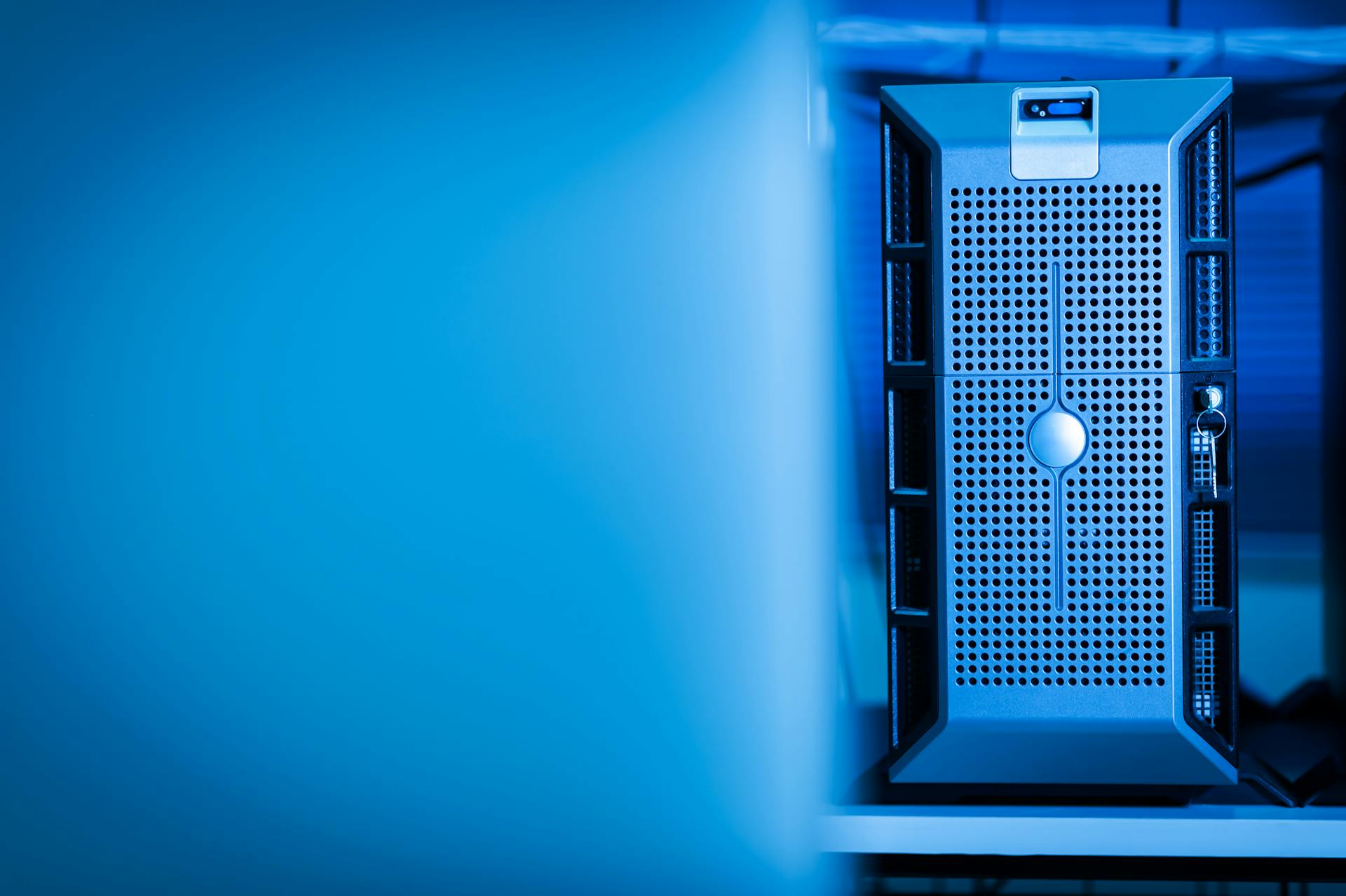
Building scalable microservices architecture on Azure requires a thoughtful approach to service design, deployment, and management. A key aspect of this is breaking down large applications into smaller, independent services that communicate with each other through APIs.
Each microservice should have its own dedicated database, which can be scaled independently of other services. This allows for more efficient use of resources and reduces the risk of a single point of failure.
To achieve scalability, Azure provides a range of services, including Azure Kubernetes Service (AKS) and Azure Service Fabric, which enable the deployment and management of containerized microservices. These services provide automated scaling, self-healing, and load balancing, making it easier to handle increased traffic and demand.
By using Azure's managed services, developers can focus on writing code and building features, rather than worrying about the underlying infrastructure. This approach enables faster time-to-market and greater agility in responding to changing business needs.
Suggestion: Azure Management
Azure Microservices Fundamentals
A microservice is a small, independent service that performs a specific task within a larger application.
Microservices are designed to be loosely coupled, meaning they don't rely on each other to function.
Each microservice has its own database and communicates with other services through APIs.
This architecture allows for greater flexibility and scalability.
With microservices, you can develop, test, and deploy individual components independently.
This makes it easier to update or replace a single service without affecting the entire application.
Azure provides a range of tools and services to support microservices development, including Azure Kubernetes Service (AKS) and Azure Service Fabric.
AKS allows you to deploy and manage containerized applications, while Service Fabric provides a runtime for microservices.
By using these tools, you can create a highly scalable and resilient microservices architecture on Azure.
See what others are reading: Pragmatic Microservices with C# and Azure
Designing and Building Microservices
Designing and building microservices on Azure involves several key considerations. Microsoft provides guidance on designing and building microservices on Azure through the Azure architecture center.
A four-step process for building a microservices architecture in Azure involves analyzing the business domain, selecting from compute options, designing APIs, and selecting a design pattern. The 12-factor app principles should be followed when building microservices applications in Azure.
To design APIs for microservices, consider using REST over HTTP for public APIs and RPC for backend APIs. Formal definitions of APIs are essential for automated documentation, auto-generated client code, and security rules. An API gateway can be used to translate backend RPC APIs to REST.
Cross-Language, Cross-Framework
Developers want to be free to choose a language or framework, depending on their skills and the needs of the service. You can write microservices in any programming language, using any framework.
For some services, you might value the performance benefits of C++ above anything else. For others, the ease of managed development that you get from C# or Java might be more important.
Azure Service Fabric is agnostic about how you build your service, and you can use any technology. It provides built-in programming APIs that make it easier to build microservices.
Here are some examples of the flexibility you have with Azure Service Fabric:
- C++ for performance
- C# or Java for ease of managed development
- Any framework you choose
Designing APIs
Designing APIs is a crucial step in building microservices. It's essential to consider the communication between microservices and define clear interfaces.
When choosing between REST and RPC, prefer REST for public APIs over HTTP, and RPC for backend APIs, which provide faster serialization and optimal payload size. This helps prevent APIs from becoming chatty.
Formal definitions of APIs are also important, enabling automated documentation, auto-generated client code, and security rules. Use the Interface Definition Language (IDL) in RPC APIs and OpenAPI for REST APIs.
API gateways can translate backend RPC APIs to REST, making it easier to communicate between microservices.
Here are some key considerations when designing APIs:
- REST vs. RPC: Use REST for public APIs and RPC for backend APIs.
- Formal definition: Use IDL for RPC APIs and OpenAPI for REST APIs.
- API gateway: Translate backend RPC APIs to REST.
- Language support: RPC API frameworks only support Java, Python, C++, and C#, while REST APIs can support any language.
By following these guidelines, you can design APIs that enable seamless communication between microservices.
Red Hat OpenShift
Red Hat OpenShift is a fully managed service that's jointly operated with Red Hat. It's a game-changer for building and managing microservices.
One of the key benefits of using Red Hat OpenShift is that it's a fully managed service, which means you don't have to worry about the underlying infrastructure. This allows you to focus on building and deploying your microservices.
Red Hat OpenShift is designed to be highly scalable and secure, making it a great choice for large-scale microservices architectures. Its joint operation with Red Hat ensures that you get the best of both worlds - the expertise of Red Hat and the scalability of a fully managed service.
Container Registry
When designing a container registry, one key consideration is the type of container images and artifacts it will store. Azure Container Registry is a great option for storing Docker and Open Container Initiative (OCI) images and artifacts.
A registry is essentially a central location for storing and managing container images, making it easier to share and reuse them across different applications and environments.
Recommended read: Azure Containerization
Architecture and Solution Design
To design a robust Azure microservices architecture, you need to start with a solid understanding of the underlying principles. This involves learning about repeatable patterns and components to develop reliable distributed systems, as outlined in "Designing Distributed Systems".
Aligning your organization around roles and responsibilities is crucial for a successful AKS solution journey. This includes defining clear roles and expectations for each team member involved in the project.
Microsoft has published a design pattern for building microservices applications in Azure, which follows the 12-factor app principles. This pattern is a four-step process that can help guide your design decisions.
The Azure architecture center is a valuable resource for guidance on designing and building microservices on Azure. You can find detailed information on best practices and design patterns to help you get started.
Using domain analysis to model microservices is a key part of the domain-driven design (DDD) framework. This approach can help you create a set of well-designed microservices that meet your business needs.
A fresh viewpoint: Contributor Role in Azure
Development and Deployment
You can independently version, deploy, and scale code and state in Azure microservices. This allows for agility and flexibility, so you can upgrade some of the microservices without having to upgrade all of them at once.
Azure's microservices offer a toolkit to create high-data-volume, low-latency applications. With the platform's advantages, engineers can build and deploy microservices with ease.
For scaling, understanding how to partition (or shard) both the code and the state is challenging. This is because the code and state often use different technologies, which is common today.
Azure provides a hybrid environment for app deployment, offering a blend of on-premises, serverless, and cloud-native architectures. This allows for flexibility in deployment options.
There are three core services used to deploy microservices applications on Azure: service fabric, containers, and serverless. These services provide different compute options for microservices.
To achieve faster and more reliable release cycles, create a good CI/CD process. This will help you to deploy microservices with ease and speed.
Microservices Principles and Best Practices
One of the key benefits of microservices is that they allow code and state to be independently versioned, deployed, and scaled.
In a microservices approach, the code and state should be able to deploy, upgrade, and scale separately, which is a challenging problem to solve due to the choice of technologies.
This separation is crucial for agility and flexibility, enabling you to upgrade some microservices without having to upgrade all of them at once.
Partitioning or sharding both the code and the state is a difficult task, especially when using different technologies for code and state, which is common today.
Fault Tolerance and Monitoring
Dealing with unexpected failures is one of the hardest problems to solve in a distributed system. Much of the code we write as developers is for handling exceptions, but it's not just about writing code to handle failures.
You need to detect the failure, which is a hard problem on its own, and then restart your microservice. For availability, a microservice needs to be resilient to failures and able to restart on another machine.
Data shouldn't be lost, and data needs to remain consistent. To achieve resiliency, a microservice needs to emit health information to make decisions about whether it can continue to move forward or roll back to a previous version.
A microservice needs to report its health and diagnostics to provide insight into its health from an operations perspective. This is often overlooked, but it's crucial for making informed decisions.
Health is about the microservice reporting its current state to take appropriate actions, while diagnostics provide more detailed information about the issue. Health events from a microservice help create self-healing services.
You need to standardize how to log health and diagnostic events that will ultimately end up in an event store for querying and viewing. This requires agreement among different teams on a single logging format.
Design Guidance and Tools
Microsoft provides detailed documentation on how to build each type of microservice in Azure. You can find guidance on designing microservices on Azure by visiting the Azure architecture center.
To design reliable distributed systems, read "Designing Distributed Systems" which teaches about repeatable patterns and components to develop systems easier and more efficiently.
For microservices on Azure, select a design pattern from the following options: Ambassador, Façade layer (anti-corruption layer), Bulkhead, Backend for frontend, Gateway aggregation, offloading or routing, Strangler, or Sidecar.
Curious to learn more? Check out: Design Azure
State Storage Approaches
In a monolithic approach, the application typically uses a single database, which can make it easy to deploy but also creates challenges in scaling individual components.
Each component can have a single table to store its state, but teams need to strictly separate state, which is a challenge. Inevitably, someone will be tempted to add a column to an existing customer table, do a join between tables, and create dependencies at the storage layer.
In the microservices approach, each service manages and stores its own state, which means each service is responsible for scaling both code and state together to meet the demands of the service.
A downside of this approach is that when you need to create views or queries of your application's data, you need to query across multiple state stores, which can be solved by a separate microservice that builds a view across a collection of microservices.
Microservices are versioned, allowing different versions of a microservice to run side by side, which is helpful for A/B testing and rolling back to an earlier version if needed.
Online Sample Chapter
If you're looking for online resources to help you design microservices, there's a wealth of information available.
For Azure users, a great place to start is the Azure architecture center, which offers guidance on designing and building microservices on the platform.
Each microservice needs a unique name, or URL, to resolve its location and make it addressable wherever it's running.
This unique name is crucial for discoverability, and it's essential to have a service registry to keep track of the service's current location.
A service registry will also notify you if a machine fails, so you know where to move the service to.
Guidance for Designing
Designing a microservices architecture requires careful consideration of several factors.
To mitigate challenges, explore design patterns that help with microservices.
Designing distributed systems can be easier and more efficient with repeatable patterns and components. Read Designing Distributed Systems to learn more.
For designing APIs for microservices, consider REST vs. RPC, formal definitions, API gateways, and languages. Here are some key points to keep in mind:
- REST vs. RPC: Prefer REST for public APIs and RPC for backend APIs that connect to each other.
- Formal definition: Use IDL for RPC APIs and OpenAPI for REST APIs.
- API gateway: Translate backend RPC APIs to REST.
- Languages: RPC API frameworks support Java, Python, C++, and C#, while REST APIs can support any language.
Remember, designing APIs for microservices is crucial for scalability and maintainability.
For selecting a design pattern, consider ambassador, façade layer, bulkhead, and other patterns that fit your needs.
Here are some design patterns to consider:
- Ambassador: Enables client connectivity tasks like routing, monitoring, and logging.
- Façade layer (anti-corruption layer): Bridges traditional monolithic applications and microservices.
- Bulkhead: Partitions critical system resources like CPU, memory, and connection pools.
- Backend for frontend: Provides separate backend services for different types of clients.
When designing your microservices architecture, consider the business domain and define microservice functionality.
To deploy a microservices application, follow these steps:
- Analyze the business domain and define microservice functionality.
- Select a compute option, such as Service Fabric, Azure Kubernetes Service, or Azure Functions.
- Design your APIs.
- Select a design pattern.
Frequently Asked Questions
What are the three types of microservices?
There are three main types of microservices: Domain Microservices, Integration Microservices, and Unit-of-work Microservices, each serving a distinct purpose in software architecture. Understanding the differences between these types is crucial for building scalable and efficient systems.
Is a rest API a microservice?
No, a REST API is not a microservice, but rather a communication mechanism used within microservices architectures
What is the difference between monolithic and microservices in Azure?
Azure microservices applications have more complex systems with many smaller, simpler services, whereas monolithic applications have a single, unified codebase. This difference affects development and testing, making microservices more challenging but also more scalable and flexible
Sources
- https://azure.microsoft.com/en-us/solutions/microservice-applications
- https://learn.microsoft.com/en-us/azure/service-fabric/service-fabric-overview-microservices
- https://www.infopulse.com/blog/microservices-in-microsoft-azure
- https://cloudnativenow.com/features/four-steps-to-running-microservices-in-azure/
- https://www.microsoftpressstore.com/store/developing-microservices-architecture-on-microsoft-9780136819387
Featured Images: pexels.com