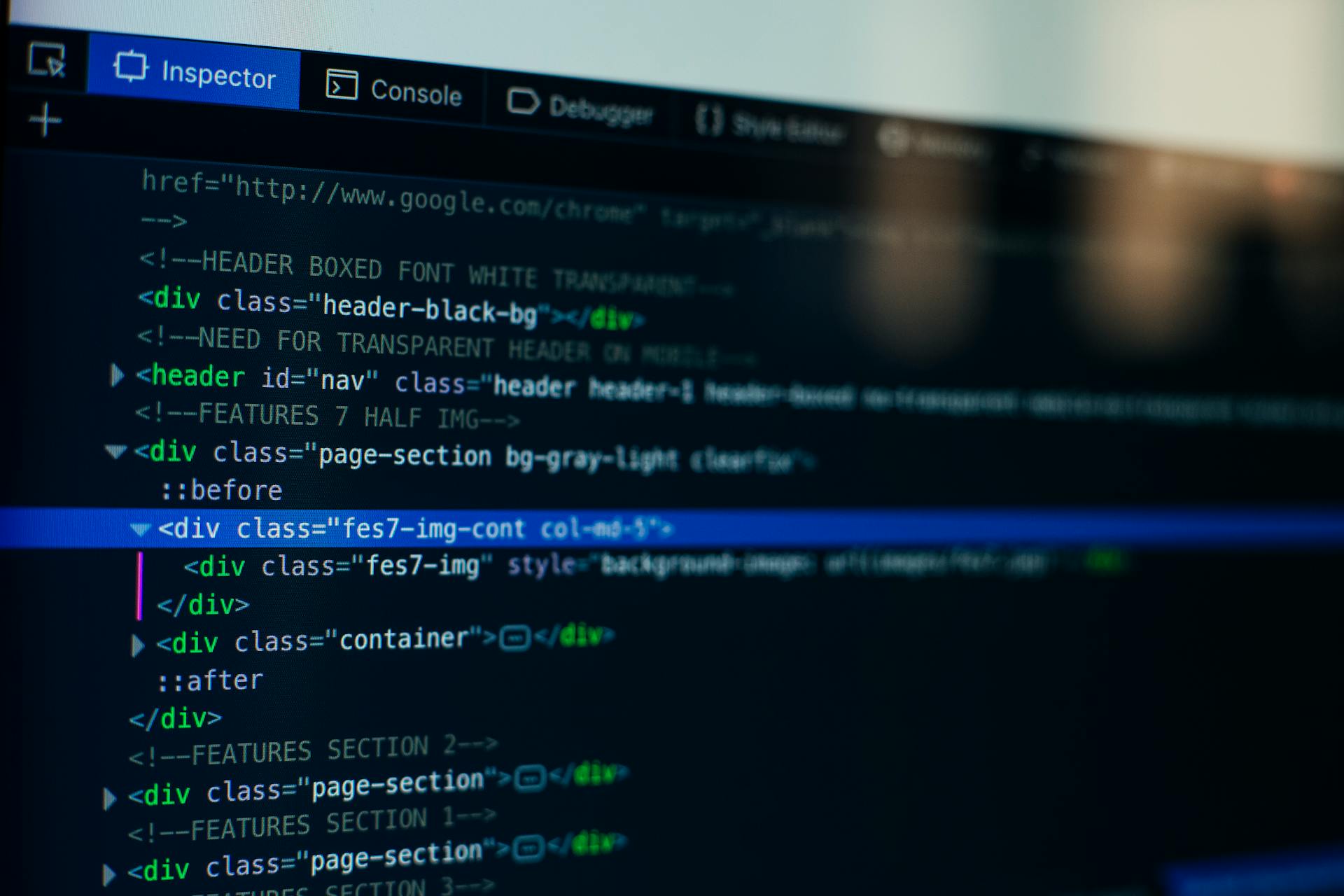
GetInitialProps is a powerful method in Next.js that allows you to pre-render your pages on the server, improving performance and SEO. It's a key feature that sets Next.js apart from other frameworks.
By using GetInitialProps, you can fetch data on the server before sending it to the client, reducing the amount of data transferred and improving page load times. This is especially useful for pages that require a lot of data to be fetched.
To get the most out of GetInitialProps, you need to understand how it works and how to use it effectively. This means learning about the different types of data that can be passed to GetInitialProps and how to handle errors and caching.
If this caught your attention, see: Nextjs App Route Get Ssr Data
GetInitialProps
GetInitialProps is a static method in Next.js that's automatically called when a page is loaded. It's used to pre-render data on the server-side, which can enhance application load time and user experience.
The getInitialProps method receives only one argument called context, which is an object with various properties. These properties include the pathname, query, asPath, req, res, and err, which provide information about the current route, URL, and HTTP request. The context object is available in both server and client environments.
For more insights, see: Nextjs Usecontext
The properties in the context object are:
Syntax
The syntax of `getInitialProps` is quite straightforward. It receives only one argument called `context`, which is an object with several properties.
This object has the following properties:
Note that `getInitialProps` is a static method, so it's defined as a property of the Page component instead of a method within it. This method is automatically called by Next.js when the page is loaded and it populates the props of the component before rendering it.
Next.js 13
Next.js 13 still supports getInitialProps for backward compatibility, which is great news for developers working on legacy projects.
This means that if you're working on a project that was built using older versions of Next.js and heavily relies on getInitialProps, it might be more practical to continue using it rather than rewriting everything.
If your data fetching logic is complex and can't be easily achieved with getStaticProps or getServerSideProps, you might continue using getInitialProps.
Here are some scenarios where getInitialProps might still be necessary:
- Complex data fetching logic
- Custom SSR logic
- Legacy projects
This is because transitioning all existing projects to the newer methods might be impractical in some cases.
GetServerSideProps
GetServerSideProps is a method in Next.js that allows you to fetch data on the server-side and pre-render the page with the fetched data before sending it to the client. This is especially useful for pages that require dynamic data or data that changes frequently.
You can use getServerSideProps for content with dynamic data or personalized data, content that requires real-time data or live updates, and content that varies per user or request. It's also a good choice for content that needs to be updated frequently.
Here's a summary of when to use getServerSideProps:
Context Parameter Table
The Context Parameter Table is a crucial part of the getServerSideProps method in Next.js. It provides valuable information about the current page request.
Here are the context parameters available in the getServerSideProps method:
These context parameters can be accessed in the getServerSideProps method to fetch data on the server-side.
When to Use GetServerSideProps
So you're wondering when to use getServerSideProps? Well, let's take a look at some scenarios where it's a great choice.
Use getServerSideProps when you need to fetch content with dynamic data or personalized data. This includes content that requires real-time data or live updates, as well as content that varies per user or request. It's also a good idea to use getServerSideProps when content needs to be updated frequently.
On the other hand, you should avoid using getServerSideProps for content with static data that should be pre-rendered for performance, or for content that needs to achieve excellent SEO without data fetching on the client-side.
Here's a quick rundown of when to use getServerSideProps:
By using getServerSideProps in these scenarios, you can ensure that your content is delivered quickly and efficiently to your users.
GetStaticProps
GetStaticProps is a powerful data fetching method in Next.js that allows you to pre-render your pages at build time. It's a great alternative to getServerSideProps, especially for pages that don't need to be updated frequently.
To use GetStaticProps, you need to replace getInitialProps with getStaticProps in your page components. The structure of getStaticProps is different from getInitialProps, and it returns an object with the props key containing the fetched data.
For your interest: Nextjs Pages
You can also include the revalidate option in the returned object to specify how often the data should be revalidated and regenerated. This is useful when you want to update the data periodically without redeploying your application.
Here's a brief overview of the steps involved in migrating to GetStaticProps:
- Remove getInitialProps: If your page components use getInitialProps, you need to remove it from those components.
- Replace getInitialProps with getStaticProps: Replace the getInitialProps method with the getStaticProps method in your page components.
- Data revalidation (optional): With getStaticProps, you can also include the revalidate option in the returned object.
Understanding GetInitialProps
GetInitialProps is a method used in older versions of Next.js to fetch data on the server-side before rendering a page. It was the primary data fetching method used in Next.js before newer data fetching methods like getServerSideProps and getStaticProps were introduced.
GetInitialProps is invoked only on the initial request for a page, including client navigation, and focuses on fetching static data that remains consistent for all users accessing the same page. This makes it more suited for fetching data that is shared across all users, such as blog posts, product listings, or common configuration settings.
Here's a table summarizing the differences between getInitialProps and getServerSideProps:
This table highlights the key differences between the two methods, making it easier to choose the right one for your application's needs.
Return Value
The return value of getServerSideProps is a crucial part of the process. It must return an object with specific properties.
getServerSideProps can return an object with three properties: props, notFound, and redirect. The props property is an object key-value pair passed to the page component, which should be a serializable object that can be serialized with JSON.stringify.
A boolean object notFound allows the page to return a 404 status and 404 Page. If notFound is set to true, the page will return a 404 error even if the page was previously generated successfully.
The redirect property is an object with the structure { destination: string, permanent: boolean }. It allows redirecting to internal and external resources.
Here's a breakdown of the return value properties:
- props: An object key-value pair passed to the page component.
- notFound: A boolean object that returns a 404 status and 404 Page.
- redirect: An object with the structure { destination: string, permanent: boolean }.
Props Definition
Props Definition is a crucial aspect of understanding getInitialProps. getInitialProps must return an object with any of the following properties: props, notFound, or redirect. This object is used to pass data to the page component.
Discover more: Next Js Props
The props property is an object key-value pair that can be serialized with JSON.stringify. It's used to pass dynamic data to the page component. The notFound property is a boolean object that allows the page to return a 404 status and 404 Page. If notFound is set to true, the page will return a 404 error even if the page was previously generated successfully.
The redirect property is an object with the following structure: { destination: string, permanent: boolean }. It allows redirecting to internal and external resources. The destination property specifies the URL to redirect to, and the permanent property specifies whether the redirect should be permanent or not.
Here's a summary of the props definition:
The props definition is essential for understanding how getInitialProps works. By returning the correct props object, you can ensure that your page component receives the necessary data to function correctly.
Best Practices
In Next.js, it's essential to use getInitialProps for server-side rendering (SSR) and static site generation (SSG) to fetch data before rendering the page. This ensures a smooth user experience, especially for complex pages that require data loading.
Curious to learn more? Check out: Nextjs Server Rendering Tailwind
To use getInitialProps effectively, make sure to return an object with the props, as seen in the example: `static async getInitialProps({ req }) { return { message: 'Hello World' }; }`. This allows Next.js to render the page with the fetched data.
Use getInitialProps only in pages that require data fetching, as it can slow down pages that don't need it.
For another approach, see: Nextjs App vs Pages
Wrapping Up in Next.js
As you continue to build with Next.js, it's essential to understand the limitations of the getInitialProps method. This legacy API can increase server load and impact performance due to repeated data fetching on each request.
The getInitialProps method can also lead to potential race conditions if multiple clients request the same page simultaneously. This is because data fetching may occur concurrently, resulting in inconsistent data.
Adding a getInitialProps method can make your components more complex and less maintainable, increasing the overall complexity of your application.
However, the getInitialProps method still has its use cases, especially when dealing with data that is consistent across users or pages. For example, it can be used to pre-render data on the server-side, enhancing application load time and user experience.
Curious to learn more? Check out: Next Js Spa
To optimize your web application's performance and SEO, it's crucial to understand the differences between getInitialProps, getServerSideProps, and getStaticProps. Here's a brief comparison:
By choosing the right method for your application's specific needs, you can ensure optimal performance and user experience.
Migrating to GetStaticProps
Migrating to GetStaticProps involves removing getInitialProps from your page components and replacing it with getStaticProps. This new method returns an object with the props key containing the fetched data.
To start, remove getInitialProps from your page components. This is a crucial step, as getStaticProps and getInitialProps have different structures and requirements.
Next, replace getInitialProps with getStaticProps in your page components. The structure of getStaticProps is different from getInitialProps, so make sure to update your code accordingly.
With getStaticProps, you can also include the revalidate option in the returned object to specify how often the data should be revalidated and regenerated. This is useful when you want to update the data periodically without redeploying your application.
Here's a quick summary of the steps involved in migrating to getStaticProps:
- Remove getInitialProps
- Replace getInitialProps with getStaticProps
- Include the revalidate option (optional)
Frequently Asked Questions
How to replace getInitialProps?
To replace getInitialProps in Next.js, use getStaticProps or getServerSideProps to fetch data from external APIs or databases. This approach provides better performance and flexibility for rendering data on the page.
Sources
- https://joelolawanle.com/blog/data-fectching-nextjs-14
- https://www.geeksforgeeks.org/next-js-getinitialprops/
- https://refine.dev/blog/next-js-getinitialprops-and-getserversideprops/
- https://blogs.purecode.ai/blogs/nextjs-getinitialprops/
- https://blog.logrocket.com/data-fetching-next-js-getserversideprops-getstaticprops/
Featured Images: pexels.com