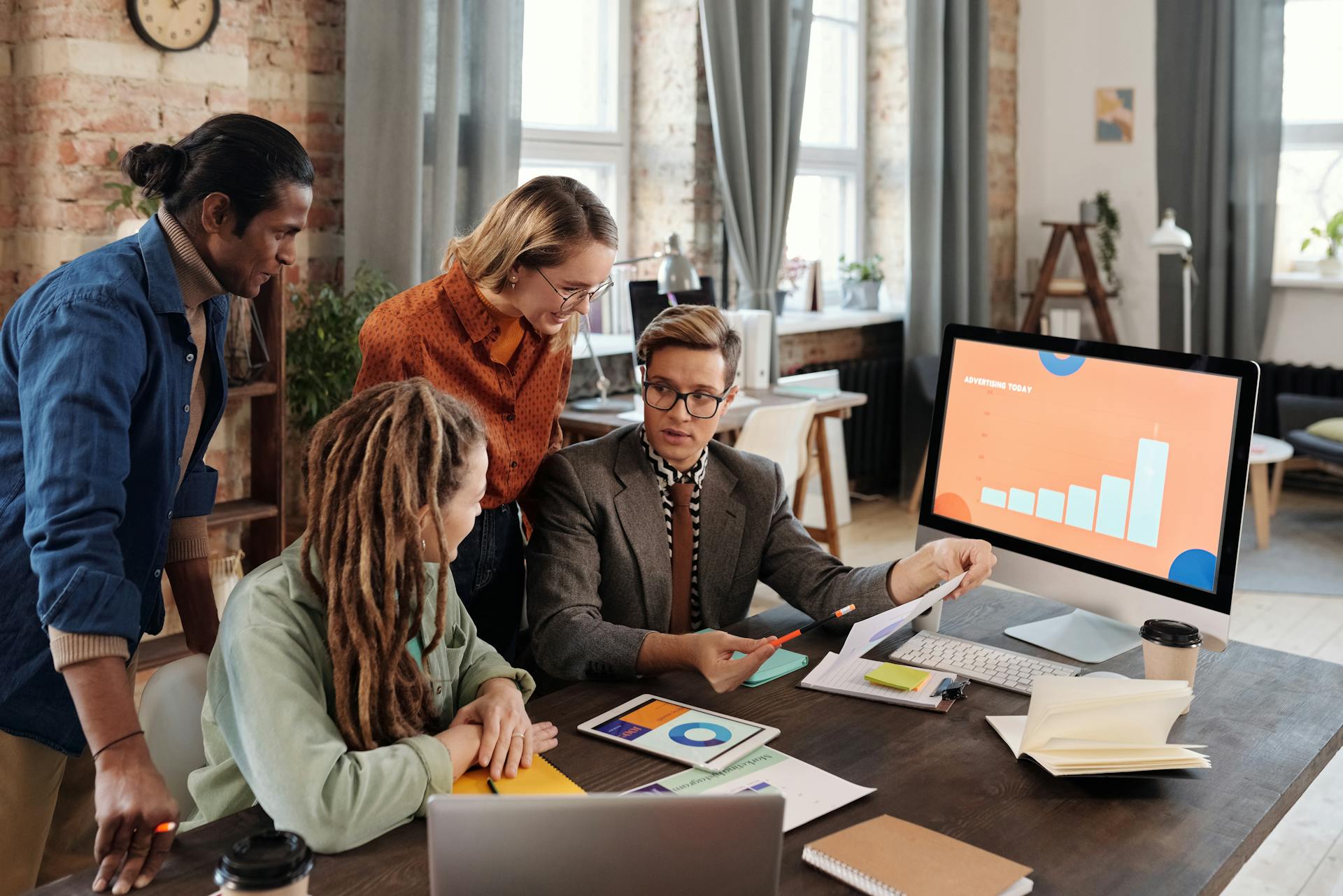
The Azure Graph API is a powerful tool that allows you to query and manage data across multiple Azure services. It's a unified interface that provides a single point of access to all your Azure resources.
The Azure Graph API is built on top of the Azure Active Directory (Azure AD) and provides a robust way to manage user identities, groups, and permissions. This is particularly useful for organizations that need to integrate their Azure services with their existing identity and access management systems.
To get started with the Azure Graph API, you'll need to create an Azure AD tenant and register an application. This will give you a client ID and client secret that you can use to authenticate with the API.
For more insights, see: Azure Active Directory Graph Api
Getting Started
You can create, read, update, and delete users with the Azure AD Graph API. This allows you to manage user accounts and their relationships with other directory entities.
To get started, you'll need to specify the resource path, which depends on whether you're targeting all users in your tenant, an individual user, or a navigation property of a specific user.
You can use the resource path to read all users or a filtered list of users, or to create one or more new users in your tenant. This is done by specifying the user_id as the object ID (GUID) or the user principal name (UPN) of the target user.
You can get the declared properties of a user by using the resource path with the user_id. This is useful for reading user information.
To modify the declared properties of a user, you'll need to use the same resource path with the user_id, but this time in the request body. This allows you to update user information.
You can delete a user by using the resource path with the user_id. This removes the user from your tenant.
To get started with the Azure AD Graph API, you'll need to understand how to specify the resource path and use it to interact with user data.
A fresh viewpoint: Azure Resource
Authentication and Authorization
Authentication and Authorization is a crucial part of working with the Azure Graph API. Every request to the Graph API must have a bearer token issued by Azure Active Directory attached, which carries information about your app, the signed-in user, authentication, and the operations on the directory that your app is authorized to perform.
The Graph API performs authorization based on OAuth 2.0 permission scopes present in the token. You can learn more about OAuth 2.0 in Azure AD, including supported flows and access tokens in OAuth 2.0 in Azure AD.
To authenticate with Azure AD and call the Graph API, you must add your app to your tenant and configure it to require permissions (OAuth 2.0 permission scopes) for Windows Azure Active Directory. You can do this by integrating your application with Azure Active Directory, as described in Integrating Applications with Azure Active Directory.
See what others are reading: Azure Auth Json Website Azure Ad Authentication
Azure AD Quickstart
To get started with the Azure AD Graph API, you must add your app to your tenant and configure it to require permissions (OAuth 2.0 permission scopes) for Windows Azure Active Directory.
The Azure Active Directory (AD) Graph API provides programmatic access to Azure AD through OData REST API endpoints. It allows applications to perform CRUD operations on directory data and objects.
To authenticate with Azure AD, you need to attach a bearer token issued by Azure Active Directory to every request to the Graph API. This token carries information about your app, the signed-in user, and the operations on the directory that your app is authorized to perform.
You can use the Graph API to create a new user, view or update user's properties, change user's password, check group membership for role-based access, disable or delete the user.
The Graph API performs authorization based on OAuth 2.0 permission scopes present in the token. For more information about the permission scopes that the Graph API exposes, see Graph API Permission Scopes.
Here are some popular API requests you can make with the Graph API:
- Check out some of these common scenarios for working with the Microsoft Graph API.
- The links take you to the Graph Explorer.
Authentication and Authorization
To authenticate with Azure AD and call the Graph API, you must add your app to your tenant and configure it to require permissions (OAuth 2.0 permission scopes) for Windows Azure Active Directory.
The Graph API performs authorization based on OAuth 2.0 permission scopes present in the token attached to the request. You can learn more about OAuth 2.0 in Azure AD, including supported flows and access tokens.
Every request to the Graph API must have a bearer token issued by Azure Active Directory attached. The token carries information about your app, the signed-in user, and the operations on the directory that your app is authorized to perform.
You use the me alias to target operations against the signed-in user. This alias is only available when using OAuth Authorization Code Grant type (3-legged) authentication. The me alias replaces the object ID or tenant domain in the URL and is not case sensitive.
Here are the common HTTP headers used in requests with the Graph API:
Request and Response
The Azure Graph API's request and response process is straightforward. A successful request returns a 200 OK response code and the user object in the response body, unless you specify specific properties with the $select parameter. This method returns a 202 Accepted response code when the request has been processed successfully, but the server requires more time to complete related background operations.
By default, the API returns a limited set of properties, including businessPhones, displayName, id, jobTitle, mail, mobilePhone, officeLocation, preferredLanguage, surname, and userPrincipalName. You can specify additional properties to return by using the $select parameter.
The API supports both HTTP standard headers and custom headers, which can be used to include additional information with your request. Some APIs require specific headers to be included, while others may include mandatory headers, such as the request-id header, in the response.
Http Request
The HTTP request is a crucial part of making a request to the Microsoft Graph API. The request URL syntax is important to get right, and one common mistake is prefixing the userPrincipalName with a $ character, which can result in a 400 Bad Request error code.
To avoid this, you should remove the slash (/) after /users and enclose the userPrincipalName in parentheses and single quotes, as follows: /users('[email protected]'). For example, /users('[email protected]').
If you're querying a B2B user using the userPrincipalName, you'll need to encode the hash (#) character by replacing it with %23. For instance, /users/AdeleVance_adatum.com%23EXT%[email protected].
Here are some common HTTP methods used in the Microsoft Graph API:
If your request exceeds the size limit, it will fail with a 413 status code and the error message "Request entity too large" or "Payload too large."
Headers
Headers are an essential part of both requests and responses in the Graph API. They provide information about the request or response, such as authentication, content type, and server details.
The Graph API supports both HTTP standard headers and custom headers. You can include additional headers in the request, but Microsoft Graph will always return mandatory headers like the request-id header.
Headers are case-insensitive, as defined in rfc 9110, unless explicitly specified otherwise. This means you can use either uppercase or lowercase letters when including headers in your requests.
Microsoft Graph will include the Retry-After header when your app hits throttling limits, and the Location header for long-running operations. These headers provide valuable information about the status of your requests.
Here are some common HTTP headers returned in responses by the Graph API:
API Endpoints
The Azure Graph API has a specific way of addressing endpoints, which is crucial for performing operations. The service root for all Graph API requests is https://graph.windows.net.
To target a specific tenant, you need to include the tenant identifier {tenant_id} in the URL. For instance, if you're targeting the contoso.com tenant, the URL would be https://graph.windows.net/contoso.com.
Resource paths {resource_path} are also essential, as they point to the resource you want to access. For example, to access a user or a group, you would use a path like /users or /groups.
You might like: Azure Resource Graph
Here are the components that comprise a complete URL:
- Service Root: https://graph.windows.net
- Tenant Identifier {tenant_id}: The identifier for the tenant that the request targets.
- Resource path {resource_path}: The path to the resource that the request targets.
- Graph API Version {api_version}: The version of the Graph API targeted by the request, expressed as a query parameter and required.
Endpoint Addressing
Endpoint Addressing is a crucial aspect of working with APIs, and the Graph API is no exception. The service root for all Graph API requests is https://graph.windows.net.
This URL is the foundation of every Graph API request, and it's essential to understand its components. The service root is followed by the tenant identifier, which is a unique identifier for the tenant that the request targets. For example, in the URL https://graph.windows.net/contoso.com/groups?api-version=1.5, contoso.com is the tenant identifier.
The resource path is the next component of the URL, and it identifies the resource that the request targets. In the example URL, groups is the resource path, indicating that the request is targeting the groups resource. The resource path can be a top-level resource, such as users or groups, or a specific entity, like a user or a group.
The Graph API version is also a critical component of the URL, and it's expressed as a query parameter. In the example URL, api-version=1.5 is the query parameter that specifies the version of the Graph API being targeted. This is required for all Graph API requests.
If this caught your attention, see: Windows Azure Service Management Api
Here's a breakdown of the components of a Graph API URL:
- Service Root: https://graph.windows.net
- Tenant Identifier: a unique identifier for the tenant, such as a verified domain name or a tenant object ID
- Resource Path: the path to the resource, such as users, groups, or a specific entity
- Query Parameters: additional parameters that customize the request, such as the Graph API version or OData query options
By understanding these components, you can construct a valid Graph API URL and make requests to the Graph API.
Create a
When creating a user through an API endpoint, you'll need to specify the required properties for the user. This includes a boolean value indicating whether the account is enabled, which is a crucial setting to get right.
The name to display in the address book for the user is another essential property, ensuring your users are easily identifiable in the system. You can also specify a mail alias for the user, which is useful for directing emails to the correct inbox.
If you're using a federated domain for the user's UPN property, you'll need to specify the domain name. The password profile for the user is also a required property, which includes the password and other relevant details.
Suggestion: Azure User Graph Domain
Here are the required properties for creating a user:
These properties will help you create a user with all the necessary details, ensuring a smooth onboarding process for your users.
Frequently Asked Questions
Is graph API being deprecated?
Yes, the Azure AD Graph API has been deprecated by Microsoft. Its planned retirement date has been extended to at least December 31, 2022.
Is the Azure graph API free?
The Azure graph API is partially free, as it includes some no-cost APIs with user subscription licenses, but others are metered and incur costs based on usage. To determine the costs, review the specific APIs and services you plan to use.
Is Microsoft Graph API a REST API?
Yes, Microsoft Graph API is a REST API, providing access to rich data via a single endpoint. It also offers SDKs for easier integration.
Sources
- https://learn.microsoft.com/en-us/graph/api/user-get
- https://weareminky.com/share/ms/docs/reference/rest/example/index.html
- https://github.com/Huachao/azure-content/blob/master/articles/active-directory/active-directory-graph-api-quickstart.md
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/graph/overview
Featured Images: pexels.com