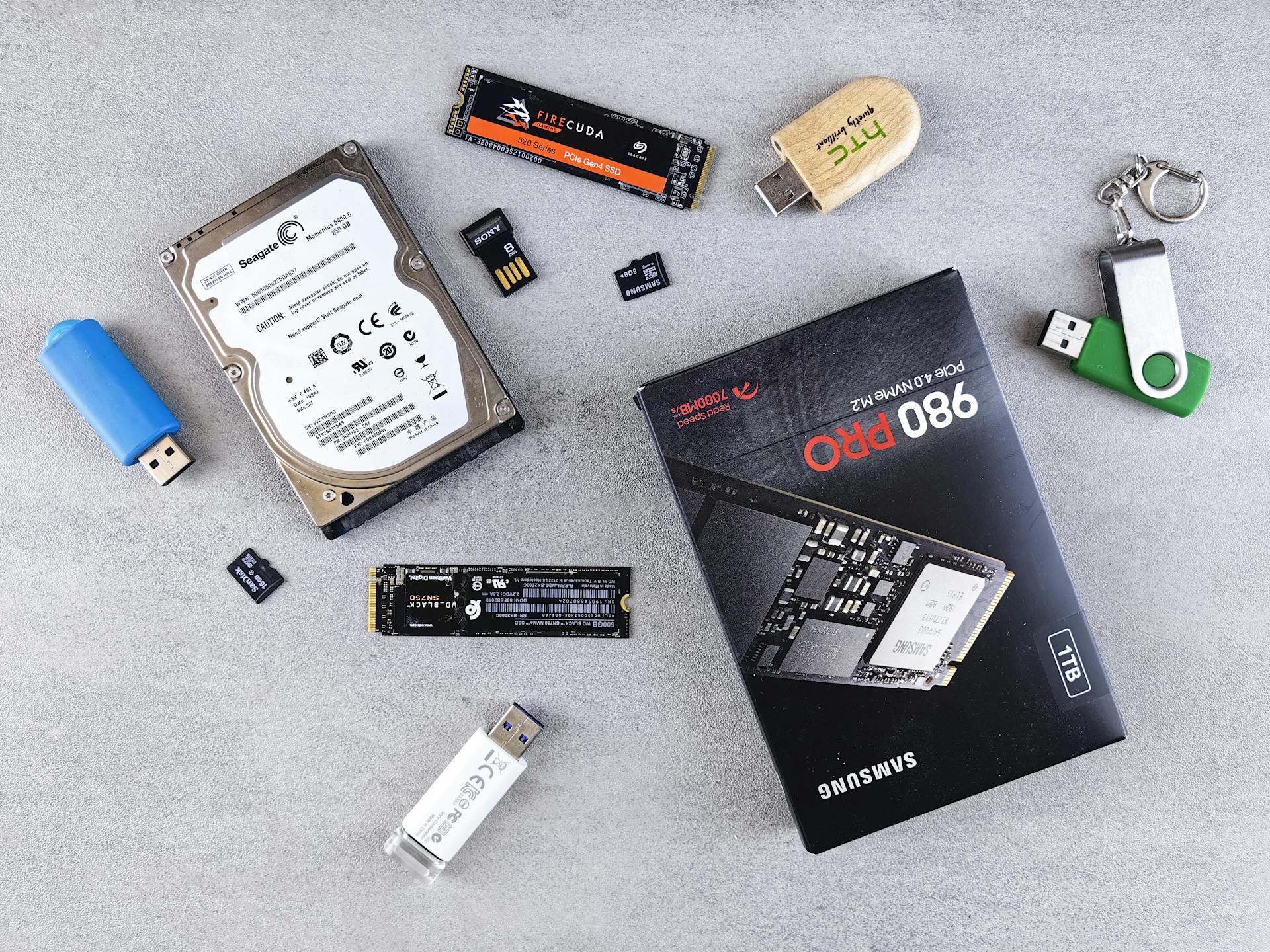
UTF-8 is a variable-length character encoding standard that allows for the representation of any Unicode character.
It's a crucial aspect of data transmission, as it enables the encoding of non-English characters and symbols in a way that's compatible with most systems.
In UTF-8, each character is represented by a unique sequence of bytes, with the first byte indicating the character's type and the subsequent bytes providing additional information.
This encoding scheme is widely used on the web and in many programming languages, making it a fundamental part of web development and data exchange.
Worth a look: Text Html Charset Utf 8
Encoding Basics
Base64 encoding is a way to convert data (typically binary) into the ASCII character set.
Base64 is not an encryption or compression technique, but it can sometimes be confused as encryption due to the way it seems to obscure data.
The size of a Base64 encoded piece of information is 1.3333 times the actual size of your original data.
Base64 is the most widely used base encoding technique with Base16 and Base32 being the other two commonly used encoding schemes.
Base64 encoding increases the size of the data, making the output of even small images fairly large.
If this caught your attention, see: Data Text Html Base64
Encoding with Node.js
Encoding with Node.js is a breeze, thanks to the Buffer object. This object is a global object in Node.js, meaning you don't need to require it to use it in your applications.
You can encode Base64 strings in Node.js using the Buffer object, which is internally an immutable array of integers capable of performing many different encodings/decodings, including Base64.
In Node.js, you can create a new buffer object and pass it a string to convert to Base64, and then call the toString method on the buffer object with "base64" as a parameter to get the Base64 string.
Base64 encoding is useful for converting binary data into textual format, and in Node.js you can use the Buffer object to load binary data into a buffer via the readFileSync() method of the fs module, and then convert it to a Base64 string.
Here's an interesting read: How to Use Inspect Element to Find Answers
Encoding Strings with Node.js
Encoding strings with Node.js is a breeze, thanks to the Buffer object. This global object in Node.js is an immutable array of integers that can perform various encodings, including Base64.
You can encode a text string to Base64 using the Buffer object by creating a new buffer object and passing it the string you want to convert. Then, call the toString method on the buffer object with "base64" as a parameter.
For example, you can save the following code in a file named encode-text.js:
```javascript
const buffer = Buffer.from('Hello, World!');
const base64String = buffer.toString('base64');
console.log(base64String);
```
This will output the Base64 counterpart for the string "Hello, World!". Make sure you have Node.js installed before running this code.
To decode a Base64 string, you can create a new buffer object and pass two parameters to its constructor: the Base64 data and "base64". Then, call the toString method on the buffer object with "ascii" as a parameter.
Here's an example:
```javascript
const buffer = Buffer.from('Tm8gdG8gUmFjaXNt', 'base64');
const decodedString = buffer.toString('ascii');
console.log(decodedString);
```
This will output the decoded string "No to Racism".
The Buffer object can also be used to decode Base64 strings to binary data. This is done by creating a new buffer object and passing the Base64 data to it, specifying that it's in base64 format.
Here's an example:
```javascript
const buffer = Buffer.from('Tm8gdG8gUmFjaXNt', 'base64');
A different take: Cloudfront Aws Webflow Example
console.log(buffer);
```
This will output the binary data represented by the Base64 string.
It's worth noting that the Buffer object has many other uses beyond encoding and decoding strings. It's a powerful tool in Node.js that can help you work with binary data and perform various encoding and decoding operations.
Here's an interesting read: Html Text Encoding
Encoding Binary Data
Encoding binary data into a format that can be easily transported or stored is a common task in Node.js. Base64 encoding is a widely used technique for this purpose.
Base64 encoding converts binary data into the ASCII character set, which is a text-based format. This means you can easily store or transmit the data, even if it's not in a traditional text format.
The size of a Base64 encoded piece of information is 1.3333 times the actual size of your original data, so keep that in mind when working with large files. This can be a significant increase in size, so it's essential to consider this when working with Base64 encoding.
Take a look at this: Img Src Base64
Base64 is not an encryption or compression technique, although it can sometimes be confused as encryption due to the way it seems to obscure data. It's simply a way to represent binary data in a text-based format.
You can use the fs module in Node.js to load binary data, such as an image, into a buffer. This can then be converted into a Base64 string, as seen in an example where an image is converted into a Base64 string.
Suggestion: Html Image and Text Side by Side
HTML Converter
You can use an HTML converter to quickly decode HTML escape codes back to human-readable UTF8 strings. This tool can convert hexadecimal and decimal HTML entities, as well as named HTML entities.
An HTML converter is a powerful tool for developers working with Node.js, as it can decode HTML escape codes and convert them into readable strings. This is especially useful when working with data that contains encoded characters.
One example of an HTML converter is the tool mentioned in the article, which can convert hexadecimal and decimal HTML entities. It also supports named HTML entities, making it a versatile tool for developers.
Using an HTML converter can save you time and effort when working with encoded data in Node.js. It's a quick and efficient way to decode HTML escape codes and get back to work.
Take a look at this: Colo Back Text Html
Encoding and Decoding
Base64 encoding is a way to convert binary data into the ASCII character set, increasing the size of the data by 1.3333 times. It's not an encryption or compression technique, but rather a way to represent binary data in a text format.
The Buffer object in Node.js is a global object that can perform many different encodings and decodings, including Base64. You can create a new buffer object and pass it your string or binary data to convert it to Base64.
To encode a string to Base64, you can use the Buffer object's toString method with "base64" as a parameter. To decode a Base64 string, you create a new buffer object and pass the Base64 data and "base64" as parameters, then call toString with "ascii" as a parameter.
See what others are reading: Html to Rich Text Converter
Decoding Strings to Binary
Decoding Base64 strings to binary data is similar to creating a Base64 string from ASCII text, but with a different output destination.
The reverse process involves loading the Base64 data into a Buffer object, telling it that it's in base64 format, which allows the buffer to parse it accordingly for internal storage.
We start with the Base64 data, which could've also been received from a socket or some other communication line, and load it into a Buffer object.
The Buffer object is created with the Base64 data, and it's used as the output destination for the decoded binary data.
Similar to creating a Base64 string from ASCII text, we tell the buffer how to store the data, and it takes care of the rest.
Decoding Base64 strings to binary data can be done using a Buffer object, just like encoding binary data to Base64 strings.
Decoding
Decoding is a crucial step in working with Base64 strings.
Decoding a Base64 string in Node.js is quite similar to encoding it. You have to create a new buffer object and pass two parameters to its constructor. The first parameter is the data in Base64 and the second parameter is "base64".
The output of the decoded data will be in the form of ASCII characters.
To decode a Base64 string, you simply have to call toString on the buffer object but this time the parameter passed to the method will be "ascii" because this is the data type that you want your Base64 data to convert to.
You can see this in action by running the code in the ascii.js file, which decodes the Base64 string "Tm8gdG8gUmFjaXNt" to display "No to Racism".
Frequently Asked Questions
What is text charset UTF-8 in HTML?
The "meta charset=utf-8" HTML tag allows you to display emojis and non-ASCII characters on your webpage. Without it, you'll need to use HTML entities to manually insert special characters.
How to display Base64 text in HTML?
To display Base64 text in HTML, prefix the text with "data:image/[mime-type];base64," where [mime-type] is the file type (e.g. jpeg, gif, or svg+xml). This allows you to embed images directly in your HTML code.
What is Base64 encoding used for?
Base64 encoding is used to convert binary data into printable text for safe transport over protocols or mediums that can't handle binary formats. This allows for easy sharing and transmission of binary data across various platforms.
Sources
- https://www.googlecloudcommunity.com/gc/Apigee/How-to-render-base64-image-on-response/m-p/729632
- https://stackabuse.com/encoding-and-decoding-base64-strings-in-node-js/
- https://onlinetools.com/utf8/convert-html-entities-to-utf8
- https://www.php.net/manual/en/function.htmlspecialchars.php
- https://developer.mozilla.org/en-US/docs/Web/URI/Schemes/data
Featured Images: pexels.com