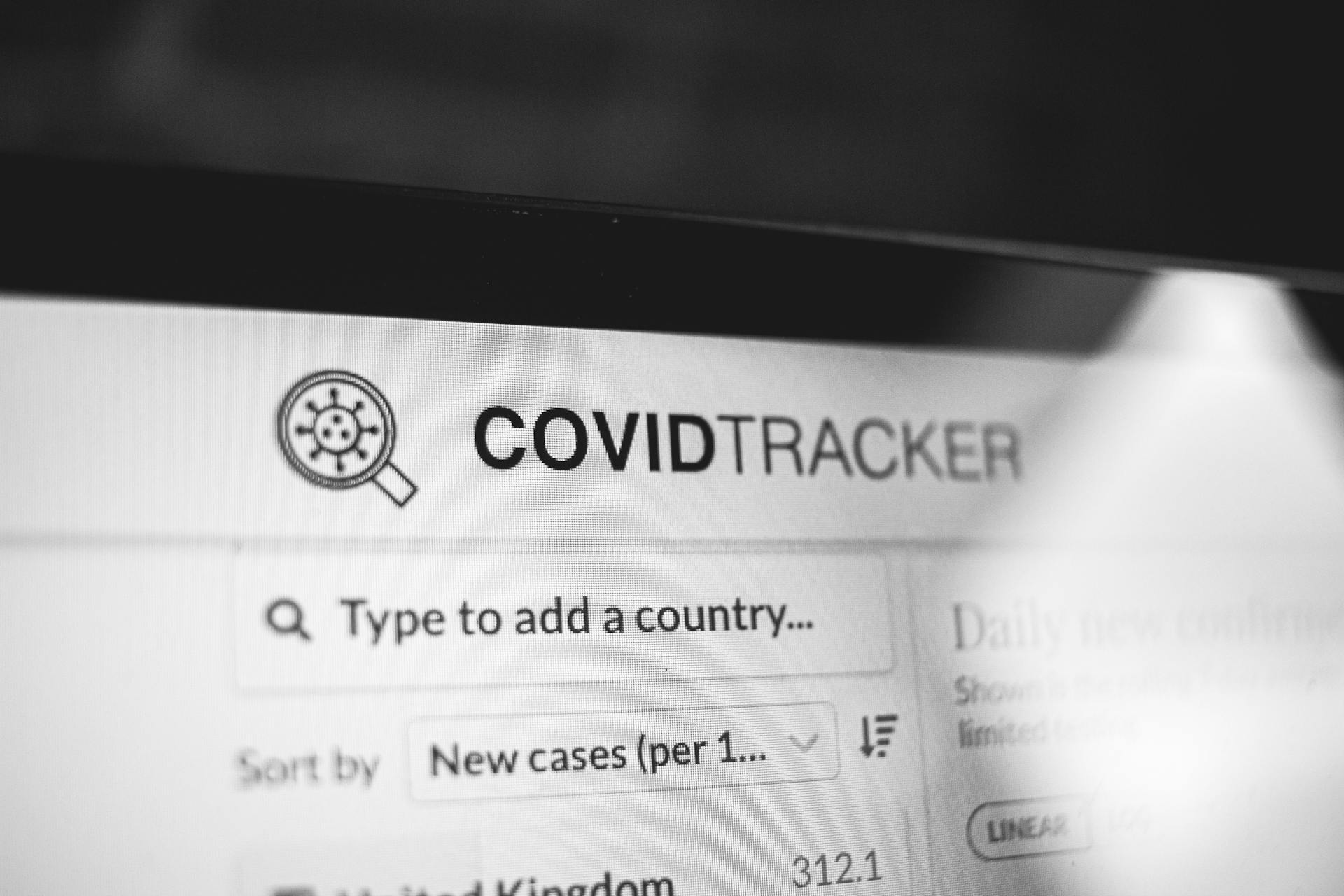
Building a sidebar with Tailwind and Next.js is a great way to add some structure and organization to your website.
First, create a new component for your sidebar by running `npx create-next-app sidebar-component --template default`. This will give you a basic Next.js project to work with.
To use Tailwind in your component, you'll need to install it by running `npm install tailwindcss`. Then, create a `tailwind.config.js` file to configure Tailwind for your project.
Tailwind comes with a set of pre-defined utility classes that you can use to style your sidebar. For example, you can use the `bg-gray-200` class to set the background color of your sidebar.
Broaden your view: How to Add Tailwind to Next Js
Project Setup
To set up a sidebar project with Tailwind NextJS, start by creating a new Next.js project using the terminal and the command: npx create-next-app my-app --ts. Then, navigate into the project folder and add Tailwind CSS.
Next, create a new file called Layout.js inside the Layout folder to organize your project's visuals. This will allow you to use React Icons to add different icons to your sidebar.
In the terminal, you can start a new Next.js project with the command: npx create-next-app my-app --ts, and then navigate into the project folder to add Tailwind CSS and other necessary files.
On a similar theme: How to Use Google Fonts in Nextjs
Why Do You Need a?
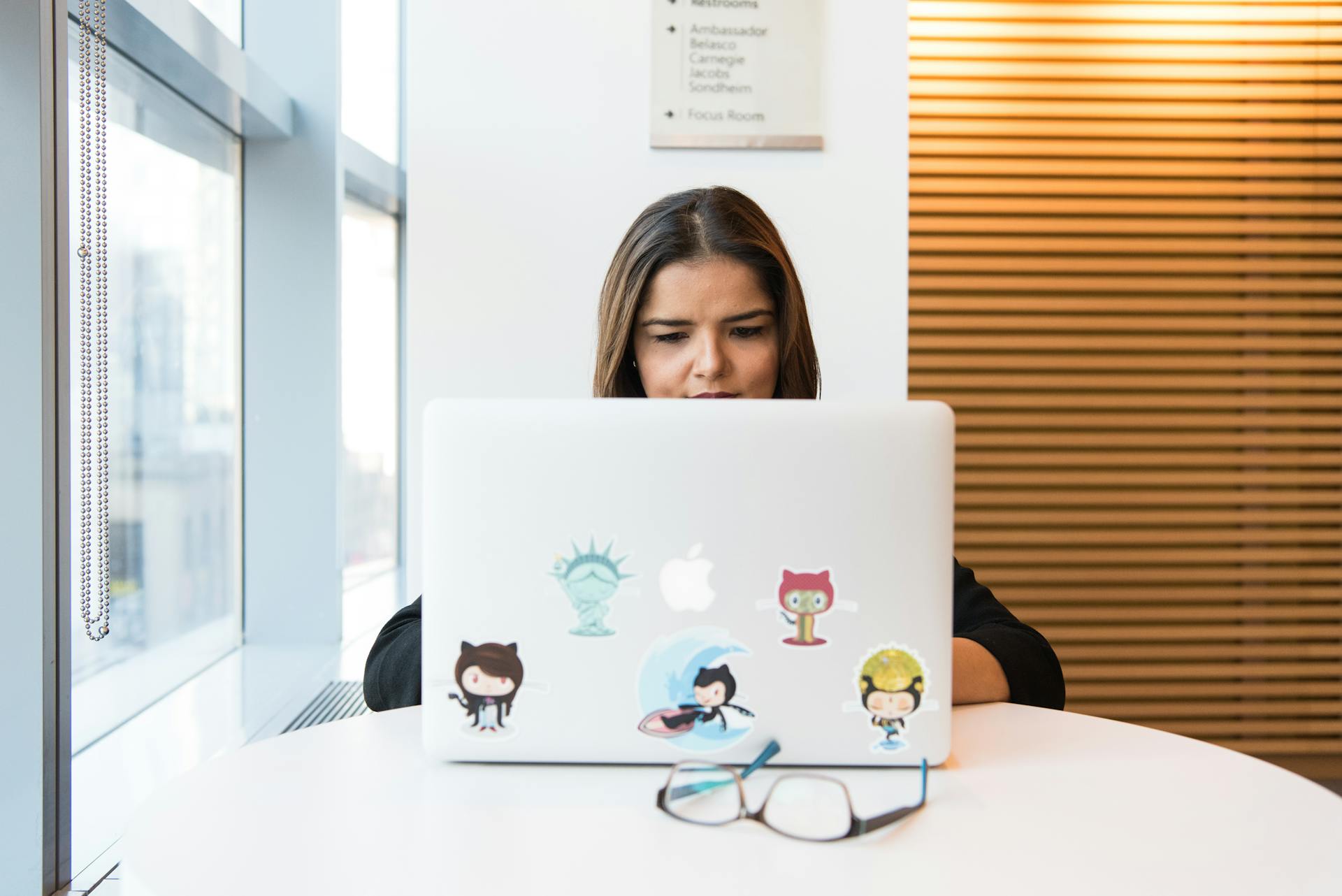
A well-designed sidebar can significantly improve the functionality and aesthetics of your web projects. It provides an efficient way to navigate between different sections of your website, enhancing user experience by organizing content.
Implementing a responsive sidebar ensures that your navigation remains user-friendly across various devices. This is especially important for users who access your website on their mobile phones or tablets.
A well-designed sidebar can also improve both design and usability by utilizing the div classname to apply different styles to each section of the sidebar. This allows you to create a visually appealing and easy-to-use navigation system.
Discover more: Next Js Navigation
Project Setup
To set up a new project, you'll need to start with a Next.js project. Open your favorite terminal and run `npx create-next-app my-app` to get started.
In the terminal, navigate into the project folder using `cd my-app`. Next, you'll need to add Tailwind CSS to your project.
You'll be using React Icons to add icons to your design. Create a new file called Layout.js inside the Layout folder.
For more insights, see: Icons in Next Js
Creating the Component
Creating the Sidebar Component is a fundamental step in building a Tailwind CSS sidebar for your Next.js project. We'll be using functional components and React Hooks to create this component.
The Sidebar component takes in two props: show and setter, which control its visibility. The show prop determines whether the sidebar is visible or not, while the setter prop is a function that updates the show prop.
We use the useLocation hook from react-router-dom to get the currently displayed route inside the Sidebar component. This allows us to highlight the corresponding menu item in the sidebar.
To display items on the sidebar, we're using the MenuItem component, which we'll write in the next section. We pass the setter function to each MenuItem component and call it on click to toggle the sidebar's visibility.
Related reading: Next Js Component Library
Creating the Component
To create a Tailwind CSS sidebar component, start by creating a new file called Sidebar.js inside the Layout folder.
Additional reading: Tailwind Css Sidebar Navigation
The Sidebar component is a functional component that takes in two props, show and setter, which control the visibility of the sidebar.
You can use the uselocation from react-router-dom to get the currently displayed route inside the Sidebar component.
The main structure of the sidebar is wrapped in a div element with a set of Tailwind CSS classes, including flex, bg-blue-800, w-[250px], transition-[margin-left], ease-in-out, duration-500, fixed, md:static, top-0, bottom-0, left-0, z-40, and pr-5.
To display the items on the sidebar, you can use the MenuItem as a component, which will be created in the next section.
Here are the Tailwind CSS classes used to style the sidebar:
- flex
- bg-blue-800
- w-[250px]
- transition-[margin-left]
- ease-in-out
- duration-500
- fixed
- md:static
- top-0
- bottom-0
- left-0
- z-40
- pr-5
To toggle the visibility of the sidebar, you pass the setter function to each MenuItem component and call it on click, which updates the show prop and triggers a re-render of the Sidebar component.
Broaden your view: Nextjs Pathname Server Component
Creating the Navbar
The Navbar is a crucial component of any website, providing users with a clear navigation path.
To create a functional Navbar, you'll need to define its structure, which involves specifying the container, header, and links.
The Navbar's container should be a fixed-width element, as specified in the previous section on "Defining the Container".
The header of the Navbar should contain the logo and title of your website, which should be aligned to the left.
The links within the Navbar should be evenly spaced and have a hover effect to indicate their active state.
You can use the CSS classes defined in the "Defining the Container" section to style the Navbar's links.
The Navbar's links should be clickable, and their corresponding pages should be defined in the "Defining the Pages" section.
Broaden your view: Hero Section Animation Nextjs
Sources
- https://purecode.ai/blogs/tailwind-sidebar
- https://thisisyinka.hashnode.dev/create-a-simple-sidebar-with-nextjs-tailwind-css-in-30-minutes
- https://purecode.ai/components/nextjs/sidebar
- https://stackoverflow.com/questions/78870178/how-to-implement-a-fixed-sidebar-and-header-in-a-next-js-admin-panel-with-condit
- https://daily-dev-tips.com/posts/creating-a-sidebar-layout-in-nextjs-with-tailwind/
Featured Images: pexels.com