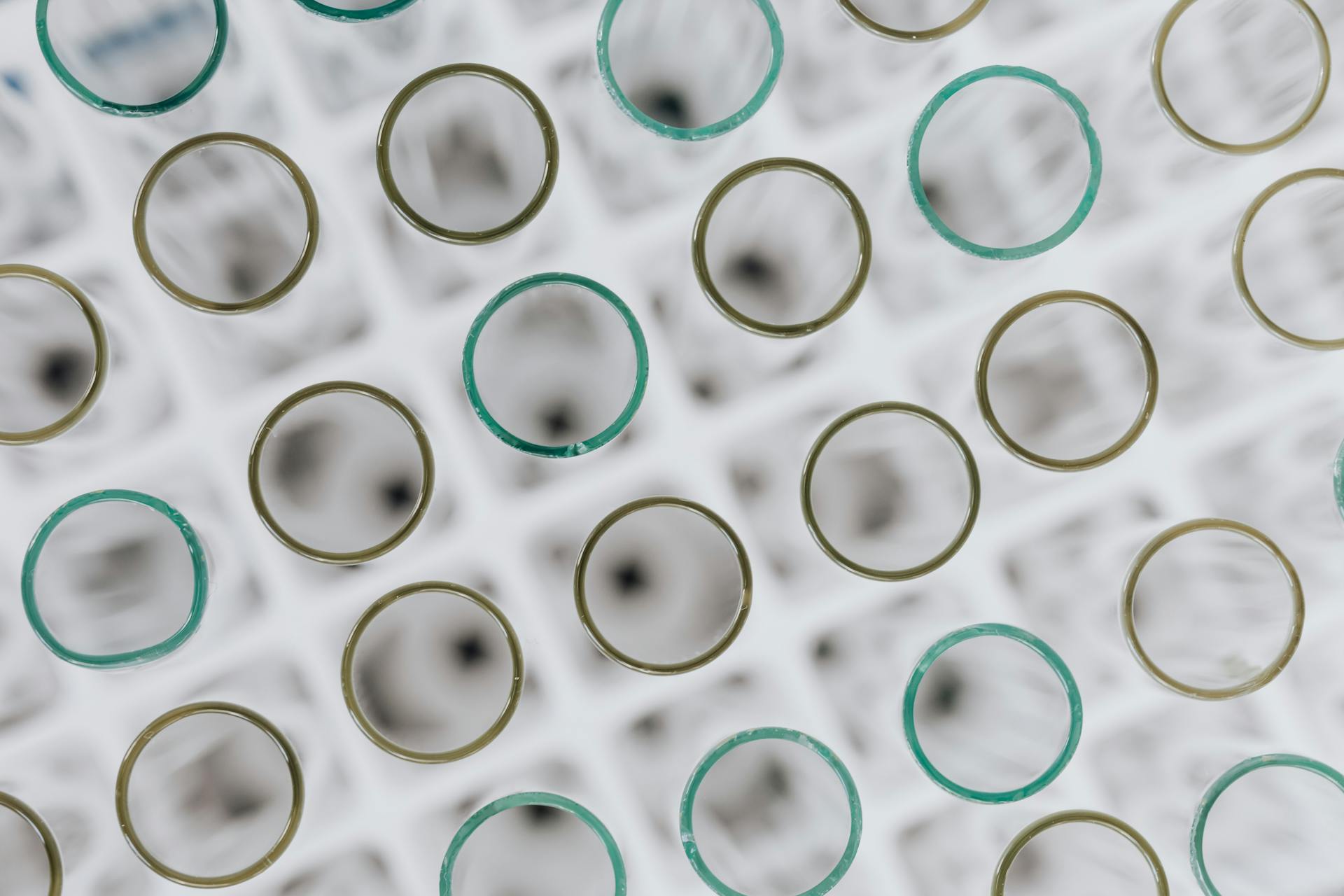
Building and deploying Azure Flask web applications can be a straightforward process.
Azure provides a managed platform for Flask applications, allowing for easy deployment and scaling. This includes support for HTTP/2 and gunicorn.
To start, you'll need to create an Azure App Service. This can be done through the Azure portal or using the Azure CLI.
You'll then need to configure your Flask application to run on the App Service. This involves creating a requirements.txt file and a runtime.txt file.
Once configured, you can deploy your application to the App Service using a Git repository.
You might enjoy: How to Deploy Flask App in Azure
Getting Started
To get started with Azure Flask, you'll need to have a few things in place. First and foremost, you'll need an Azure account where you can deploy a web app to Azure App Service and Azure Container Registry.
If you don't already have an Azure subscription, don't worry – you can create a free account before you begin. Having an Azure account will give you access to the necessary tools and resources to deploy your Flask app.
Worth a look: Azure Developer Account
You'll also need to install Azure CLI to create a Docker image and deploy it to App Service. This will allow you to manage your Azure resources and deploy your app with ease.
Additionally, you may also want to install Docker and the Docker CLI to create a Docker image and test it in your local environment. This will give you a chance to test and debug your app before deploying it to Azure.
Here are the prerequisites you'll need to get started:
- An Azure account where you can deploy a web app to Azure App Service and Azure Container Registry.
- Azure CLI to create a Docker image and deploy it to App Service.
- Docker and the Docker CLI to create a Docker image and test it in your local environment (optional).
Creating a Web App
To create a web app, you can use the Azure portal or the Azure CLI. In the portal, click on "App Services" in the left navigation bar, then "Add", and select "Web App". Fill in the "Create Web App" form with the app name, subscription, resource group, Linux as OS, Code as publishing mode, and Python 3.x as runtime stack.
You'll also need to create an App Service Plan, which defines the compute resources for your web app. For a basic demo, the Basic tier is a suitable option. You can do this by clicking the App Service plan button and filling in the form with the pricing tier.
You can also create an App Service plan using the Azure CLI with the `az appservice plan create` command. This will create a plan with the specified name, resource group, and pricing tier.
If this caught your attention, see: Azure Static Apps
Create a Web
To create a web app, you can use the Azure portal or the Azure CLI. On the portal, click on "App Services" in the left navigation bar and then click on "Add" to create a new web app. Select "Web App" and click "Create" to start the process.
You'll need to enter the app name, select your subscription and existing resource group, and choose Linux as the OS. You'll also need to select the publishing mode and runtime stack, such as Python 3.x.
To create an App Service Plan, click on the App Service plan button and then click on "Create new" to fill out the form. This will define a set of compute resources for your web app to run on.
Here are the steps to create an App Service Plan using the Azure CLI:
1. Create an App Service plan with the `az appservice plan create` command.
2. Set an environment variable to your subscription ID using the `az account show` command.
For your interest: Azure App Service Plans
3. Create the web app with the `az webapp create` command, specifying the App Service plan, resource group, and other details.
Here's a summary of the Azure CLI commands:
Remember to replace the placeholders with your own values and to use the correct syntax for your environment.
Database and Authentication
The sample web app uses DefaultAzureCredential to authenticate to two different data stores: Azure blob storage and Azure Database for PostgreSQL - Flexible Server. This allows the app to run under different identities depending on the environment, without making changes to the code.
In a local development environment, the app can run under the identity of the developer signed in to the Azure CLI, while in Azure, it can run under its system-assigned managed identity. The security principal that the app runs under must have a role on each Azure resource that permits it to perform the actions on the resource that the app requires.
Readers also liked: Azure Environment Setup and Adf Setup
The app uses service connectors to automatically enable the system-assigned managed identity on the Azure resources and assign it appropriate roles. This enables the app to use DefaultAzureCredential to authenticate with the required Azure resources.
Here's a summary of the authentication process:
Access Settings via Environment Variables
Accessing settings via environment variables is a convenient way to store and retrieve sensitive information, such as API keys and endpoints, in your app code.
You can define these variables in App Settings within the Azure portal, which makes them available to your app as environment variables.
In the Azure portal, navigate to your web app and select Configuration under Settings on the left pane. From there, you can create new application settings and name them COG_SERVICE_KEY, COG_SERVICE_REGION, COG_SERVICE_ENDPOINT, and ENDPOINT.
These environment variables can be accessed using the os.environ method in your app code, which is a more secure way to handle sensitive information than hardcoding it into your code.
If this caught your attention, see: Azure Function Local Settings Json
To access these variables, you'll need to modify your app code to use the os.environ method, like this: key = os.environ["COG_SERVICE_KEY"], region = os.environ["COG_SERVICE_REGION"], endpoint = os.environ["ENDPOINT"], and COG_endpoint = os.environ["COG_SERVICE_ENDPOINT"].
Here's a summary of the steps to access app settings via environment variables:
Set up PostgreSQL Server
To set up a PostgreSQL server, you'll need to create a resource group and a PostgreSQL server using Azure CLI commands.
First, you'll need to set up environment variables for the tutorial, including LOCATION, RAND_ID, RESOURCE_GROUP_NAME, APP_SERVICE_NAME, DB_SERVER_NAME, ADMIN_USER, and ADMIN_PW.
The ADMIN_PW must contain 8 to 128 characters from three of the following categories: English uppercase letters, English lowercase letters, numbers, and nonalphanumeric characters.
You can create a resource group with the az group create command, specifying the location and name of the resource group.
Here's a step-by-step guide to creating a PostgreSQL server:
- Create a PostgreSQL server with the az postgres flexible-server create command, specifying the resource group, name, location, admin user, and admin password.
- Create a database named restaurant using the az postgres flexible-server execute command, specifying the name of the PostgreSQL server, admin user, admin password, and database name.
The sku-name for the PostgreSQL server is the name of the pricing tier and compute configuration.
Examine Authentication Code
Authentication code is a crucial part of any web app, especially when it comes to accessing sensitive data stores like Azure blob storage and Azure Database for PostgreSQL.
The sample web app in this tutorial uses DefaultAzureCredential to authenticate to both data stores, which allows it to run under different service principals depending on the environment. This is a game-changer for developers, as it eliminates the need to make code changes to accommodate different environments.
In a local development environment, the app can run under the identity of the developer signed in to the Azure CLI, while in Azure, it can run under its system-assigned managed identity.
To authenticate to Azure resources, the security principal that the app runs under must have a role on each Azure resource that permits it to perform the required actions.
Here's a breakdown of the authentication process:
The app uses DefaultAzureCredential to acquire access tokens to perform operations against Azure storage, and to get an access token for Azure Database for PostgreSQL in the get_conn.py file.
By using DefaultAzureCredential, the app can automatically authenticate to the required Azure resources without requiring any additional code changes.
Passwordless Connectors
Passwordless Connectors are a game-changer for secure authentication. They allow you to configure Azure resources to use managed identity and Azure role-based access control.
To create a passwordless connector, you'll need to use the Service Connector commands. These commands configure Azure Storage and Azure Database for PostgreSQL resources to use managed identity and Azure role-based access control.
You can add a PostgreSQL service connector using the `az webapp connection create postgres-flexible` command. This command uses the system-assigned managed identity to authenticate the web app to the target resource, PostgreSQL.
Here are the steps to add a PostgreSQL service connector:
- Add a PostgreSQL service connector with the `az webapp connection create postgres-flexible` command.
- Add a storage service connector with the `az webapp connection create storage-blob` command.
With a passwordless connector, your web app can write images to a container using its system-assigned managed identity for authentication and authorization. This is a huge security win, as you don't have to worry about storing or managing passwords.
The `az webapp connection create storage-blob` command also adds a storage account and adds the web app with role Storage Blob Data Contributor to the storage account. This allows your web app to access the storage account and retrieve images for display.
See what others are reading: Azure Web App Asp.net V4.8
Frequently Asked Questions
What is Flask tool used for?
Flask is a lightweight framework for web development, ideal for building dynamic websites, APIs, and microservices. It's a popular choice for development teams due to its flexibility and feature-rich offerings.
Sources
- https://techcommunity.microsoft.com/t5/educator-developer-blog/deploy-a-flask-ai-web-app-to-azure-app-service/ba-p/3274448
- https://medium.com/@nikovrdoljak/deploy-your-flask-app-on-azure-in-3-easy-steps-b2fe388a589e
- https://learn.microsoft.com/en-us/azure/developer/python/tutorial-containerize-simple-web-app-for-app-service
- https://stackoverflow.com/questions/78000012/deploy-flask-python-on-azure-webapp
- https://learn.microsoft.com/en-us/azure/developer/python/tutorial-python-managed-identity-cli
Featured Images: pexels.com