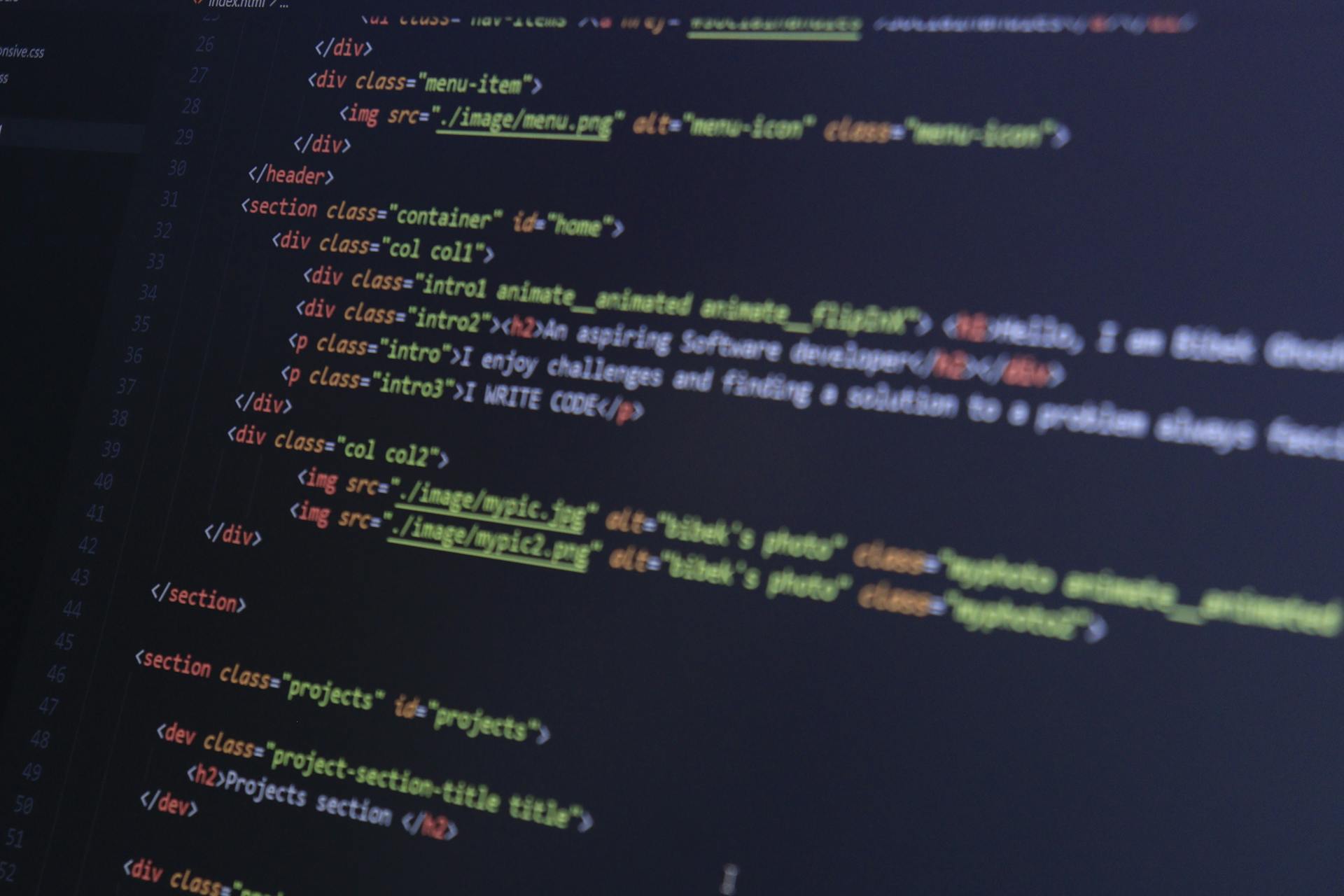
Using Next JS with Redis allows you to manage data efficiently and scale your application with ease. Next JS is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications.
By integrating Redis with Next JS, you can leverage Redis's in-memory data store capabilities to improve performance and reduce latency.
Redis can be used as a caching layer to reduce the number of database queries, resulting in faster page loads and improved user experience.
A different take: Next Js Build Collecting Page Data
Server Setup
To set up a Redis server, you can install it on a local machine or a cloud server. You can download the Redis binary from the official website and extract it to a directory of your choice.
Once you've extracted the binary, you can start Redis by running the redis-server executable. This will make Redis accept connections on the default port (6379).
To install Redis on a cloud server, you can use a cloud provider's managed Redis service, which can be easily provisioned and scaled. However, this may come with additional costs.
Recommended read: Install Nextjs
Alternatively, you can choose to install Redis manually on a cloud server using a package manager like apt-get or yum, or by building Redis from source.
Once you have a running Redis server, you can connect to it using the Redis Next.js library. This library provides several methods for creating connections to Redis servers, and it also provides options for configuring the connection, such as setting the Redis server host and port.
In a Next.js application, you would normally add your environment variables to a .env file, which would include the Redis server configuration.
Suggestion: Nextjs Loading Indicator Server
Data Management
In Next.js, managing data is crucial for a smooth user experience. Upstash Redis is a great option for handling the Redis database.
To save data to Redis, you'll need to use the Upstash Redis REST API, which is perfect for interacting with the Redis database inside your middleware. This is because making a REST API request is less prone to bugs compared to using a Redis client connection.
Check this out: Next Js Cache
Creating a new database in Upstash Redis is a straightforward process that can be done by navigating to console.upstash.com and signing up or logging in to your Upstash account.
Once you've created the database, you'll need to initialise a Redis REST client using the @upstash/redis npm package. This can be done by copying the code snippet from the Upstash Redis REST API section and pasting it into your lib/redis.js file.
The lib/redis.js file should then be exported with a Redis REST client that interacts with the REST API. This client can be imported into your _middleware.js file, where you can use it to save data to Redis.
To save log data to Redis, you'll need to add a code snippet to your _middleware.js file that pushes the logData object to a list called "api-request-log" using the REST API of Upstash Redis. This will effectively save your data to Redis.
Additional reading: Next Js Fetch Data save in Context and Next Route
Middleware and Caching
Middleware is a crucial part of a Next.js Serverless API, and we'll start by creating a middleware that gets information from the request and formats it to the logData object.
Expand your knowledge: Nextjs Middleware App Router
To create the middleware, navigate to the /pages/api folder and create a new file called _middleware.js. This file will act as a middleware, and its name is essential for Next.js to recognize it.
The middleware will get information from the request and store it to the logData object, which we'll then save to Redis. To test it, start your server with yarn dev, open localhost:3000/api/hello in the browser, and check out your terminal to see the request information logged to the console.
Redis is an in-memory cache that's perfect for scaling high-traffic websites due to its speed. We'll use Redis to cache payloads from expensive computations, prevent API servers from being overloaded, store low-ttl values, share temporary data, and even limit requests by IP for security reasons.
Here are some common caching use-cases for Redis in a Next.js API:
- Caching payloads from expensive computations
- Preventing API servers from being overloaded
- Storing low-ttl values
- Sharing temporary data
- Limiting requests by IP for security reasons
To use Redis in a Next.js API endpoint, we can initialize Redis and import it into any of our API endpoints. We'll create a key using the request's body to retrieve requests with the same payload, try getting a cached value, fetch data from the database if not cached, cache the value using the key and fresh data, and return the data to the client.
Client Setup
To set up a Redis client in your Next.js application, start by installing ioredis, the most famous Redis client for Node.js.
ioredis is a popular choice for its reliability and ease of use. You can install it using npm or yarn.
Once installed, create a function to initialize Redis, which you can export as createRedisInstance. This function will serve as the entry point for interacting with Redis in your application.
With ioredis up and running, you're ready to connect it to your Next.js application.
API and Deployment
Deploying your Next.js app with Redis Cloud on Vercel is a straightforward process. You'll need to add Redis as a store in the Vercel UI, which will automatically set your REDIS_URL environment variable.
To set up authentication providers, use the URL of your deployed app. You can find detailed instructions in the Configuration section.
You can choose from GitHub and Discord authentication providers, and only one is required.
Explore further: Next Js App Router Tailwind Spinner Loading Page
API Endpoint Usage
API endpoints are the backbone of a serverless API like Next.js. They're the entry points for clients to interact with your application.
To use Redis in a Next.js API endpoint, you first need to initialize the Redis client. This is a crucial step, as it allows you to store and retrieve data efficiently.
You can import the Redis client into any API endpoint, making it easily accessible for use. Assuming you want to cache a request to your database for 1 hour, you can use the following steps:
- You initialize Redis
- You create a key using the request's body
- You try getting a cached value, and if it's there, you return it to the client
- If not, you fetch the data from the database
- You cache the value using the key and the fresh data
- You return the data to the client
This approach is necessary because serverless APIs don't store data in between executions, making caching a common practice.
Deploy with Vercel
To deploy your application with Vercel, add Redis as a store in the Vercel UI, which will automatically set your REDIS_URL environment variable.
You can deploy your application with Vercel using Redis Cloud. To do this, follow the steps outlined below:
- Add Redis as a store in the Vercel UI.
- Once deployed, use the URL of your deployed app to set up the authentication providers.
By default, the template is configured to use GitHub and Discord, but only one provider is required.
Sources
- https://www.tuomokankaanpaa.com/blog/nextjs-middleware-save-data-to-redis
- https://makerkit.dev/blog/tutorials/nextjs-redis
- https://dev.to/rafalsz/scaling-nextjs-with-redis-cache-handler-55lh
- https://github.com/redis-developer/session-store-nextjs
- https://generalscience.io/articles/pub-sub-system-redis-nextjs
Featured Images: pexels.com