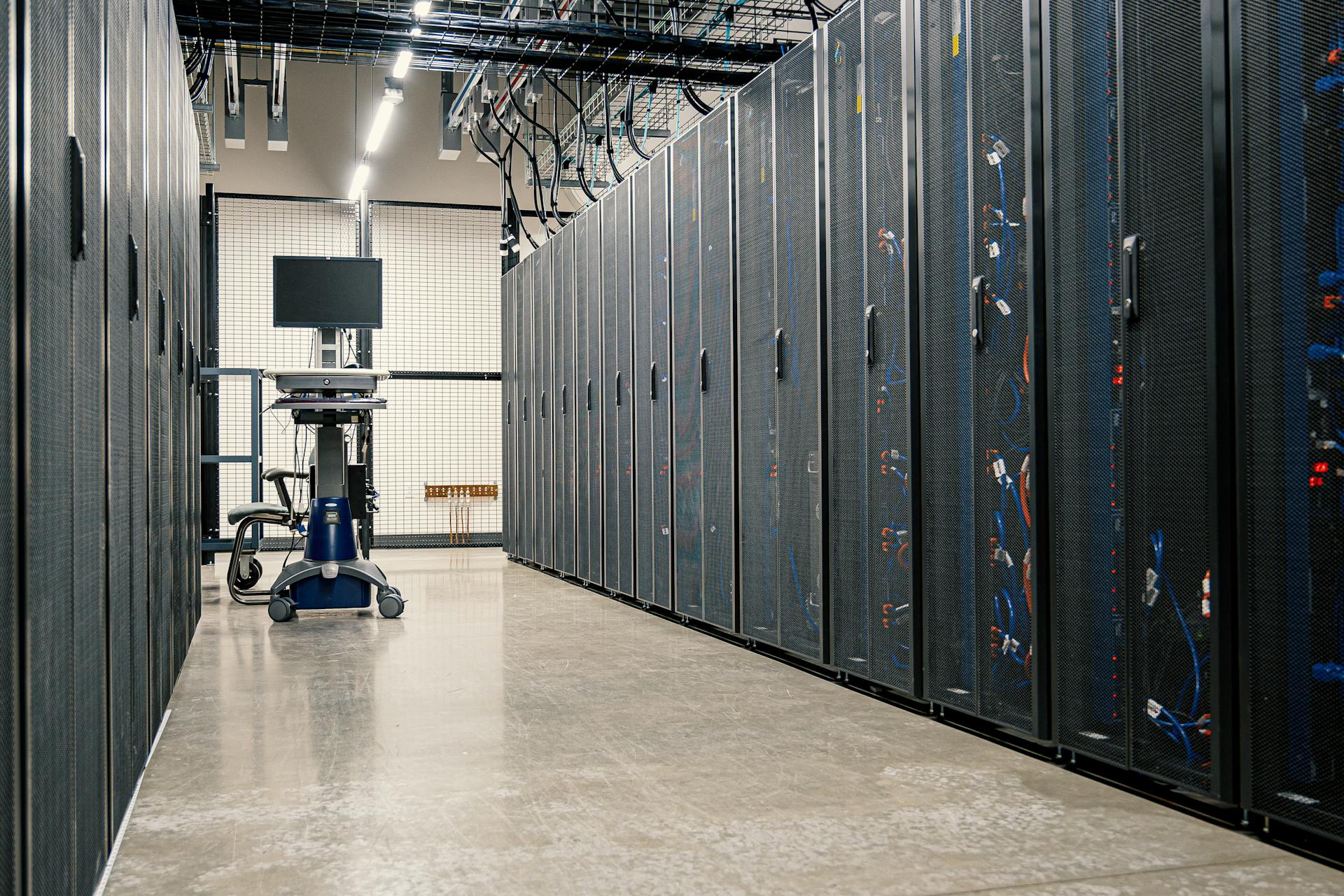
Building pragmatic microservices with C# and Azure requires a clear understanding of the benefits of microservices architecture.
Breaking down a monolithic application into smaller, independent services allows for greater scalability, flexibility, and fault tolerance.
C# is an ideal choice for building microservices due to its strong typing, object-oriented design, and extensive libraries.
Azure provides a robust platform for deploying and managing microservices, with features like load balancing, autoscaling, and container orchestration.
By leveraging C# and Azure, developers can build robust, scalable, and maintainable microservices that meet the needs of modern applications.
A well-designed microservices architecture can lead to significant improvements in system resilience, deployment frequency, and overall business outcomes.
On a similar theme: Azure Microservice
Architecture and Design
Choosing an architectural style for your microservices-based application is crucial, and it's not just about following a trend. You need to understand the underlying principles and constraints of that style to reap its benefits.
Each architecture style has its own trade-offs, so it's essential to identify the nature of the problem you're trying to solve and the business drivers that correspond to it. This will help you prioritize the architecture characteristics, such as maintainability, testability, and reliable by rapid deployment capabilities.
For example, if you need to deploy your application quickly, you might prioritize maintainability and testability. On the other hand, if you have a constrained budget, you might prioritize feasibility and simplicity.
Here's a table summarizing how each style manages dependencies and the types of domains that are best suited for each:
Choosing and maintaining an architectural style is not a one-off activity, but a continuous approach. The architecture should be continuously measured, validated, and fine-tuned over time.
Benefits and Challenges
Pragmatic microservices with C# and Azure offer several benefits, including improved scalability, as we can scale individual microservices independently without affecting the entire application. This allows for more efficient use of resources.
One of the key benefits of microservices is the ability to use different programming languages and frameworks for each service, as seen in our example of using C# for some services and other languages for others.
With Azure, we can take advantage of cloud-based services to support our microservices architecture, such as using Azure Kubernetes Service (AKS) for container orchestration.
For another approach, see: How to Use Azure
However, implementing microservices also presents several challenges, including increased complexity due to the need for multiple communication channels between services.
The use of API gateways, such as Azure API Management, can help simplify communication between services and provide a single entry point for clients.
Another challenge is the need for more robust monitoring and logging to diagnose issues in a distributed system, which can be addressed using Azure Monitor and Application Insights.
Communication and Data
Microservices communicate with each other through HTTP protocol using REST APIs, but this can lead to dependencies between services, affecting performance and reliability.
Using asynchronous communication patterns like pub/sub helps keep components loosely coupled, allowing services to react to events without affecting each other's performance.
In a pub/sub pattern, a service publishes events to an event bus, which acts like a FIFO queue, ensuring messages are processed in the order they were received.
Events are often published on an event bus, which is a component that works like a queue, and services can subscribe to events to react to them.
Using asynchronous patterns like pub/sub allows services to be completely independent from each other, making it easier to scale and maintain the application.
The Notifications service, for example, listens to the Service Bus queue and triggers an event when a new message is received, demonstrating how services can communicate and react to events.
Communication Between
Communication between microservices is a crucial aspect of building a cloud-native application.
In traditional applications, components communicate with each other through abstraction, but this isn't possible in microservice architecture since the code base is different.
Using HTTP protocol and REST APIs is one way for microservices to communicate, but this can introduce dependencies and affect the performance of other services.
Asynchronous communication patterns can help keep components loosely coupled and independent from each other.
Pub/sub is a common pattern where a service publishes events and others may subscribe to them, often using an event bus that works like a FIFO queue.
This approach allows service B to react to events from service A without affecting its performance or knowing who the consumers are.
In some cases, synchronous communication may be unavoidable, but it's generally considered an antipattern.
A fresh viewpoint: Windows Azure Components
Configuring Continuous Integration:
To set up continuous integration, you can use a CI trigger that builds on each commit. This trigger is defined in the build-deploy.yml file under the .github/workflows folder.
You can set up a job to understand which paths are changed and trigger the right jobs. This is achieved by introducing a job that sets dependencies to this job through the keywords needs.
In the build-deploy.yml file, you can define environment variables declarations, such as ASPNETCORE_ENVIRONMENT, PROJECT_PRODUCT_CATALOG, REGISTRY_NAME, and DB_CONNECTION_STRING.
These variables are used to set the environment for the ASP.NET application, the path to the ProductCatalog csproj file, the ACR name to query, and the database connection string.
To optimize the deployment process, you can introduce a job to understand which paths are changed and trigger the right jobs. This is done by adding a job with the aim to understand which paths are changed and trigger the right jobs.
Here's a list of environment variables that can be declared in the build-deploy.yml file:
- ASPNETCORE_ENVIRONMENT
- PROJECT_PRODUCT_CATALOG
- REGISTRY_NAME
- DB_CONNECTION_STRING
- ... (other variables will be added later on)
By defining these variables, you can ensure that the correct environment is set for the ASP.NET application and the database connection string is correctly configured.
To configure continuous integration, you can also use GitHub Secrets to store sensitive information, such as Azure AD credentials and database connection strings. This is done by adding secrets to the GitHub repo web page, under Settings > Secrets.
Here's a list of secrets that can be added to the GitHub repo:
- AZURE_AD_CLIENT_ID
- AZURE_AD_CLIENT_SECRET
- AZURE_AD_PASSWORD
- AZURE_AD_TENANT_ID
- AZURE_CREDENTIALS
- AZURE_SUBSCRIPTION_ID
- DB_ADMIN_USERNAME
- DB_ADMIN_PASSWORD
- DB_HOST
- DB_NAME
- DB_PORT
By storing these secrets in the GitHub repo, you can ensure that sensitive information is securely stored and can be used by the continuous integration pipeline.
Technologies and Tools
The technologies and tools used in this pragmatic microservices approach are quite fascinating. ASP.NET applications are at the core, running on .NET 6, which allows for seamless integration with Continuous Integration (CI) and deployment techniques.
A fresh viewpoint: Windows Azure Integration
GitHub hosts the repository and the build and deployment pipelines are scripted on top of GitHub Actions. This streamlines the development process and ensures that the application is always up-to-date.
Cloud infrastructure is scripted using Terraform, providing a full Infrastructure as a Code (IaC) experience. This allows for easy management and versioning of the infrastructure.
Sensitive data such as connection strings are stored in a secure place on Azure, not checked into the code repository.
Here's a brief rundown of the Docker setup:
- -t gives the name to the image
- -f specifies the Docker file location and the build context
- COPY refers to the ProductCatalog folder
In addition, environment variables can be set using the -e flag, as seen in the Docker run command. This allows for easy configuration of the application.
Technologies Used
ASP.NET applications are used to build both services, running on .NET 6. This allows for efficient and scalable development.
GitHub hosts the repository for these services, using Continuous Integration (CI) and deployment techniques. The build and deployment pipelines are scripted on top of GitHub Actions.
Take a look at this: Azure Deployments
Cloud infrastructure is scripted using a declarative approach with Terraform, providing a full Infrastructure as a Code (IaC) experience.
Sensitive data, such as connection strings, are stored securely on Azure and not checked into the code repository.
A Postgresql database is used to store data for the Pc service, while the Nts service communicates with it through an event bus with a queue.
A unique perspective: Azure Code
Docker
Docker is a crucial tool for building and deploying containerized applications. It allows you to package your code and its dependencies into a single container that can be run anywhere.
To get started with Docker, you'll need to create a Dockerfile in your project folder. This file will contain the instructions for building your Docker image. Don't forget to add a .dockerignore file as well, which will help exclude unnecessary files from your Docker build.
If your Docker build is stuck on the dotnet restore command, you might encounter a bug that's been documented online. To fix it, add a specific node to your csproj file and modify the Dockerfile commands accordingly.
To build your Docker image locally, navigate to your project folder in the CLI and run the following command: `docker build -t productcatalog -f Docker\Dockerfile .`. This will give your image the name "productcatalog" and specify the Dockerfile location and build context.
Here's a breakdown of the command options:
- `-t` gives the name to the image
- `-f` specifies the Docker file location and the build context, represented by the dot that stands for the current folder
Once you've built your Docker image, you can run it using the `docker run` command. This will start your application and make it accessible on your local machine. The command to run your Docker image is: `docker run --name productcatalogapp -p 8080:80 -it productcatalog -e ConnectionStrings:ProductCatalogDbPgSqlConnection="Host=localhost;Port=5432;Username=sqladmin;Password=Password1!;Database=product_catalog;Include Error Detail=true"`.
Here's a breakdown of the command options:
- `--name` gives the name to the container
- `-p` binds host and container ports
- `-e` sets an environment variable, in this case the connection string
Sources
- https://www.microsoftpressstore.com/store/developing-microservices-architecture-on-microsoft-9780136819387
- https://learn.microsoft.com/en-us/azure/architecture/guide/architecture-styles/
- https://www.infoq.com/articles/cloud-native-development-azure/
- https://stackoverflow.com/questions/34593341/trade-offs-and-best-practices-building-microservices-with-azure-service-fabric
- https://medium.com/@raphaelyoshiga/spinning-up-a-microservice-under-60-minutes-with-net-core-vsts-and-azure-app-services-c60b6fa7d4bd
Featured Images: pexels.com