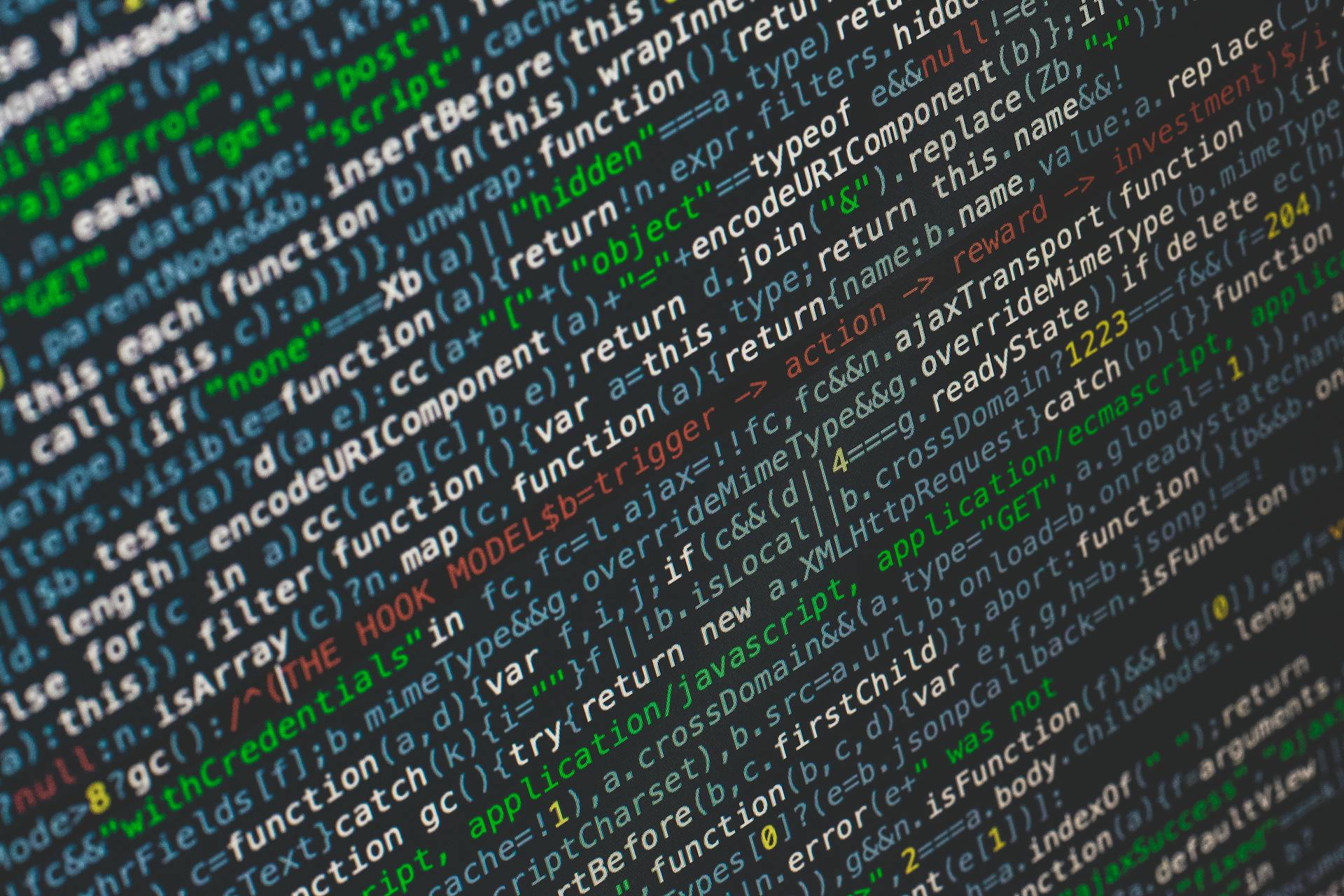
Changing the source of an image in JavaScript can be a bit tricky, but it's definitely doable.
You can change the `img` src attribute using the `setAttribute` method, as shown in the example `document.getElementById("myImage").setAttribute("src", "new-image.jpg");`.
To update the image dynamically, you can use the `src` property of the `img` element, like this: `document.getElementById("myImage").src = "new-image.jpg";`.
This method is more efficient and widely supported than using the `setAttribute` method.
Using jQuery
Using jQuery can be a convenient way to change the src attribute of an img element. You can use the attr() method to change the value of the src attribute.
The attr() method takes two parameters: the name of the attribute you want to change, and the new value you want to assign to it. For example, to change the src attribute of an img element, you can use the following syntax: attr('src', 'new-image.jpg').
You can also use the prop() method, which is similar to the attr() method, but it's specifically designed for changing properties of elements. The prop() method takes the same two parameters as the attr() method.
Expand your knowledge: Inspect Element Javascript
It's worth noting that using jQuery can slow down your page load, especially if you're using it to change the src attribute of multiple img elements. In that case, it's better to use the native JavaScript method, which is faster and more efficient.
Here are some examples of how to use the attr() and prop() methods to change the src attribute of an img element:
- Using attr(): attr('src', 'new-image.jpg')
- Using prop(): prop('src', 'new-image.jpg')
You can also use the attr() method to change the src attribute of multiple img elements at once, by selecting all img elements with a specific class or id.
For example, to change the src attribute of all img elements with the class "image2", you can use the following code: var images = document.getElementsByClassName("image2"); for (var i = 0; i < images.length; i++) { images[i].src = "new-image.jpg"; }
Similarly, to change the src attribute of an img element with a specific id, you can use the following code: var image = document.getElementById("image-id"); image.src = "new-image.jpg";
Take a look at this: Image Class in Css
Setting Attributes in JavaScript
Setting attributes in JavaScript can be achieved through various methods. You can use the square brackets syntax to set the src attribute of an image tag, but this method only works for a single image element.
The querySelector() method can be used to target a single image element, but it's not the most efficient way to set attributes for multiple elements. To get a reference to all image elements, you can use querySelectorAll().
You can also use the src property in JavaScript to get or update the value of the src attribute of an image element. This method is commonly used to change the value of the src attribute of an img element.
Here are some methods to change the src attribute of an image element:
- Using the src property in JavaScript
- Using the attr() method in jQuery
- Using the prop() method in jQuery
The attr() method in jQuery takes two parameters: the attribute name and the new value. The prop() method is similar, but it's used to change the value of a property.
Here are some key differences between the attr() and prop() methods:
In practical terms, the src property in JavaScript can be used to toggle between two images every time a button is clicked. This can be achieved by assigning a different value to the src attribute of the image element using the src property.
By understanding these methods and their differences, you can effectively set and change attributes in JavaScript, making it easier to work with HTML elements and create dynamic web applications.
For your interest: Add Css Property to a Predefined Class Javascript
Sources
- https://nextjs.org/docs/pages/api-reference/components/image
- https://www.tutorialspoint.com/how-to-change-the-src-attribute-of-an-img-element-in-javascript-jquery
- https://dev.to/rajatamil/create-image-elements-in-javascript-41ke
- https://dev.to/santiagocodes/replacing-empty-img-src-attributes-using-javascript-2pc8
- https://stackoverflow.com/questions/11722400/programmatically-change-the-src-of-an-img-tag
Featured Images: pexels.com