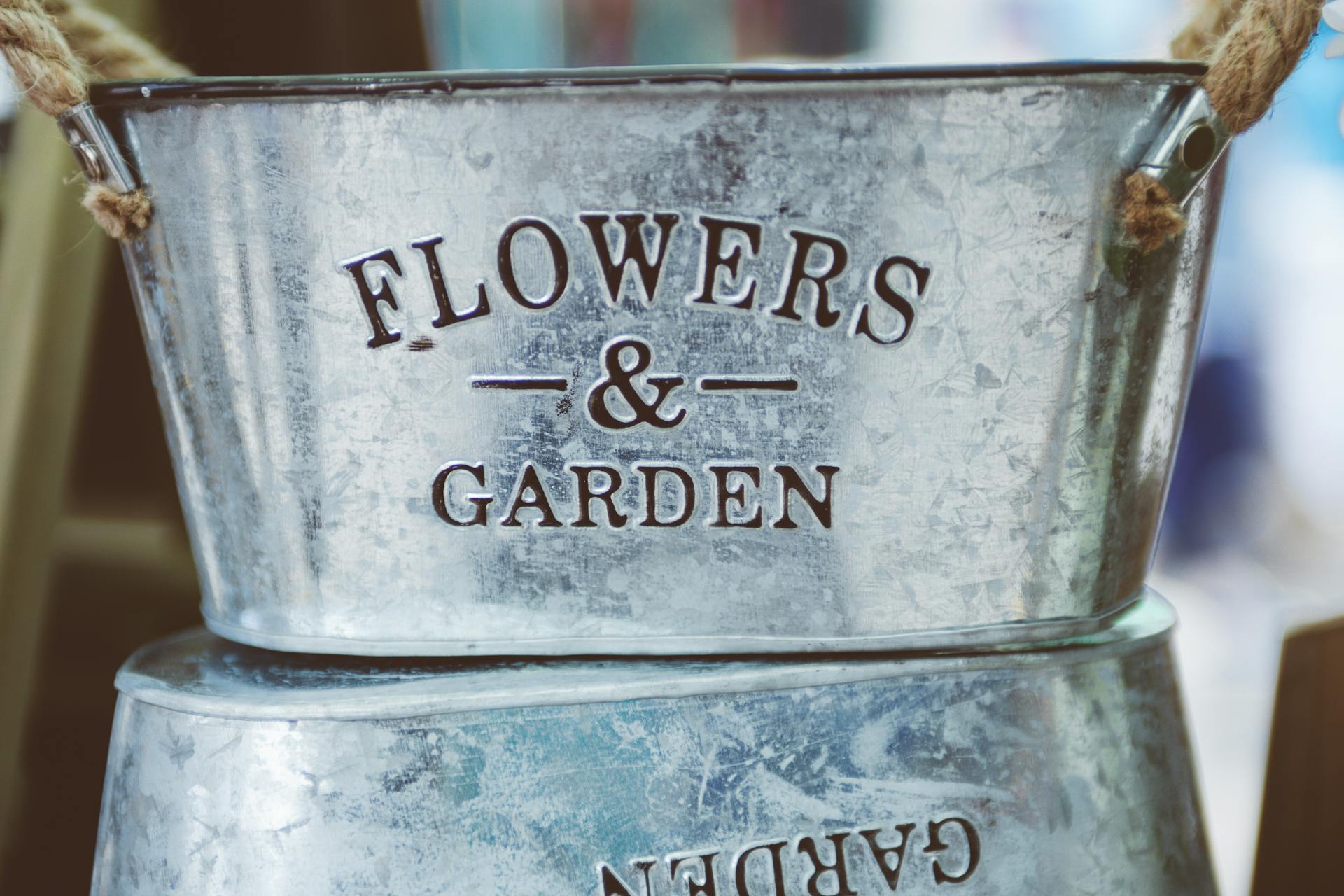
Creating an S3 bucket with AWS CDK is a straightforward process. You can create a new S3 bucket using the `s3.Bucket` class.
To create a bucket, you need to provide a unique name for it. The name must be between 3 and 63 characters long, and it must not contain any special characters except for dots.
The `s3.Bucket` class takes two main arguments: the name of the bucket and the object of the `s3.BucketProps` class. The `s3.BucketProps` object allows you to specify additional properties for the bucket, such as its versioning configuration.
In the next section, we'll explore how to configure versioning for your S3 bucket.
Additional reading: Aws S3 Object
Setting Up an Amazon S3 Bucket
You can create an S3 bucket in a simple one-liner, but adding important properties helps secure the bucket.
To simplify access control, you can disable access control lists (ACLs) and take ownership of every object in your bucket by setting the objectOwnership property.
The blockPublicAccess property ensures permissions on new objects are private by default and don't allow public access.
You can encrypt S3 bucket objects in rest by using a customer managed KMS key with the encryptionKey property.
The BucketAccessControl enum has several values, including PRIVATE, PUBLIC_READ, and PUBLIC_READ_WRITE, which you can use to set up bucket policies.
Here are some of the values of the BucketAccessControl enum:
- PRIVATE
- PUBLIC_READ
- PUBLIC_READ_WRITE
- AUTHENTICATED_READ
- LOG_DELIVERY_WRITE
- BUCKET_OWNER_READ
- BUCKET_OWNER_FULL_CONTROL
- AWS_EXEC_READ
Alternatively, you can set publicReadAccess to true for a more straightforward way to set the PUBLIC_READ policy for the bucket.
Project Setup
To set up your project for building an Amazon S3 Bucket using AWS CDK TypeScript, you need to create a new AWS CDK TypeScript project. You can do this by running the following command in an empty directory:
```bash
cdk init app --language typescript
```
This command will create a basic AWS CDK TypeScript project. Make sure you have installed the AWS CDK and TypeScript NPM packages, installed the AWS CLI, and configured an AWS profile before running this command.
To confirm you have completed the necessary prerequisites, check the following list:
- Install AWS CDK and TypeScript NPM packages
- Install the AWS CLI and configure an AWS profile
- Create an AWS CDK TypeScript project
By following these steps, you'll be ready to start building the Amazon S3 Bucket construct.
Synthesizing and Deploying
To synthesize your Amazon S3 bucket in AWS CDK, run the AWS CDK Synthesize command. This will print the stack output in YAML format in stdout.
The output will display the stack output in YAML format, giving you a clear view of your S3 bucket configuration. This is an essential step before deploying your S3 bucket to AWS Cloud.
To deploy your S3 bucket to AWS Cloud, run the deploy command. This command will sync all the changes you made in your code to the AWS server infrastructure.
Here are the steps to deploy your S3 bucket:
- Run the cdk deploy command.
- Verify that the S3 bucket was created in the AWS console.
- Check the CloudFormation section in the AWS console to see the newly created cloud formations.
Remember, the AWS CDK will pick a random name extension based on the prefix you provided, so be sure to check the AWS console for the actual bucket name.
Worth a look: S3 Bucket Naming
Cloud Deployment
To deploy your S3 bucket to AWS Cloud using AWS CDK, run the deploy command. This is a crucial step to sync all the changes you made in your code to the AWS server infrastructure.
The deploy command is straightforward - simply run 'cdk deploy' and wait for the process to complete. As it can be seen above, the S3DeployStack was successfully deployed.
After deployment, navigate to the AWS S3 section to verify that your newly created bucket is there. The AWS CDK has picked a random name extension based on the prefix we provided, which in this case was S3 Deploy Stack.
A unique perspective: Azure Cdk
Cloud Deployment Overview
Cloud deployment is a crucial step in making your application or data accessible to a wider audience. You can deploy your application or data to the cloud using various tools and services.
AWS CDK is a great tool for deploying your application to the cloud. With AWS CDK, you can deploy your Amazon S3 Bucket to your AWS account by running the deploy command.
Cloud deployment involves setting up and configuring your application or data in the cloud. This can include setting up security measures to protect your data and configuring access controls to ensure only authorized users can access it.
To deploy your application or data to the cloud, you'll need to choose a cloud provider. AWS is a popular choice for cloud deployment, offering a wide range of services and tools to help you get started.
Deploying your application or data to the cloud can be done using various tools and services. AWS CDK is one such tool that can help you deploy your application or data to the cloud quickly and efficiently.
Related reading: Is S3 a Data Lake
How to Deploy
To deploy your AWS S3 bucket using AWS CDK Python, you'll need to run the cdk deploy command. This command syncs all the changes you made in your code to the AWS server infrastructure.
The final step of the deployment process is to verify that everything works as expected. To do this, navigate to the AWS S3 section and check if your newly created bucket is visible.
AWS CDK installed two new cloud formations during the deployment process. You can find these formations in the Cloud formation section of the AWS console.
If you previously had a CloudFormation deployed by AWS, you may encounter a potential error during the deployment process. This error can be silently suppressed in the AWS bootstrap command, and it will look like this: 'fail: No bucket named 'cdk-assets'. Is account bootstrapped?'.
To fix this error, simply navigate to the Cloud Formation section in AWS console and remove the previously created CDKToolkit. Once this is done, you can proceed with running the 'cdk bootstrap' command to re-create the CDKToolkit CloudFormation.
Take a look at this: Aws S3 Bucket Cloudformation
Frequently Asked Questions
Can you write the CDK code to launch an S3 bucket?
To launch an S3 bucket, add the S3 bucket and CORS code blocks to your CDK stack in the lib folder. This will create the necessary AWS resources for your S3 bucket.
What is the difference between stack and construct in AWS CDK?
In AWS CDK, a Stack represents the overall template, while a Construct represents individual AWS resources, such as Lambda functions or S3 buckets, that you want to create. Understanding the difference between these two concepts is key to building robust and scalable cloud applications.
What is the use of an S3 bucket in AWS?
An S3 bucket in AWS is a container that stores objects, such as files and metadata, in a centralized location. Understanding how to use S3 buckets effectively is key to leveraging the full potential of Amazon S3.
What does CDK stand for in AWS?
CDK stands for Cloud Development Kit, a software framework for defining cloud infrastructure as code. It's a key component of the AWS Cloud Development Kit.
Sources
- https://towardsthecloud.com/aws-cdk-s3-bucket
- https://binaryguy.tech/aws-cdk/create-s3-bucket-using-cdk-complete-guide/
- https://unbiased-coder.com/setup-aws-s3-bucket-cdk-python/
- https://dev.to/aws-builders/create-your-first-s3-bucket-using-aws-cdk-cj7
- https://stackoverflow.com/questions/60310575/how-to-add-s3-bucketpolicy-with-aws-cdk/62803892
Featured Images: pexels.com