in the Firebase console and wait for your new rollout to complete.
- In the web app, select > Add sample restaurants. If your snapshot function is implemented correctly, the restaurants appear in real-time without a page refresh.
Adding Documents to Firestore
Adding documents to Firestore is a straightforward process. Create a new file called addData.js inside the Firestore directory.
To start, you'll need to add the following code to the addData.js file. This code exports a function that adds data to your Firestore database.
You can now use this addData() function from any component to add data to your database. This makes it easy to manage and update your data in real-time.
To get started, make sure you have a Firestore database set up, and then you can use the addData() function to add new documents to your database.
Sources
- https://firebase.google.com/codelabs/firebase-nextjs
- https://hackernoon.com/using-firebase-authentication-with-the-latest-nextjs-features
- https://theankurtyagi.com/how-to-create-blog-with-nextjs-and-firebase/
- https://www.freecodecamp.org/news/create-full-stack-app-with-nextjs13-and-firebase/
- https://wideawakeben.medium.com/adding-firebase-analytics-and-firestore-to-a-react-next-js-app-bffffc2f638e
Featured Images: pexels.com