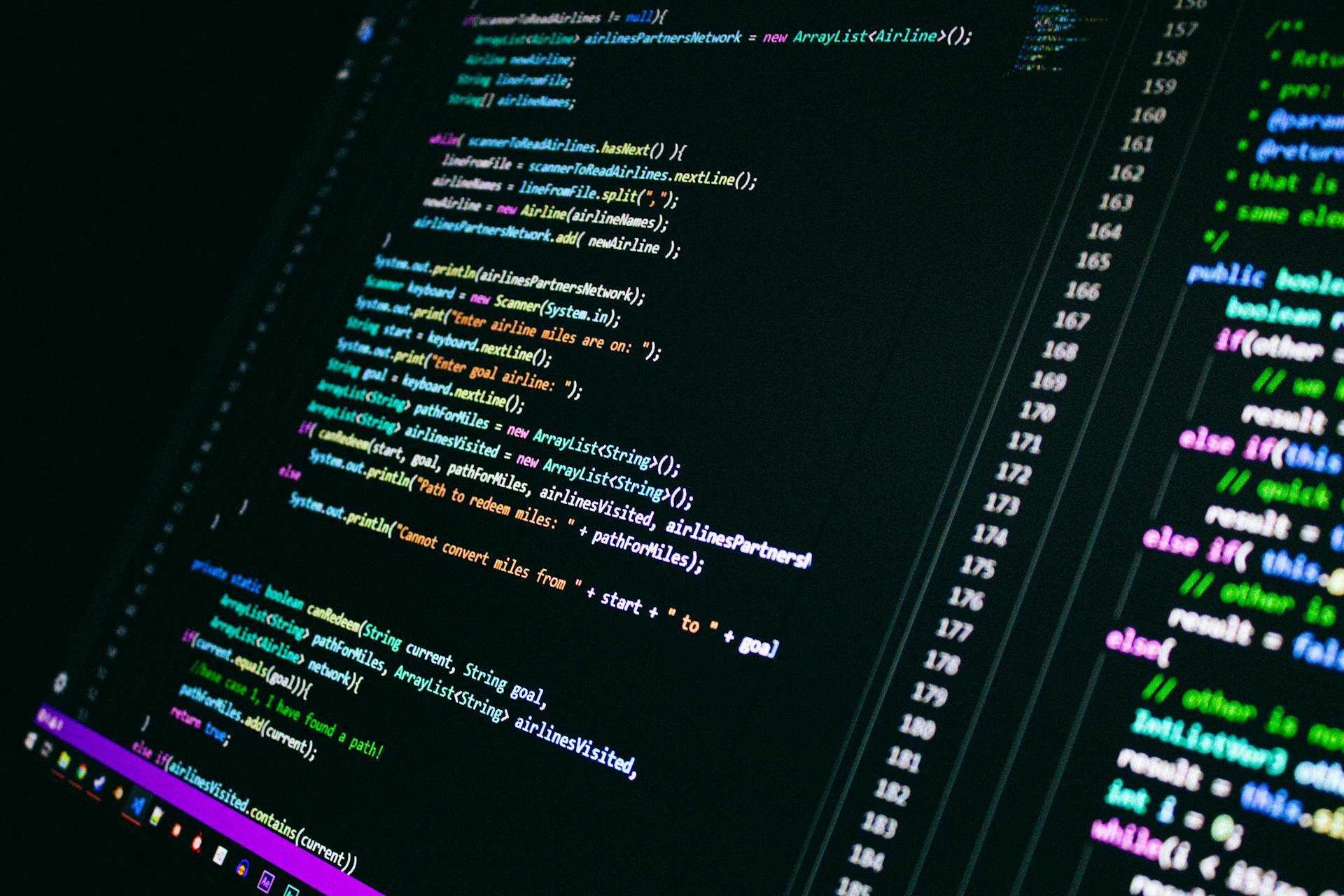
To get started with Azure Go SDK for Speech Services, you'll need to install the SDK using the go get command. This command downloads and installs the SDK and its dependencies.
The Azure Go SDK for Speech Services provides a simple and intuitive way to interact with Azure Speech Services. You can use it to build applications that can recognize and transcribe speech, as well as synthesize text into speech.
First, ensure you have the Azure Go SDK installed on your machine. If not, you can install it by running the command `go get github.com/Azure/azure-sdk-for-go/sdk/speech`. This command downloads and installs the SDK and its dependencies.
The Azure Go SDK for Speech Services uses a client library to interact with the Azure Speech Services. This library provides a set of APIs that you can use to perform tasks such as speech recognition and synthesis.
Prerequisites and Setup
To get started with the Azure Go SDK, you'll need to meet some prerequisites. You'll need to have the Azure Developer CLI, Docker Desktop, and Go 1.21 or newer installed on your machine.
If you don't have an Azure account, you can create a free account before you begin. This will give you access to the necessary tools and resources.
Here are the specific tools you'll need:
- Azure Developer CLI
- Docker Desktop
- Go 1.21 or newer
Once you have these tools installed, you'll be ready to move on to the next step.
Azure Speech SDK
The Azure Speech SDK is a powerful tool that can help you convert speech to text. It's a great addition to the Azure Go SDK.
To use the speech service, you'll need to create a NewWrapper constructor, where you can set the language autodetection to Finnish, as the author did in their example. This can help prevent confusion with other languages.
You'll also need to provide the key and region of the Speech service, and then call the function to process your file. The author used a goroutine to pump the contents of the file to a stream that the speech SDK can read.
When the SDK produces events during reading, you can choose what to do with them. In the author's case, they wanted to concatenate the results in a slice of strings. Just be aware that there might be bugs in the code, like the author mentioned.
Installing Speech SDK
Installing the Speech SDK can be a bit tricky, especially on a Mac where the official documentation doesn't provide any guidance.
You'll need to use CGO, which is a C compiler for Go, and this can take some tinkering to get working.
CGO requires a C++ library that the SDK depends on, so you'll need to install that first.
If you're on Debian or Ubuntu, you'll also need to install OpenSSL 1.x.
Installing the Go packages is the final step in the process.
Integrating Speech-to-Text with the Speech Service
The Speech service can be used in your code for speech-to-text, but the quickstart might be a bit tricky to follow. Thankfully, the examples provide a ready-made wrapper you can augment.
You'll need to set the autodetection of the language of the speech to Finnish in the NewWrapper constructor, as it's essential for accurate results. This is especially important when dealing with languages that might get confused with others, like Finnish.
To create a new wrapper, you'll need the key and the region of the Speech service. You can then call the function to process your file found at an URL.
You can also set up a goroutine to pump the contents of the file to a stream the speech SDK will read. This allows for efficient processing of large files.
When the SDK produces events during reading, you can specify what should happen to the results. In this case, the results are being concatenated in a slice of strings.
Authentication and Authorization
To authenticate with Azure, you need to create a credential using the azidentity package. This can be done using the DefaultAzureCredential instance, which relies on environment variables set earlier in the process.
You can authenticate using client/secret, certificate, or managed identity, but DefaultAzureCredential is the default option. In your Go code, you create an azidentity object to facilitate authentication.
Once you have a credential from Azure Identity, you can create a client to connect to the target Azure service. This involves installing the relevant package, such as armcompute for Azure Compute service, and using its NewClient method to create a client.
Go Packages and Resources
The Azure Go SDK offers a wide range of packages that can be used to interact with Azure services. These packages are categorized into four main groups: Client - New Releases, Client - Previous Versions, Management - New Releases, and Management - Previous Versions.
You can find the most up-to-date list of new packages on the latest page, which is located in the repository under the sdk directory. The new libraries can be identified by their location under the sdk directory.
The last stable versions of packages are production-ready and can be used to ensure your code is ready for production use. These libraries might not implement the Azure Go SDK guidelines or have the same feature set as the New releases, but they do offer a wider coverage of services.
Here are the locations of the previous Go SDK packages and management libraries:
To create a Resource Management client, you need to have a credential from Azure Identity. This credential is used to connect to the target Azure service. For example, to connect to the Azure Compute service, you can use the armcompute.NewVirtualMachinesClient type to create a client to manage virtual machines.
The same pattern is used to connect with other Azure services. For example, you can install the armnetwork package and create a virtual network client to manage virtual network (VNET) resources.
Azure Client and Resource Management
To create an Azure client, you need a credential from Azure Identity. This credential is used to connect to the target Azure service.
You can create a client to manage virtual machines by using the armcompute.NewVirtualMachinesClient type. This client groups a set of related APIs, providing access to its functionality within the specified subscription.
To connect with other Azure services, you can use the same pattern. For example, you can install the armnetwork package and create a virtual network client to manage VNET resources.
Install Client Library
To install the client library, start by opening a terminal and navigating to the /src folder. You can do this by typing `cd ./src`.
If you haven't already installed the azcosmos package, use the command `go install github.com/Azure/azure-sdk-for-go/sdk/data/azcosmos` to install it. Similarly, if you haven't already installed the azidentity package, use the command `go install github.com/Azure/azure-sdk-for-go/sdk/azidentity` to install it.
You can verify that the packages have been installed correctly by opening and reviewing the `src/go.mod` file. This file should contain entries for `github.com/Azure/azure-sdk-for-go/sdk/data/azcosmos` and `github.com/Azure/azure-sdk-for-go/sdk/azidentity`.
Resource Management Client
To authenticate with Azure services, you'll need to create a client using a credential from Azure Identity. This credential is used to connect to the target Azure service.
You can create a client to manage virtual machines by using the armcompute.NewVirtualMachinesClient type. This type groups related APIs, providing access to virtual machine functionality within the specified subscription.
The same pattern is used to connect with other Azure services, such as the armnetwork package, which allows you to create a virtual network client to manage virtual network resources. This client provides access to VNET resources.
To create a client, you'll need to install the relevant package, such as armcompute or armnetwork, and then use the NewClient type to create an instance of the client.
Long-Running Operations
Long-running operations can be a challenge when working with Azure resources. Functions like BeginCreate and BeginDelete are designed to handle these long-running tasks via asynchronous calls.
These functions return a poller object immediately, allowing your code to continue executing without blocking. A polling interval is required to check the status of the operation, which should be short according to the documentation for the specific Azure resource.
The PollUntilDone function is used to check the status of the operation at regular intervals. The recommended polling interval varies depending on the Azure resource being used. For example, the LRO section of the Go Azure SDK Design Guidelines page provides a more advanced example and guidelines for long-running operations.
A good rule of thumb is to use a polling interval that is short enough to avoid overwhelming the system, but long enough to avoid unnecessary resource usage.
Sources
- https://learn.microsoft.com/en-us/azure/cosmos-db/nosql/quickstart-go
- https://www.huuhka.net/using-azure-ai-speech-sdk-go/
- https://pkg.go.dev/github.com/Azure/azure-sdk-for-go
- https://learn.microsoft.com/en-us/azure/developer/go/management-libraries
- https://azure.github.io/azure-sdk/releases/latest/go.html
Featured Images: pexels.com