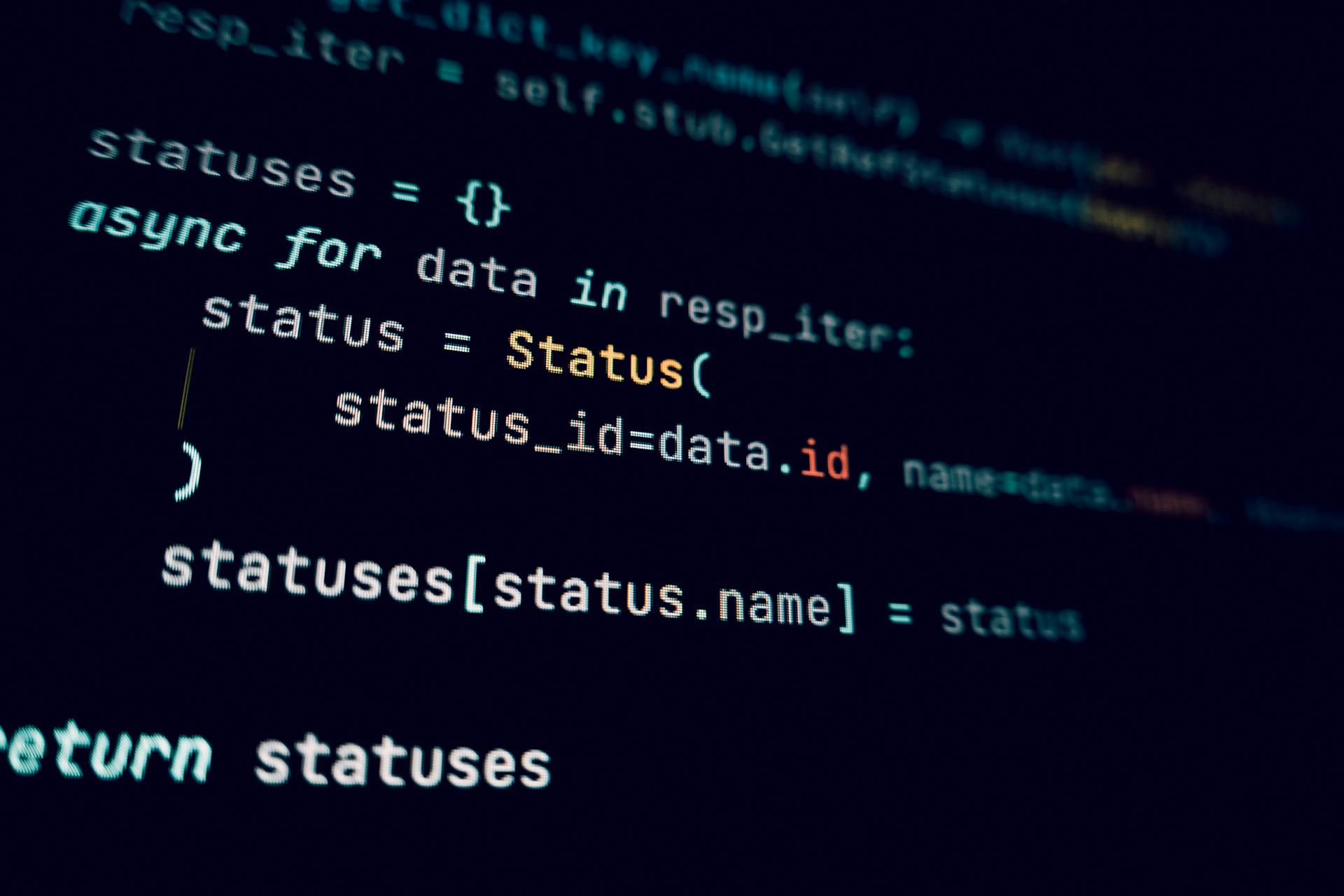
Azure Function triggers are a powerful tool for building scalable web APIs. They enable you to run serverless code in response to various events.
With Azure Functions, you can create custom triggers to handle HTTP requests, changes to Azure Blob Storage, and even changes to Azure Cosmos DB documents.
One of the key benefits of Azure Function triggers is that they allow you to decouple your application logic from the underlying infrastructure. This makes it easier to scale your application as needed.
Azure Function triggers can be used to build event-driven architectures, which are more efficient and scalable than traditional request-response architectures.
Azure Function Triggers
Azure Function Triggers are what cause a function to run. A trigger defines how a function is invoked and a function must have exactly one trigger.
Triggers have associated data, which is often provided as the payload of the function. This data is essential for the function to perform its intended task.
A trigger is the starting point for your Azure Function, and it determines when and how the function is executed.
Trigger Types
Azure Function triggers are a crucial part of building serverless applications, and understanding the different types can help you create more efficient and effective workflows.
The webHookType binding property is used to indicate the type of webhook supported by a function, which also dictates the supported payload. This property can be set to one of the following values: genericJson, github, or slack.
Azure Functions support a range of webhook types, each with its own specific use case. For example, the genericJson type is a general-purpose webhook endpoint that restricts requests to only those using HTTP POST and with the application/json content type.
Here are the different webhook types supported by Azure Functions:
When setting the webHookType property, be sure to follow the guidelines and don't also set the methods property on the binding, as this can cause issues with the webhook functionality.
HTTP Triggers
HTTP triggers are a fundamental part of Azure Functions, allowing your functions to respond to HTTP requests, such as GET or POST requests.
Intriguing read: Http Trigger Azure Function
This trigger gets fired when the HTTP request comes, making it a crucial component for building RESTful APIs or web applications that require a serverless backend.
The HttpTrigger allows you to respond to HTTP requests, and is useful for building RESTful APIs or web applications that require a serverless backend. To use HttpTrigger in your Azure Function, you need to add the [HttpTrigger] attribute to your function.
The [HttpTrigger] attribute specifies that the function should respond to GET and POST requests, and the AuthorizationLevel.Function parameter specifies that the function should be authorized using a function-level token. The Route parameter specifies the URL pattern for the function.
You can customize the route of your HTTP trigger using the optional route property on the HTTP trigger's input binding. You can use any Web API Route Constraint with your parameters.
Here are some examples of how to use route parameters in your HTTP trigger:
By default, all function routes are prefixed with api, but you can customize or remove the prefix using the extensions.http.routePrefix property in your host.json file. The following example removes the api route prefix by using an empty string for the prefix in the host.json file.
The function execution context is exposed via a parameter declared as func.HttpRequest, which allows a function to access data route parameters, query string values, and methods that allow you to return HTTP responses.
On a similar theme: Azure Auth Json Website Azure Ad Authentication
Authorization and Security
You can allow anonymous requests, which don't require keys, or require the master key to be used. This is done by changing the default authorization level using the authLevel property in the binding JSON.
To include an access key in the request, you can add it as a query string variable named code or in an x-functions-key HTTP header. The value of the key can be any function key defined for the function or any host key.
When running functions locally, authorization is disabled, regardless of the specified authorization level setting. This means keys are still required when running locally in a container.
If your function app is using App Service Authentication / Authorization, you can view information about authenticated clients from your code. This information is available as request headers injected by the platform.
Authenticated client information is available as a ClaimsPrincipal, which is part of the request context. You can access this information via HTTP Headers or by including it as an extra parameter in the function signature.
Webhook authorization relies on a key, which can be specified in the webhook provider to send the key name with the request. This can be done through a query string parameter named clientid or an x-functions-clientid header.
Curious to learn more? Check out: Azure Access
Webhooks
Webhooks are a powerful feature in Azure Functions that allow you to respond to external events. They're available only for version 1.x of the Functions runtime, which was a deliberate design choice to improve the performance of HTTP triggers in version 2.x and higher.
In version 1.x, webhook templates provide an additional layer of validation for webhook payloads, making it easier to ensure that incoming data is correct.
The webHookType binding property determines the type of webhook supported by the function, which in turn dictates the supported payload. There are three types of webhooks: genericJson, github, and slack.
Here are the details of each webhook type:
To respond to GitHub webhooks, you need to create an HTTP Trigger function and set the webHookType property to github. Then, copy its URL and API key into the Add webhook page of your GitHub repository.
Webhook authorization is handled by the webhook receiver component, which relies on a key. By default, the function key named "default" is used. However, you can use a different key by configuring the webhook provider to send the key name with the request. There are two ways to do this: by passing the key name in the clientid query string parameter or in the x-functions-clientid header.
Azure Function Bindings
Azure Function Bindings are a powerful feature that allows you to connect your function to various data sources and services. A binding is a connection to data within your function, and it can be either an input or output binding.
Input bindings receive data from external sources, such as Azure Blob Storage or Service Bus queues. Output bindings, on the other hand, send data to external services, like Azure Blob Storage or Service Bus topics.
Bindings can be used in conjunction with triggers, which are the events that trigger your function to run. For example, a BlobTrigger can be used to trigger a function when a new or updated blob is detected in Azure Blob Storage.
Discover more: Azure Function Service Bus Trigger
You can mix and match different bindings to suit your needs, and they are optional, so you can choose to use them or not. Bindings provide a way to declaratively connect other resources to your function, making it easier to manage and maintain your code.
Here's a breakdown of the different types of bindings:
Some bindings support a special direction, "in-out", which allows for both input and output operations. However, this direction is only available in the Advanced editor via the Integrate tab in the portal.
When using attributes in a class library to configure triggers and bindings, the direction is provided in an attribute constructor or inferred from the parameter type.
In Azure Functions, you can use bindings to connect to various services, such as Azure Blob Storage, Service Bus queues and topics, and more. By using bindings, you can simplify your code and make it more maintainable.
Testing
Testing your Azure Functions is a crucial step to ensure they're working as expected. You can start by testing the triggers, which involve adding a file to a blob container.
To validate the triggers, you'll need to use Azure Storage Explorer to upload a file to the blob container marketing. This involves creating the blob container, uploading a file, and selecting the file to upload.
Here's a step-by-step guide to testing triggers:
- Create the blob container marketing
- Click to upload a file
- Select Upload Files
- Click to select a file
- Upload it
Testing bindings is another important aspect of validating your Azure Functions. This involves creating specific containers and uploading files to test how the function handles them.
To validate the Input binding, create the container marketing-txt and upload a .txt file to it. To validate the Output binding, create the container marketing-csv and then upload a .csv file to the marketing-txt container.
Curious to learn more? Check out: Azure Function C
Real-Time Processing and Scheduling
Azure Functions can process data in near real-time using low-latency event triggers like Event Grid and real-time outputs like SignalR. This allows you to respond to events as they happen, making it ideal for applications that require instant processing.
Event Hubs can create a stream of all events, which can then be processed in different ways. For example, you can use an event hub trigger to respond to an event sent to an event hub event stream.
Azure Functions can also be used for real-time stream and event processing, such as with the event hubs trigger to read from an event hub and the output binding to write to an event hub after debatching and transforming the events.
Worth a look: Azure Events
Run Scheduled Tasks
You can run your code based on a cron schedule that you define using Azure Functions.
Azure Functions enables you to run your code based on a cron schedule that you define. This can be useful for tasks that need to run at specific times, such as every 15 minutes or every hour.
The Timer trigger is a type of trigger that is called on a predefined schedule. You can set the time for execution of the Azure Function using this trigger.
To create a function that runs on a schedule, you can use the Azure portal. The process involves logging in to the Azure Portal, clicking on the top left + icon, and selecting Function App.
Here's a step-by-step guide to creating a function that runs on a schedule:
- Log in to the Azure Portal
- Click on the top left + icon and select Function App
- Provide a unique Function App name, Subscription, Resource Group, Hosting Plan, Location, Storage, and click on the Create button
- Once the function is deployed, click on Notifications and check the Functions details and add the trigger
- Select the desired template from the available templates and type the trigger name and schedule
- The Schedule value is a six-field CRON expression that determines how often the function will run
- For example, by providing 0 0/5 * * * *, the function will run every 5 minutes from the first run
Some common use cases for scheduled tasks include:
- Analyzing a financial services customer database for duplicate entries every 15 minutes
- Running database backups or data processing every hour
- Sending a heartbeat message to a monitoring service every 5 minutes
Real-Time Processing
Azure Functions can process data in near real-time, making it ideal for applications that require instant responses. This can be achieved through event triggers like Event Grid, which enables functions to process data as it arrives.
You can use event hubs triggers to read from an event hub and transform the events before writing them to another event hub. This is particularly useful for streaming data at scale.
Azure Functions can also integrate with Azure Event Hubs, Cosmos DB, and other services to process data in real-time. For example, you can use the event hubs trigger to read from an event hub and write to an event hub after debatching and transforming the events.
Some examples of real-time processing with Azure Functions include:
- Streaming at scale with Azure Event Hubs, Functions and Azure SQL
- Streaming at scale with Azure Event Hubs, Functions and Cosmos DB
- Streaming at scale with Azure IoT Hub, Functions and Azure SQL
- Azure Event Hubs trigger for Azure Functions
- Apache Kafka trigger for Azure Functions
These examples demonstrate the versatility of Azure Functions in real-time processing, allowing you to process data from various sources and integrate it with other services.
Sources
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-bindings-http-webhook-trigger
- https://turbo360.com/blog/azure-functions-triggers-and-bindings
- https://medium.com/@paularun.prasath/azure-functions-with-visual-studio-and-azure-devops-part-2-guide-to-triggers-with-examples-39486d8f95f9
- https://tech.playgokids.com/azure-functions-trigger-bindings-part2-blob/
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-scenarios
Featured Images: pexels.com