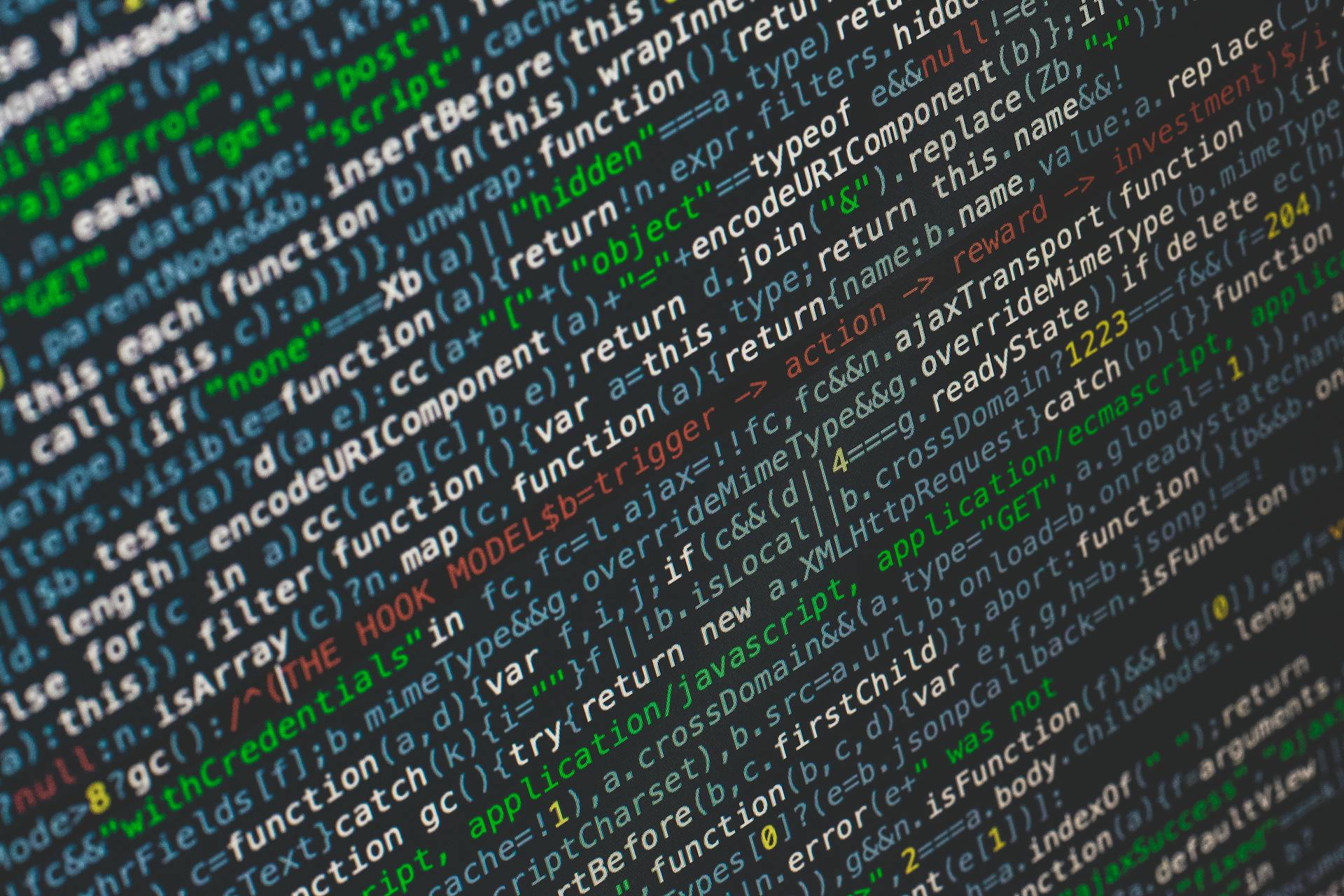
Coding JavaScript in HTML can be a bit tricky, but with the right steps, you can master it in no time. You can start by adding a script tag to your HTML file, which is the most common way to include JavaScript code.
This script tag should be placed inside the head or body of your HTML document, but it's generally recommended to put it at the end of the body tag to ensure that the JavaScript code runs after the HTML content has loaded.
To write JavaScript code, you'll need to use a text editor or an Integrated Development Environment (IDE) like Visual Studio Code. The JavaScript code should be placed inside the script tag, and it should be written in a way that is easy to read and understand, with proper indentation and syntax.
JavaScript code can be written in different ways, but one of the most common ways is by using functions, which are blocks of code that can be executed multiple times with different inputs.
Script Attributes
The async attribute loads the script asynchronously, meaning the script will be downloaded and executed as soon as it is available, without blocking the page. This is particularly useful for scripts that can be executed independently of the page's loading order.
The defer attribute delays the execution of the script until the entire HTML document has been parsed, which is useful for scripts that manipulate the DOM. This ensures that the script doesn't interfere with the page's loading process.
The async and defer attributes allow you to control how scripts are loaded and executed in relation to the page's loading process.
Async Attribute
The async attribute is a game-changer for web developers, allowing scripts to be downloaded and executed as soon as they are available, without blocking the page.
This attribute loads the script asynchronously, meaning it will be downloaded and executed in the background, not interfering with the user's experience.
A blog website can use asynchronous loading for a better user experience and faster page load.
The async attribute should only be used when the src attribute is present, i.e., only with external JavaScript files.
If you're not careful, using the async attribute without the src attribute can cause problems, so make sure to use them together.
This attribute allows downloading the script file in parallel to the parsing of the page and starts its execution of the script as soon as the download is completed.
Once the download of the script file is completed, it interrupts the parsing of the rest of the page till the execution of the script file is completed.
The async attribute is particularly useful for scripts that don't rely on the DOM being fully loaded, but it's essential to understand its implications on page parsing.
You might like: Html Code for Download Pdf File
Event Handlers
Event handlers are simple functions that can be called against any mouse or keyboard event.
Event handlers can contain any kind of logic, from a single line of code to thousands of lines. This flexibility makes them a powerful tool in JavaScript.
We can define event handlers in the header of a document, as seen in the example where a function named EventHandler() is written. This function can then be called in response to user interactions.
Event handlers can be used to respond to a wide range of events, including mouseovers and keyboard inputs.
Loading Strategies
Loading scripts asynchronously can significantly impact the performance and behavior of web pages. By using the async attribute, a script is fetched asynchronously and executed as soon as it's ready.
Synchronous loading, on the other hand, blocks page rendering until execution is complete. This can be avoided by using asynchronous or deferred loading strategies. Deferred loading delays script execution until the page's HTML content is fully loaded.
Here are some key differences between synchronous, asynchronous, and deferred loading:
The async attribute loads the script asynchronously, meaning the script will be downloaded and executed as soon as it is available, without blocking the page. This can be especially helpful for larger scripts, as it allows them to load in the background while the user continues to interact with the page.
Inline vs
As you're deciding how to load your JavaScript code, you'll want to consider the pros and cons of inline JavaScript. Inline JavaScript is useful for quick scripts and prototyping, making it a great choice for small projects or testing out new ideas.
You can write JavaScript code directly inside the HTML element using the onclick, onmouseover, or other event handler attributes, as shown in Example 1. This is a great way to get started with JavaScript quickly.
However, for larger projects, it's often better to link to external script files. External JavaScript is ideal for reusable and maintainable code, as it separates the code from the HTML and makes it easier to update.
Here's a quick rundown of the differences between inline and external JavaScript:
In general, it's a good idea to use inline JavaScript for small projects or testing out new ideas, and external JavaScript for larger projects where code reusability and maintainability are important.
Loading Strategies
Loading scripts asynchronously can significantly impact the performance and behavior of web pages. This approach ensures the script is fetched asynchronously and executed as soon as it's ready.
Asynchronous loading is a great strategy for a better user experience and faster page load, especially for blog websites. The async attribute is used to load scripts asynchronously, allowing the script to be downloaded and executed as soon as it's available.
Using the defer attribute delays script execution until the page's HTML content is fully loaded, which is particularly useful for scripts that manipulate the DOM.
Here are the key differences between synchronous, asynchronous, and deferred loading:
The async attribute should only be used with external JavaScript files, as it allows downloading the script file in parallel to the parsing of the page and starts its execution as soon as the download is completed.
File References
File references play a crucial role in loading external JavaScript files. You can reference an external script in three ways in JavaScript: by using a full URL, by using a file path, or by creating an external JavaScript file.
Expand your knowledge: External Javascript File Html
The src attribute is used to specify the location of the external script file used. The value of the attribute should always be wrapped inside double (“..”) or single (‘..’) quotes.
You can also reference an external script by creating a separate JavaScript file and calling it in your HTML file by using the src attribute of the script tag. This method allows us to use the same script file in multiple HTML documents by simply linking it using a single line.
Here are the three ways to reference an external script in JavaScript:
- By using a full URL:
- By using a file path:
- By creating an external JavaScript file:
Remember, the async attribute should only be used when the src attribute is present, and it allows downloading the script file in parallel to the parsing of the page.
Load Frameworks and Libraries
When building a portfolio website, it's essential to consider the types of files that need to be loaded. A portfolio website might include popular JavaScript frameworks like jQuery to extend functionality.
Curious to learn more? Check out: Free Basic Html Website Templates
You can also include other libraries like Bootstrap to enhance the user interface and experience.
Including these frameworks and libraries can significantly impact the loading speed of your website.
However, it's worth noting that not all libraries are created equal, and some may load more slowly than others.
For example, a website that includes a large library like jQuery might take longer to load than one that uses a smaller library like Lodash.
Frequently Asked Questions
How to write JavaScript code in HTML?
To write JavaScript code in HTML, simply wrap it in a