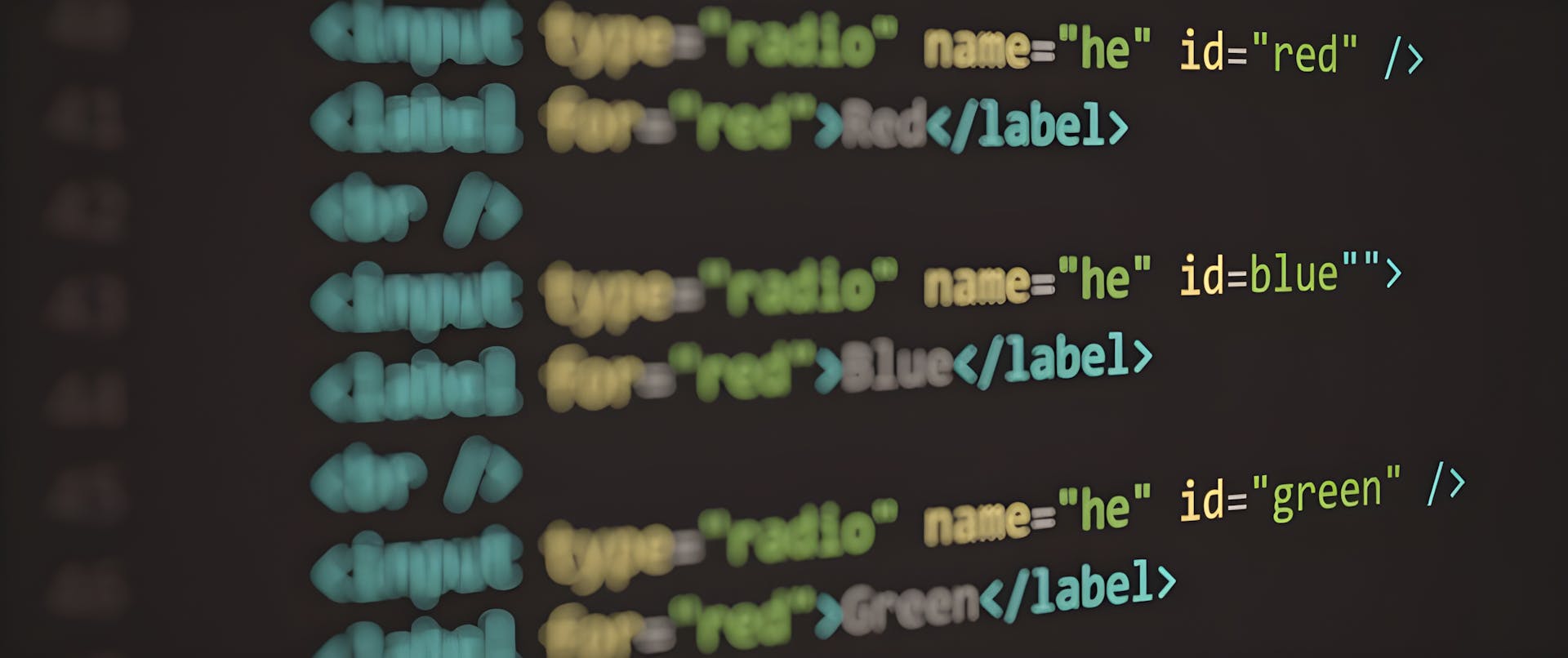
Reading JSON data in HTML can be a bit tricky, but don't worry, I've got you covered. It's all about understanding the basics of JSON and how to integrate it into your HTML code.
To start, JSON (JavaScript Object Notation) is a lightweight data interchange format that's easy to read and write. It's often used to store and transmit data between web servers, web applications, and web browsers.
The key to reading JSON data in HTML is to use the `JSON.parse()` method, which converts a JSON string into a JavaScript object. This allows you to easily access and manipulate the data within your HTML code.
For example, let's say you have a JSON string like this: `{"name": "John", "age": 30, "city": "New York"}`. You can use `JSON.parse()` to convert this string into a JavaScript object that you can work with in your HTML code.
For your interest: Html to Json Parser Nextjs
What Is JSON
JSON is a lightweight data-interchange format that's quick and easy to parse and generate. It's perfect for exchanging data between a server and a client.
JSON is based on two basic structures: objects and arrays. An object is an unordered collection of key/value pairs, while an array is an ordered list of values.
Objects in JSON are defined by a left curly bracket { and end with a right curly bracket }. Multiple key/value pairs are separated by a comma ,. For example, an object might look like this: { "key": "value", "another key": "another value" }.
Property names or keys in JSON are always strings, while the value can be a string, number, true or false, null, or even an object or an array.
Strings in JSON must be enclosed in double quotes " and can contain escape characters such as
, \t, and \.
Here's a quick rundown of the basic JSON structures:
- Object: An unordered collection of key/value pairs.
- Array: An ordered list of values.
In JSON, values can be a string, number, true or false, null, or even an object or an array. This flexibility makes JSON a powerful tool for exchanging data between a server and a client.
Discover more: How to Display Json File in Html
Parsing JSON Data
Parsing JSON Data is a crucial step in working with JSON data in JavaScript. You can use the JSON.parse() method to convert a JSON string into a JavaScript object.
The JSON.parse() method is a built-in JavaScript function that parses a JSON string and constructs the JavaScript value or object described by the string. This method is useful when you receive JSON data from a web server and need to access individual values using the dot notation (.).
To parse JSON data, you can simply use the JSON.parse() method, like this: JSON.parse(json_string). This will convert the JSON string into a JavaScript object that you can work with.
For example, if you receive a JSON-encoded string from a web server, you can use the JSON.parse() method to convert it into a JavaScript object. The JSON-encoded string might look like this: {"name": "John", "age": 30}. You can then use the dot notation to access individual values, like this: object.name or object.age.
The JSON.parse() method is a powerful tool for working with JSON data in JavaScript, and it's essential to understand how to use it when working with JSON data.
A unique perspective: Running Json Nextjs
Displaying Data on an HTML Page
Displaying data on an HTML page is a crucial step in working with JSON data. To do this, you can use JavaScript's DOM to access the part of the website where you want to display the data.
You'll need to create a new file with a .js extension, such as script.js, to hold your JavaScript code. In this file, create an empty function and call it at the end of the file. This function will be used to fetch data from the JSON file and display it on the HTML page.
The fetch() function is used to fetch data from external files or resources, and it has a simple syntax: fetch() takes in the URL or path to the resource, followed by .then() blocks to return the response in the desired format and display the result. If something goes wrong, the .catch() block will run the specified code.
You can test for success using the console, which will display the data fetched from the JSON file. Once you have the data, you can use template literals to display it on the HTML page. Template literals allow you to use variables and add HTML tags to the string.
You might enjoy: Basic Html Email Template
To display the data, you'll need to access the part of the website where you want to display it, such as a div with an id of 'country'. Using the .innerHTML property, you can parse in the variable output where you created the list from the data fetched.
The code to display the data is straightforward: you grab the div with the id of 'country' and use the .innerHTML property to parse in the variable output. This will display the list of countries and their abbreviations on the HTML page.
Consider reading: Css Styling Id
Reading JSON Data
JSON stands for JavaScript Object Notation, a lightweight data-interchange format for data exchange between server and client. JSON is based on two basic structures: objects and arrays.
JSON data is represented in (key, value) pairs, where a unique key identifies each piece of data and its value can be of various types, including strings, numbers, arrays, objects, booleans, and null.
You can easily parse JSON data received from the web server using the JSON.parse() method in JavaScript. This method parses a JSON string and constructs the JavaScript value or object described by the string.
There are multiple methods for loading a JSON file in JavaScript, including using Require/Import modules, the Fetch API, and the FileReader API.
The Fetch API is a web API provided by modern browsers to fetch resources from the network, including JSON files. It returns a Promise that can be resolved to a response object and used as required.
Here are the methods for loading a JSON file in JavaScript:
- Using the Fetch API: `fetch('data.json')`
- Using the FileReader API: `new FileReader().readAsText('data.json')`
- Using Require/Import modules: `const data = require('./data.json')`
Make sure the file structure aligns with the specified path for successful retrieval of the JSON file.
The Fetch API returns a Promise that can be resolved to a response object and used as required. The response object contains the data from the JSON file, which can be parsed using the JSON.parse() method.
Here's an example of how to use the Fetch API to load a JSON file:
```javascript
fetch('data.json')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
```
This code snippet uses the Fetch API to retrieve the `data.json` file and parse it as JSON. The parsed data is then logged to the console.
Sources
- https://medium.com/@akshaykrdas001/how-to-fetch-data-from-local-json-file-and-render-it-to-html-document-with-using-vanilla-javascript-a0191a894f25
- https://spark.apache.org/docs/3.5.1/sql-data-sources-json.html
- https://chrisdevcode.hashnode.dev/how-to-display-json-data-on-an-html-page-using-vanilla-javascript
- https://oxylabs.io/blog/javascript-read-json-file
- https://www.tutorialrepublic.com/javascript-tutorial/javascript-json-parsing.php
Featured Images: pexels.com