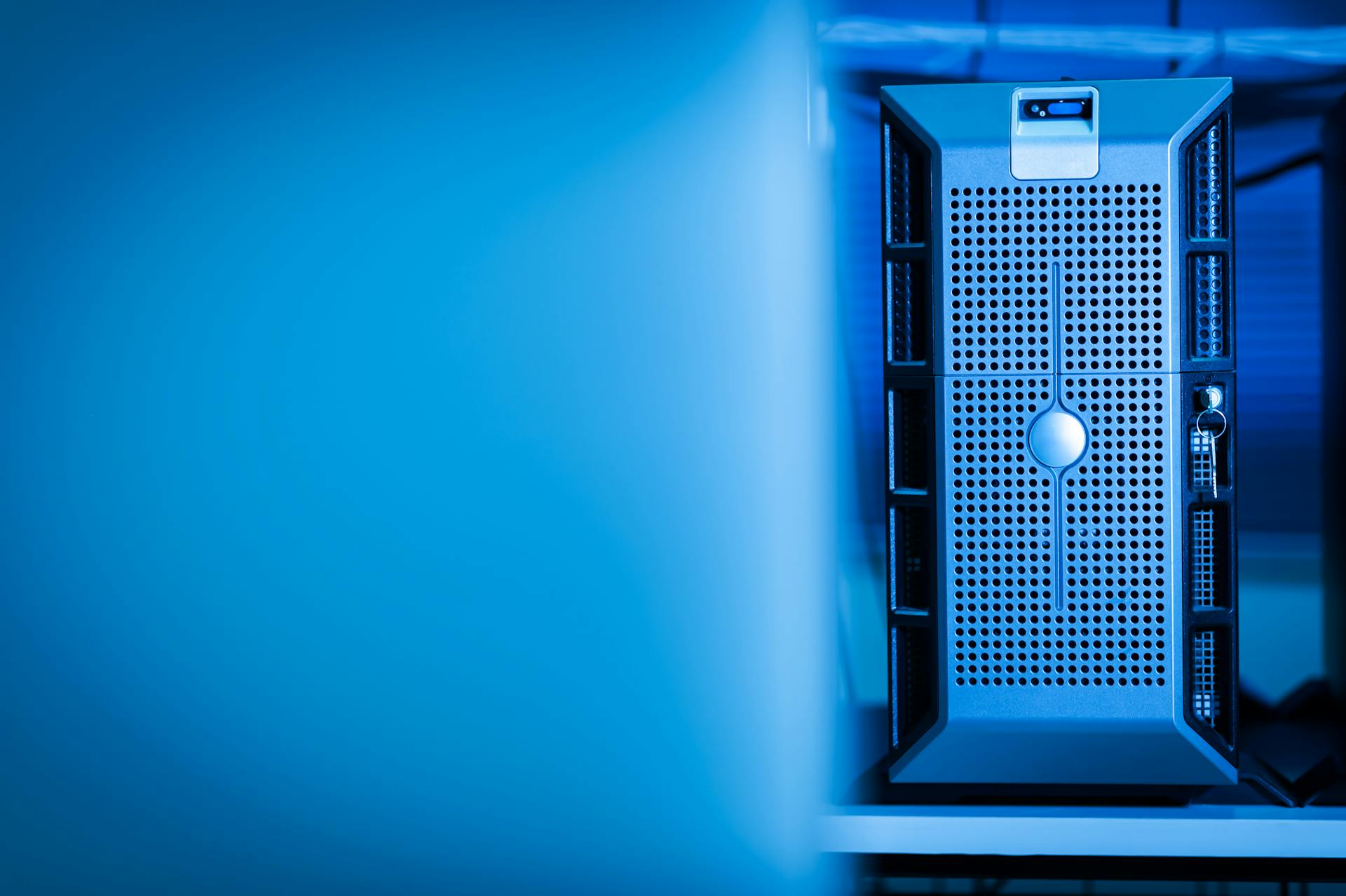
Service Bus Trigger is a type of Azure Function trigger that allows you to run code in response to messages arriving in a Service Bus queue or topic.
It's a powerful tool for building event-driven applications.
With Service Bus Trigger, you can process messages from Service Bus queues or topics without having to write custom code to poll the queue.
This approach is more efficient and scalable than traditional polling methods.
To get started with Service Bus Trigger, you need to create a Service Bus namespace and a queue or topic in the Azure portal.
You can then use the Azure Functions tool to create a new function that listens to the Service Bus queue or topic.
The Service Bus Trigger is a great way to integrate Azure Functions with other Azure services, such as Azure Storage and Azure Cosmos DB.
Discover more: Azure Function C
Endpoint Queue Creation
Creating an endpoint queue for your Azure Function Service Bus trigger is a crucial step. If the asb-tranport command-line tool is not used to create the queue, it's recommended to set the MaxDeliveryCount setting to the maximum value.
You can create the endpoint queue without using the asb-tranport command-line tool. In this case, set the MaxDeliveryCount setting to the maximum value.
Setting the MaxDeliveryCount to the maximum value ensures that your queue can handle a large number of messages. This is especially important for high-traffic applications.
By following these steps, you can create a robust endpoint queue that meets the needs of your Azure Function Service Bus trigger.
Discover more: Azure Service Endpoints
Trigger Function
A trigger function is a crucial part of an Azure Function service bus trigger. It's what gets fired when a new message comes from a service bus queue or topic.
The trigger function name is auto-generated by default, but you can customize it by providing the NServiceBusTriggerFunction attribute with an additional parameter to set the function name. An invalid trigger function name will generate an NSBFUNC004 error.
To run a function every time a message is sent to a Service Bus queue or subscription, apply the ServiceBusTrigger attribute to a string, byte[], or ServiceBusReceivedMessage parameter. This will enable per-message triggers.
Here are the two types of service bus triggers:
- Service bus queue trigger: a queue is basically for first-in-first-out messages.
- Service bus topic trigger: the topic is useful for scaling to numbers of recipients.
Overriding Trigger Function
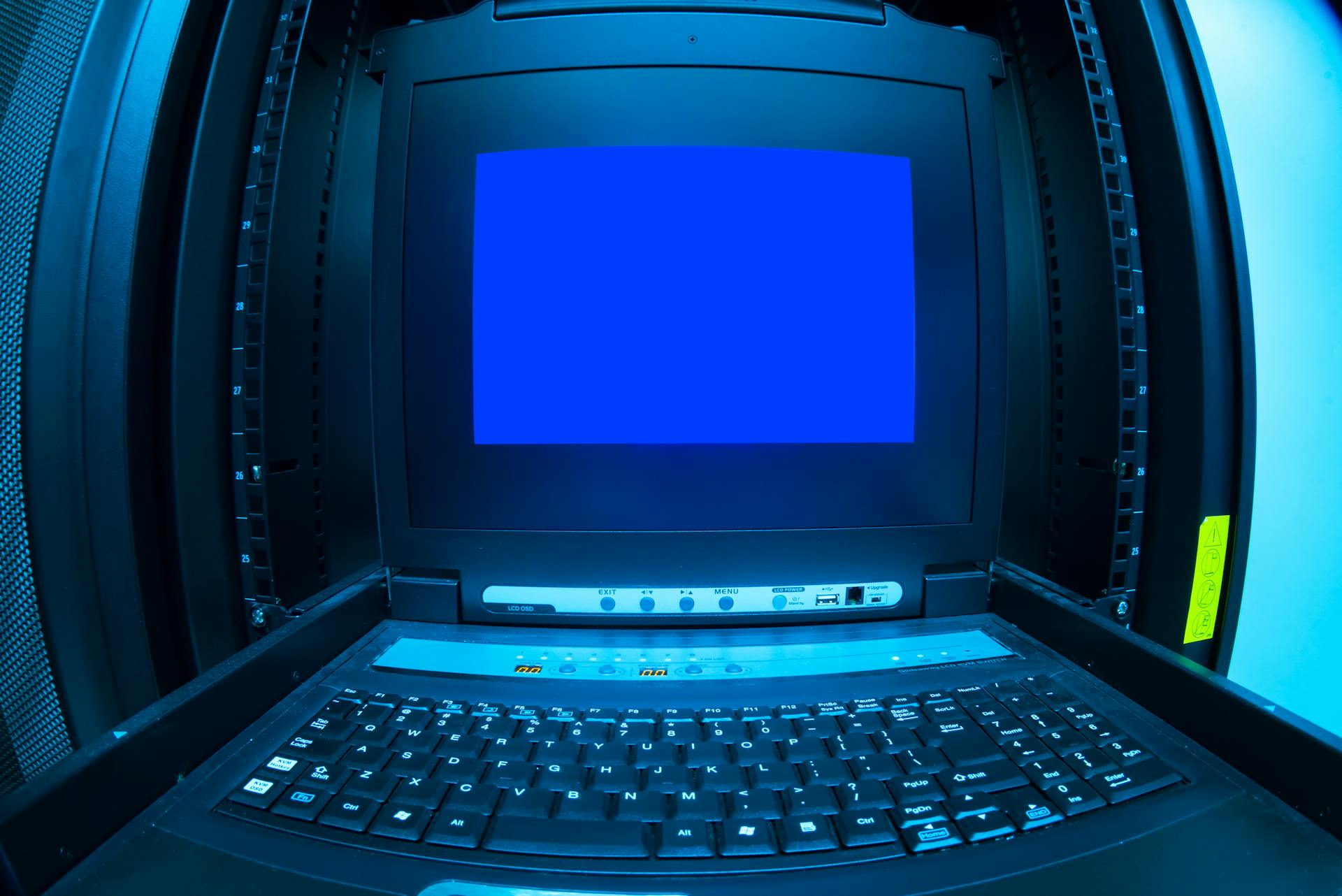
Overriding the trigger function name is a straightforward process. You can customize the function name by providing the NServiceBusTriggerFunction attribute with an additional parameter to set the function name.
An invalid trigger function name will result in an NSBFUNC004 error with the message "Trigger function name is invalid and cannot be used to generate trigger function." So, make sure to double-check your function name.
To override the trigger function name, you can use the following syntax: [NServiceBusTriggerFunction("your_function_name")].
This will allow you to customize the function name to your liking.
Session Triggers
Session triggers allow you to receive messages from a session-enabled queue or topic.
To enable session triggers, you can set the IsSessionsEnabled property on the ServiceBusTrigger attribute. This property is crucial for working with sessions.
By setting this property, you'll gain access to session-specific functionality, including message settlement methods. These methods are essential for managing messages within a session.
You can bind to the SessionMessageActions to get access to these message settlement methods. This is a key step in working with sessions and managing messages effectively.
See what others are reading: Azure Function Triggers
Binding and Receiving
You can use binding to strongly-typed models by applying the ServiceBus attribute to the model parameter, which will attempt to deserialize the ServiceBusMessage.Body into the strongly-typed model.
This is particularly useful when working with ServiceBus, as it allows for more precise control over the data being processed.
Binding to ReceiveActions is another option, which enables you to receive additional messages from within your function invocation. Any additional messages received will be subject to the same AutoCompleteMessages and MaxAutoLockRenewalDuration configuration as the initial message delivered to your function.
Peeking messages is also possible with ReceiveActions, which allows you to view messages without locking them, making them exempt from AutoCompleteMessages and MaxAutoLockRenewalDuration configuration.
Discover more: Azure App Config
Binding to ServiceBusClient
Binding to ServiceBusClient is a useful technique for dynamically creating a sender based on the message received. You can bind to the same ServiceBusClient that the trigger is using, which can be particularly helpful in certain scenarios.
This allows you to create a sender that's tailored to the specific message being processed. By binding to the same ServiceBusClient, you can leverage the existing configuration and settings.
You may need to dynamically create a sender based on the message, and binding to the same ServiceBusClient can help you achieve this. This approach can save you from having to manually create and configure a sender for each message.
Worth a look: How to Create Service Principal in Azure
Binding to Receive Actions
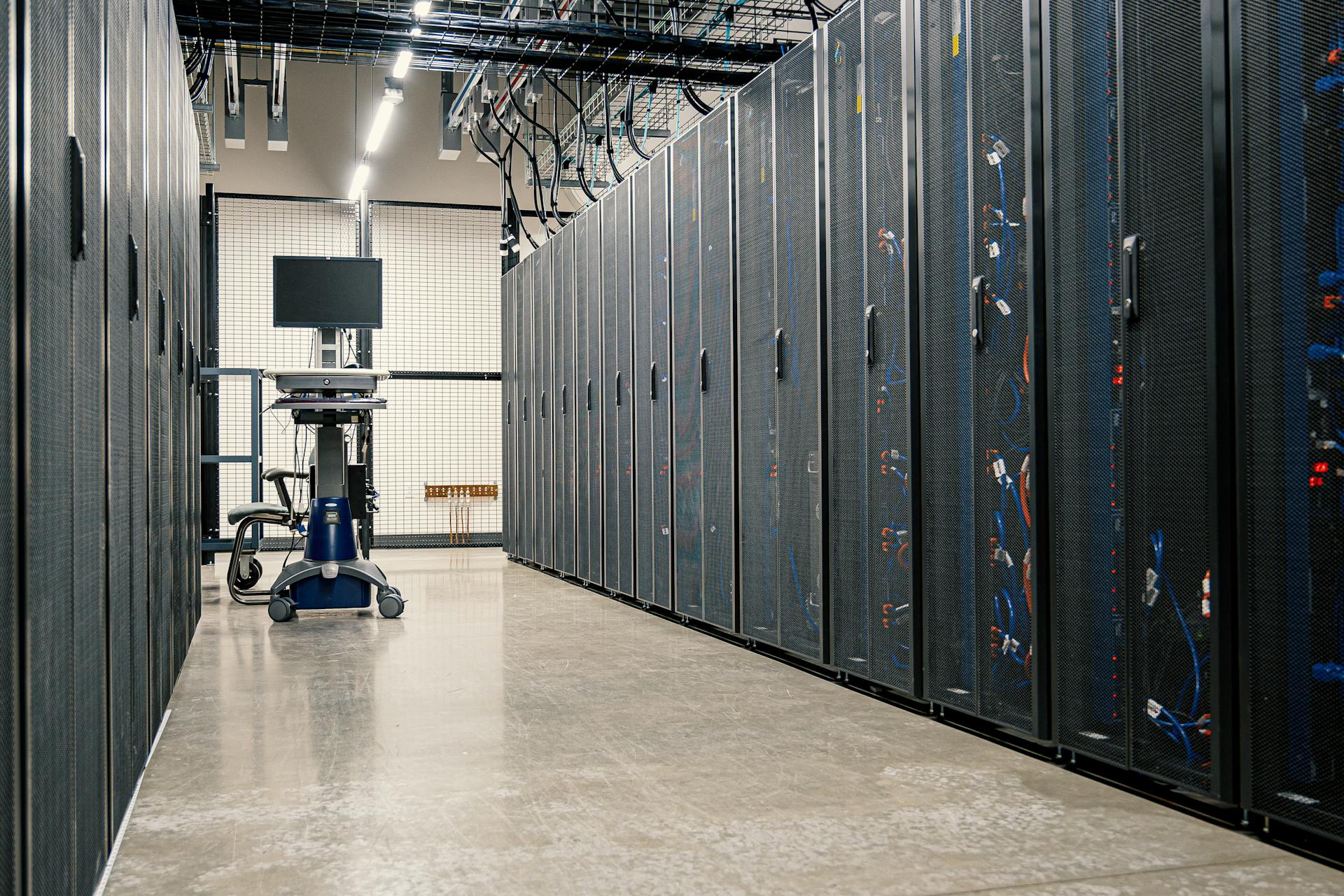
Binding to Receive Actions can be useful if you need more control over how many messages to process within a function invocation based on some characteristics of the initial message delivered to your function via the binding parameter.
Any additional messages that you receive will be subject to the same AutoCompleteMessages and MaxAutoLockRenewalDuration configuration as the initial message delivered to your function.
You can also peek messages, which are not subject to the AutoCompleteMessages and MaxAutoLockRenewalDuration configuration because these messages are not locked and therefore cannot be completed.
Error Handling
Error handling is crucial in Azure Function Service Bus triggers. Repeatedly failing messages are sent to the error queue by default.
The error queue can be configured or disabled completely. This allows the native Azure Service Bus dead-lettering configuration to handle failures.
You can customize error handling to fit your needs.
Testing and Troubleshooting
Testing and Troubleshooting is crucial when working with Azure Function Service Bus Triggers.
If your function triggers an unhandled exception and you haven't already settled the message, the extension will attempt to abandon the message so that it becomes available for receiving again immediately.
For integration tests, you can use ActivatorUtilities to activate your function with all dependencies injected, and pass in the service provider from your test harness. This allows you to test the contract of your service bus messages by specifying them as json, making it easier to catch breaking changes.
Related reading: Azure Dev Test
Integration Test
Integration tests are a crucial part of ensuring our code works as expected in a real-world scenario.
We activate our function with all dependencies injected using ActivatorUtilities and pass in the service provider from our test harness.
This allows us to test the contract of our service bus messages by specifying them as json, which helps us catch any accidental changes.
A mocked input message is created using the ServiceBusModelFactory.ServiceBusReceivedMessage method from the Azure.Messaging.ServiceBus namespace.
We only use one other mock: a mocked instance of ServiceBusMessageActions, which we could use to check if messages have been manually completed or moved to the dead-letter queue.
The function itself is triggered manually by calling the Run method and passing in the mocks.
For your interest: Azure Key Vault Tutorial C#
Troubleshooting
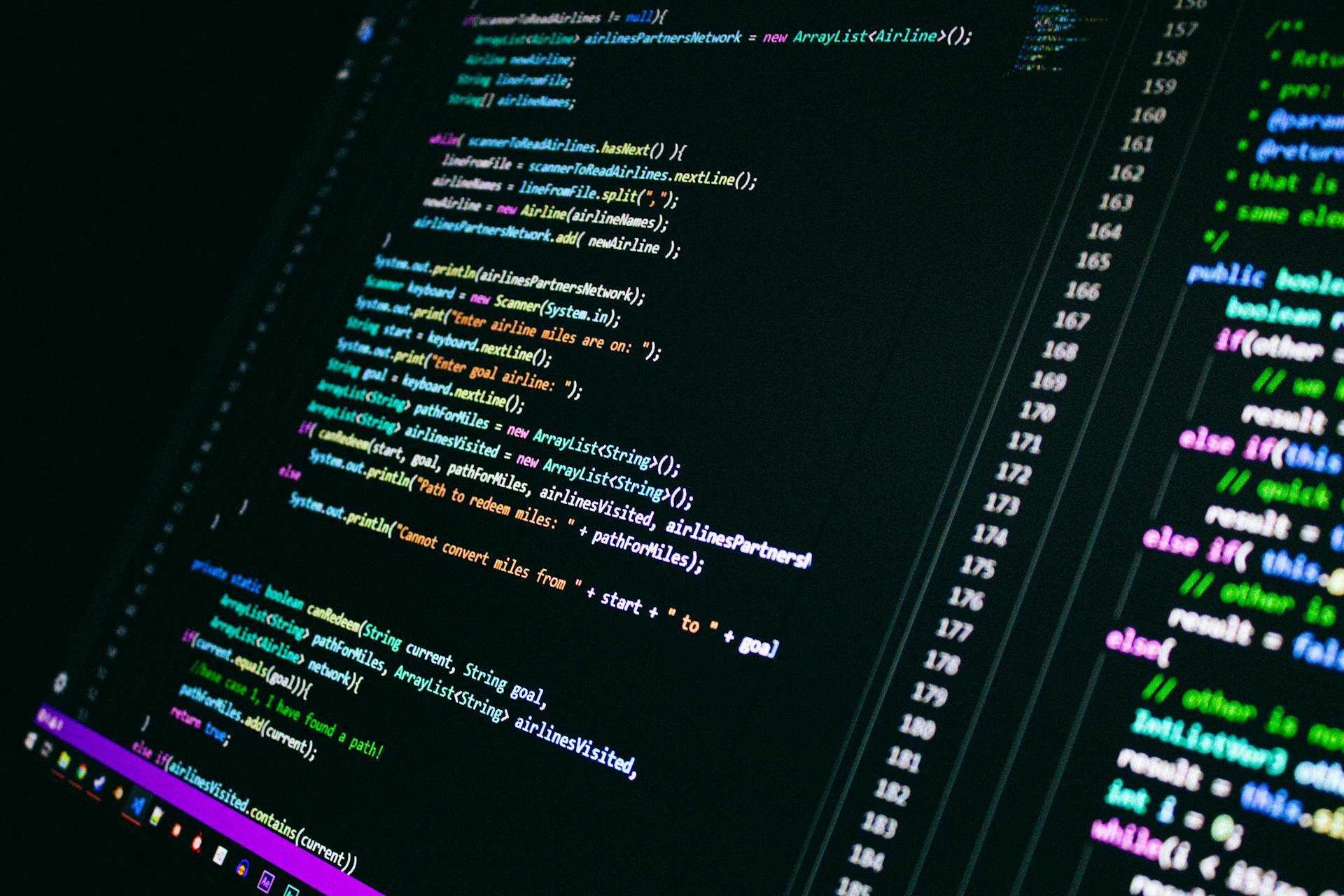
Troubleshooting can be a real challenge, especially when dealing with unhandled exceptions in your code. If your function triggers an unhandled exception, the extension will attempt to abandon the message so it becomes available for receiving again immediately.
This can be a lifesaver when you're trying to debug and identify the issue. If you haven't already settled the message, the extension will take care of it for you, allowing you to move forward with troubleshooting.
If you're stuck, don't forget to refer to Monitor Azure Functions for more troubleshooting guidance. It's always a good idea to have a resource like that available when you're troubleshooting.
Prerequisites and Setup
To use Azure services, including the Azure Function Service Bus trigger, you'll need to have an Azure Subscription. You can sign up for a free trial or use your Visual Studio Subscription benefits when creating an account.
You'll also need a Service Bus namespace to interact with Azure Service Bus. If you're not familiar with creating Azure resources, you can follow the step-by-step guide for creating a Service Bus namespace using the Azure portal.
To quickly create the needed Service Bus resources in Azure, you can deploy our sample template by clicking on the provided link. This will give you a connection string for your Service Bus entity.
Here are the specific steps you'll need to take:
- Azure Subscription: Sign up for a free trial or use your Visual Studio Subscription benefits.
- Service Bus namespace: Create a namespace using the Azure portal or use the Azure CLI, Azure PowerShell, or Azure Resource Manager (ARM) templates.
Sources
- https://docs.particular.net/nservicebus/hosting/azure-functions-service-bus/in-process/
- https://learn.microsoft.com/en-us/dotnet/api/overview/azure/microsoft.azure.webjobs.extensions.servicebus-readme
- https://turbo360.com/blog/azure-functions-triggers-and-bindings
- https://docs.particular.net/nservicebus/hosting/azure-functions-service-bus/
- https://www.wagemakers.net/posts/testing-service-bus-trigger-azure-functions/
Featured Images: pexels.com