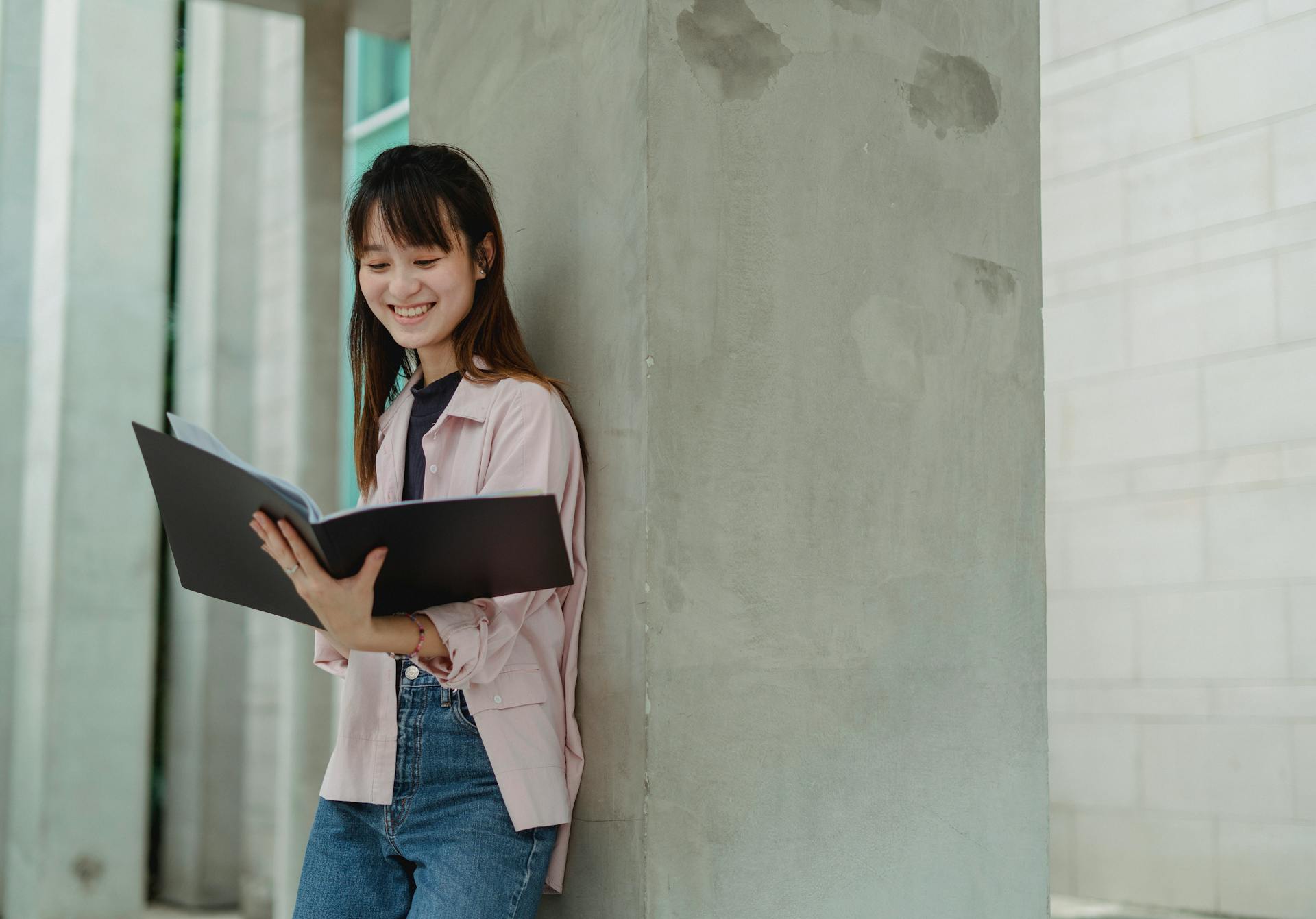
Creating a two-column design can be a great way to organize content, but it requires some careful planning.
To create a two-column design, you can use the CSS grid property, which allows you to divide a container into rows and columns.
The grid-template-columns property is used to define the number of columns and their widths. For example, grid-template-columns: 1fr 3fr; creates two columns where the first column takes up one fraction of the available space and the second column takes up three fractions.
The gap property is used to add space between the columns. For example, grid-gap: 1rem; adds a 1rem gap between the columns.
HTML Layout Basics
Creating a two-column layout with Flexbox requires a bit more work than Grid.
You have to apply some style rules to the child elements as well. The HTML part of the code is fairly simple, you basically need 2 div blocks, one for each column. The "container" div is merely a block enclosing both the two columns, and is useful if you want to apply certain styles that affect both columns as a unit.
The words "Navigation" and "Content here" are merely placeholders for the navigation side bar and main content. You should remove those words when you put your real content.
To add a third column, the HTML is easily updated to include another div block. This seamlessly nests itself in the row.
Additional reading: Web Page Navigation Design
Customizing the Layout
You can easily add more columns to your layout by updating the HTML and CSS, for example, adding a third column is as simple as updating the HTML.
The options for layouts are endless, you can create a double column layout by adding a .double-column class, or mix and match different column sizes like 60/40 or 10/10/10/10.
To make your layout more responsive, you can use media queries to adjust the flex properties, such as moving the flex: 1 and flex: 2 into a media query.
Changing Width Correctly
To change the width of your columns correctly, you need to make sure that the total width of both columns is 100% or less of the browser width. If the total width exceeds 100%, the browser will place one column below the other.
See what others are reading: Css Grid Equal Width Columns
The easiest way to avoid this issue is to use percentage values for the width of your columns, such as 20% and 80%, which add up to 100%. However, remember that percentage is a relative measurement tied to the visitor's browser window width, so what works on your system may not work on a system with a different window width.
To solve this problem, you can decrease the percentage used for the width of your columns until the browser renders it the way you want it to. Alternatively, you can create a nested DIV block within your columns and put your padding, margin, border, and real content there.
Here are the two solutions outlined:
- Decrease the percentage used for the width of your columns.
- Create a nested DIV block within your columns.
By using a nested DIV block, you can leave your percentages at 20% and 80% for your outer blocks without the nuisance of including paddings, margins, and borders in your calculations. This approach is preferred because it keeps the measurements for the outer ones unchanged, and the two column layout is preserved.
Moving Navigation to Right
Moving the navigation column to the right can be a game-changer for your website's layout.
You can see this code in action in the Two Column Layout Demo, where clicking the button switches the navigation column to the right.
To achieve this, add the following CSS code to your existing style sheet, placing it after the styles you created earlier.
This code is what makes the magic happen, allowing you to easily switch the navigation column to the right with a simple button click.
The Two Column Layout Demo showcases this feature, giving you a live example of how it works in practice.
On a similar theme: Edit Html Code
Layout Techniques
Creating a two-column layout is straightforward with CSS Grid Layout, and it's relatively simpler than Flex.
You can easily update the HTML to add a third column, which will nest itself in the row.
The options for column combinations are endless, and you can achieve any layout you want, from 1 x 100 to 10 x 1, 20 x 3, 30 x 1, and so on.
For your interest: Mac Os X Html Editor Wysiwyg
To add some responsiveness to your layout, you can use media queries, moving the flex values into a media query that depends on your application.
At a screen size of > 800px, you can define the layout, and the final codepen will show you the difference in the responsive layout when you click the 1x or 0.5x buttons in the lower right corner.
Flexbox is a powerful tool for creating row/column layouts, and it's easily adapted to a wide variety of uses, allowing a large amount of customizability.
Discover more: Css Media Selector
CSS Styling
CSS Styling is a crucial part of creating a 2-column layout. Line 7 sets the section tag as the Flexible box layout.
To prevent horizontal content overflow, the columns are wrapped on small screens, specifically those equal to or below 1024px. This is done using the Flex-related changes in the CSS style rules.
The flex shorthand property allows the columns to grow or shrink within the Flex container, depending on the available space. This is set in Line 16.
Here's an interesting read: Flex Grid Css
Website Code
Writing CSS code for a 2 column website is straightforward. You can see how it works out in practice on the Two Column Layout Demo.
To get started, you'll need to create two div blocks, one for each column. The HTML part of the code is fairly simple, and it's a good idea to remove placeholder text like "Navigation" and "Content here" to make room for your real content.
The container div is useful for applying styles to both columns as a unit. You can put your CSS code either in the style section of your web page or an external style sheet.
If this caught your attention, see: Web Designers Code Crossword Clue
Style Rules
Style Rules are a crucial part of CSS Styling, and they can make or break the layout of your website.
Setting a Flexible box layout is as simple as assigning the Flexible box layout to a section tag, like we did in our example.
You can use the flex shorthand property to allow columns to grow or shrink within a Flex container, depending on the available space.
Take a look at this: CSS Flexible Box Layout
Setting the column width to 100% and the flex value to auto allows columns to stack on each other on small screens.
Making sure to wrap columns on small screens prevents horizontal content overflow and keeps your layout clean.
By setting a breakpoint, like 1024px, you can control when certain styles take effect, like when columns start stacking on each other.
This is especially useful when designing for different screen sizes and devices, like desktops, laptops, and mobile phones.
For another approach, see: Small Business Web Page Design
Grid Layout
Grid Layout is a powerful tool for creating two-column designs. It's straightforward and relatively simpler than Flex, making it a great choice for many projects.
To create a two-column grid, you can use the grid-template-columns property, which sets the grid to have two columns, each 50% of the width of the container. This is achieved using the value 50% 50%.
However, a neater way to achieve the same thing is to use grid-template-columns: 1fr 1fr;, which has the same effect as 50% 50% but is a better pattern for when you start moving to other divisions. This is because it allows for more flexibility and ease of use.
Grid items can be spaced using the gap property, which is a shortcut that sets both the horizontal and vertical gap between the grid items to a specified value. For example, gap: 10px sets a 10px gap between the grid items.
To create a grid layout, you need to switch the element into CSS grid mode using the display: grid property. Every direct descendant of that element becomes a grid item.
Grid lines can be used to specify regions of the grid in more complex layouts, but they're not necessary for simple layouts like the one we're discussing. The grid lines are represented by numbers, which can be used to define different regions of the grid.
You might like: Css Truncate after 2 Lines
Running the Website
Running the Website is a breeze. Open the website (index.html) in your web browser, and you should see two columns laid out side-by-side with a purple background color on each.
The layout should look great on a desktop screen, but things change when you resize your browser below the 1024px breakpoint.
Frequently Asked Questions
How to split text into 2 columns in CSS?
To split text into 2 columns in CSS, create a container element and use the CSS property column-count with a value of 2. Adjusting this value allows you to easily change the number of columns.
How to put two sections side by side in CSS?
To put two sections side by side in CSS, set the parent container's display property to inline-flex or inline-grid. This simple adjustment will align your child elements horizontally.
Sources
- https://w3things.com/blog/two-column-layout-html/
- https://til.simonwillison.net/css/simple-two-column-grid
- https://www.thesitewizard.com/css/design-2-column-layout.shtml
- https://dev.to/drews256/ridiculously-easy-row-and-column-layouts-with-flexbox-1k01
- https://blog.logrocket.com/css-flexbox-vs-css-grid/
Featured Images: pexels.com