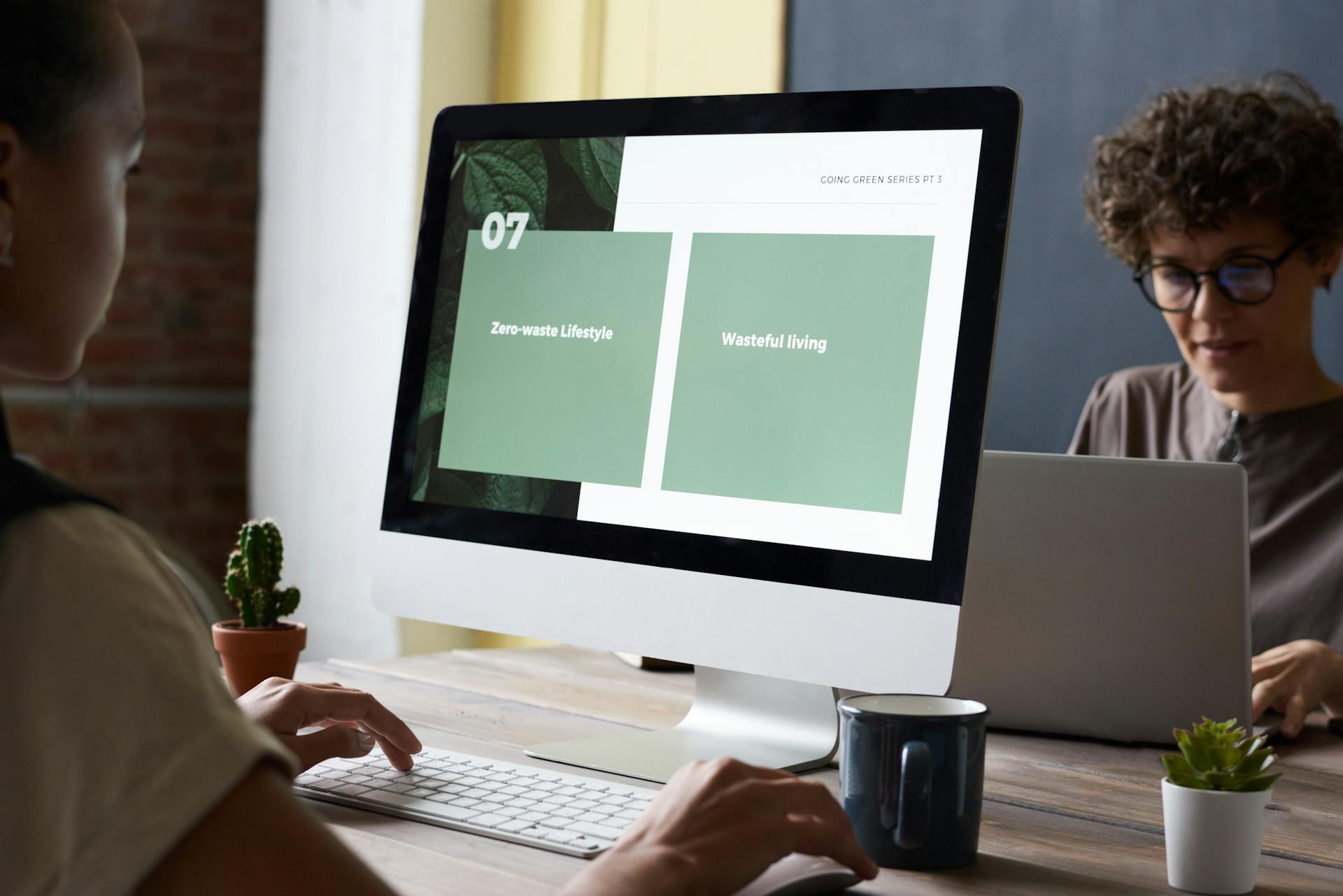
Azure OpenAPI is a powerful tool for designing, building, and documenting RESTful APIs. It's a great choice for developers who want to create scalable and maintainable APIs.
To get started with Azure OpenAPI, you'll first need to install the Azure OpenAPI extension for Visual Studio Code. This extension provides a set of tools and features that make it easy to work with OpenAPI definitions.
You can find the Azure OpenAPI extension in the Visual Studio Code marketplace. Simply search for "Azure OpenAPI" and click the "Install" button to get started.
Once you've installed the extension, you can create a new OpenAPI definition by clicking on the "Azure OpenAPI" icon in the Visual Studio Code sidebar. This will open a new file with a basic OpenAPI definition template.
Explore further: Azure Data Studio vs Azure Data Explorer
Prerequisites
To get started with Azure OpenAPI, you'll need to have a few things in place.
First and foremost, you'll need an existing API Management instance. If you haven't already, go ahead and create one.
You'll also need an Azure OpenAI resource with a model deployed. Take note of the ID (name) of the deployment, as you'll need it later when testing the imported API in API Management.
Finally, make sure you have the necessary permissions to grant access to the Azure OpenAI resource from the API Management instance.
If this caught your attention, see: Azure Api Management Whitelist Ip
Importing OpenAPI
Importing OpenAPI is a straightforward process in Azure API Management. You can import an OpenAPI specification from a URL or a path to a specification using the az apim api import command.
To import an OpenAPI specification from a URL, you can use the az apim api import command with the --specification-url parameter. This command imports an OpenAPI specification from the specified URL to an API Management instance named apim-hello-world.
If you need to update the settings of the imported API, you can use the az apim api update command. This command updates the settings of the API Management instance named apim-hello-world.
Explore further: How to Use Azure
You can also import an OpenAPI specification from a path to a specification using the --specification-path parameter. This parameter allows you to import the specification from a local file or a path to a specification.
To import an OpenAPI specification using the Import-AzApiManagementApi Azure PowerShell cmdlet, you can use the -SpecificationPath parameter. This cmdlet imports an OpenAPI specification from the specified path to a specification to an API Management instance named apim-hello-world.
After importing the API, you can update the settings using the Set-AzApiManagementApi cmdlet. This cmdlet updates the settings of the API Management instance named apim-hello-world.
Expand your knowledge: Azure Api Management Timeout Settings
Adding OpenAPI
Adding OpenAPI to Azure API Management is a straightforward process. You can manually download the OpenAPI specification for the Azure OpenAI REST API and add it to API Management as an OpenAPI API.
To do this, navigate to your API Management instance in the Azure portal and select APIs > + Add API. Under Define a new API, select OpenAPI and enter a Display name and Name for the API.
Readers also liked: Azure Api Management Policy
You'll also need to enter an API URL suffix ending in /openai to access the Azure OpenAI API endpoints in your API Management instance. This is typically in the format of my-openai-api/openai.
Here's a step-by-step guide to adding an OpenAPI specification to API Management:
- In the Azure portal, navigate to your API Management instance.
- In the left menu, select APIs > + Add API.
- Under Define a new API, select OpenAPI.
- Enter a Display name and Name for the API.
- Enter an API URL suffix ending in /openai.
- Select Create.
Once you've completed these steps, the API is imported and displays operations from the OpenAPI specification.
Configuring OpenAPI
To define the swagger.json endpoint, you need to create an HTTP Trigger Function and delete its contents. Then, use the generateOpenApi3_1Spec helper function from the npm package to define the shared metadata and components that your OpenAPI spec requires.
This Function will be merged with the annotated Functions at runtime, so you need to define the shared components first. You can start by telling consumers about the API, which requires defining the info object with title and version.
The minimum information you need to provide is the title and version of your API, but since you're using $ref to reference a shared component schema, you should define that as well. For example, you can define a Game schema with properties such as id, state, questions, players, and answers.
Here's a list of the properties you can define in the Game schema:
Configure Authentication
Configuring authentication for Azure OpenAI API is a straightforward process. If you've imported the Azure OpenAI API directly to your API Management instance, authentication using the API Management instance's managed identity is automatically configured.
You can use an API key or a managed identity to authenticate to the Azure OpenAI API. For API keys, you'll need to provide the key when making API calls.
If you added the Azure OpenAI API from its OpenAPI specification, you'll need to configure authentication manually. This involves using API Management policies to set up authentication.
For more insights, see: Azure Auth Json Website Azure Ad Authentication
Defining Swagger.json Endpoint
To define a Swagger.json endpoint, you'll need to create a new HTTP Trigger Function in Azure Functions. Delete its contents and use the `generateOpenApi3_1Spec` helper function from the `@aaronpowell/azure-functions-nodejs-openapi` npm package.
You can import the `generateOpenApi3_1Spec` function and export it as the default export, like this: `import { generateOpenApi3_1Spec } from "@aaronpowell/azure-functions-nodejs-openapi"; export default generateOpenApi3_1Spec({});`
This will define the shared metadata and components that your OpenAPI spec requires, which will be merged with the annotated functions at runtime.
To define the API, you'll need to provide some basic information, such as the title and version of your API. You can do this by adding an `info` object to the `generateOpenApi3_1Spec` function, like this: `export default generateOpenApi3_1Spec({ info: { title: "Awesome trivia game API", version: "1.0.0" } });`
You'll also need to define any shared components, such as schema objects, that will be used throughout your API. For example, you can define a `Game` schema object like this: `export default generateOpenApi3_1Spec({ info: { title: "Awesome trivia game API", version: "1.0.0" }, components: { schemas: { Game: { type: "object", properties: { id: { type: "string", description: "Unique identifier for the game" }, state: { type: "string", description: "The status of the game", enum: ["WaitingForPlayers", "Started", "Complete"] }, questions: { type: "array", items: { $ref: "#/components/schemas/Question" } }, players: { type: "array", items: { $ref: "#/components/schemas/Player" } }, answers: { type: "array", items: { $ref: "#/components/schemas/PlayerAnswer" } } } } } });`
By defining these shared components, you can reference them throughout your API using the `$ref` keyword.
Here's an example of what the complete `generateOpenApi3_1Spec` function might look like:
```javascript
import { generateOpenApi3_1Spec } from "@aaronpowell/azure-functions-nodejs-openapi";
export default generateOpenApi3_1Spec({
For another approach, see: Azure Api Version
info: {
title: "Awesome trivia game API",
version: "1.0.0"
},
components: {
schemas: {
Game: {
type: "object",
properties: {
id: {
type: "string",
description: "Unique identifier for the game"
},
state: {
type: "string",
description: "The status of the game",
enum: ["WaitingForPlayers", "Started", "Complete"]
},
questions: {
type: "array",
items: {
$ref: "#/components/schemas/Question"
}
},
players: {
type: "array",
items: {
$ref: "#/components/schemas/Player"
}
},
answers: {
type: "array",
items: {
$ref: "#/components/schemas/PlayerAnswer"
}
}
}
}
}
}
});
```
Note that this is just an example, and you'll need to customize the `generateOpenApi3_1Spec` function to fit your specific use case.
On a similar theme: Azure Game
Testing OpenAPI
Testing OpenAPI is a crucial step to ensure your Azure OpenAI API is working as expected. You can test it in the API Management test console by supplying a model deployment ID configured in the Azure OpenAI resource.
To get started, select the API you created and go to the Test tab. From there, select an operation that's compatible with the model you deployed. The page will display fields for parameters and headers. Enter the necessary values, including any query parameters or request body, and select Send.
When the test is successful, the backend responds with a successful HTTP response code and some data. Appended to the response is token usage data to help you monitor and manage your Azure OpenAI API consumption.
You can also test the new API in the portal, which provides a convenient way for administrators to view and test the operations of an API. To do this, select the API you created and go to the Test tab. Select an operation, and the page will display fields for query parameters and headers.
Depending on the operation, enter query parameter values, header values, or a request body. Select Send, and the test console will send a request to API Management's CORS proxy, which forwards the request to the API Management instance, which then forwards it to the backend.
Here are the steps to test the Azure OpenAPI:
- Select the API you created in the previous step.
- Select the Test tab.
- Select an operation that's compatible with the model you deployed in the Azure OpenAI resource.
- Enter other parameters and headers as needed.
- Depending on the operation, enter query parameter values, header values, or a request body.
- Select Send.
Frequently Asked Questions
What is OpenAPI in Azure?
OpenAPI in Azure is a standardized format for describing RESTful APIs, enabling easy development and consumption of APIs by mapping resources and operations. It's a key component in building and documenting APIs in Azure, facilitating collaboration and automation.
Sources
- https://learn.microsoft.com/en-us/azure/api-management/azure-openai-api-from-specification
- https://learn.microsoft.com/en-us/azure/api-management/import-api-from-oas
- https://www.softwarecraftsperson.com/posts/2021-09-19-az-fn-swagger-openapi/
- https://www.aaron-powell.com/posts/2022-02-08-openapi-for-javascript-azure-functions/
- https://stuartleaver.dev/apis-using-the-openapi-specification-and-autorest-along-with-azure-api-management/
Featured Images: pexels.com