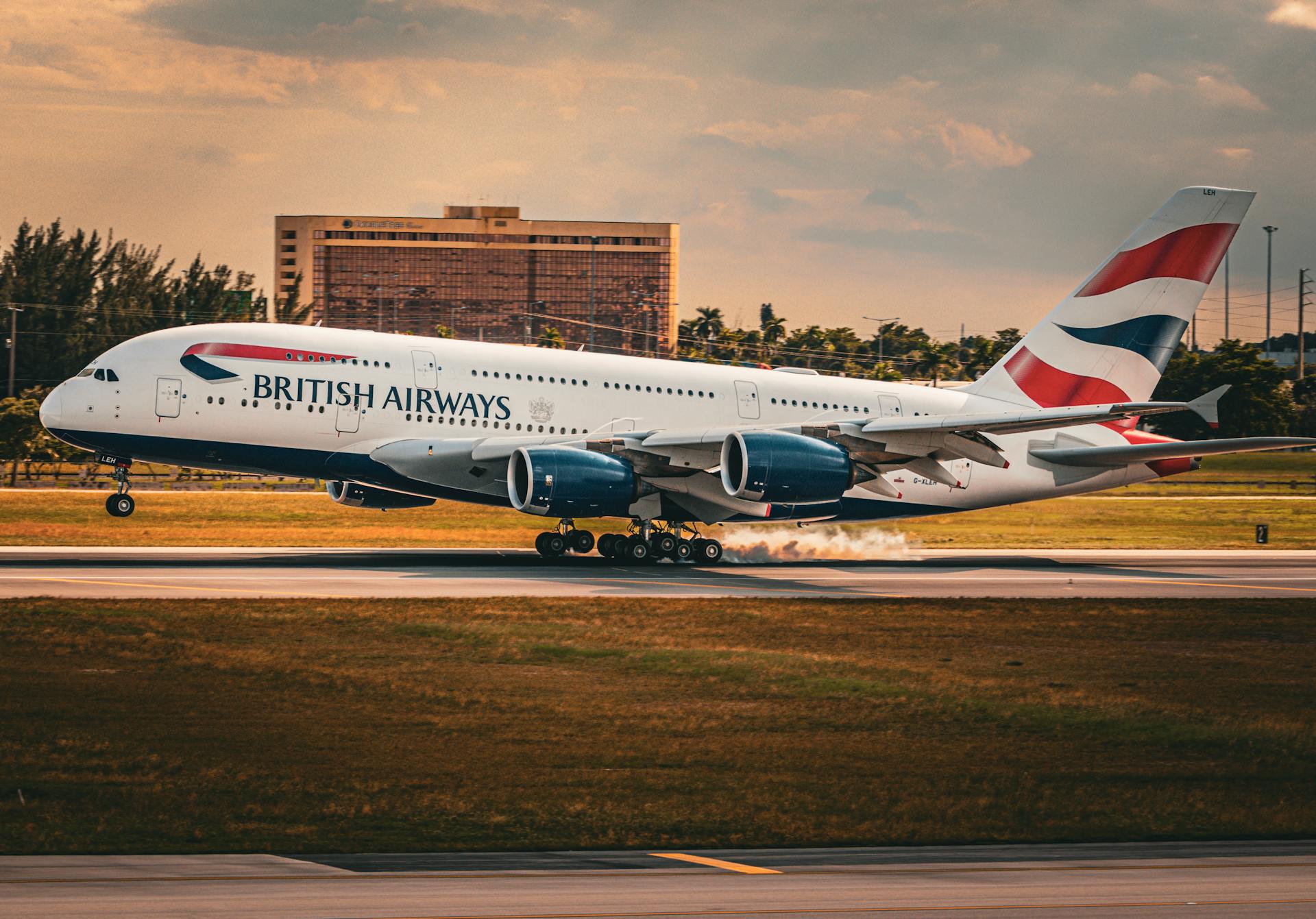
Customizing fonts in your Next.js project with Tailwind CSS is a great way to add a personal touch to your application.
You can create a custom font by adding a new entry to the `fonts` object in your `tailwind.config.js` file.
Tailwind CSS supports various font formats, including WOFF, WOFF2, and TTF.
To use a custom font, you'll need to import it in your CSS file using the `@font-face` rule.
Setting Up Custom Fonts
To set up custom fonts in your Next.js app, you can use the next/font/google package to import fonts from Google Fonts. This allows you to easily add multiple fonts to your app, such as Open Sans and Roboto Mono.
You can import your fonts and load them with the variable option to define the CSS variable name and assign it to a variable, like openSans and robotoMono.
To make your fonts available to your entire app, you can attach the CSS variables to your HTML document using {${openSans.variable} ${robotoMono.variable}}.
Here's a step-by-step guide to setting up custom fonts:
- Import your fonts from next/font/google
- Load your fonts with the variable option
- Attach the CSS variables to your HTML document
For example, you can add the following code to your Next.js app to import Open Sans and Roboto Mono fonts:
```html
import { Open_Sans, Roboto_Mono } from 'next/font/google';
```
Then, you can load the fonts with the variable option like this:
```html
const openSans = Open_Sans({
variable: 'openSans',
});
const robotoMono = Roboto_Mono({
variable: 'robotoMono',
});
```
Finally, you can attach the CSS variables to your HTML document like this:
```html
{${openSans.variable} ${robotoMono.variable}}
```
By following these steps, you can easily set up custom fonts in your Next.js app using next/font and Tailwind CSS.
Adding Fonts to Components
To add fonts to components, you can use the next/font package and Tailwind CSS. This is especially useful when you want to use a specific font on a particular component, like a header or a button.
You can create a new component, such as a Header component, and apply a font to it by importing the font as a variable and adding the font-mono class to the component's CSS. This will allow you to use the custom font on that specific component.
By following these steps, you can easily use your custom fonts throughout your Next.js app using Tailwind's utility classes. This is a typical requirement for most web projects, and with next/font, you can load fonts from Google Fonts or use local font files.
Applying a Style to a Component
To apply a style to a component, you can use the Tailwind CSS classes. For example, you can add the font-mono class to your component to apply a custom font.
You can see this in action by adding the font-mono class to your Header.js file. This will apply the custom font to your component.
To display your component, you can update your page.js file to import and display your new component. This will allow you to see the changes you made to your component.
Now, if you inspect your component, you can see that it is using the global Open Sans font. To fix this, you can add the font-mono class to your component's className.
By doing this, you can see that your page displays both fonts. The header is using the Roboto Mono font, and the paragraph element is using the Open Sans font.
This is a great way to customize the look and feel of your components without having to write a lot of code.
Add Inter Family
You can use the Inter font family in your project by adding it via the CDN. First, add the Inter font to your Next.js site using the next/font package.
To do this, add the Inter font to your Tailwind config by adding "InterVariable" to your "sans" font family. This will make the Inter font available for use in your project.
Here's how to do it:
- Add the Inter font to your Next.js site using the next/font package.
- Add "InterVariable" to your "sans" font family in your Tailwind config.
This will make the Inter font available for use in your project.
Customizing Fonts
You can extend your code to be used with Tailwind CSS by configuring Open Sans to output a CSS variable, also known as a CSS custom property. This allows you to reference it easily through Tailwind CSS classes.
Import your fonts from next/font/google, and load them with the variable option to define the CSS variable name and assign it to a variable. To attach the CSS variables to your HTML document, use {${variable} variable} rather than {variable.className}.
You can also add the Inter font family, which is a beautiful and open-source font that's great for UI design. To use Inter, add it via the CDN and then add "InterVariable" to your "sans" font family in your Tailwind config.
After loading custom fonts, generate CSS variables for each font. Then, pass the generated variables into the wrapper class in your _app.tsx component to make them available to any child of that element in the DOM.
Here's a step-by-step guide to customizing fonts in your Next.js app:
1. Import your fonts from next/font/google
2. Load your fonts with the variable option
3. Attach the CSS variables to your HTML document
4. Add the Inter font family (if desired)
5. Generate CSS variables for each font
6. Pass the generated variables into the wrapper class
By following these steps, you can customize your fonts and make them available to any child of the App component, essentially all pages and components on your site.
Optimizing Fonts
Using font-display: auto can lead to layout shift due to FOUT or FOIT.
To prevent this, use the optional value, which doesn't allow a swap in fonts once the page is loaded.
The optional value ensures that your custom web font doesn't swap with a different font after the page has loaded, avoiding the risk of layout shift.
However, there's a potential downside to using optional: if the font loading isn't optimized or the network is slow, your custom font might not show up at all.
To use font-display: swap, you'll need to look into font metrics override descriptors.
Using Fonts in Next.js
Using fonts in Next.js is a bit more involved than in other frameworks, but with the right tools and techniques, you can achieve beautiful typography on your website.
To load custom fonts into your Next.js app, you can use the next/font/google package if you're using Google Fonts as your custom font provider. This is a great way to easily add fonts to your site.
You can also use Tailwind CSS to style your fonts, and define the main font-family on the body tag. This is what Cruip templates do, and it's a good practice to follow.
One thing to keep in mind is that you can't define the font for headings directly in CSS to avoid repeating it for each element. Instead, you should use Tailwind CSS classes to define element styles, as Cruip templates do.
To define a variable for the font, you can use the next/font package and configure your template paths with the CSS variables.
Here are some tips for using fonts in Next.js:
- Use a variable font to make it easier to switch between different fonts.
- Preload your font file to prevent layout shift.
- Self-host your font file instead of using Google Fonts.
- Use font-display: optional to prevent layout shift.
- Subset your imports to reduce the size of your font file.
By following these tips and using the right tools and techniques, you can achieve beautiful typography on your website and make it look great on all devices and operating systems.
SEO Benefits of Correctness
Using custom fonts on your Next.js site can have a positive impact on your Lighthouse scores and SEO. This is because implementing custom fonts can improve the user experience, which is a key factor in determining your site's performance score.
Implementing custom fonts carefully is crucial to avoid performance issues. Using too many or too large font files can slow down your site's loading time and hurt your Lighthouse performance score.
A good balance between using custom fonts to enhance the design and ensuring they don't negatively impact performance or user experience is key. This balance is essential to achieve better Lighthouse scores and improve SEO.
Here are the key benefits of using custom fonts correctly on your Next.js site:
- Improved user experience
- Better Lighthouse scores
- Improved SEO
By implementing custom fonts correctly, you can create a better user experience, which is essential for improving SEO and Lighthouse scores.
Template and Replacement
To add a new Google Font to a template, you can load the font using next/font in the layout.tsx file, or directly in the component where it's needed. This helps avoid loading unnecessary fonts throughout the entire website.
To use the font across all pages, import the font in the layout.tsx file by replacing spaces with underscores and capitalizing the first letter of each word. For example, the font "Nothing You Could Do" becomes "Nothing_You_Could_Do".
You can then add the font to the Tailwind CSS configuration file by adding the font-nycd variable to your theme in the tailwind.config.js file.
Cruip Template Usage
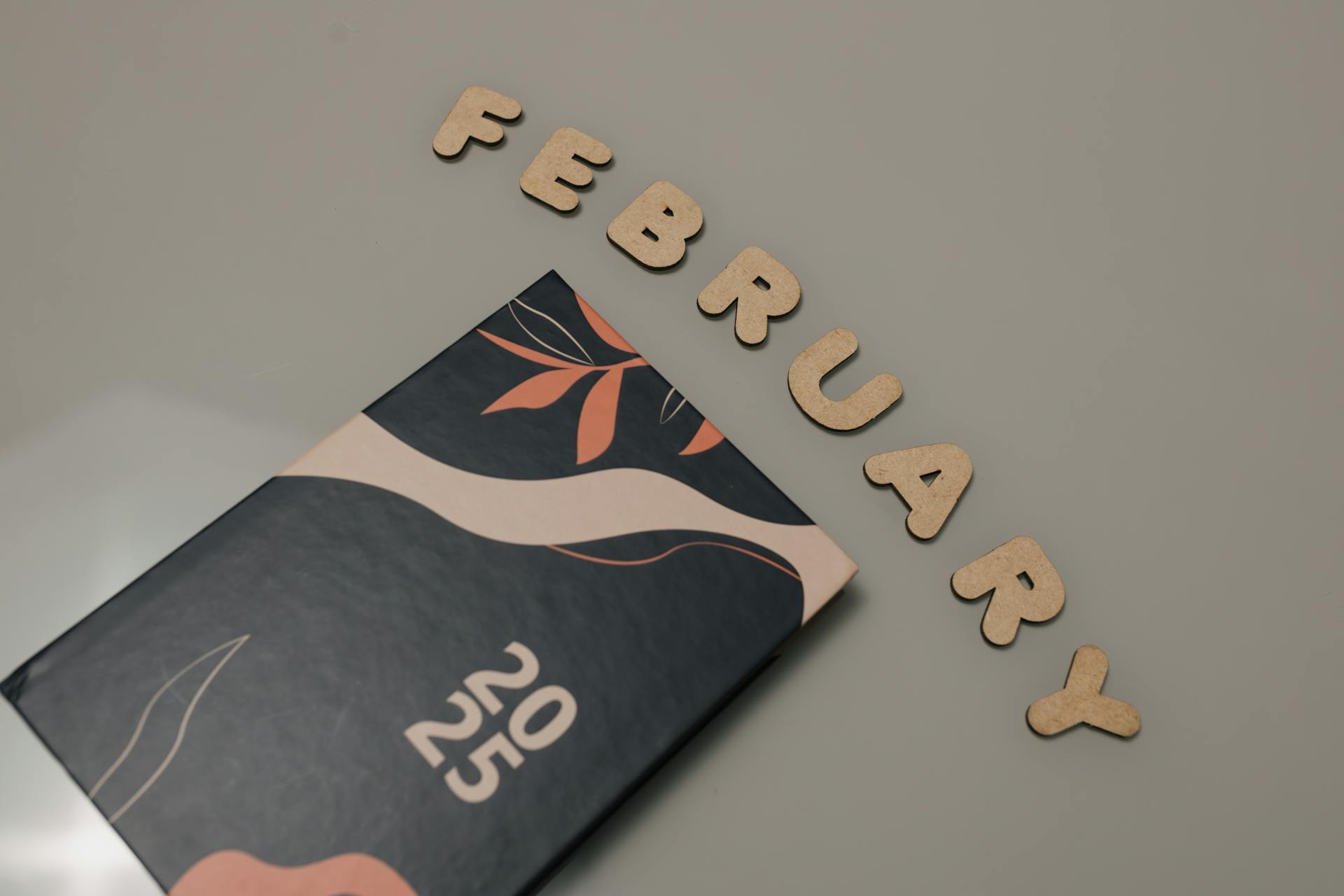
Using Cruip templates involves defining the main font-family on the body tag, as seen in the Tidy template where Inter is the base font defined with the font-inter class.
We define a secondary font, like Playfair Display, directly on each element using Tailwind CSS classes, such as font-playfair-display for headings.
Defining a secondary font directly in CSS would require repeating it for each element, which is why we prefer to use classes to define element styles, as encouraged by Tailwind CSS.
This approach makes it easy to switch to a different font for headings by simply modifying the class, which is a big advantage of using Tailwind CSS classes.
Testing the typography of your website on different devices and operating systems is a good idea to ensure that the text design meets your expectations.
Replacement
Replacement is a crucial aspect of template design, and it's essential to know how to replace fonts, classes, and other elements seamlessly. In Next.js, you can replace one Google Font with another by modifying the font import in the layout.tsx file.
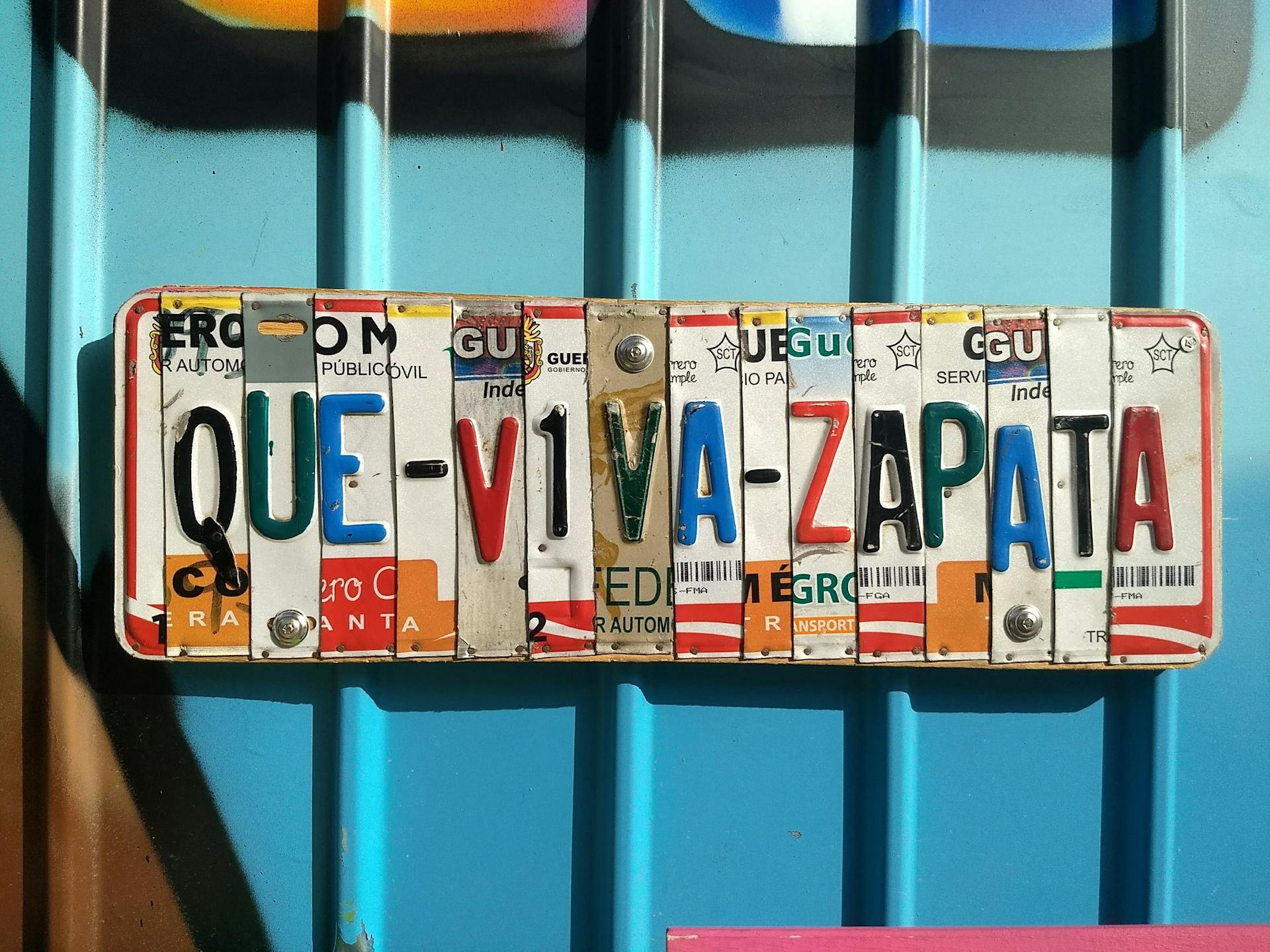
To do this, you can change the font import from the original font to the new one, just like in Example 7, where Playfair Display is replaced with Poppins. This is done by simply replacing the font name in the import statement.
For instance, if you have the following code:
becomes this:
And then, you need to update the class name used for the headings to match the new font. You can do this by searching for the old class name, "font-playfair-display", and replacing it with the new one, "font-poppins", as shown in Example 7.
Here's a summary of the steps:
By following these steps, you can replace one Google Font with another in your Next.js template, ensuring a smooth and seamless experience for your users.
Sources
Featured Images: pexels.com