
Nx is a powerful tool for Next Js app development and deployment. It allows you to manage multiple projects and generate boilerplate code with ease.
With Nx, you can create a single workspace that contains multiple Next Js applications, making it easier to manage and deploy your applications.
Nx provides a unified command line interface (CLI) for managing your Next Js applications, allowing you to run commands across multiple projects with a single command.
This streamlined workflow enables you to focus on building and deploying your applications without worrying about the underlying infrastructure.
Nx also provides a built-in support for popular deployment platforms like Vercel and Netlify, making it easy to deploy your Next Js applications with a single command.
Configuring Nx
Configuring Nx involves defining the main configuration files, which are workspace.json and nx.json. The workspace.json file defines the projects in the workspace, such as apps and libs, and the Nx executor used to run operations on these projects.
The workspace.json file is the main configuration file of an Nx workspace, and it also defines the Nx executor used to run operations on the projects.
To configure Nx, you'll also need to install the required Nx plugins, such as the NextJs and ESBuild plugins, which are essential for an Integrated Monorepo setup.
Install Required Plugins
Installing the right plugins is crucial for a smooth Nx experience. We'll be using Nx in an Integrated Monorepo setup, which relies on Nx plugins to handle most of the heavy lifting.
To get started, you'll need to install the NextJs plugin. This plugin will help you build and manage your NextJs applications.
Next, install the ESBuild plugin. ESBuild is a fast and efficient build tool that will help you compile your code quickly.
Config Files: Workspace.json
The workspace.json file is the main configuration file of an Nx workspace. It defines the projects in the workspace, such as apps and libs.
This file is essential for setting up your Nx workspace and is the foundation for all other configurations.
It determines which projects are included in the workspace and how they interact with each other.
For example, you can define multiple apps and libs within a single workspace.json file.
This allows for a high degree of flexibility and customization.
App Development
In app development, it's essential to consider how to load pages dynamically. This means we can't give them a static name like we did with the About page.
You can use the Nx Console VSCode plugin for a more user-friendly experience, especially if you're not a terminal kind of person.
To simplify things, we can generate a set of "pages" to work with, rather than querying the API or reading the file system for all the pages we want to render.
Fixing Build-Custom-Server Target
Fixing Build-Custom-Server Target can be a challenge, especially when you're working with a custom server build. This is why we need to configure the build-custom-server target using the @nx/esbuild nx plugin.
The @nx/esbuild plugin is installed in our workspace, and we'll call its init generator to install all the required dependencies. This plugin is similar to what @nx/node generated apps do for their build target.
We'll update our NextJS app's project.json to configure the build-custom-server target. This new build configuration will bundle all the imported libraries into a single file, resulting in smaller containers.
This configuration is perfect for deploying our application without needing the rest of our monorepo dependencies. We'll add the following to our nx.json to tell Nx to first build the project dependencies before building the custom server.
App Development
If you're not the terminal kind of person, you can also use the Nx Console VSCode plugin.
To create a dynamic blog, you'll need to load pages dynamically, which means you can't give them a static name like the About page (about.tsx) had.
If you want to render multiple pages, you'll need to read your file system or query the API for all the pages you want to render, but that's a topic for later.
To simplify things, you can generate a set of "pages" to work with.
Generating the About Page
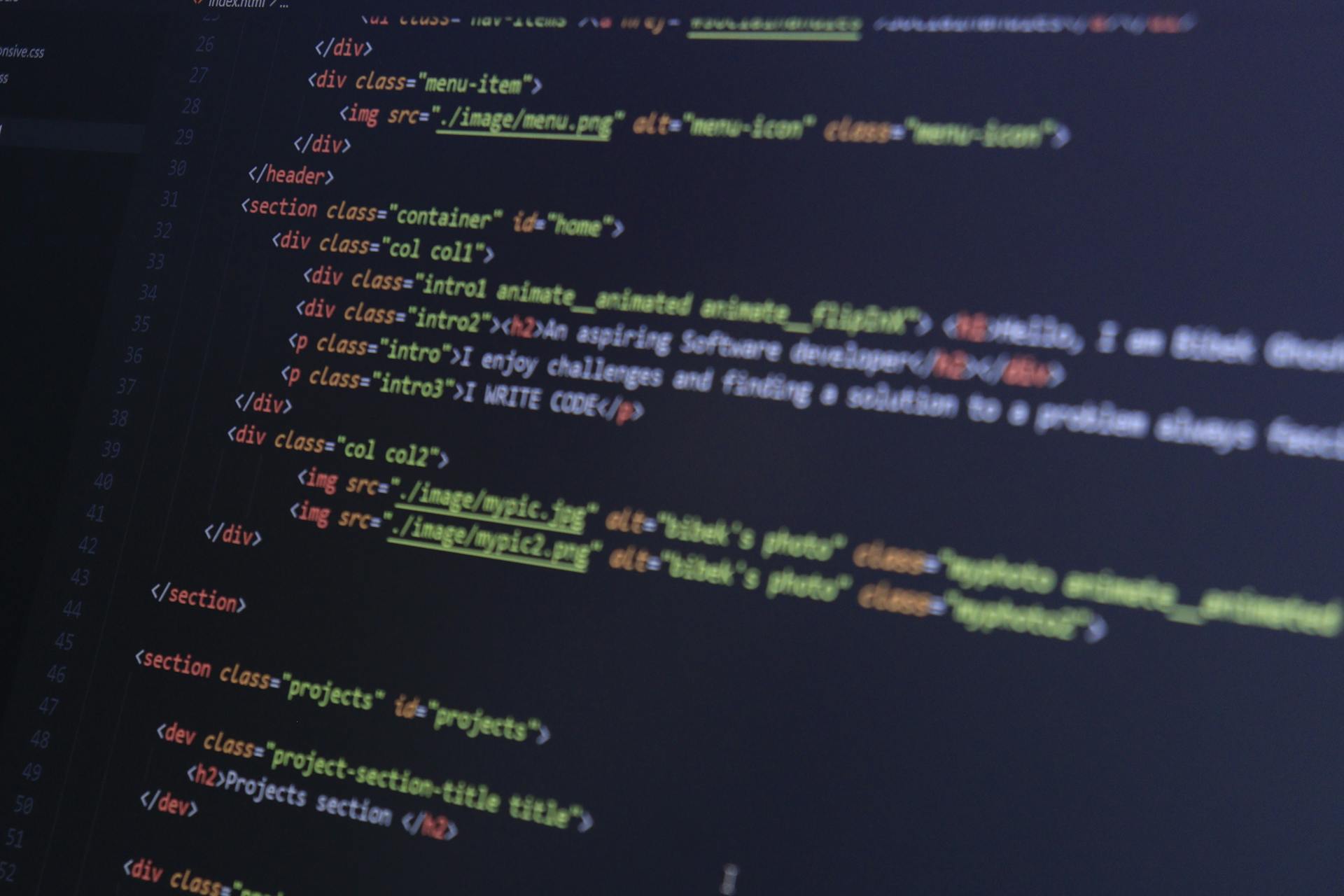
Generating the About Page is a straightforward process that can be accomplished with the help of Nx generators.
To create a new About page, you can type `nx g page about` in your terminal, which will generate a new `about.tsx` file along with its corresponding styling file.
The `about.tsx` file is where you'll write the React component that will render on the About page. This file is generated in the `pages` folder, which is where Next.js looks for files returning React components to generate new pages.
If you serve your app with `npx nx serve site` and navigate to `/about`, you should see the About page rendered correctly.
Understanding GetStaticProps
getStaticProps is a powerful tool in Next.js that allows us to return props to our React component that's going to be pre-rendered.
We can write our getStaticProps as follows, using TypeScript to type the return value of our function to match our AboutProps from the about.tsx component.
The returned params object can be accessed from within the getStaticProps and potentially remapped to something else.
We can process and convert markdown content to HTML here, making it a great place to further elaborate the content of our component.
By using getStaticProps, we can pre-render our React component and return props that are already processed and ready to use.
Build on Change
Building on change is a game-changer for app development. It allows you to only run certain commands when something in your codebase has changed, saving you time and resources.
Nx's "affected commands" feature is a powerful tool for this. It figures out which projects need to be updated based on your Git history and dependency graph.
You can use Vercel's ignored build step feature to only run your build if something has changed. It requires you to respond with an exit code of 1 if the build is required or 0 if it should be canceled.
To set up affected builds for Vercel, you'll need to create a script in the tools/vercel-deploy/vercel-affected-deploy.sh file. Copy the script from the Nx guide and adjust the APP variable to reflect your own app name.
Here are the key steps to set up affected builds:
- Copy the script from the Nx guide
- Place it in the tools/vercel-deploy/vercel-affected-deploy.sh file
- Adjust the APP variable to your app name
Make sure to set the base and head references in the script to HEAD~1 and HEAD respectively. This will compare the changes made from the last commit.
Note that it's not currently possible to get a reference to the last successful deployment commit SHA on Vercel. If that were possible, you could pass it to --base and get an even more efficient affected command evaluation.
Deployment
You can deploy your Next.js site as a set of static files using tools like Jekyll, Eleventy, or Hugo, which transform markdown files into static HTML files that can be served by any web server.
These tools are opinionated on how to structure your site, but for a simple portfolio website, you might not need a backend.
Next.js allows you to statically or dynamically render your site from the server, giving you the flexibility to decide when to use static rendering.
To deploy your site statically, you can run the command to generate a set of static HTML, CSS, and JS files at dist/apps/site/exported.
With a plain HTTP server that can serve static files, you can run the exported Next.js application.
You can also configure GitHub Pages to deploy your website directly from your GitHub repository, and even use CloudFlare to enable SSL.
To test this locally, you can run the command to quickly test the deployment.
For production deployment, you can use Vercel, which takes over and deploys your app successfully once you hit deploy.
Vercel fetches the source code, executes the build command, and deploys it into production, and you can see more information about the deployment on the dashboard.
You can also go to the deployment URL and see the site live.
To configure Nx affected deploys on Vercel, you need to add the script to the Ignored Build Step section.
When you visualize the dependency graph with nx dep-graph, you should see the vercel-affected-deploy.sh script being used.
If you make a change in your shared-ui library, which your React application and Next.js site application depend on, a deployment would be triggered.
To set up your project on Vercel, you need to go to the platform, create an account, and choose "Create a New Project".
You can configure the project, giving it a name to identify it in your Vercel dashboard later.
Before deploying, you need to configure the "Build and Output Settings" to use the underlying Nx build commands.
To build your Next.js app for Vercel, you can run the build command, which generates the output in the dist/apps/site directory.
You can customize the output path in the project configuration of your application workspace.json.
Building and Exporting
Building and Exporting your Next.js application with Nx is a breeze. You have two main options: build and export.
The build option generates an optimized bundle that can be served by the next CLI, ideal for deploying to Vercel infrastructure. It requires a Node environment that can run the application.
You can invoke the build command using `nx build site` or `nx build site --configuration=production` to use the production configuration.
The export option generates a static site out of your Next.js application, perfect for serving from a static CDN without a Node environment.
You can use `nx export site` to export your application, which will automatically build the Next.js app first.
If you need to customize the output path, you can do so in the project configuration of your application workspace.json.
Here are the main differences between the build and export options:
Sources
- https://blog.nrwl.io/create-a-next-js-web-app-with-nx-bcf2ab54613
- https://dev.to/sebastiandg7/nx-nextjs-docker-the-nx-way-creating-the-nextjs-application-1efl
- https://juri.dev/blog/2021/09/nextjs-deploy-vercel-with-nx/
- https://nextjs.org/docs/pages/api-reference/next-config-js/output
- https://docs.sentry.io/platforms/javascript/guides/nextjs/manual-setup/
Featured Images: pexels.com