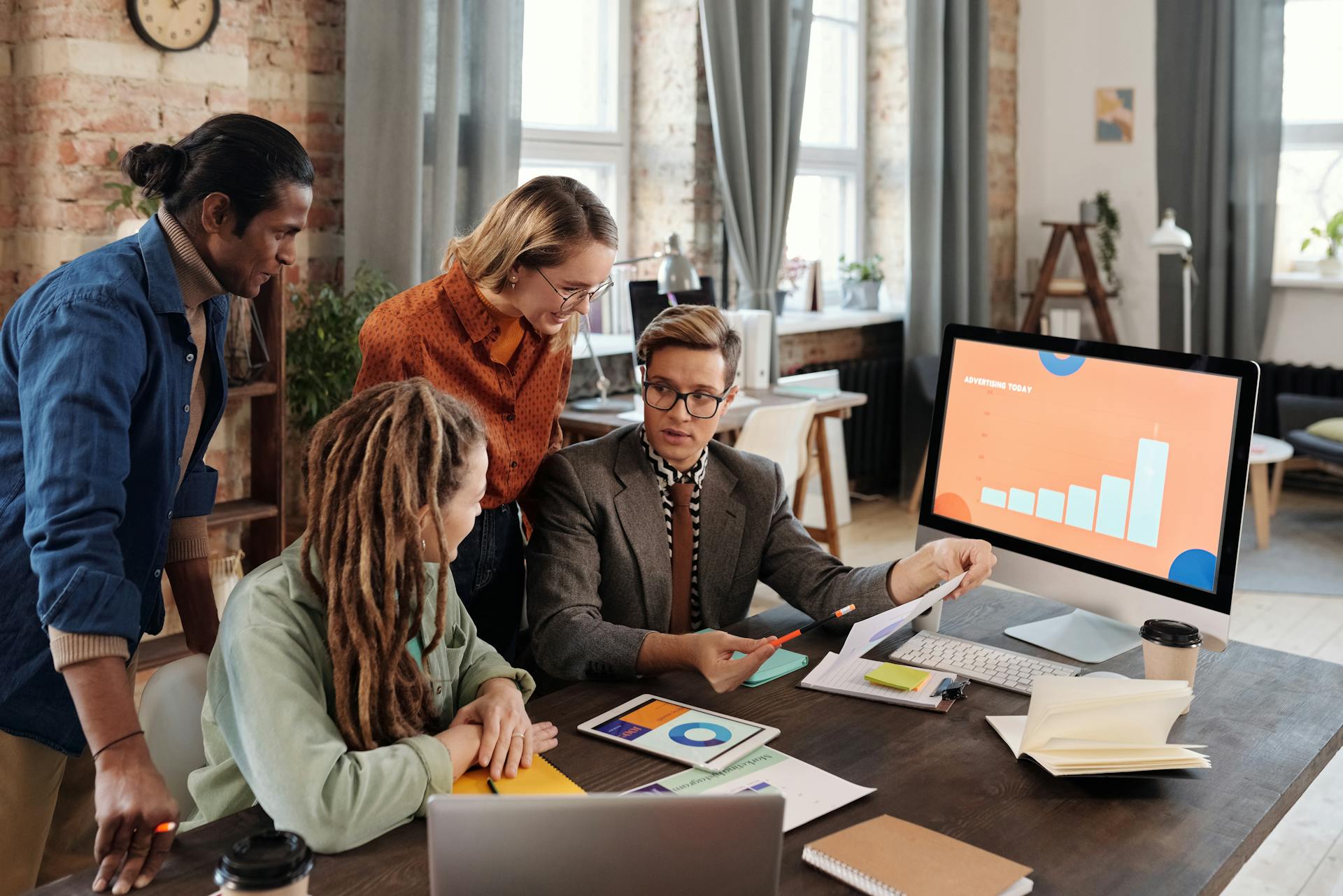
Azure Graph API is a powerful tool for developers, allowing them to access and manage data across multiple Azure services.
It's built on top of Microsoft's Graph API, which provides a unified interface for accessing data from various services, including Azure Active Directory, Intune, and Microsoft 365.
Azure Graph API supports a wide range of query languages, including Azure Resource Graph, which enables developers to query and analyze data from Azure resources.
To get started with Azure Graph API, you'll need to register an application in Azure AD and obtain a client ID and secret key.
You might like: Azure Graph Api
Azure Graph API Basics
To access services within O365, you need to request permissions from four APIs. There are four APIs that must be requested for permissions.
The Microsoft Graph API is one of the four APIs that needs to be requested for permissions. Azure Active Directory Graph is a supported legacy API that will not be required in the future.
Here are the four APIs that require permissions:
- Microsoft Graph
- SharePoint
- Azure Active Directory Graph (supported legacy API)
OData Namespace
The OData namespace is where the Microsoft Graph API defines its resources, methods, and enumerations. This is done in the microsoft.graph namespace, found in the Microsoft Graph metadata.
Most API sets are defined in the microsoft.graph namespace, but a few are defined in their sub-namespaces, such as the call records API.
Unless explicitly specified, assume types, methods, and enumerations are part of the microsoft.graph namespace.
Intriguing read: Azure Active Directory Graph Api
Http Methods
The HTTP methods used in the Azure Graph API are crucial for determining what action you're performing on a resource.
The API supports several methods, including GET, POST, PATCH, PUT, and DELETE.
Each method has a specific purpose, such as reading data with GET or creating a new resource with POST.
The GET method is used to read data from a resource, while POST is used for creating a new resource or performing an action.
The PATCH method allows you to update a resource with new values or upsert a resource (create if it doesn't exist, update otherwise).
Take a look at this: Azure Resource Graph
The PUT method replaces a resource with a new one, and the DELETE method removes a resource.
Here are the HTTP methods used in the Azure Graph API, along with a brief description of each:
The request body size limit is 4 MB for POST, PATCH, and PUT methods, but some requests may have a lower limit, such as attaching a file to a user event, which has a 3 MB limit.
The API returns a status code of 413 and an error message of "Request entity too large" or "Payload too large" if the request exceeds the size limit.
Authentication and Permissions
To authenticate with the Microsoft identity platform, you need to understand the access scenarios. There are two main methods: delegated access and app-only access.
Delegated access is when an app acts on behalf of a signed-in user. This requires delegated permissions, also known as scopes, which represent the operations an app can perform on behalf of a user.
Additional reading: Azure User Graph Domain
To request delegated permissions, you need to expand the "Delegated Permissions" category and check the box for the required permissions, such as the ability to read user profiles or send emails.
The Microsoft Graph exposes granular permissions that control the access apps have to Microsoft Graph resources, like users, groups, and mail. These permissions are categorized into two types: delegated permissions and application permissions.
Delegated permissions allow the application to act on behalf of the signed-in user, while application permissions allow the app to access data on its own, without a signed-in user.
Here are the two types of permissions:
- Delegated permissions: allow the application to act on behalf of the signed-in user.
- Application permissions: allow the app to access data on its own, without a signed-in user.
To add permissions to your app, click on the "API permissions" and then click on "Add a Permissions." This will allow you to request permissions from APIs like Microsoft Graph, SharePoint, and Azure Active Directory Graph.
The qualified user to be able to perform these functions needs to be an Azure Active Directory Global Administrator. This is because they need to be able to update permissions and add APIs to the app.
Requesting the least privileged permissions that your app needs is a best practice to ensure security and user consent. This means only requesting the permissions that are necessary for your app to function correctly, rather than requesting permissions with more than the necessary privileges.
Curious to learn more? Check out: Azure Web App Permissions
App Configuration
To configure the Graph App for version 5.0 and above, you'll need to use a thumbprint in the "Certificates & secrets" section.
A purchased certificate or self-signing certificate can be used for this purpose. The certificate must be of length 2048, and you'll also need a text version of the certificate and its password for the AppManager tenant configuration.
If you're using a purchased certificate, don't forget to have the password ready for configuration.
Expand your knowledge: Azure Ssl Cert
Headers
Headers are case-insensitive as defined in rfc 9110 unless explicitly specified otherwise.
Microsoft Graph supports both HTTP standard headers and custom headers.
Specific APIs may require additional headers to be included in the request.
Microsoft Graph will always return mandatory headers, such as the request-id header in the response.
The Retry-After header is included when your app hits throttling limits.
The Location header is included for long-running operations.
If this caught your attention, see: Azure Cloud Applications
Configuring the App for Version 5.0+
Configuring the App for Version 5.0+ requires some extra steps.
For versions 5.0 and above, you'll need to configure the Graph App's "Certificates & secrets" to use a thumbprint.
A purchased certificate or self-signing certificate can be used, but if you opt for a purchased certificate, it must be of length 2048.
You'll also need a text version of the certificate and the password for the AppManager tenant configuration.
Here's a summary of the requirements:
Advanced Topics
Azure Graph allows for the querying of multiple data sources with a single query, making it a powerful tool for data analysis.
This is achieved through the use of the $select and $expand operators, which enable users to specify the properties and relationships they want to retrieve.
Azure Graph supports a wide range of data types, including strings, integers, and dates, making it a versatile platform for data modeling.
Azure Graph's query language is based on OData, which provides a standardized way of querying data across different sources.
The $filter operator is used to filter data based on specific conditions, such as equality, inequality, and range comparisons.
Azure Graph's support for $select and $expand operators allows users to retrieve only the data they need, reducing the amount of data transferred and improving query performance.
Azure Graph's query language also supports functions such as $count, $sum, and $avg, which enable users to perform calculations on the data.
Permissions and Tokens
To create an Azure Graph App, you need to be an Azure Active Directory Global Administrator. This is because the qualified user needs to be able to perform the functions described in the document.
There are four APIs you must request permissions from: Microsoft Graph, SharePoint, Azure Active Directory Graph, and another legacy API that will not be required in the future.
Microsoft Graph exposes two types of permissions: delegated permissions, which allow the application to act on behalf of the signed-in user, and application permissions, which allow the app to access data on its own without a signed-in user.
Consider reading: Azure Active Directory Tutorial
Request the least privileged permissions that your app needs to access data and function correctly, as requesting more than necessary is poor security practice.
Delegated access requires delegated permissions, also referred to as scopes, which represent the operations that an app can perform on behalf of a user. Both the client and the user must be authorized to make the request.
Here are the types of permissions for Microsoft Graph:
- Delegated permissions: Also called scopes, allow the application to act on behalf of the signed-in user.
- Application permissions: Also called app roles, allow the app to access data on its own, without a signed-in user.
Access tokens, which are short-lived but with variable default lifetimes, contain claims about the application and in delegated access scenarios, the user. Web APIs use these claims to validate the caller and ensure they have the proper privileges.
To call Microsoft Graph, the app makes an authorization request by attaching the access token as a Bearer token to the Authorization header in an HTTP request.
Expand your knowledge: Sas Tokens Azure
Accessing Data
Accessing data in Azure Graph can be done in a few ways. You can use Microsoft Graph connectors to bring external data sources into Microsoft Graph, such as an organization's human resources database or product catalog.
Microsoft Graph Data Connect is another option, which allows you to access data on Microsoft Graph at scale, while giving administrators granular consent and control over their data. This can be useful for building intelligent apps that analyze data, such as detecting fraud or automating knowledge base creation.
You can access data using either the Microsoft Graph API or Microsoft Graph Data Connect. The choice between the two depends on your specific needs, as outlined in the following table:
Query Parameters
Query parameters are a powerful way to customize your data responses, and they can be used with OData system query options or other strings accepted by a method.
You can use optional OData system query options to include more or fewer properties than the default response, filter the response for items that match a custom query, or provide additional parameters for a method.
Adding a filter parameter, for instance, can restrict the messages returned to only those with a specific property value, such as [email protected].
Some methods require parameter values specified as part of the query URL, like getting a collection of events that occurred during a time period in a user's calendar.
To query a calendarView relationship of a user, you need to specify the period startDateTime and endDateTime values as query parameters.
You can customize your responses and get the data you need by using query parameters effectively.
Broaden your view: Risky User Azure
Fetch External Data
Microsoft Graph connectors can bring data from external content sources into Microsoft Graph.
These connectors create connections to external data sources and index the data, storing it as external custom items and files.
External data can come from an organization's human resources database or product catalog, hosted on-premises or in the public or private clouds.
Once indexed, this external data can show up in Microsoft Search and for apps that use the Microsoft Search API.
Microsoft Graph connectors make it possible to integrate external data with Microsoft Graph, expanding the scope of what's accessible within the platform.
Access Data at Scale
Accessing data at scale is a game-changer for organizations looking to unlock the full potential of their Microsoft 365 data estate. Microsoft Graph Data Connect makes it possible to access data on Microsoft Graph at scale, while giving administrators granular consent and full control over their data.
With Data Connect, you can use Azure tools to build intelligent apps that analyze meeting requests to provide insights into conference room utilization, detect fraud with productivity and communication data, automate knowledge base creation, and even find the closest expert on a topic to you in your organization.
Microsoft Graph Data Connect is particularly useful when you need to access data on many users or groups, rather than just a single user or the entire tenant. This is where Data Connect's unique set of tools shines, allowing you to stream data to Azure Data Factory on a recurrent schedule.
Here's a comparison of Microsoft Graph API and Data Connect to help you decide which one is right for you:
Pricing for Microsoft Graph Data Connect is simple and straightforward, with a flat, metered rate for every thousand objects extracted through Data Connect in your Azure Data Factory instance.
Recommended read: Azure Data Studio Connect to Azure Sql
Frequently Asked Questions
What is an Azure Graph database?
Azure Cosmos DB for Apache Gremlin is a powerful graph database service that stores massive graphs with billions of connections. It enables fast querying and easy evolution of complex graph structures.
What is Microsoft Graph in Azure AD?
Microsoft Graph is a RESTful API that provides access to Microsoft Cloud service resources, enabling you to build applications that integrate with Azure AD services. It allows you to make requests to the Microsoft Graph API after registering your app and obtaining authentication tokens.
Is the Azure graph API free?
Microsoft Graph APIs, including Azure Graph API, are free with user subscription licenses, but metered usage incurs additional costs
What is an Azure resource graph?
Azure Resource Graph is a service that helps you explore and manage your Azure resources efficiently. It extends Azure Resource Management with fast and effective resource discovery capabilities.
Featured Images: pexels.com