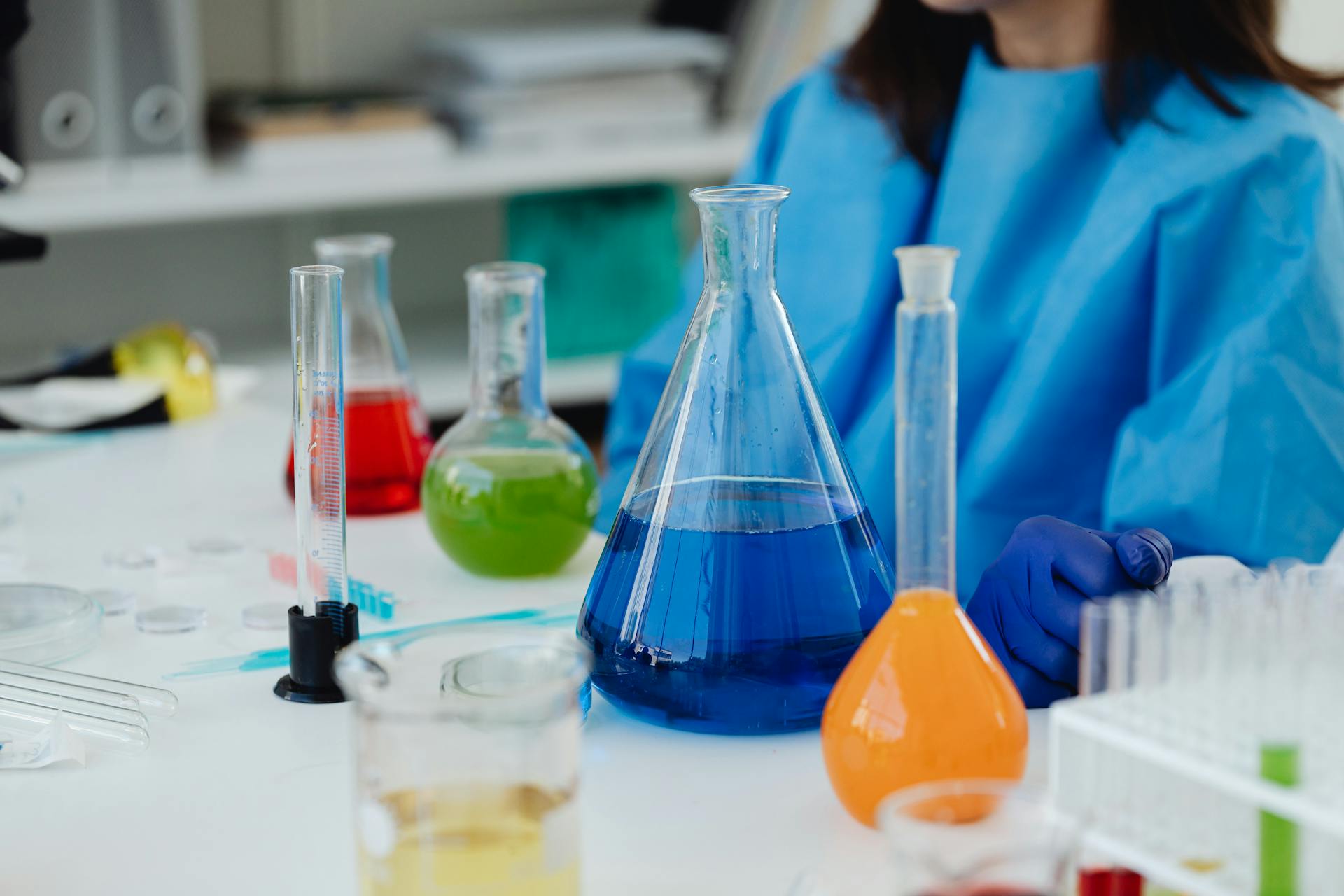
Flask is a popular Python web framework, and Azure is a leading cloud platform, making it a great combination for deploying web applications.
You can create a Flask app and deploy it to Azure using various methods, including Azure App Service and Azure Functions.
To start, you'll need to create a new Azure App Service and configure it to run your Flask app.
Azure supports a wide range of Python versions, including Python 3.9, which is the recommended version for Flask apps.
Recommended read: Azure Flask
Prerequisites
To get started with deploying your Flask app in Azure, you'll need to meet some prerequisites.
You'll need an Azure account where you can deploy a web app to Azure App Service and Azure Container Registry. If you don't have an Azure subscription, create a free account before you begin.
You'll also need to install the Azure CLI to create a Docker image and deploy it to App Service. Optionally, you can use Docker and the Docker CLI to create a Docker image and test it in your local environment.
Here are the specific tools you'll need to get started:
- Windows, Mac, or Linux
- Git
- Text/Code Editor (Notebook, atom.io, Visual Studio, etc.)
For Git on Windows, we recommend using Git for Windows or GitHub for Windows.
Preparing the Environment
To prepare your environment for deploying a Flask app in Azure, you'll need to create a virtual environment for your application. This can be done using a tool like virtualenv, but for macOS or Linux, you can simply create a virtual environment by running a command in your terminal.
Clone your web app repository from GitHub to get started. Navigate to your web app's folder once it's cloned. You'll need to install dependencies for your Flask app, which can be done by installing the dependencies listed in requirements.txt.
Here's a step-by-step guide to installing dependencies:
- Install dependencies from requirements.txt
Next, access your application locally by opening a web browser and visiting http://localhost:5000. You'll know your deployment is complete when you receive a notification in the lower right corner of Visual Studio Code.
Creating a Resource Group
You'll need to create a resource group to deploy your Flask app in Azure.
To create a resource group, you'll need to run the az login command to sign in to Azure. This step is crucial to authenticate your Azure CLI.
Discover more: Azure Create New App Service
Next, run the az upgrade command to ensure your Azure CLI version is current. This will guarantee you have the latest features and updates.
Create a group with the az group create command, specifying a name and location. For example, you can create a group with the name "web-app-simple-rg" and location "eastus" by running az group create --name web-app-simple-rg --location eastus.
Here are the basic steps to create a resource group:
- Run az login to sign in to Azure
- Run az upgrade to ensure your Azure CLI version is current
- Run az group create to create a group with a specified name and location
Deploying to Azure
Deploying to Azure is a straightforward process, especially when you're using Azure CLI. To create an App Service plan, you can use the az appservice plan create command, specifying the name, resource group, SKU, and operating system.
First, create an App Service plan with the az appservice plan create command, using the --name, --resource-group, --sku, and --is-linux parameters. For example, you can create a plan called "webplan" in the "web-app-simple-rg" resource group with the B1 SKU and Linux operating system.
For more insights, see: Wordpress Azure App Service Linux Login Authentication
You'll also need to set an environment variable to your subscription ID, which will be used in the --scope parameter of the next command. You can do this using the az account show command and setting the SUBSCRIPTION_ID variable.
To create the web app, you'll need to use the az webapp create command, specifying the resource group, plan, name, and other parameters. For example, you can create a web app called "webappsimple123" in the "web-app-simple-rg" resource group, using the "webplan" plan and assigning an identity with the AcrPull role.
Here's a summary of the steps:
Make sure to replace the placeholders with your actual values.
Build and Deployment
To build and deploy your Flask app in Azure, you'll need to start by creating an Azure App Service plan using the az appservice plan command. This will set up the necessary infrastructure for your app to run on.
You'll also need to create an environment variable to store your subscription ID, which is used in the --scope parameter of the next command. This can be done using the az account show command in the Bash shell.
Explore further: Azure App Service Plans
Next, you'll need to build the Docker image in Azure using the az acr build command. This command uses the Dockerfile in the current directory and pushes the image to the registry. The --registry option specifies the registry name, and the --image option specifies the image name in the format registry.azurecr.io/repository:tag.
Here are the steps to create the web app in Azure:
- Create an App Service plan with the az appservice plan command.
- Create the web app with the az webapp create command.
You can also use the Azure portal or Azure CLI to set up the necessary settings for your app. For example, you'll need to set the SCM_DO_BUILD_DURING_DEPLOYMENT app setting to true to enable build automation.
To deploy your Flask web app on Azure, you'll need to create an Azure App Service web app in Azure. You can do this using the Azure portal, VS Code, or Azure CLI. Make sure you have the Azure Tools extension pack installed and are signed in to Azure from VS Code.
Here are some best practices to keep in mind when deploying your Flask web app on Azure:
- Consider using Gunicorn as your Flask application server.
- Implement Flask caching mechanisms to improve Flask response times.
- Explore Azure CDN for serving Flask static assets efficiently.
To debug your Flask application locally, you can use tools like Visual Studio Code. It's also a good idea to implement robust error handling and clear error messages to ensure a smooth user experience.
You might like: Azure App Gateway Cutom Error Pages
A Container
To deploy your Flask app in Azure, you'll need to create a container in your storage account. This allows you to store and serve your app's files securely.
Update your storage account to allow anonymous read access to blobs. You can do this using the az storage account update command, specifying the name of your storage account and resource group.
Enabling anonymous access on the storage account doesn't affect access for individual blobs. You must explicitly enable public access to blobs at the container-level.
Create a container called photos in the storage account, allowing anonymous read (public) access to blobs. You can use the az storage container create command to do this, specifying the name of your storage account, container name, and public access setting.
Note that several Azure roles permit you to create containers in a storage account, including "Owner", "Contributor", "Storage Blob Data Owner", and "Storage Blob Data Contributor".
If this caught your attention, see: Azure Logic App Blob Storage Trigger
Security and Authentication
Security and Authentication is a top priority when deploying a Flask app in Azure. You can use DefaultAzureCredential to authenticate to Azure resources such as Azure blob storage and Azure Database for PostgreSQL - Flexible Server database.
To ensure secure hosting, Azure App Service provides a robust array of security features including HTTPS support, authentication with Azure AD, and a Web Application Firewall (WAF) to protect against common vulnerabilities and attacks.
To create passwordless connectors to Azure resources, you can use Service Connector commands to configure Azure Storage and Azure Database for PostgreSQL resources to use managed identity and Azure role-based access control. This allows your web app to authenticate to these resources using its system-assigned managed identity.
Here are the steps to create a PostgreSQL service connector and a storage service connector:
- Add a PostgreSQL service connector with the az webapp connection create postgres-flexible command.
- Add a storage service connector with the az webapp connection create storage-blob command.
Examine Authentication Code
In a web app, authentication code is crucial for secure access to data stores. The sample web app in this tutorial needs to authenticate to two different data stores: Azure blob storage and Azure Database for PostgreSQL - Flexible Server.
To do this, the app uses DefaultAzureCredential, which allows it to run under different service principals depending on the environment. For example, in a local development environment, the app can run under the identity of the developer signed in to the Azure CLI.
DefaultAzureCredential must be configured to run under a security principal that has a role on each Azure resource used by the app. In this tutorial, service connectors are used to enable the system-assigned managed identity on the app in Azure and assign it the required roles.
Here are the Azure resources used by the app, along with the roles required for each:
- Azure blob storage: Storage Blob Data Contributor role
- Azure Database for PostgreSQL - Flexible Server: PostgreSQL Server Admin role
The app uses DefaultAzureCredential to acquire access tokens for these resources, which are then used to perform operations against Azure storage and the PostgreSQL database.
Passwordless Access
You can configure Azure resources to use managed identity and Azure role-based access control, eliminating the need for passwords. This is achieved through Service Connector commands.
These commands create app settings in the App Service that connect your web app to Azure Storage and Azure Database for PostgreSQL resources. The output from the commands lists the service connector actions taken to enable passwordless capability.
To add a PostgreSQL service connector, use the az webapp connection create postgres-flexible command. This command authenticates the web app to the target resource, PostgreSQL, using the system-assigned managed identity.
A storage service connector is added using the az webapp connection create storage-blob command. This command also creates a storage account and adds the web app with role Storage Blob Data Contributor to the storage account.
Here's a summary of the steps to create a passwordless connector to Azure resources:
- Add a PostgreSQL service connector with the az webapp connection create postgres-flexible command.
- Add a storage service connector with the az webapp connection create storage-blob command.
The app uses its system-assigned managed identity for authentication and authorization when writing images to the container. This identity is configured to access the storage account for the browser to display photos.
Testing and Updates
Testing and updates are crucial parts of deploying a Flask app in Azure. After making code changes, you can redeploy to App Service with the az acr build and az webapp update commands.
To test the Python web app in Azure, browse to the deployed application at the URL http://$APP_SERVICE_NAME.azurewebsites.net. It can take a minute or two for the app to start.
You can test the functionality of the sample app by adding a restaurant and some reviews with photos for the restaurant. The restaurant and review information is stored in Azure Database for PostgreSQL and the photos are stored in Azure Storage.
Test the Python
To test the Python web app in Azure, start by browsing to the deployed application at the URL http://$APP_SERVICE_NAME.azurewebsites.net. It can take a minute or two for the app to start.
If you see a default app page that isn't the default sample app page, wait a minute and refresh the browser. This will ensure you're seeing the correct application.
To test the functionality of the sample app, add a restaurant and some reviews with photos for the restaurant. The restaurant and review information is stored in Azure Database for PostgreSQL and the photos are stored in Azure Storage.
Here's a step-by-step guide to testing the app:
- Browse to the deployed application at the URL http://$APP_SERVICE_NAME.azurewebsites.net.
- Test the functionality of the sample app by adding a restaurant and some reviews with photos.
Make Updates
After you make code changes, you can redeploy to App Service with the az acr build and az webapp update commands. This process is straightforward and efficient.
Redeploying your application ensures that any new features or bug fixes are available to users.
Sources
- https://learn.microsoft.com/en-us/azure/app-service/quickstart-python
- https://learn.microsoft.com/en-us/azure/developer/python/tutorial-containerize-simple-web-app-for-app-service
- https://learn.microsoft.com/en-us/azure/developer/python/tutorial-python-managed-identity-cli
- https://vivasoftltd.com/how-to-deploy-flask-web-app-on-azure/
- https://github.com/Cojacfar/FlaskWeb
Featured Images: pexels.com