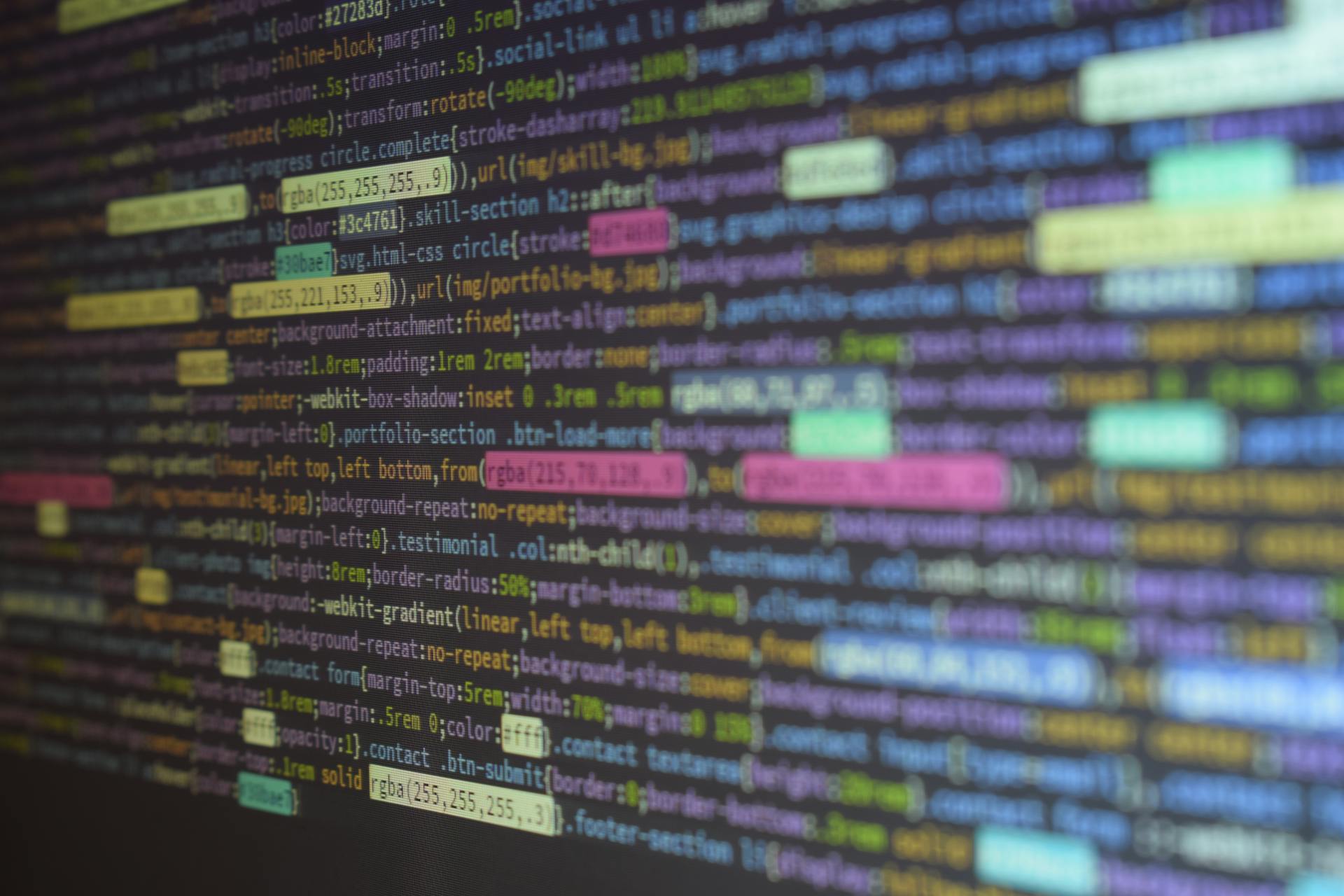
The Chrome Devtools Protocol is a game-changer for web automation and testing. It allows developers to automate browser interactions and test web applications programmatically.
With the Chrome Devtools Protocol, you can send commands to the browser to perform actions like navigating to a URL, clicking on an element, and filling out a form. This can be done using the Chrome Devtools Protocol's Command API.
The Protocol's Command API is based on the JSON-RPC 2.0 specification, which makes it easy to work with. It uses a simple request-response mechanism to send and receive data between the client and the browser.
By using the Chrome Devtools Protocol, you can automate tasks that would otherwise require manual intervention, saving you time and effort. For example, you can automate the process of testing a web application by sending commands to the browser to simulate user interactions.
APIs and Tools
You can use PyChromeDevTools to execute a page in Chrome headless mode and interact with Chrome DevTools APIs in Python. To get started, install PyChromeDevTools using pip3 install PyChromeDevTools.
Automation tools like Puppeteer, Playwright, and Selenium 4 leverage the Chrome DevTools Protocol to provide enhanced browser control and debugging capabilities.
Puppeteer is a browser automation tool that runs on NodeJS and uses the Chrome DevTools Protocol under the covers. It provides an API for creating automated tests and scripts that can be a great time-saver.
Here are some popular APIs and tools that interact with the Chrome DevTools Protocol:
- Puppeteer: A browser automation tool that runs on NodeJS and uses the Chrome DevTools Protocol.
- Playwright: A browser automation tool developed by Microsoft that also uses the Chrome DevTools Protocol.
- Selenium 4: A testing framework that supports the Chrome DevTools Protocol and provides access to its features.
- PyChromeDevTools: A Python library that provides wrappers for events, types, and commands specified in the Chrome DevTools Protocol.
Playwright
Playwright is a browser automation tool developed by Microsoft, initially by the same team that created Puppeteer at Google. It leverages Chrome DevTools Protocol to interact with Chromium-based browsers.
Playwright is similar to Puppeteer in many ways, offering high-level APIs that simplify browser automation tasks. It was released in July 2020 as a public version.
One exciting feature in Playwright is BrowserContexts, which allows you to operate multiple independent browser sessions. If a page opens another window, that page gets added to the parent context.
You can use CDPSession to directly interact with Chrome DevTools Protocol if you don't want to use the high-level methods provided by Playwright.
Here's a comparison of the two:
Playwright also offers a higher-level API, similar to the Chrome Debugger Extension API, which exposes a JSON message transport interface. This allows you to instrument the browser from its own extension and interact with the protocol.
Emulate Device
To emulate a device in Chrome using Chrome DevTools Protocol, you need to initialize a ChromeDriver instance.
You can initialize a ChromeDriver instance by creating a new instance of ChromeDriver.
The Chrome DevTools Protocol has a dedicated documentation page where they have documented all the available APIs, including Device Emulation.
Device Emulation allows you to change the page width, height, viewport, and more.
To perform Emulation in Chrome, you need to follow three steps: initialize the driver instance, create a DevTools instance, and create a session.
Here are the steps in a concise list:
- Initialize Chrome Driver
- Create DevTools Instance
- Create Session
Once you have created a session, you can change the screen size by using the setDeviceMetricsOverride method.
The setDeviceMetricsOverride method has several parameters, including width, height, deviceScaleFactor, and mobile, which are mandatory.
You can call the setDeviceMetricsOverride method using the send() command or the executeCdpCommand() method.
Using the send() command makes everything ready for you, but using the executeCdpCommand() method requires you to create a HashMap with all the required fields.
Here is an example of how to call the setDeviceMetricsOverride method using the executeCdpCommand() method:
driver.executeCdpCommand("Emulation.setDeviceMetricsOverride", Map.of(
"width", 500,
"height", 600,
"deviceScaleFactor", 2,
"mobile", true
));
This method calls the CDP command directly without using any Selenium wrapper, but Selenium recommends using the send() method over the executeCdpCommand() method due to the instability of the CDP.
Example: Retrieving Cookies
Retrieving cookies is a useful task that can be accomplished with the help of the Network domain in the PyChromeDevTools API. This can be done using the getCookies() command.
The command is used in conjunction with the ChromeInterface to extract the cookies associated with a page. In the example, the url "https://www.google.com" is navigated to and the cookies are retrieved after the page has finished loading.
Here's a step-by-step guide on how to retrieve cookies using the getCookies() command:
The getCookies() command returns a dictionary containing the cookies, which can be accessed using the "result" key. The cookies are then printed to the console using the print() function.
Object Parameters
Working with object parameters in the editor is a breeze. You can edit the values of these parameters directly, and this feature works for all types of nested parameters.
The editor lists the keys of the object, making it easy to access and modify the parameters. This is especially useful for complex commands that require multiple parameters.
Entering a command that accepts object parameters will trigger this feature, and you can start editing the values right away.
Working with the Protocol
You can interact with the Chrome DevTools Protocol using Selenium 4 with the send() and executeCDPCommand() commands. These commands are used for Chrome DevTools Protocol interaction, but there are differences between them.
To capture Console Logs, you need to enable logs and use the Log.EntryAdded event to iterate over the Console Logs. This can be done by sending the Log.enable() command and adding a listener to the Log.entryAdded() event.
To access the browser target, you can use the webSocketDebuggerUrl endpoint, which is exposed as /json/version. This endpoint is also written to both stderr and the DevToolsActivePort file in the browser profile folder.
Here are the main commands and APIs you can use to interact with the protocol:
- send()
- executeCDPCommand()
- Log.enable()
- Log.entryAdded()
- Browser.getVersion()
- Browser.getUserAgent()
How to Use
To use the Chrome DevTools Protocol in Selenium 4, you'll want to start with the basics.
You have two main options: send() and executeCDPCommand().
The send() method is a wrapper provided by Selenium, which internally makes a call to the Chrome DevTools Protocol. This is your first choice when interacting with dev tools.
The executeCDPCommand() method, on the other hand, is available directly from ChromiumDriver and bypasses Selenium's implementation of CDP commands.
To access Chrome Console Logs in Selenium, you need to enable logs and use the Log.EntryAdded event to iterate over the Console Logs.
Here's a step-by-step guide:
- Enable logs using devTool.send(Log.enable());
- Add a listener to the Log.entryAdded() event to capture console logs.
Here's an example of what the listener code might look like:
```html
devTool.addListener(Log.entryAdded(), logEntry -> {
System.out.println("Log Text : " + logEntry.getText());
System.out.println("URL : " + logEntry.getUrl().toString());
});
```
This will allow you to access Log Text, Log Level, Log Source, and URL.
Remember to add the navigation step to navigate to the website you want to capture console logs from.
Loading a Page Via CDP
Loading a page via CDP is a straightforward process that involves enabling the necessary domains, navigating to the page, and waiting for it to load successfully.
To start, you'll need to import the necessary modules, including PyChromeDevTools, and create a Chrome interface object.
Enabling the Network and Page domains is crucial for loading a page via CDP. This can be done using the enable() method of the Network and Page objects.
Navigating to a page can be done using the navigate() method of the Page object, which takes a URL as an argument. In Example 1, the page navigates to https://example.com/.
Waiting for the page to load can be done using the wait_event() method of the Chrome interface object. This method takes the event name and a timeout as arguments. In Example 1, the page waits for the Page.loadEventFired event to be sent.
The time it takes to load a page can be measured by recording the time before and after navigating to the page. In Example 1, the page loads in about 1.6 seconds.
Here's a summary of the steps involved in loading a page via CDP:
- Import the necessary modules
- Create a Chrome interface object
- Enable the Network and Page domains
- Navigate to the page using the navigate() method
- Wait for the page to load using the wait_event() method
- Measure the time it takes to load the page
Get /json/activate/{targetid}
To activate a target, you'll need to send a GET request to the /json/activate/{targetId} endpoint. This is a straightforward process that requires you to specify the target ID in the URL.
The response to this request will be a 200 status code with the message "Target activated", indicating that the target has been successfully activated. If the target is invalid, you'll receive a 404 status code with the message "No such target id: {targetId}".
Get /Json/Close/{TargetId}
The GET /json/close/{targetId} endpoint is used to close a target page. This is a simple yet effective way to manage your targets.
To use this endpoint, you'll need to provide a valid targetId. If the targetId is valid, the response will be 200 with the message "Target is closing".
If the targetId is invalid, the response will be 404 with an error message that includes the targetId. For example, "No such target id: {targetId}".
This endpoint is a useful tool for managing your targets, but make sure to use it correctly to avoid errors.
Monitoring
Monitoring the protocol is a powerful feature that lets you see all requests and responses as they happen. This is especially useful for understanding how the DevTools frontend uses the protocol.
To access the Protocol Monitor, click the gear icon in the top-right of the DevTools, select Experiments, and turn on "Protocol Monitor". Then, close and reopen DevTools.
You can view all requests and responses in the Protocol Monitor panel. The panel also allows you to issue your own commands using Protocol Monitor.
To issue a command, type it into the prompt at the bottom of the Protocol Monitor panel and press Enter. For example, you can type "Page.captureScreenshot" to capture a screenshot.
If a command requires parameters, provide them as JSON. For example, you can type {"cmd":"Page.captureScreenshot"",args":{"format": "jpeg"}} to capture a screenshot in JPEG format.
Alternatively, you can open the command editor by clicking the icon next to the command input. The editor creates a structured form based on the protocol definitions that allows you to edit parameters and view their documentation and types.
You can also execute commands from the DevTools console by opening devtools-on-devtools and using Main.MainImpl.sendOverProtocol() in the console.
Frequently Asked Questions
What is the DevTools protocol in Python?
The Chrome DevTools Protocol in Python is a library that provides remote control of a web browser through JSON messages over a WebSocket. PyCDP is a Python wrapper for this protocol, making it easier to interact with web browsers programmatically.
Sources
- https://reflect.run/articles/introduction-to-chrome-devtools-protocol/
- https://www.gkbrk.com/chrome-devtools-protocol
- https://rahulshettyacademy.com/blog/index.php/2021/11/04/selenium-4-feature-chrome-dev-tools-protocol/
- https://chromedevtools.github.io/devtools-protocol/
- https://developer.chrome.com/blog/cdp-command-editor
Featured Images: pexels.com