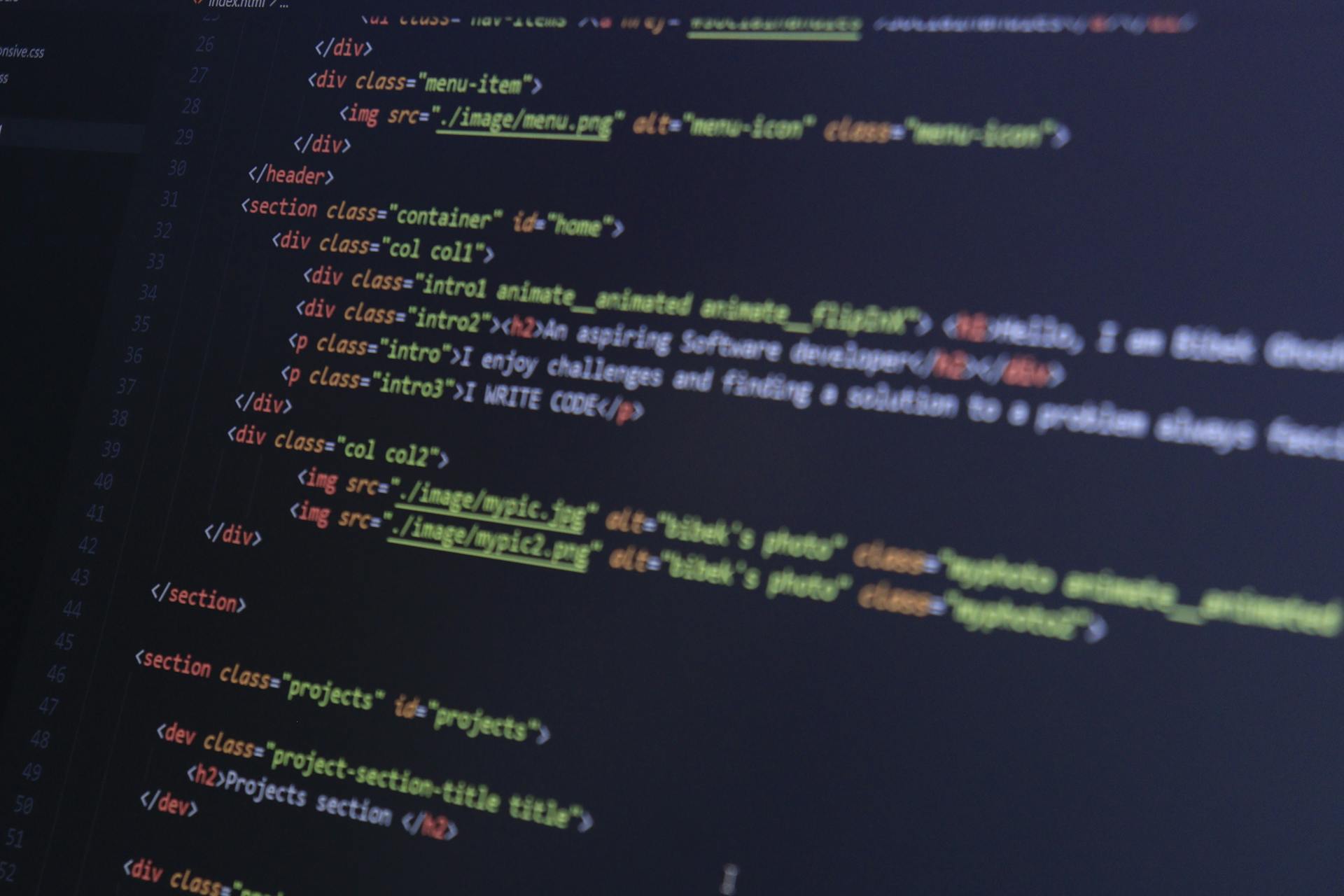
Next Js Version 15 introduces a new feature called "Automatic Code Splitting" which enables developers to split their code into smaller chunks, making it easier to manage and maintain their applications.
This feature allows developers to create smaller, more modular codebases, which in turn improves the overall performance of their applications.
With Automatic Code Splitting, developers can now focus on writing clean and efficient code without worrying about the performance implications.
Automatic Code Splitting can be enabled by adding a simple configuration to the Next Js configuration file.
Related reading: Next Js Feature Flags
Server Components and Interactions
Server Components in Next.js can reduce the amount of JavaScript sent to the client, enabling faster initial page loads.
You should add "use client" to a component when using useState or useEffect client hooks from React, depending on certain browser APIs, or wanting to add certain event listeners. This directive is essential for Next.js to render the component correctly.
Next.js will fail to render and show an error if you decide to utilize useState or any other client hooks without specifying "use client".
Consider reading: Nextjs Hooks
The "use client" directive is not automatic, but it's hard to make a mistake because Next.js made the developer experience great.
You can show a loading state simply by creating a loading.tsx file, like in the example where app/dashboard/loading.tsx is created.
Here's a list of when to use "use client":
- using useState or useEffect client hooks from React
- depending on certain browser APIs
- wanting to add certain event listeners
By utilizing async/await in Server Components, you can break down the UI using React's Suspense and make sure the layout renders while a specific component in the layout waits for data to be fetched.
Client-Side Enhancements
You can use Client Components by specifying a "use client" directive at the top of the component meant for the client. This is necessary when using useState or useEffect client hooks from React, or when depending on certain browser APIs or adding certain event listeners.
You should add "use client" when:
- using useState or useEffect client hooks from React
- you depend on certain browser APIs
- you want to add certain event listeners
If you decide to utilize useState or any other client hooks, Next.js will fail to render and show an error, so it's best to only add "use client" when necessary to keep client-side JavaScript code to a minimum.
Layouts
Layouts are a game-changer in Next.js 13, making it easier to extract shared code between multiple pages.
Layouts accept another layout or a page as a child, allowing you to reuse code across different pages.
With layouts, you can now create a consistent look and feel across your application by defining a shared layout in app/layout.tsx.
To get started, you'll need to create a new file called app/layout.tsx and copy the boilerplate CSS from pages/globals.css to app/global.css.
Including the global CSS in app/layout.tsx is as simple as adding a single line of code.
By leveraging layouts, you can streamline your development process and create a more maintainable codebase.
Readers also liked: Nextjs Code Block
Image Improvements
The new Image component in Next.js is a game-changer. It comes with less client-side JavaScript, making it a more efficient choice.
One of the biggest changes is that the next/image import has been renamed to next/legacy/image, and the next/future/image import is now simply next/image. A helpful codemod is available to make the migration process smoother.
You'll also need to set the width and height properties for non-static images, or images without the fill property, which is a small but important change.
Font
Font enhancements can make a big difference in the user experience. You can use Google Fonts (or any custom font) without sending any requests from the browser with the new @next/font feature.
This feature lets you download CSS and font files at build time with the rest of the static assets. No more waiting for fonts to load.
To try it out, you need to install the package. Then, you can use it like this: @next/font.
Worth a look: Nextjs Loader
Client Component Streaming and Fetching
To stream and fetch data in Client Components, you need to use the use hook, which is a new React function that accepts a promise and handles it in a way compatible with components, hooks, and Suspense.
The use hook is conceptually similar to await and is essential for handling promises returned by functions in Client Components. You can use it to fetch data and display a loading screen until the data is prepared for rendering.
Take a look at this: Nextjs Use Server
In Next.js 13, you can stream parts of the UI to the client with React Suspense, making it easier to show a loading state while data fetching is in progress. This is especially useful when fetching data that takes some time to complete.
To fetch data in Client Components, you should use the use hook to handle the promise returned by the function, as it will cache and dedupe requests to avoid the same data being fetched more than once.
You can fetch data anywhere in the app directory, but it's recommended to fetch data directly in the components that use it, even if you need to request the data in multiple components.
A different take: Nextjs App Route Get Ssr Data
Combining Static and Dynamic
In Next.js 14, Partial Prerendering is an experimental feature that combines the benefits of static site generation (SSG) and server-side rendering (SSR). This hybrid approach promises to simplify development by offering the performance of staticity with the flexibility of dynamic content.
To implement Partial Prerendering, you'll use React Suspense features to define which parts of your application can be statically prerendered and which need to be loaded dynamically.
The Suspense component boundaries determine which parts of the application are static and which are dynamic. Static parts are prepared as HTML, while dynamic parts are updated only when necessary.
You'll define the dynamic parts of your component using Suspense, so they're loaded after the static shell is loaded.
Explore further: Next Js Dynamic Routes
Turbopack: Turbo Compiler
Turbopack is a game-changer for Next.js developers.
Built on the Rust programming language, Turbopack is a turbo compiler that replaces Webpack, offering a significant boost in local development performance.
Local server startup times are a whopping 53.3% faster with Turbopack, making it a substantial leap forward in development speed.
To test Turbopack, ensure you have version 14 of Next.js in your project, and update it by modifying the dependency in your package.json file and running npm install or yarn install.
Some versions of Next.js 14 may not have Turbopack enabled by default, but you can easily enable it by adding a flag to your start command.
Modify your dev script in package.json from “dev”: “next dev” to “dev”: “next dev –turbo” to get the benefits of Turbopack.
Check this out: Next Js Development Company
Frequently Asked Questions
Should I use Next.js 13 or 14?
For better performance and faster load times, consider using Next.js 14, which offers enhanced data fetching methods and server-side rendering capabilities. Next.js 14 is a more optimized choice, but check the documentation for specific use cases and requirements.
Is Next.js 14 stable?
Yes, Next.js 14 is stable, with Server Actions now a standard feature. This update simplifies web app development and enables seamless server-side function invocation from React components.
What is the difference between Next.js 14 and 15?
Next.js 14 and 15 differ in their default caching behavior for GET functions, with 14 caching them by default and 15 not caching them by default, unless a static route config option is used
What is the difference between Nextjs 13 and Nextjs 14?
Next.js 13 introduced Incremental Static Regeneration (ISR), while Next.js 14 takes it further with on-demand ISR, enabling even faster and more efficient updates to static content. This upgrade streamlines the development process, making it ideal for large-scale projects.
Featured Images: pexels.com