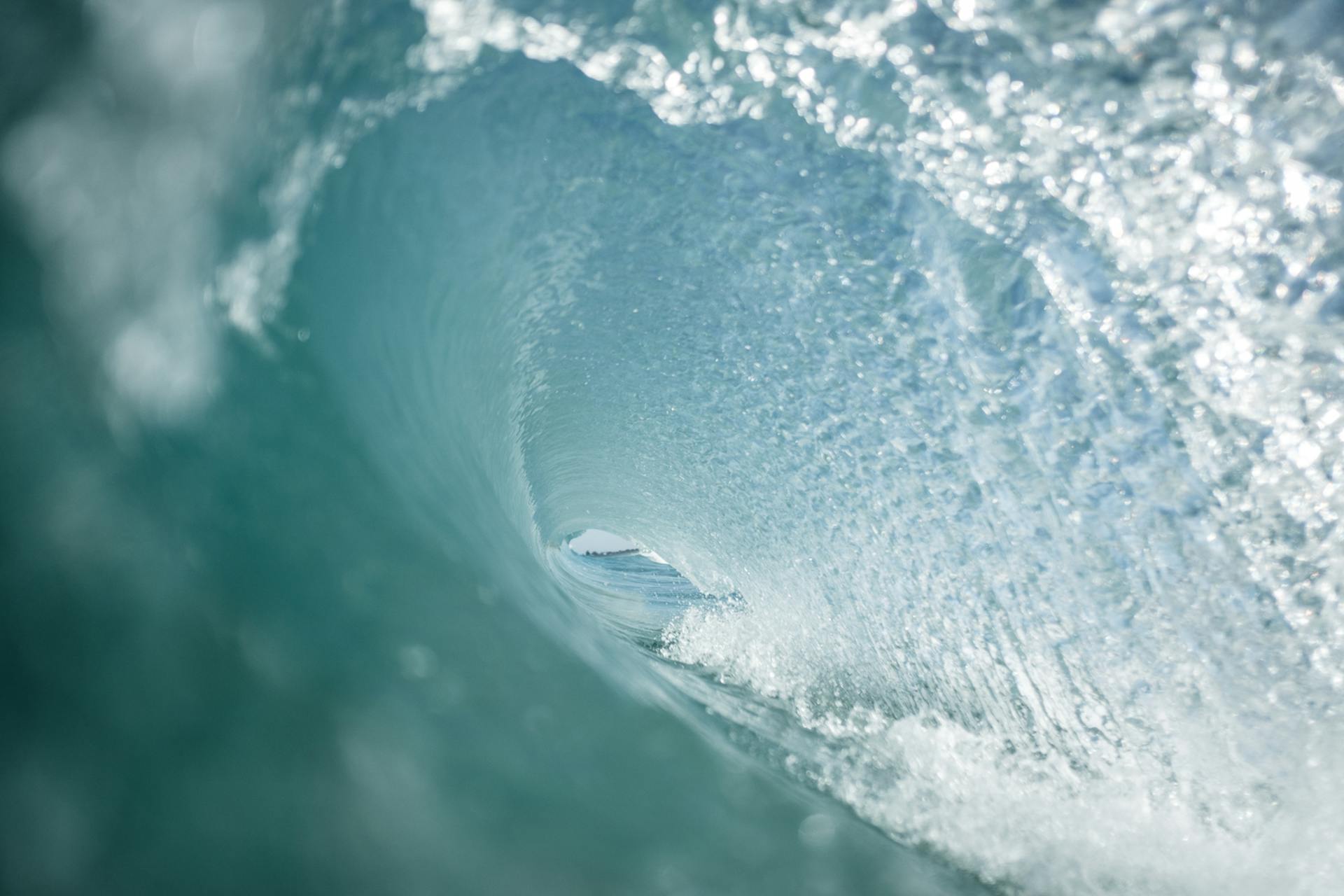
Azure Bicep is a declarative language used for defining and deploying Azure resources. It's a game-changer for infrastructure as code.
Bicep is a more concise and readable language compared to ARM templates, with a syntax that's easier to learn and use. This makes it a great choice for infrastructure automation.
In this tutorial, we'll cover the fundamentals and advanced topics of Azure Bicep. We'll explore how to create and manage resources, and how to use Bicep to automate your Azure infrastructure.
By the end of this tutorial, you'll have a solid understanding of Azure Bicep and be able to create and deploy your own Azure resources with ease.
Here's an interesting read: How to Use Windows Azure
Installation and Setup
To install Azure Bicep, you need to install some tools on your local system, which Microsoft provides for Windows, Linux, and macOS.
You can install Azure CLI to get started with Bicep. To install a specific version, use the command that you can find in the Azure CLI documentation.
If this caught your attention, see: Azure Cli vs Azure Powershell
To validate your Bicep CLI installation, use the command that checks the installation.
Azure PowerShell doesn't automatically install the Bicep CLI, so you must manually install it.
When you manually install the Bicep CLI, run the Bicep commands with the bicep syntax instead of the az bicep syntax for Azure CLI.
To check your Bicep CLI version, run the command that shows the version.
You can install the Bicep CLI using multiple methods, which add it to your PATH.
To create a deployment with your Bicep file, use the Azure CLI, and Bicep is provided as an extension to the CLI.
Check if you've already installed Bicep by running az bicep version.
If it's not installed, install it by running az bicep install in the console.
Make sure you're logged into Azure with the CLI and set the subscription where you want to create these resources.
To set the subscription, use the Azure CLI to first get a list of all the subscriptions you have access to and then set it.
Next, make sure VSCode has the Bicep extension installed.
For another approach, see: Azure Cli 2
Azure Bicep Basics
In Azure Bicep, resources are declared using the resource keyword, followed by the resource name, type, and API version. This is the foundation of creating resources in Bicep.
The resource declaration includes several key pieces of information, such as the symbolic name, resource type, API version, and resource properties. The symbolic name represents the resource within the Bicep template and is case-sensitive.
Here are the basic components of a resource definition in Bicep:
- Symbolic name: The name given to the resource in the Bicep template.
- Resource type: The type of resource being deployed, such as Microsoft.Network/virtualNetworks or Microsoft.Storage/storageAccounts.
- API version: The version of the API used to define the resource.
- Resource properties: The properties of the resource, including its name and deployment location.
These components work together to create a resource definition in Bicep.
String Interpolation
String Interpolation is a powerful feature in Bicep templates that allows you to define strings using parameter or variable names, and Bicep will evaluate the final string using the actual values.
You can use string interpolation instead of concatenation functions like concat() to create variable values. For example, instead of using concat() in the variable declaration, you can surround the parameter name with ${ }.
The syntax for string interpolation in Bicep is straightforward: surround the parameter or variable name with ${ }. This is demonstrated in the example: var stgAcctName = '${name}2468'.
This approach is more readable and efficient than using concatenation functions, and it's a great way to create variable values in your Bicep templates.
Curious to learn more? Check out: Create Schema Azure Data Studio
Conditional Assignment
Conditional Assignment allows you to set a value based on different conditions. This is particularly useful when you need to make decisions based on input parameters or other factors.
You can use a ternary operator to determine the value, which is essentially an if-then-else statement in disguise. For example, if a boolean parameter is set to true, you can use the ternary operator to assign a specific value.
A ternary operator is as simple as using the ? operator to specify the value when the condition is true, and the : operator to specify the value when the condition is false. This can be seen in the example where the sku is set to 'Premium_LRS' when useSSD is true, and 'Standard_LRS' otherwise.
Here's a basic example of how to use a ternary operator in a Bicep template:
```bicep
resource stgAcct 'Microsoft.Storage/storageAccounts@2021-02-01' = {
name: stgAcctName
location: location
kind: 'StorageV2'
sku: {
name: useSSD ? 'Premium_LRS' : 'Standard_LRS'
}
}
```
This is a powerful feature that can help you create more flexible and dynamic templates.
On a similar theme: Azure Service Bus Topic Filter Example
ARM Template Relationships
ARM Template Relationships are a key aspect of Azure Bicep, allowing you to visualize and manage resources in a more intuitive way.
The VSCode Bicep Extension can help with this, providing a visualization of resources in a Bicep file as you add code. This can be especially helpful when working with resources like the Function app, which requires a serverFarmId property.
Resource Manager templates, also known as ARM templates, had to be written in a special JSON format until Bicep was available. This made it difficult to get started with writing ARM templates in JSON.
The VSCode Bicep Extension is also capable of picking up dependencies and drawing links between resources, making it easier to see how different resources are related. This is especially useful when working with complex resource dependencies.
Behind the scenes, Resource Manager still operates based on the same JSON templates, even when using Bicep. The Bicep tooling converts your template to a JSON format in a process called transpilation.
Additional reading: Azure Bicep Existing Resource
Multiple-Line Declarations
You can now use multiple lines in function, array, and object declarations, a feature that requires Bicep CLI version 0.7.X or higher.
This feature allows for more readable and maintainable code. For example, the resourceGroup() definition can be broken into multiple lines.
The Bicep CLI version 0.7.X or higher is required to take advantage of multiple-line declarations.
You might like: Azure Cli Upload File to Blob Storage
Template Creation and Management
To create and manage Azure Bicep templates, you'll need to start with a JSON template, which Azure Resource Manager still operates on. You can define Azure resources in the Bicep template, and Bicep will perform the conversion to JSON for you.
You can deploy a Bicep template using Azure PowerShell with the New-AzResourceGroupDeployment command, specifying the resource group and template file. For example, you can use the -nameFromTemplate parameter to specify the storage account name for the template.
To deploy using the Azure CLI, you can use the az deployment group create command, specifying the resource group name and template file. You can use the --parameters option to specify the storage account name for the template.
For your interest: Tutorial on Azure Storage
Parameters and Variables
Parameters and variables are the backbone of dynamic and reusable Bicep templates. They allow you to specify values at deployment time, making your templates more flexible and adaptable to different environments.
You can declare parameters using the `param` keyword, and variables using the `var` keyword. For example, you can declare a parameter named `name` and a variable named `stgAcctName` using the following syntax: `param name stringvar stgAcctName = concat(name, '2468')`.
Bicep templates use the same parameter files written in JSON as ARM templates, making it easy to reuse your existing ARM parameter files.
Parameters can have default values, such as the `location` parameter which defaults to `westus2` if no value is provided. You can also add constraints to parameters, like the `name` parameter which has a minimum length of 3 and a maximum length of 24 characters.
Here are some examples of parameter types:
- `string`
- `integer`
- `boolean`
- `object`
- `array`
You can also use decorators to add more functionality to your parameters and variables, such as specifying allowed values or default values.
To make your Bicep file more readable, you can encapsulate complex expressions in variables. For example, you can create a variable to construct a resource name by concatenating several values together.
Here is an example of how you can declare parameters and variables in your Bicep template:
```
param environment string = 'prod'
param location string = resourceGroup().location
var storageAccountName = concat(environment, 'StorageAccount')
resource storageAccount 'Microsoft.Storage/storageAccounts@2021-02-01' = {
name: storageAccountName
location: location
kind: 'StorageV2'
sku: {
name: 'Standard_LRS'
tier: 'Standard'
}
}
```
Creating Files
Creating files is a crucial step in template creation. To get started, you'll want to create a file with a .bicep extension, such as storageAccount.bicep.
The Bicep extension for Visual Studio Code provides language support and resource autocompletion. This means you'll get intellisense for virtual machine properties, making it easier to define them in your template.
In Visual Studio Code, you can install the Bicep extension by searching for "bicep" in the marketplace and installing the Microsoft-provided extension. This will give you the necessary tools to create and validate Bicep files.
To create a Bicep file, think about your dependency tree and the order in which you'll create your resources. You can use the VSCode Bicep extension to visualize the resources you're creating.
A fresh viewpoint: Yaser Adel Mehraban Infrastructure as Code with Azure Bicep Pdf
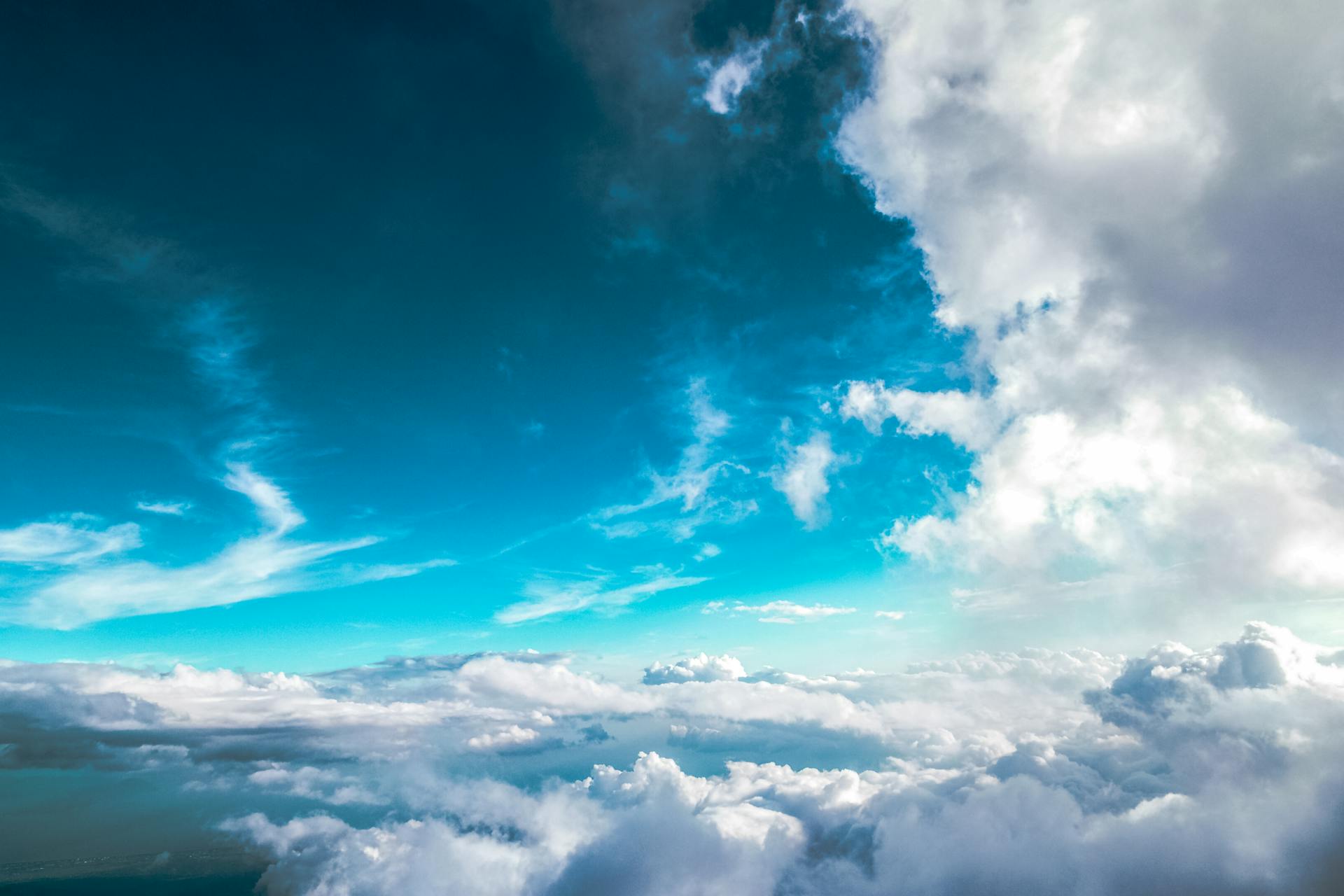
Here's a step-by-step guide to installing the Bicep extension:
- In Visual Studio Code, select the Extension icon on the left side.
- Search the marketplace for "bicep."
- Find the Bicep extension from Microsoft, and click Install.
With the Bicep extension installed, you'll have access to autocomplete entries for resource properties, making it easier to write your Bicep templates.
Using the Template
You can deploy a Bicep template to Azure using either Azure PowerShell or the Azure CLI.
To deploy using Azure PowerShell, you'll need to specify the resource group and template file using the New-AzResourceGroupDeployment command. You can also use inline parameters to specify values for template parameters.
The New-AzResourceGroupDeployment command has a -Name parameter, but you can use -nameFromTemplate to specify the parameter for the Bicep template. This is useful when the template has default values for certain parameters.
To deploy using the Azure CLI, you'll need to specify the resource group and template file using the az deployment group create command. You'll also need to use the --parameters option to specify the values for template parameters.
Here are the basic steps for deploying a Bicep template using both Azure PowerShell and the Azure CLI:
Make sure to replace the placeholder values with the actual values for your deployment.
Converting Templates
You can manually convert a Bicep template to an ARM template using the bicep build command specifying the path to the Bicep template file. This process is called transpilation, which means converting one programming language to a different language.
The bicep build command can convert the storageAccount.bicep Bicep template to an ARM template. You can then deploy the converted ARM template using the Azure PowerShell New-AzResourceGroupDeployment command or the Azure CLI az deployment command.
To convert an existing ARM JSON template to a Bicep template, use the bicep decompile command. This command is a best-effort process, and Microsoft does not guarantee a direct mapping from ARM JSON to Bicep.
You can use the bicep decompile command to convert the storageAccount.json ARM template to a Bicep template. However, you will need to review the Bicep template and fix warnings and errors that may have occurred during the decompilation process.
Here are the commands to manually convert a Bicep template to an ARM template and to convert an ARM template to a Bicep template:
- To convert a Bicep template to an ARM template: `bicep build storageAccount.bicep`
- To convert an ARM template to a Bicep template: `bicep decompile storageAccount.json`
Note that you can report any issues or inaccuracies found during the decompilation process on the Bicep GitHub Issues page.
Parameters and Variables
Parameters and variables are the backbone of making your Azure Bicep templates more dynamic and reusable. You can specify values at deployment time instead of hard-coding resource values.
You declare parameters using the param keyword, and variables using the var keyword. For example, you can declare a parameter called name and a variable named stgAcctName, which performs a string concatenation of the name parameter and some numbers.
Here are some examples of Bicep parameters called name and location, and a variable named stgAcctName:
- param name string
- param location string = 'westus2'
- var stgAcctName = concat(name, '2468')
You can also add constraints to your parameters, such as minimum and maximum length for the name parameter, and allowed values for the SKU parameter.
For example, the name parameter can have a minimum length of 3 and a maximum length of 24 characters, and the SKU parameter can have allowed values of 'Standard_LRS' and 'Premium_LRS'.
Here are some examples of parameter declarations with constraints:
- This parameter takes the location of the resource group as its default value.
- This parameter declares the name of the storage account with constraints on the length (minimum 3 and maximum 24 characters).
- This parameter defines the type of the storage account with a set of allowed values. The default value is set to ‘Standard_LRS’.
You can also use default values for your parameters, which are used if no value is provided during deployment.
For example, you can add a default value for the location parameter using the resourceGroup() function, which defaults to the resource group's location if you don't specify the location at deployment time.
Here's an example of a parameter declaration with a default value:
- param location string = 'westus2'
In addition to parameters, you can also use variables to make your Bicep templates more readable and maintainable. Variables store intermediate values that can be used throughout your template.
For example, you can add a variable for a resource name that's constructed by concatenating several values together, and use this variable wherever you need the complex expression.
Here's an example of a variable declaration:
- var stgAcctName = concat(name, '2468')
By using parameters and variables, you can make your Azure Bicep templates more dynamic, reusable, and maintainable, and reduce the complexity of your templates.
Modules
Modules are a powerful feature in Azure Bicep that allow you to reuse code from one Bicep file in another.
You can create your own modules by encapsulating and reusing resource definitions, making it easier to manage complex deployments.
A module is a separate Bicep file that can be linked to from other Bicep files, enabling you to reuse code and resources.
To create a module, simply move a resource definition into a new Bicep file, such as storage.bicep, and then update the main Bicep file to use the module.
In the main Bicep file, you can use the module keyword to import the module and pass parameters to it, making it easy to manage complex deployments.
Modules can also be used to create reusable components, such as app settings, that can be used across multiple resources.
You can create a new Bicep file, like appservice-appsettings-config.bicep, to set app settings on the slots, and then use the module keyword to call it from the main Bicep file.
By using modules, you can break down complex deployments into smaller, reusable components, making it easier to manage and maintain your infrastructure.
On a similar theme: Azure Azure-common Python Module
Compile and Deploy
To compile your Bicep file, use the bicep build command to generate an ARM template equivalent.
This command will create a file named main.json in the same directory as your Bicep file, which is the ARM template equivalent.
You can also use the bicep build command to convert a Bicep template to an ARM template, specifying the path to the Bicep template file.
For example, to convert a Bicep template to an ARM template, run the command: bicep build storageAccount.bicep
The bicep build command can be used to manually convert a Bicep template to an ARM template.
To deploy your Bicep template, you can use either Azure PowerShell or Azure CLI. In both cases, you'll need to connect to your Azure tenant and select the subscription with the resource group you want to deploy to.
Here are the steps to deploy using Azure PowerShell:
1. Connect to your Azure tenant using the Connect-AzAccount command.
You might like: Azure Data Studio Connect to Azure Sql
2. Select the subscription with the resource group using the Set-AzContext command.
3. Use the New-AzResourceGroupDeployment command specifying the -ResourceGroupName and -TemplateFile path.
And here are the steps to deploy using Azure CLI:
1. Connect to your Azure tenant using the az login command.
2. Select the subscription with the resource group using the az account set command.
3. Use the az deployment group create command specifying the --resource-group and --template-file path.
When deploying your Bicep template, you can specify template parameter values using inline parameters with Azure PowerShell, or using the --parameters option with Azure CLI.
For example, to deploy a Bicep template using Azure CLI, you can use the following command: az deployment group create --resource-group bicep-rg --template-file storageAccount.bicep --parameters name='stgacctbicepazcli'
A fresh viewpoint: Azure Networking Learning Path
Sources
- https://www.varonis.com/blog/azure-bicep
- https://vaibhavji.medium.com/an-introduction-to-azure-bicep-a-hands-on-tutorial-5882e6c2ce85
- https://learn.microsoft.com/en-us/azure/azure-resource-manager/bicep/file
- https://4sysops.com/archives/azure-bicep-getting-started-guide/
- https://www.voitanos.io/blog/how-to-create-azure-function-apps-with-bicep-step-by-step/
Featured Images: pexels.com