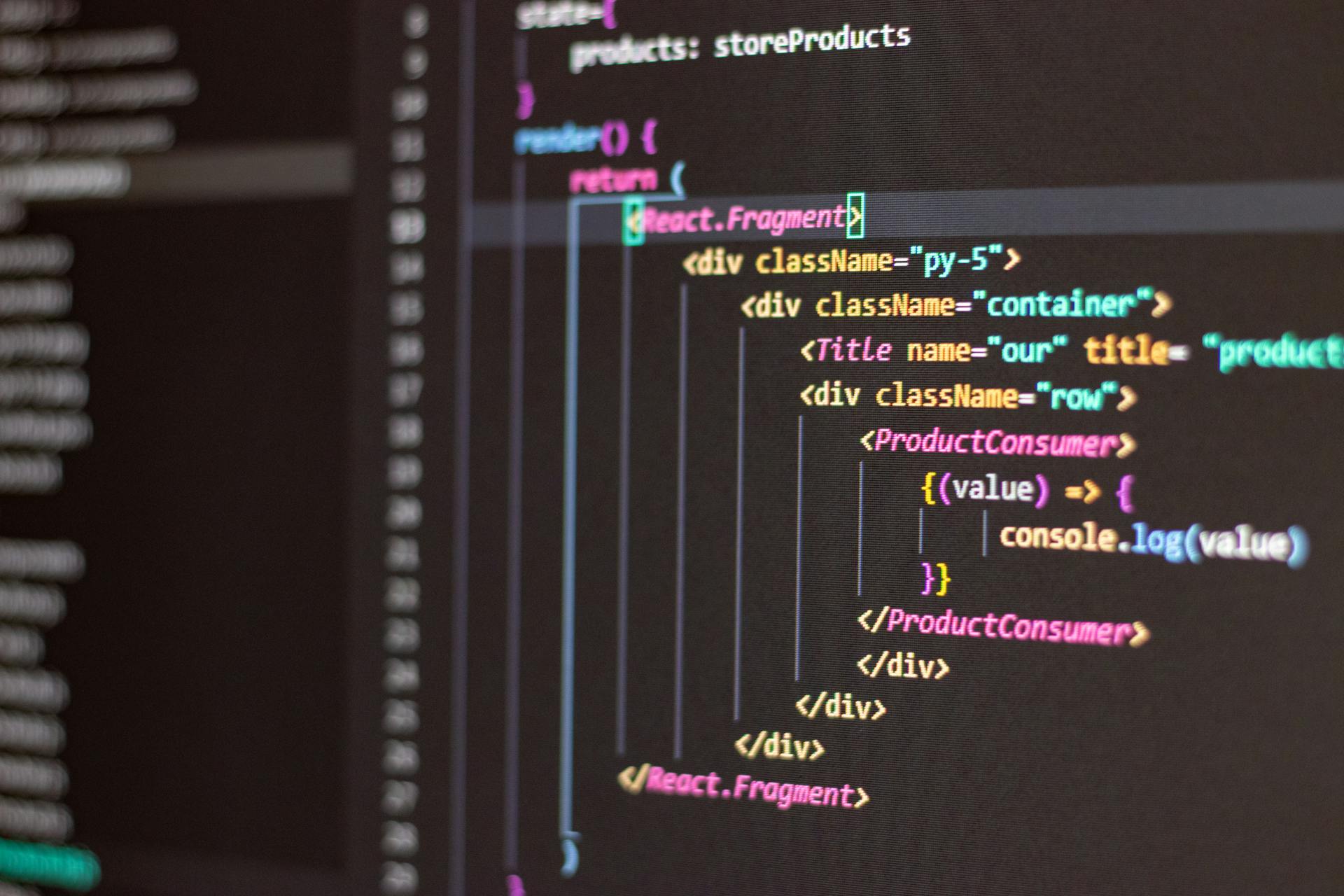
Session storage in Next.js can get out of hand if not managed properly. This is because Next.js doesn't automatically clear session storage when the user navigates away from a page.
As we discussed earlier, session storage can grow rapidly, leading to performance issues and difficulties in debugging. Clearing session storage regularly can help mitigate these problems.
By clearing session storage, you can improve the overall performance and management of your Next.js application. This is especially important for applications with a large number of users or those that rely heavily on client-side storage.
Recommended read: Save Api Data on Local Storage Next Js
Impact on User Experience
Session storage has a significant impact on user experience. A well-managed session can make a huge difference in how users interact with your application.
Personalized experiences are a key benefit of sessions. Sessions allow websites to display a user's name and preferences throughout the site, making the user feel more connected to the application.
Security measures are also crucial for a good user experience. Sessions can be used to automatically log out inactive users, reducing the system's exposure to data breaches and giving users peace of mind.
Convenience is another area where sessions shine. Sessions can remember shopping cart contents between pages of a user, making it easier for them to complete their purchase.
Here are some key benefits of sessions on user experience:
- Personalized experience
- Security measures
- Convenience
Session Storage Performance
Session Storage Performance can be a major bottleneck in your Next.js application, especially as the number of users and sessions increases. Storage requirements for session data can become significant, leading to performance issues.
To address this challenge, consider using optimized storage solutions like Redis or Memcached. These solutions can help reduce the load on your server and improve overall performance.
One technique to reduce storage requirements is to implement session expiration. By setting a time limit for inactive sessions, you can remove them from storage and free up space. This can be especially effective for users who abandon their sessions after a short period.
Another technique is garbage collection, which involves regularly cleaning up unused session data. This can be done manually or automatically, depending on your application's needs.
Here are some optimization techniques to consider:
By implementing these techniques, you can ensure that your server-side session management is secure, efficient, and scalable.
Session Management
Session management is crucial for Next.js applications, and it's essential to implement it correctly to avoid security risks and performance issues. Server-side session management is particularly important, as it helps prevent session fixation and hijacking attacks.
To ensure secure session management, implement HTTPS to encrypt session data and set the Secure flag on session cookies. Regenerating session IDs after login is also a good practice. Secure cookie storage is another essential aspect of session management.
Here are some key considerations for session management:
- Prevent session fixation and hijacking attacks
- Optimize storage solutions and implement techniques like session expiration and garbage collection
Redis is a popular choice for session storage due to its advantages, including data architecture, data expiry, low latency, and scalability. It's also less vulnerable to user tampering and requires less data to be transferred between the client and server.
A different take: What Is Azure Storage
Server-Side Management
Server-Side Management is crucial for building secure and performant Next.js applications. It's the backbone of session management, and getting it right is essential.
To implement server-side session management, you need to choose a suitable library based on your project's requirements. This will help you tailor your session management to fit your specific needs.
Expand your knowledge: Azure Storage Lifecycle Management
Implementing HTTPS is a must to encrypt session data. This ensures that even if an attacker intercepts your session data, they won't be able to read it.
Setting the Secure flag on session cookies is another crucial step. This flag indicates to the browser that the cookie should only be sent over a secure connection.
Regenerating session IDs after login is a good practice to prevent session fixation and hijacking attacks.
Here are some best practices to keep in mind when implementing server-side session management:
- Use secure cookie storage to protect your session data.
- Optimize storage solutions to improve performance.
By following these guidelines, you can ensure that your server-side session management is secure, performant, and tailored to your project's needs.
Redis for Session Management
Redis is an excellent choice for session management due to its in-memory key-value store structure. This allows for fast and efficient storage of session data.
One of the key advantages of Redis is its data architecture, which is perfectly suited for storing session data in a key-value format. This makes it easy to maintain all session data, such as username and user preferences, in a structured way.
Redis also has a built-in functionality to delete expired data, which helps web applications keep user sessions time-bound. This is a significant advantage over other storage solutions that require manual cleanup.
Another benefit of Redis is its low latency, making it extremely fast and suitable for user sessions and cache data. This is due to its in-memory nature, which allows for quick access to data.
Redis is also highly scalable, built to handle large volumes of data and high-throughput traffic. This makes it an ideal choice for web applications with a large number of users and sessions.
Here are some key advantages of using Redis over client-side cookies:
- Less vulnerable to user tampering: Cookies are visible to users and can be manipulated, while Redis protects user data from being tampered with.
- Less data transferred between client and server: With Redis, we only need to store the sessionId on the client machine, reducing the amount of data transferred.
- Storing only "SessionId" in client machine: This makes it easier to manage sessions and reduces the risk of data corruption or loss.
Frequently Asked Questions
What clears session storage?
Clearing session storage removes all items stored in it, and can be done using the clear() method on a sessionStorage object
Sources
- https://docs.amplify.aws/gen1/nextjs/build-a-backend/auth/manage-user-session/
- https://www.cclsolutionsgroup.com/post/chromium-session-storage-and-local-storage
- https://articles.wesionary.team/securing-sensitive-data-in-a-next-js-application-d7d5cce67f23
- https://nextjsstarter.com/blog/nextjs-13-server-side-session-management-guide/
- https://upstash.com/blog/session-management-nextjs
Featured Images: pexels.com