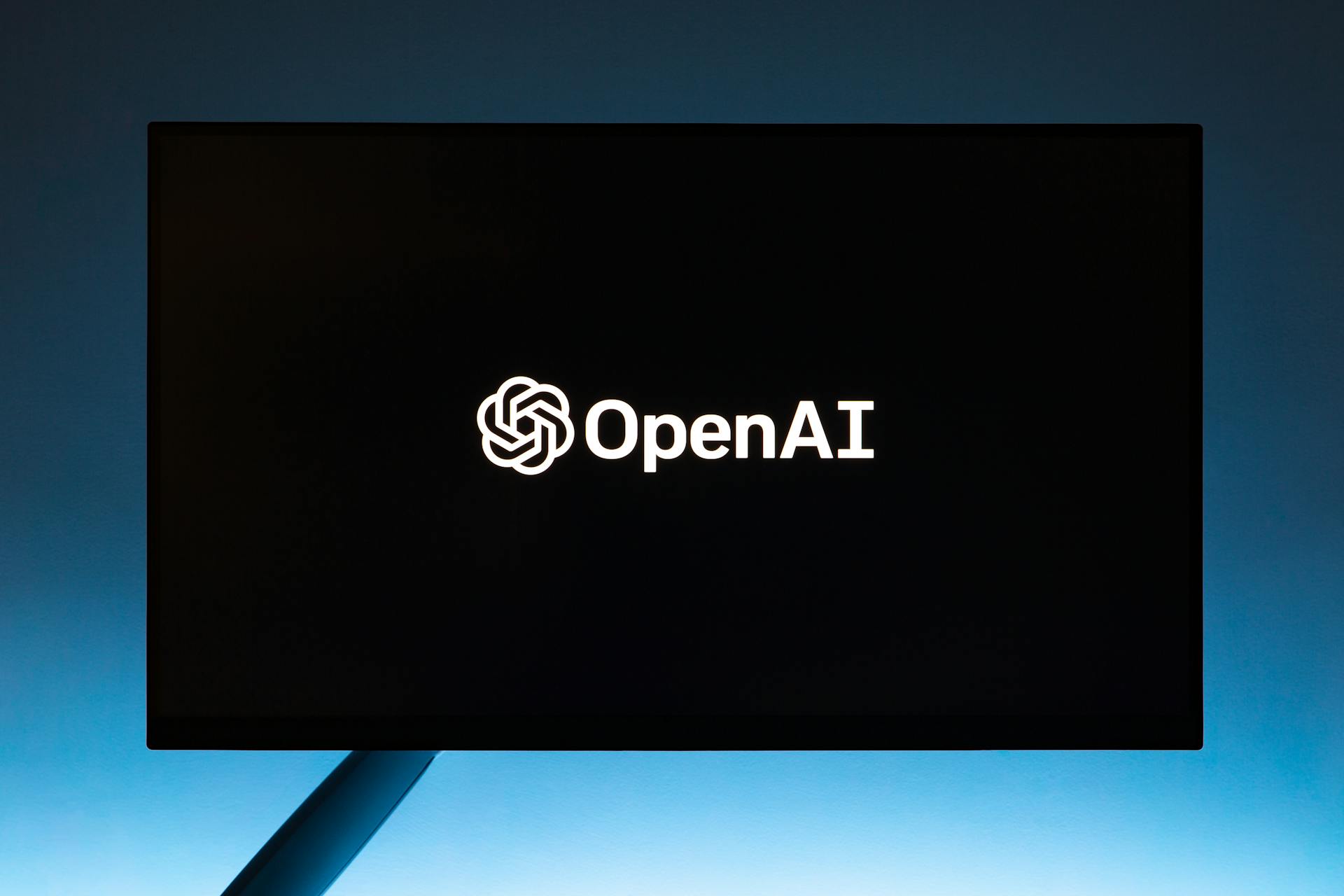
To get started with Azure OpenAI Python, you'll need to install the Azure OpenAI SDK, which can be done using pip with the command "pip install azure-ai-openai".
The SDK provides a simple and intuitive interface for interacting with the OpenAI API, making it easy to get started with building AI-powered applications.
To authenticate with the Azure OpenAI API, you'll need to create an Azure OpenAI resource and obtain an API key, which can be done through the Azure portal.
With the SDK installed and your API key in hand, you can start exploring the capabilities of the OpenAI API, including text generation, completion, and more.
Intriguing read: Azure Openai Key
Setting Up Your Environment
To set up your environment for Azure OpenAI Python, start by creating an Azure account if you don't already have one. You can sign up at the Azure Portal.
Next, create an OpenAI Resource by navigating to the Azure portal, creating a new resource, and selecting 'OpenAI Service'. Fill in the required details and create the resource.
To authenticate your requests, you'll need to obtain your API key, which can be found on the resource page after creating the resource.
Here's a quick checklist to get you started:
- Create an Azure Account
- Create an OpenAI Resource
- Obtain your API key
Installing Libraries
To set up your environment, you need to install the required libraries. You can do this using pip.
Installing the openai Python package is a straightforward process. Simply open your terminal or command prompt and type "pip install openai".
This will download and install the necessary files for you to interact with the Azure OpenAI API.
Setting Up Your Environment
To set up your environment for the Azure OpenAI API, start by creating an Azure account if you don't already have one. You can sign up at the Azure Portal.
Once you have an Azure account, create an OpenAI resource by navigating to the Azure portal, creating a new resource, and selecting 'OpenAI Service'. Fill in the required details and create the resource.
You'll need to obtain an API key from the resource page, which is essential for authenticating your requests. This key is unique to your account and resource.
To interact with the Azure OpenAI API, you'll need to install the openai Python package using pip.
Curious to learn more? Check out: Azure Developer Account
Here's a quick rundown of the steps to create an OpenAI resource:
- Create an Azure Account: Sign up at Azure Portal.
- Create an OpenAI Resource: Navigate to the Azure portal, create a new resource, and select 'OpenAI Service'. Fill in the required details and create the resource.
- Obtain API Key: Go to the resource page to find your API key.
Active Directory Authentication
Active Directory Authentication is a great way to secure your Azure OpenAI setup. You can authenticate using Azure Active Directory (AAD) instead of an API key.
There are two ways to authenticate with Azure OpenAI: API Key and Azure Active Directory. If you have complex security requirements, you may want to use AAD.
To use AAD, you need to add a role assignment to your Azure OpenAI resource. This allows you to get a token from AAD to use with Azure OpenAI. You can grant this role assignment to a user, group, service principal, or managed identity.
You can use the DefaultAzureCredential class to get a token from AAD. This class is an easy way to get started with AAD authentication. It tries Managed Identity first, then falls back to the Azure CLI.
To use AAD in Python with LangChain, install the azure-identity package. Then, set OPENAI_API_TYPE to azure_ad. Finally, set the OPENAI_API_KEY environment variable to the token value.
Here are some options for granting role assignments:
- User
- Group
- Service principal
- Managed identity
Making API Calls
Making API calls with the Azure OpenAI API in Python is a straightforward process. You can start making API calls once your environment is configured.
To make API calls, you'll need to use the openai Python package. Here’s a simple example of how to call the Azure OpenAI API: send a message to the model and print the response.
Ensure that you replace the model name with the one you are using. This will allow your application to authenticate and communicate with the Azure services securely.
By following these steps, you can successfully configure and utilize the Azure OpenAI API in your Python applications, enabling you to leverage powerful AI capabilities seamlessly.
You can configure the openai package to use Azure OpenAI using environment variables. The following is for bash: configure the API right within your running Python environment.
Alternatively, you can install the Azure CLI and log in to configure the API. This will allow you to interact with the Azure OpenAI service directly from your Python application.
Consider reading: How to Use Azure
Integrating with LangChain
Integrating with LangChain is a straightforward process that can be completed in a few steps. First, you need to install LangChain in your project.
To set up the connection to your Azure OpenAI instance, you can use the provided methods in LangChain. This will allow you to harness the power of generative AI models provided by Azure OpenAI.
Here's a step-by-step guide to setting up the integration:
- Install LangChain: Make sure you have LangChain installed in your project.
- Configure the Integration: Use the provided methods in LangChain to set up the connection to your Azure OpenAI instance.
By following these steps, you can seamlessly integrate LangChain with Azure OpenAI and unlock the full potential of this powerful combination.
Writing and Deploying AI Code
To write and deploy AI code with Azure OpenAI, you'll need to use your deployment's REST endpoints or the OpenAI Python libraries. The latter is probably your quickest route to live code.
First, gather the necessary information: the endpoint URL, an authentication key, and the name of your deployment. Set the appropriate environment variables for your code, but don't hard-code keys - use a tool like Azure Key Vault to manage them instead.
Curious to learn more? Check out: Azure Deployments
You can call an endpoint using the openai.Completion.create method, setting the maximum number of tokens needed to contain your prompt and its response. The response object contains the text generated by your model, which can be extracted and formatted for use in your code.
To specify a deployment, use the engine parameter in the openai Python API, such as 'gpt-35-turbo-instruct-prod'. This will ensure you're using the correct model for your task.
Tools and Pricing
Azure OpenAI's tools and pricing are surprisingly straightforward. The function metadata is declared in JSON, which is then passed to OpenAI through the tools parameter.
The OpenAI pricing model is token-based, with each token representing roughly four characters of text. You can expect 75 words to require about 100 tokens, roughly a paragraph of normal text.
Here's a rough estimate of the costs involved: the base model Ada comes in at about $0.0004 per 1,000 tokens, while the high-end Davinci is $0.02. Keep in mind that actual pricing can vary depending on your organization's account relationship with Microsoft.
For another approach, see: Create an Ai Model in My Azure Tenant
Tools
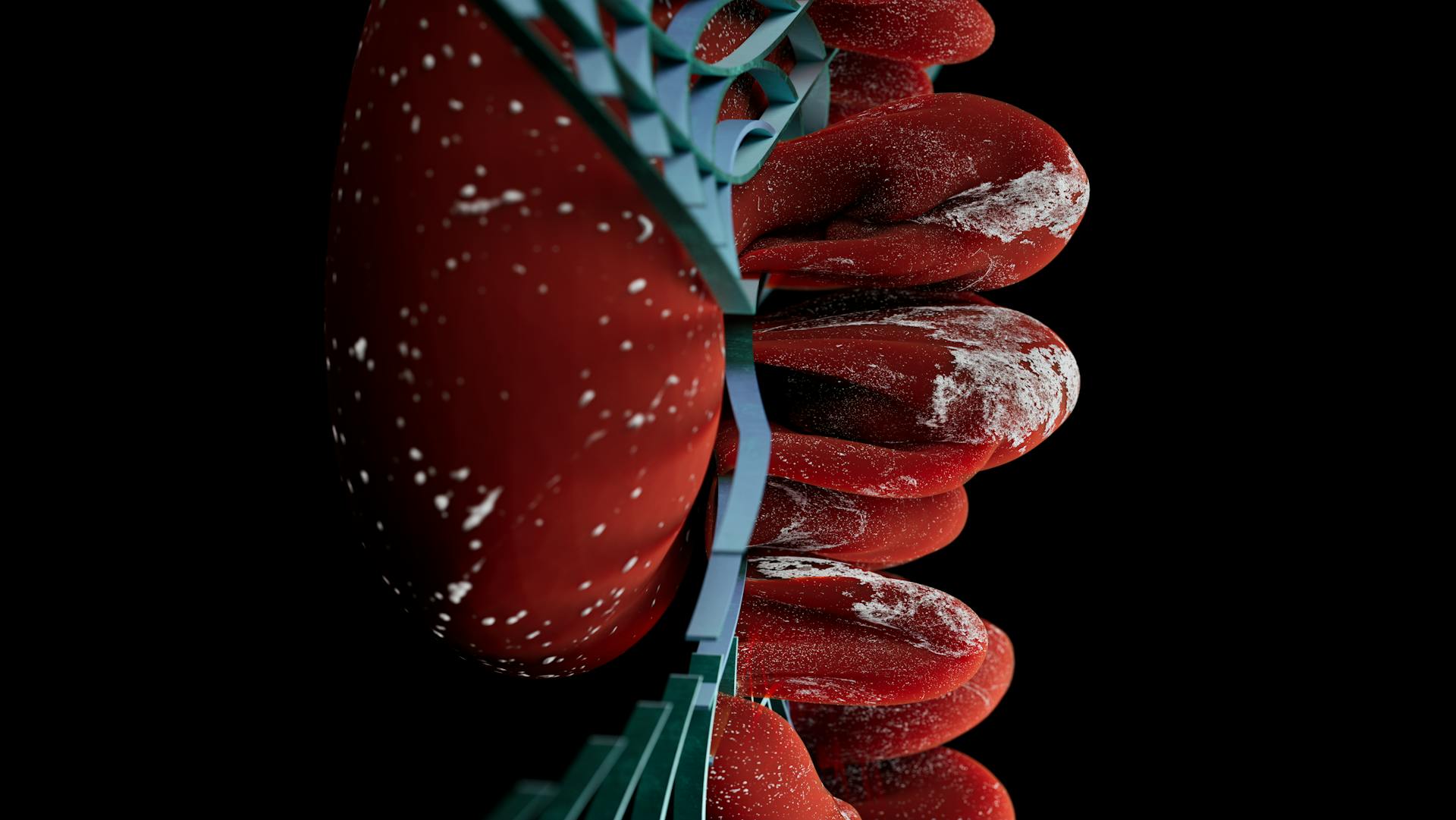
The function metadata is declared in JSON, which is a crucial step in using OpenAI's tools. This metadata is then passed to OpenAI in the tools parameter when making a call.
Function calling is a key feature of OpenAI's tools, and it allows you to pass function metadata in the tools parameter. This metadata is used by OpenAI to verify the function is to be called.
The tools_calls field in OpenAI's response contains a function name, which can be used to verify the function is being called correctly. For example, the function name "search_sources" is used in the provided example.
In order to use function calling, you need to parse the search_query parameter and value from the JSON passed back from OpenAI. This is done by the get_search_query() method, which verifies the function is to be called and parses the search_query parameter and value.
Here's a step-by-step summary of the function calling process:
- The function metadata is declared in JSON.
- The function metadata is passed to OpenAI in the tools parameter.
- OpenAI responds with a tools_calls field containing a function name.
- The get_search_query() method verifies the function is to be called and parses the search_query parameter and value.
Pricing
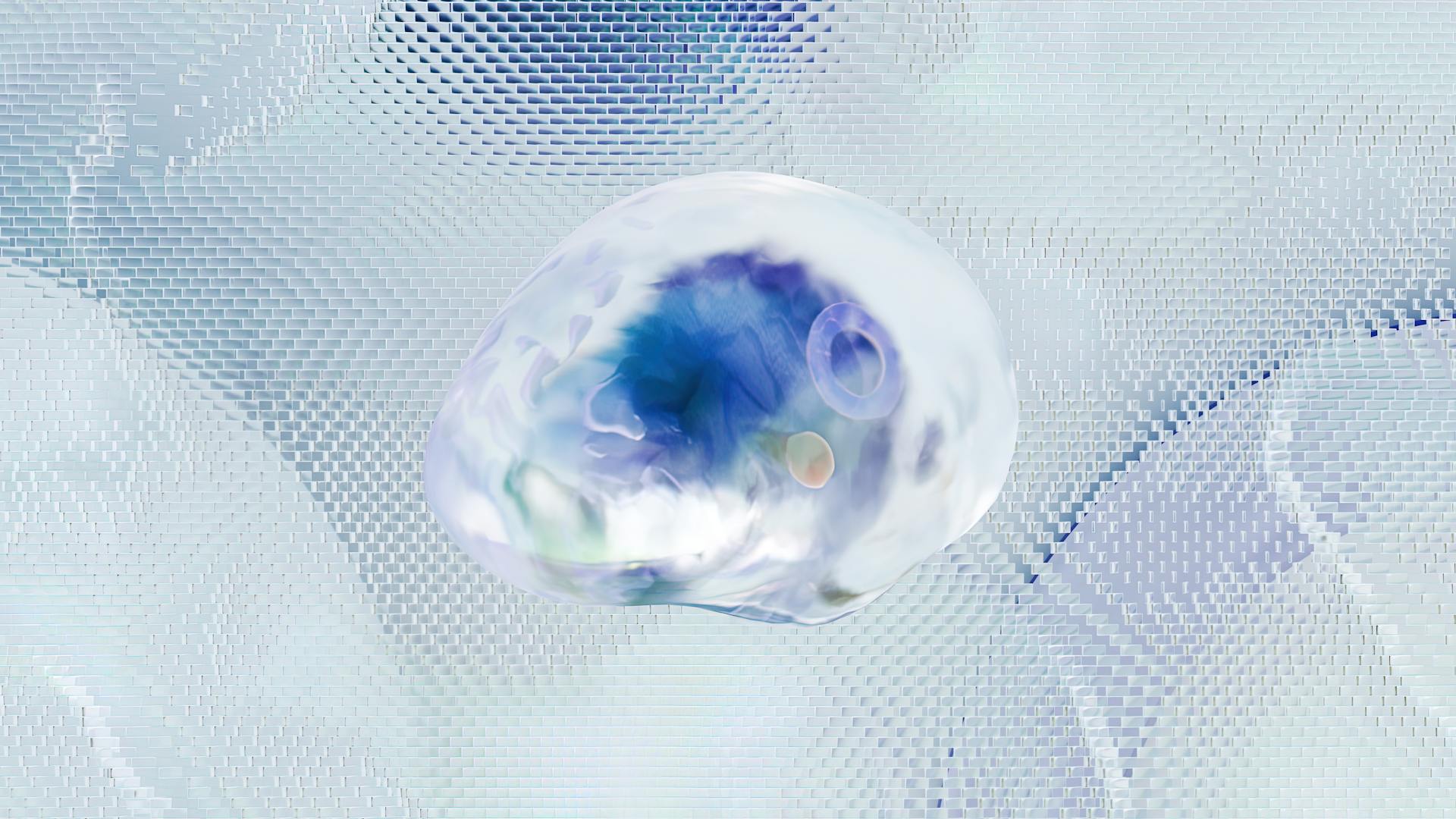
Azure OpenAI's pricing model is based on tokens, not the familiar authentication tokens. Tokens are essentially sections of strings created using an internal statistical model.
You can expect a token to be roughly four characters of text, though it can be less or more.
The more complex the model, the higher priced the tokens. The base model Ada comes in at about $0.0004 per 1,000 tokens, while the high-end Davinci is $0.02.
Exploring Azure OpenAI
To get started with Azure OpenAI, you'll need to create an Azure resource from the portal, the Azure CLI, or Arm templates. This will allocate the necessary resources to your account and resource group.
You can then deploy a model using Azure OpenAI Studio, which is where you'll do most of your work with OpenAI. Currently, you can choose between members of the GPT-3 family of models, including the code-based Codex.
The GPT-3 family includes models like Ada, which is the lowest cost and least capable, and Davinci, which is the highest. Each model is a superset of the previous one, so as tasks get more complex, you don't need to change your code, you simply choose a different model.
Microsoft recommends starting with the most capable model when designing an OpenAI-powered application, as this lets you tune the underlying model for price and performance when you go into production. This approach can save you time and effort in the long run.
Curious to learn more? Check out: Azure Ai Models
Best Practices and Examples
Implementing error handling is crucial when making API calls to Azure OpenAI. This helps manage exceptions that may arise during the process.
Be aware of the rate limits imposed by Azure OpenAI to avoid throttling. This will ensure your application doesn't get blocked.
Keep your API key secure and do not expose it in public repositories. This is a security best practice to prevent unauthorized access.
Here are some resources to learn more about integrating Azure OpenAI into your applications:
- Openai Api Tutorial Github
- Open Ai Api In Python
- Azure OpenAI Assistants API Python
Sources
- https://www.restack.io/p/azure-openai-api-knowledge-use-python
- https://www.linkedin.com/posts/azure-cosmos-db_github-azurecosmosdbazure-openai-python-developer-guide-activity-7232386267596947456-CShy
- https://jasonhaley.com/2024/02/23/azure-search-openai-demo/
- https://www.infoworld.com/article/2337918/getting-started-with-azure-openai.html
- https://python.langchain.com/docs/integrations/llms/azure_openai/
Featured Images: pexels.com