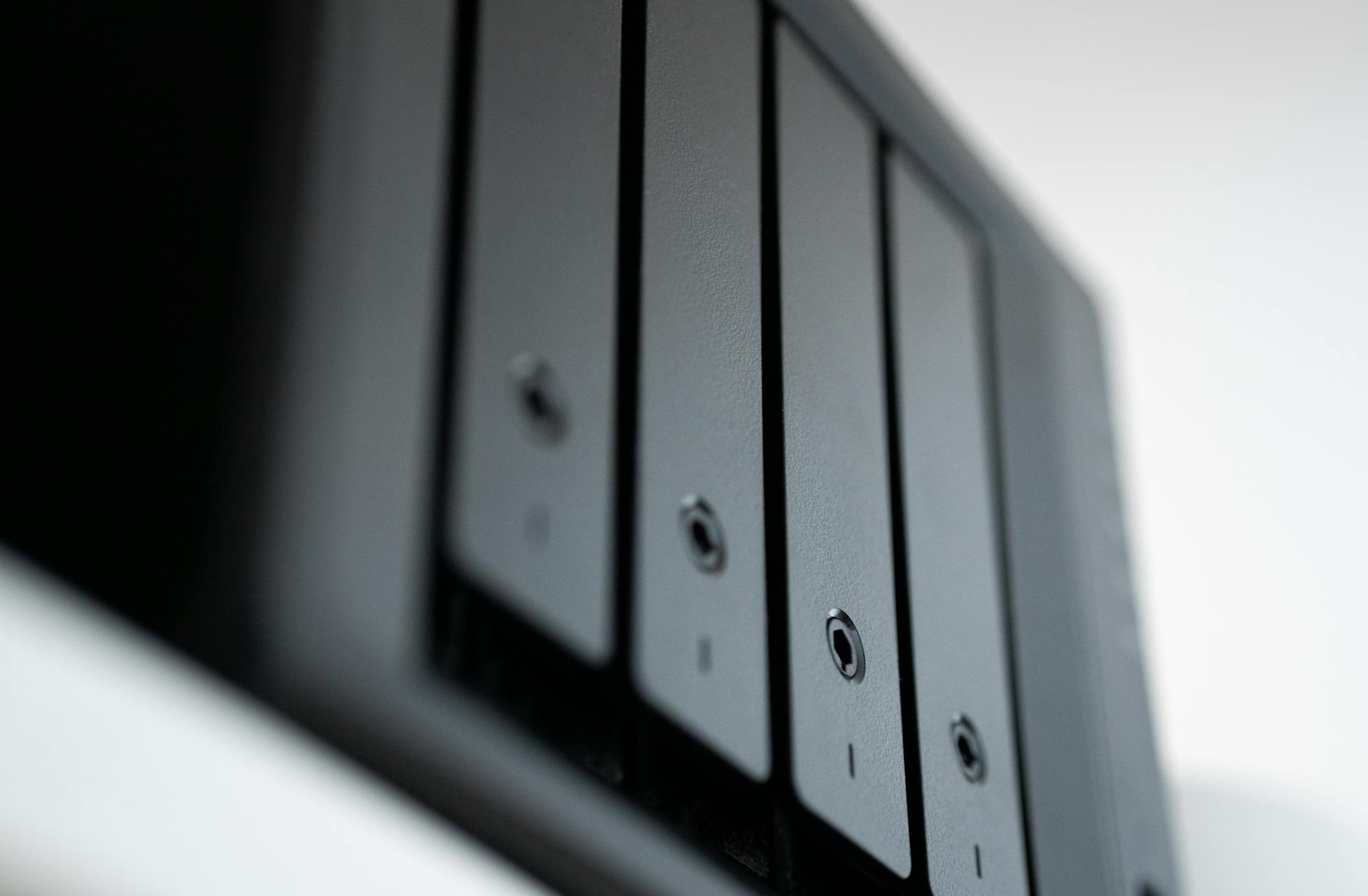
Azure .NET Filestorage is a reliable and secure way to store and manage files in the cloud. It's designed to work seamlessly with .NET applications, making it a great choice for developers.
With Azure .NET Filestorage, you can store files up to 5 TB in size, and access them from anywhere with an internet connection. This is especially useful for large files or datasets that need to be shared with team members or clients.
You can also use Azure .NET Filestorage to manage file permissions and access controls, ensuring that sensitive data is only accessible to authorized users. This is a key feature for businesses that need to comply with data protection regulations.
Azure .NET Filestorage is highly available, with a 99.9% uptime guarantee, so you can rely on it to keep your files safe and accessible. This is a major advantage over traditional file storage solutions that can be prone to downtime and data loss.
For your interest: Azure Key Value Store
Getting Started
To get started with Azure .NET File Storage, you'll want to begin with single client registration, which is the simplest way to configure the Azure Blob Storage SDK. This involves creating only one BlobServiceClient in an ASP.NET Core application.
The Azure Blob Storage SDK can be configured in various ways, but single client registration is a great starting point, especially for small-scale applications.
Additional reading: Create Azure Blob Storage
Authentication and Authorization
To authenticate to Azure and authorize access to blob data, you can use the DefaultAzureCredential class provided by the Azure Identity client library, which is the recommended approach for implementing passwordless connections to Azure services.
This class supports multiple authentication methods and determines which method to use at runtime, enabling your app to use different authentication methods in different environments without implementing environment-specific code.
The DefaultAzureCredential class looks for credentials in a specific order and locations, which can be found in the Azure Identity library overview.
Here's an interesting read: Azure Auth Json Website Azure Ad Authentication
You can also authorize requests to Azure Blob Storage by using the account access key, but this approach should be used with caution, as anyone who has the access key can authorize requests against the storage account and effectively has access to all the data.
To use the account access key, you'll need to provide the full connection string, including the storage account access key, and make sure to never expose the keys in an unsecure location.
Here are the two main options for authorizing requests to Azure Blob Storage:
- Passwordless (Recommended)
- Connection String
Single Client Registration
Single Client Registration is a straightforward process. You can configure the Azure Blob Storage SDK to create only one BlobServiceClient in an ASP.NET Core application. This is the simplest way to get started.
To achieve this, you'll need to add the Azure Blob Storage SDK to your project. You can do this by running the following command in the terminal: `dotnet add package Azure.Storage.Blobs`.
See what others are reading: Azure Storage Blob Download
Once the package is installed, you can create a single BlobServiceClient instance in your code. This client will be used to interact with your Azure Blob Storage account.
Here's a step-by-step guide to creating a single BlobServiceClient:
1. Install the Azure Blob Storage SDK package using the command `dotnet add package Azure.Storage.Blobs`.
2. Create a new instance of the BlobServiceClient class, passing in the URI of your Azure Blob Storage account and a DefaultAzureCredential instance.
3. Use the BlobServiceClient instance to perform operations on your Azure Blob Storage account.
By following these steps, you'll have a single BlobServiceClient instance that you can use to interact with your Azure Blob Storage account. This is a great starting point for building applications that use Azure Blob Storage.
Broaden your view: Azure Function C
Storage Operations
Storage Operations are a crucial part of working with Azure .NET File Storage. You can upload a file to Azure File Storage using the Upload operation. This operation returns the uploaded file.
To configure Azure Blob Storage SDK, you can use Microsoft.Extensions.Azure, which provides several ways to configure the Azure Storage SDK. The simplest way is to create a corresponding section in your configuration.
You can then retrieve the instance of BlobServiceClient via dependency injection, which uses reflection to find the appropriate constructor.
Related reading: .net Azure Sdk
Create
Creating a file in Azure File Storage is a straightforward process. You can create a file by specifying the folder path, file name, and file content. This can be achieved by using the Azure File connector, which allows you to create a file in Azure File Storage.
To create a file, you need to provide the following information:
This information can be provided in the Azure File connector, allowing you to create a file in Azure File Storage.
Get Content via Path
To get content via path, you specify a unique file path. This operation is used to retrieve file contents.
The file path must be a string, and it's required to specify the unique path to the file. You can infer the content type based on the file's extension, but this is optional.
Here's a summary of the required input for this operation:
Note that you can choose to infer the content type, but it's not mandatory. If you don't specify the content type, the operation will still retrieve the file contents.
Container Upload
Container Upload is a key feature of Azure File Storage. You can upload files to a container using the UploadAsync method.
To upload a file, you'll need to specify the folder path, file name, and content of the file. The folder path is required and should be a string, as shown in the "Create file" example.
Here are the required fields for uploading a file:
The UploadAsync method returns the result of the operation, which is to upload a file to Azure File Storage, as stated in the "Returns" example.
Storage Configuration
To configure Azure Blob Storage, you can use Microsoft.Extensions.Azure, which provides several ways to do so, ranging from simple to flexible configurations.
You can create a corresponding section in your configuration to retrieve the instance of BlobServiceClient via dependency injection. Microsoft.Extensions.Azure uses reflection to find the appropriate constructor to use to create the BlobServiceClient instance based on the provided configuration section.
For a more straightforward approach, you can simply create the section in your configuration and let Microsoft.Extensions.Azure handle the rest.
Here are the ways to configure Azure Blob Storage SDK with Microsoft.Extensions.Azure:
- Simple configuration
- Flexible configuration
Parameters
Storage Configuration involves specifying key details to ensure seamless storage operations.
To get started, you'll need to provide the source URL, which is a must-have for the process.
The source URL is a string that should point to the source file.
A destination file path is also required, and it should include the target filename.
The destination file path is also a string, and it's essential to specify it correctly.
You'll also need to decide whether the destination file should be overwritten or not.
Here's a summary of the required parameters:
Planning Your Deployment
Planning your deployment is an essential step in configuring your storage setup. It's crucial to consider the specific needs of your project or organization.
First, you'll want to plan your Azure Files deployment, which involves setting up a cloud-based file share that can be accessed from anywhere. This is a great option for teams that need to collaborate on files.
To plan your Azure Files deployment, you'll need to decide on the storage size and replication options that best suit your needs. Azure Files offers a range of storage options, from 5 TB to 100 TB, so choose the one that fits your budget.
Next, you'll want to plan your Azure File Sync deployment, which allows you to synchronize files across multiple locations. This is particularly useful for organizations with multiple offices or teams that need to access the same files.
To plan your Azure File Sync deployment, you'll need to set up a cloud endpoint and configure the sync settings. This will ensure that your files are synced across all locations in real-time.
If you're migrating from StorSimple, you'll need to plan your migration carefully to ensure a smooth transition. This may involve assessing your current storage setup and determining the best way to migrate your data to Azure.
Here are the key steps to consider when planning your deployment:
- Plan your Azure Files deployment
- Plan your Azure File Sync deployment
- Migrate from StorSimple
Additionally, you'll want to consider how to deploy Azure Files and Azure File Sync. This will involve setting up the necessary infrastructure and configuring the services to meet your needs.
By following these steps and considering your specific requirements, you'll be well on your way to planning a successful deployment.
Sources
- https://www.nuget.org/packages/microsoft.azure.storage.file/
- https://learn.microsoft.com/en-us/connectors/azurefile/
- https://anthonysimmon.com/best-practices-azure-storage-sdk-integration-in-dotnet-apps/
- https://azure.microsoft.com/en-us/products/storage/files
- https://learn.microsoft.com/en-us/azure/storage/blobs/storage-quickstart-blobs-dotnet
Featured Images: pexels.com