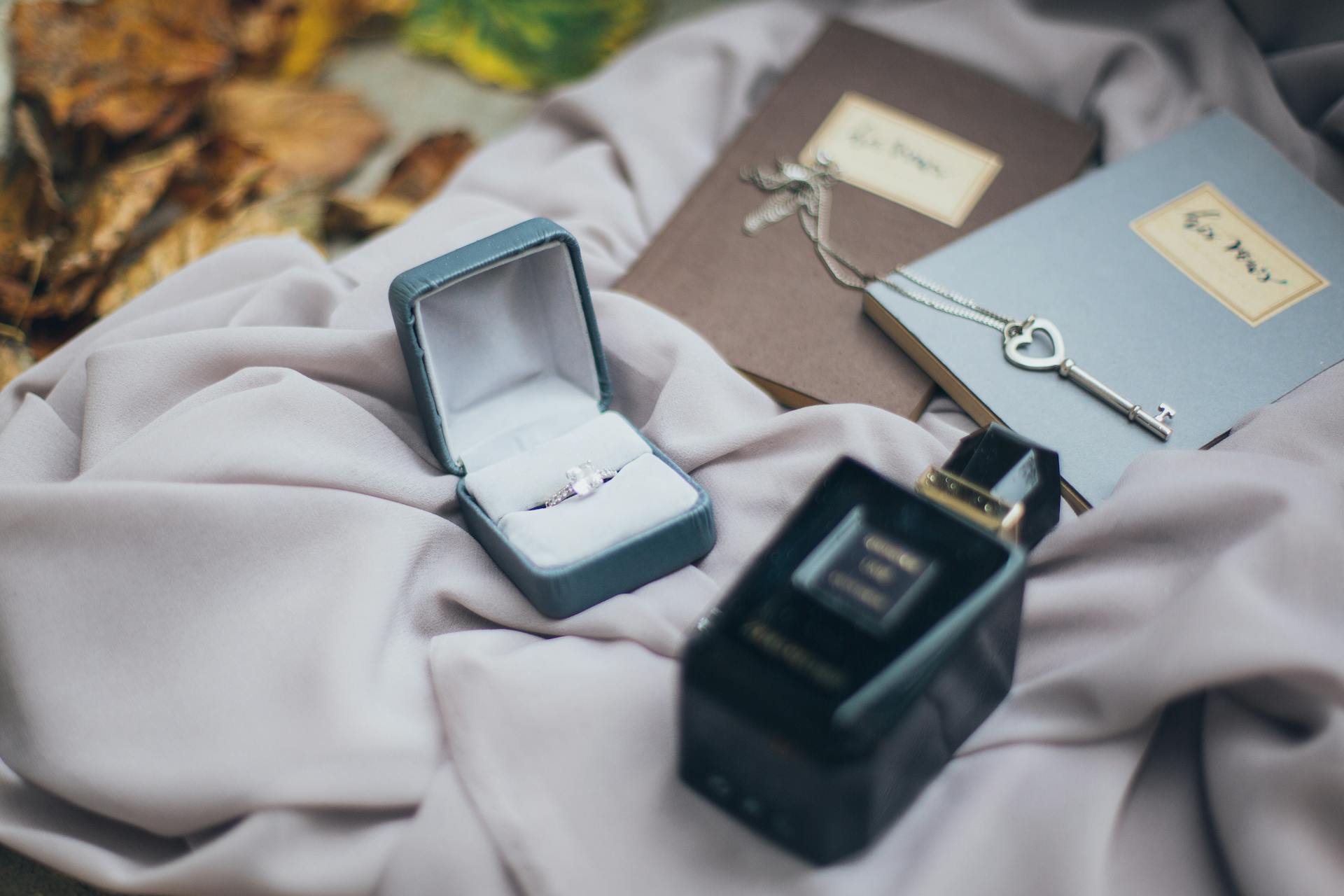
Azure Key Vault .NET is a powerful tool for securing sensitive data in your applications. It provides a secure way to store and manage secrets, such as API keys, connection strings, and certificates.
By using Azure Key Vault .NET, you can encrypt and protect your secrets from unauthorized access. This is especially important for applications that handle sensitive user data.
Azure Key Vault .NET is highly scalable and can handle large volumes of secrets with ease. This makes it an ideal solution for large-scale applications.
With Azure Key Vault .NET, you can easily integrate secrets into your .NET applications using the managed client library. This library provides a simple and secure way to access and use your secrets.
Creating and Configuring Azure Key Vault
To create an Azure Key Vault instance, navigate to the Azure Portal and follow these steps. You'll need to select "Create a resource" in the Azure Portal menu or the Home page.
Specify "Key Vault" in the search box, and when the results are listed, choose "Key Vault" and click "Create". In the "Create key vault" screen, provide the name, subscription, resource group name, and location. Leave the other options to their default values.
You can also create a new Azure Key Vault instance by selecting the Create a resource option in the upper-left corner of the Azure portal and following the same steps. To configure your Azure Key Vault, you'll need to provide the necessary information in the "Create key vault" screen.
Here's a summary of the required information:
Once you've provided the necessary information, click "Review + Create" and review the entered configuration. If all is fine, click "Create" to create your Azure Key Vault instance.
Managing Secrets
To create a secret in Azure Key Vault, select "Secrets" from the "Settings" section of the "Key Vault configuration" page and click "Generate/Import" to add a secret.
You can name and value the secret, and optionally specify the "Content type" and set the "activation and expiration date" options.
When accessing secrets from Azure Key Vault, you can use the SecretClient class to retrieve secrets stored inside the Azure Key Vault.
To add a secret to Key Vault, select Secrets from the Key Vault properties pages, select Generate/Import, and enter the secret values.
Expired secrets are included by default in the configuration provider, but you can exclude them by updating the expired secret or providing the configuration using a custom configuration provider.
Here's a list of the benefits of using Azure Key Vault for secret management:
- Enhanced Security: Safely store and tightly control access to sensitive information like passwords, API keys, certificates, and encryption keys.
- Simplified Management: Seamless integration with Azure services and applications, ensuring easy access and updates to secrets.
- Compliance and Auditing: Meet regulatory requirements with built-in auditing, logging, and access control features.
Configuring .NET Core
Configuring .NET Core involves several key steps. To get started, you'll need to install the required NuGet packages, including Microsoft.Extensions.Azure and Azure.Security.KeyVault.Secrets.
You can install these packages using the NuGet Package Manager in Visual Studio or by running commands in the Package Manager Console. The commands are: Install-Package Microsoft.Extensions.Azure and Install-Package Azure.Security.KeyVault.Secrets.
To work with Azure Key Vault, you'll need to add a reference to the required NuGet packages in your .NET Core project. This can be done by running the command dotnet add package Azure.Identity.
Once you've installed the required packages, you can update your code to use a Key Vault reference. This involves adding a reference to the Azure.Identity package, using the App Configuration by calling the AddAzureAppConfiguration method, and including the ConfigureKeyVault option.
Here's a summary of the required packages and steps:
To configure Key Vault in your .NET Core project, you'll need to provide the Key Vault name, Application ID, and certificate thumbprint in the app's appsettings.json file. This involves creating a PKCS#12 archive (.pfx) certificate, installing it into the current user's personal certificate store, and exporting the certificate as a DER-encoded certificate (.cer).
Once you've configured Key Vault in your project, you can access the values of Key Vault references in the same way you access the values of regular App Configuration keys. This involves using the IConfiguration class to retrieve the values of the Key Vault references.
Security and Access
Granting your app access to Key Vault is a crucial step in securing your secrets. You can do this using a Key Vault access policy or Azure role-based access control.
To access Key Vault, you'll need to grant your app the necessary permissions. You can do this by setting the AZURE_TENANT_ID, AZURE_CLIENT_ID, and AZURE_CLIENT_SECRET environment variables, and using the DefaultAzureCredential class to authenticate with your key vault.
Alternatively, you can use a managed identity for your Azure service, such as Azure App Service, Azure Kubernetes Service, or Azure Container Instance. This allows your app to authenticate with Key Vault using Microsoft Entra ID authentication without storing credentials in the app's code or configuration.
To authorize your Web App to access your Key Vault, you'll need to select "Access policies" from the "Key Vault" screen, click "Add Access Policy", and provide the "Get" and "List" permissions. You'll also need to specify the value for the "Object ID" of your Azure Web App and click "Add" and "Save" to persist the changes.
Here are the benefits of using Azure Key Vault:
- Enhanced Security: Safely store and tightly control access to sensitive information like passwords, API keys, certificates, and encryption keys.
- Simplified Management: Seamless integration with Azure services and applications, ensuring easy access and updates to secrets.
- Compliance and Auditing: Meet regulatory requirements with built-in auditing, logging, and access control features.
Using a managed identity for your Azure service can simplify the process of granting access to Key Vault. You can create a managed identity for your Azure App Service app, and then use the app's Object ID to provide the app with list and get permissions to access the vault.
If you're using a user-assigned managed identity, you can configure the managed identity's Client ID by setting the AZURE_CLIENT_ID environment variable or using the DefaultAzureCredentialOptions.ManagedIdentityClientId property.
Best Practices and Troubleshooting
To ensure Azure Key Vault is used securely and efficiently, it's essential to follow best practices and troubleshoot common issues. You can prevent configuration loading errors by verifying that the app or certificate is correctly configured in Microsoft Entra ID.
To troubleshoot, check if the vault exists in Azure Key Vault, and if the app is authorized to access the vault. Ensure the access policy includes Get and List permissions, and the configuration data is correctly named and enabled in the vault.
Here are some common issues that can prevent configuration from loading:
- The app or certificate isn't configured correctly in Microsoft Entra ID.
- The vault doesn't exist in Azure Key Vault.
- The app isn't authorized to access the vault.
- The access policy doesn't include Get and List permissions.
- In the vault, the configuration data (name-value pair) is incorrectly named, missing, or disabled.
- The app has the wrong Key Vault name (KeyVaultName), Microsoft Entra ID Application ID (AzureADApplicationId), or Microsoft Entra ID certificate thumbprint (AzureADCertThumbprint), or Microsoft Entra ID Directory ID (AzureADDirectoryId).
- When adding the Key Vault access policy for the app, the policy was created, but the Save button wasn't selected in the Access policies UI.
By following these best practices and troubleshooting tips, you can ensure Azure Key Vault is used securely and efficiently in your .NET applications.
Expired Secrets
Expired secrets are included by default in the configuration provider. This means they'll be included in your app configuration unless you take action to exclude them.
To avoid including expired secrets, you can update the expired secret or provide the configuration using a custom configuration provider. I've found that custom providers can be a game-changer when dealing with sensitive data.
Disabled secrets cannot be retrieved from Key Vault and are never included in your app configuration. This is a security feature that prevents sensitive data from being accessed or exposed.
By understanding how expired and disabled secrets work, you can take steps to ensure your app configuration is secure and up-to-date.
Best Practices
To ensure your Azure Key Vault is secure, follow these best practices:
Using managed identities to authenticate applications without exposing credentials is a must. This approach helps prevent unauthorized access to sensitive information.
Implementing Azure RBAC for access control is crucial. It allows you to grant and revoke access to specific resources, reducing the risk of data breaches.
Regularly rotating secrets with versioning is essential for security. This practice ensures that even if a secret is compromised, it can be easily updated to prevent further damage.
To track access and detect anomalies, enable auditing and monitoring. This feature provides valuable insights into who is accessing your Key Vault and when.
Here are the best practices for using Azure Key Vault:
Securely configuring Key Vault is also vital. Avoid hardcoded URIs and use environment variables or Azure App Configuration instead. This approach helps prevent accidental exposure of sensitive information.
Troubleshoot
If the app fails to load configuration using the provider, an error message is written to the ASP.NET Core Logging infrastructure.
The app or certificate isn't configured correctly in Microsoft Entra ID. Make sure you've set everything up properly.
The vault doesn't exist in Azure Key Vault. Double-check that you've created the vault and it's available.
The app isn't authorized to access the vault. Verify that you've granted the necessary permissions.
The access policy doesn't include Get and List permissions. Ensure that the policy has the correct permissions.
In the vault, the configuration data (name-value pair) is incorrectly named, missing, or disabled. Review your configuration data carefully.
The app has the wrong Key Vault name (KeyVaultName), Microsoft Entra ID Application ID (AzureADApplicationId), or Microsoft Entra ID certificate thumbprint (AzureADCertThumbprint), or Microsoft Entra ID Directory ID (AzureADDirectoryId). Check your app's configuration settings.
The policy was created, but the Save button wasn't selected in the Access policies UI. Don't forget to save your changes.
Here are the common issues that can prevent configuration from loading:
- The app or certificate isn't configured correctly in Microsoft Entra ID.
- The vault doesn't exist in Azure Key Vault.
- The app isn't authorized to access the vault.
- The access policy doesn't include Get and List permissions.
- In the vault, the configuration data (name-value pair) is incorrectly named, missing, or disabled.
- The app has the wrong Key Vault name (KeyVaultName), Microsoft Entra ID Application ID (AzureADApplicationId), or Microsoft Entra ID certificate thumbprint (AzureADCertThumbprint), or Microsoft Entra ID Directory ID (AzureADDirectoryId).
- The policy was created, but the Save button wasn't selected in the Access policies UI.
Azure Key Vault Features and Options
Azure Key Vault provides several key features and options that make it a powerful tool for managing secrets and encryption keys. Its configuration options allow for customization to suit your needs.
You can add an AzureKeyVaultConfigurationOptions object to the AddAzureKeyVault method to control secret loading and configure reload intervals. The Manager property allows you to specify a KeyVaultSecretManager instance for loading secrets.
The reload interval can be set to a TimeSpan value to control how often the vault is polled for changes. If not specified, the default value is null, and configuration is not reloaded.
Azure Key Vault offers several key features, including vaults for storing software and HSM-backed keys, secrets, and certificates, as well as managed HSM pools for HSM-backed keys.
Here are the main features of Azure Key Vault:
Azure Key Vault also offers two types of service tiers: Standard and Premium. The Standard tier encrypts with a software key, while the Premium tier includes hardware security module (HSM)-protected keys.
Frequently Asked Questions
What is the Azure key vault key client library for net?
The Azure Key Vault key client library for .NET enables secure management of RSA and Elliptic Curve keys, with support for hardware security modules (HSMs). It provides a range of key management operations for creating, updating, and deleting keys.
What is vault Azure net?
Azure Key Vault is a cloud service for securely storing and accessing sensitive information, such as API keys and passwords. A "vault" is a type of container within Key Vault that stores and manages these sensitive assets securely.
Sources
- https://www.loginradius.com/blog/engineering/guest-post/using-azure-key-vault-with-an-azure-web-app-in-c-sharp/
- https://learn.microsoft.com/en-us/azure/azure-app-configuration/use-key-vault-references-dotnet-core
- https://learn.microsoft.com/en-us/aspnet/core/security/key-vault-configuration
- https://pumpingco.de/blog/use-azure-keyvault-with-asp-net-core-running-in-an-aks-cluster-using-aad-pod-identity/
- https://www.ottorinobruni.com/azure-key-vault-for-csharp-developers-securing-secrets-in-net-applications/
Featured Images: pexels.com