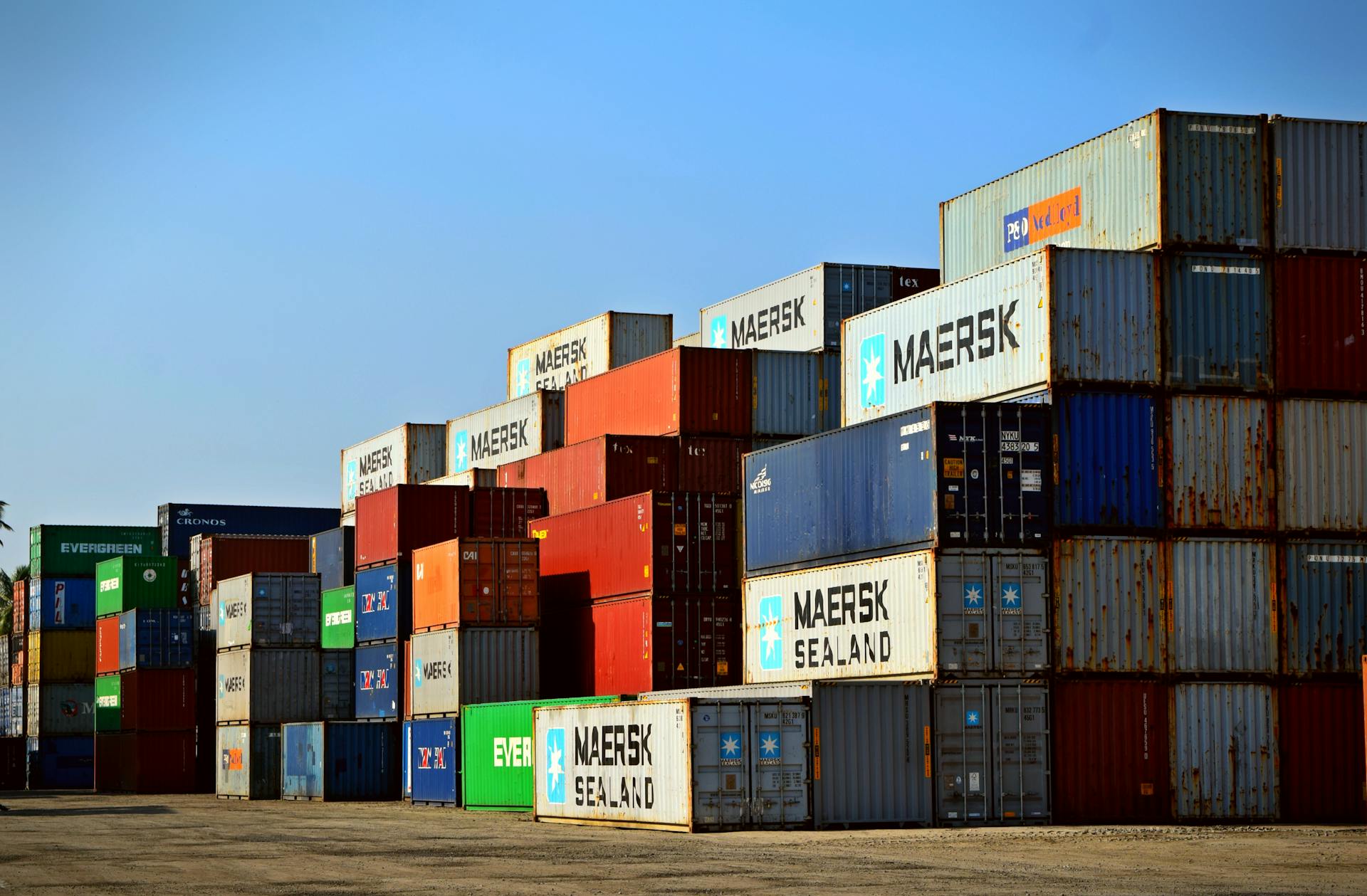
To set up a Next.js app on Docker, you'll need to create a Dockerfile that includes the necessary dependencies and configurations. This involves specifying the base image, copying the project files, and installing the required packages.
The official Next.js Docker image is not available, so we'll use the Node.js image as a base. In the example, the Dockerfile starts with `FROM node:14-alpine` to use the Node.js 14 image with Alpine Linux.
This choice of base image is significant because it helps keep the image size small, which is essential for efficient deployment. By using Alpine Linux, we can reduce the image size without sacrificing performance.
Readers also liked: Next.js
Setting Up the Project
To set up a Next.js project with Docker, start by creating a new directory for your project and navigating into it in your terminal.
The first step is to create a new Next.js project using the `npx create-next-app` command, which will create a basic file structure for you to work with.
Next, create a new file called `Dockerfile` in the root of your project directory, which will contain the instructions for building your Docker image.
Related reading: Next Js App Folder
Setting the Working Directory
The working directory is a crucial part of setting up your project. It's defined inside the container, which means you can call it whatever you want at this point.
For consistency, it's a good idea to set it as /app, but it doesn't need to match the name of the directory on your local machine. This allows for flexibility in your project's file structure.
You can think of the working directory as the root of your project, where all your files and folders will reside. It's a central location that makes it easy to navigate and manage your project's files.
Consider reading: Src Directory Nextjs
Install Dependencies
To install dependencies, start by creating a layer called deps using the package.json and package-lock.json files. This layer will contain our dependencies, which are less likely to change than the rest of our app.
Docker's caching ensures we don't have to reinstall dependencies every time we make a change to our app files. We can take advantage of this caching by copying over our dependencies first.
We'll use the package.json and package-lock.json files to install our dependencies. These files contain the list of dependencies our app needs to run.
We'll inject the NODE_ENV environment variable at image build time, setting it to production. This will prevent dev dependencies from being installed, as per the npm install docs.
A different take: Install Next Js 13
Node.js 20, Yarn 4
You can run a Next.js app in a Docker container using Docker Compose, which makes it easy to spin up the project with a single command: docker compose up. This command builds and runs the project as defined.
To build a Docker image, navigate to your project's root directory in the terminal and run the command docker build -t my-app . This command tells Docker to build an image based on the Dockerfile in the current directory and tag it with the name my-app.
Explore further: How to Run Next Js App
Configuring the App
To configure your Next.js app for a Docker deployment, you need to edit the next.config.js file. This file determines how your app is built and prepared for production.
Next.js can automatically create a standalone folder that copies only the necessary files for a production deployment, including all your node_modules. This makes it easier to manage and deploy your app.
Recommended read: How to Build Next Js App for Production
Writing an App File
Writing an App File is a crucial step in configuring your app. Our project's Dockerfile will be incredibly simple and standard.
You can create a Dockerfile in your project's root. This is where you'll define the configuration for your app. Consider using a lighter-weight Docker image for this, but for a classic Next.js app, one Dockerfile with limited config is all you'll need.
Keep in mind that a single-service app like ours requires minimal configuration.
Create the
Create the Dockerfile that describes how your image gets built. It will be placed on the root of your project and should look like this: FROM node:20-alpine.
We will divide our building process into multiple steps, allowing us to utilize Docker's caching mechanism. Our base image is node:20-alpine, which is relatively small in size.
Consider reading: Image Next Js
Defining the Service
You can define the Next.js service with a name relevant to the project. It's best practice to choose a name that makes sense.
To define the service, you'll need to create a Docker Compose file, which will specify the Docker image and other settings for the service.
The service name doesn't have to be exact, but it's a good idea to keep it concise and descriptive.
You can name the service whatever you like, but it's a good idea to stick with a consistent naming convention throughout your project.
See what others are reading: Nextjs Prisma Docker
Setting Build Context
Setting Build Context is a crucial step in configuring your app.
The build context defines the path that the Docker build has access to.
Setting the context to . will include your entire repository in the build, which is useful for local development.
You can also set the context to a specific directory, but this is less common.
By setting the build context correctly, you'll avoid errors caused by missing files or directories.
Discover more: Nextjs Usecontext
Setting Ports
You can access your app via localhost:3000, which is the default port.
The ports option maps the host port to the container port, allowing you to forward requests made to the host to the container. This is done by specifying the port number in the Docker Compose file.
To bind port 3000 to your container, you'll need to include the ports option in your Docker Compose file.
You can find the building details about your image by using the docker images command, which will show you the cached building process and the building logs.
Here's an interesting read: Nextjs Server Actions File Upload
Edit Next.config.js
To edit the Next.config.js file, you need to change the configuration of your project. This is necessary to build your Next.js app as a container.
Next.js can automatically create a standalone folder that copies only the necessary files for a production deployment including all your node_modules. This helps keep your build times efficient and reduces the risk of security issues.
Creating a .dockerignore file is also essential to exclude unnecessary files that can slow down your build times and pose security risks.
Suggestion: File Upload Next Js Supabase
Building and Running the Image
Building and Running the Image is a straightforward process. You can build the Docker image by navigating to your project's root directory in the terminal and running the command `docker build -t my-app .`.
To build the image, you'll need to specify the Dockerfile in the current directory using the `.` notation. If you have any build-time environment variables, you can pass them using the `--build-arg` flag. For example, if you want to set the `NODE_ENV` variable to `production`, you can run `docker build --build-arg NODE_ENV=production -t my-app .`.
Once the image is built, you can run the container by executing `docker run -p 3000:3000 my-app`. This will map port 3000 from the container to port 3000 on the host machine, allowing you to access your Next.js application on localhost:3000.
Here's an interesting read: How to Run Nextjs to Build
Running the App
To run your Next.js app, you'll need to launch the Docker daemon and use the `docker compose up` command from the app's root directory. This will build and run the project as defined, making it accessible on localhost:3000.
You can also use Docker Compose to streamline the process. This involves creating a `docker-compose.yml` file that specifies all the necessary parameters and settings for building and running your Docker container.
With Docker Compose, you only need to remember a single command to run your Next.js application. This makes it easier to manage and deploy your app.
To build and run your Next.js Docker image, navigate to your project's root directory and run the command: `docker build -t my-app .`. This will build an image based on the Dockerfile in the current directory and tag it with the name `my-app`.
If you have any build-time environment variables, you can pass them using the `--build-arg` flag.
Running your Next.js app with Docker provides several advantages, including consistent behavior across environments, easy deployment and scaling, portability, cost-effective infrastructure, and streamlined dependency management.
Here's a summary of the benefits:
- Consistent behavior across environments
- Easy deployment and scaling
- Portability
- Cost-effective infrastructure
- Streamlined dependency management
To start your container, you'll need to bind port 3000 to your container and spin up the image. You can do this by running the command: `docker run -p 3000:3000 my-app`.
Sources
Featured Images: pexels.com