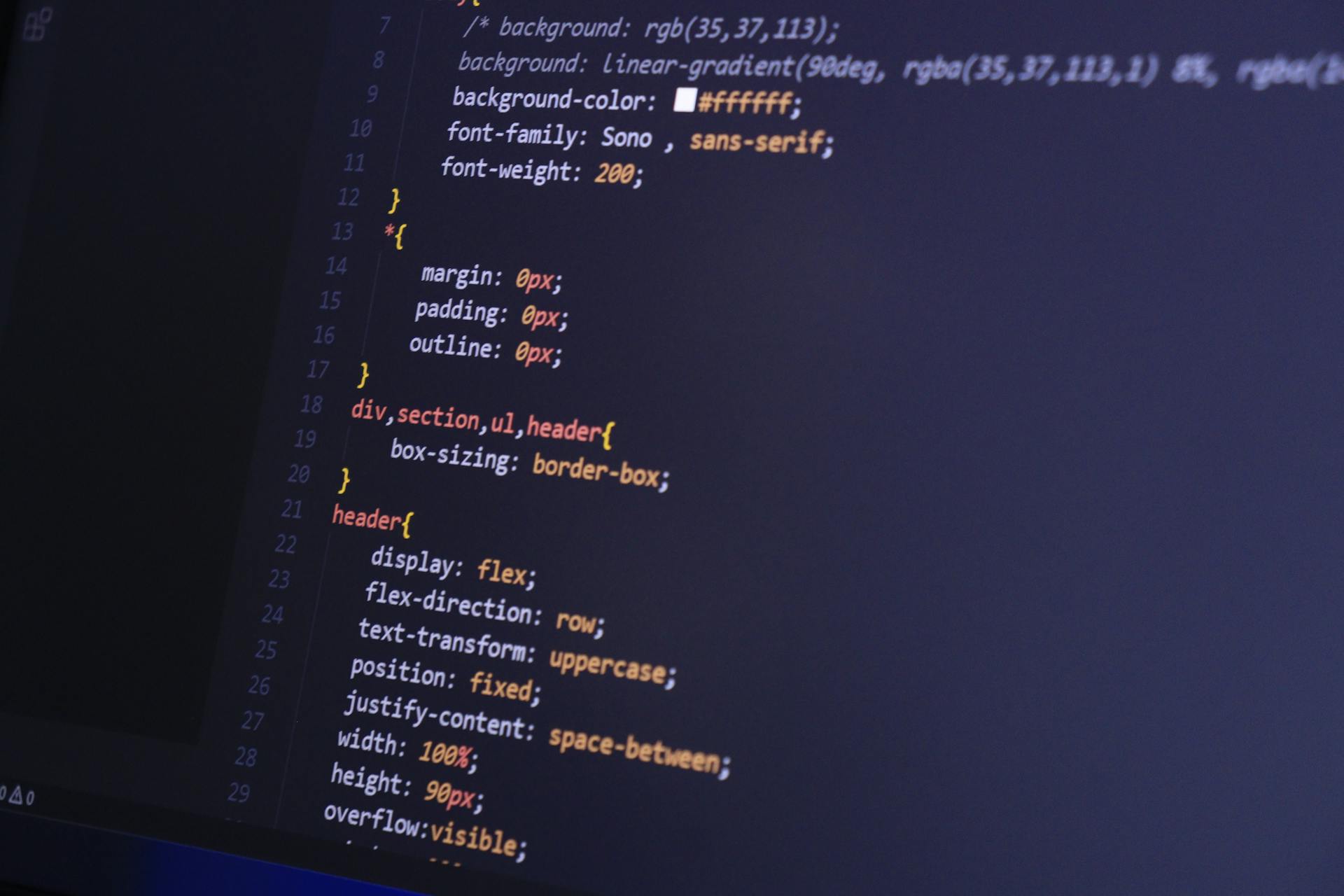
CSS class extend is a powerful feature that allows you to create a new class by extending an existing one, inheriting all its properties and adding new ones.
This approach helps to avoid code duplication and makes it easier to manage complex CSS styles.
By extending a class, you can create a new class with a subset of the original class's properties, or even override some of them.
For example, if you have a class called `.button` with some basic styles, you can extend it to create a new class called `.primary-button` that inherits the original styles and adds some additional ones.
In the example from the article, `.primary-button` extends `.button` and adds a new background color and padding.
What is CSS Class Extend
CSS class extend is a method that allows you to inherit the properties of a base class to its modifiers. This way, you can use them all at once.
You can use the @extend function in Sass to do so, which will turn into a CSS snippet that combines the base class with its modifiers. Our HTML would look like this:
You'll end up with a single class with an explicit name, making your code more readable. You can still use the base class alone, but if you need a variation, you only need to append the modifier part on it instead of chaining a new class.
This method results in a clutter-free and lighter HTML, but it can also lead to a heavier CSS file. Every time you use @extend, the class definition is moved to the top and added to a list of selectors sharing the same ruleset.
Benefits and Drawbacks
Chaining classes keeps your CSS codebase clean and non-repetitive, making it easy to read and understand.
Separate modifiers are great for representing state, making it easier for JavaScript engineers to add and remove classes.
Chaining classes can save you a lot of time on large projects, especially when removing components only requires deleting the HTML.
On the other hand, extending classes results in a heavier CSS file, which can lead to weird style overrides and more generated code.
Extending classes can also make it harder to maintain and results in more code, especially when creating new combinations of classes.
Expand your knowledge: Css Selector Two Classes
Pros of Extending
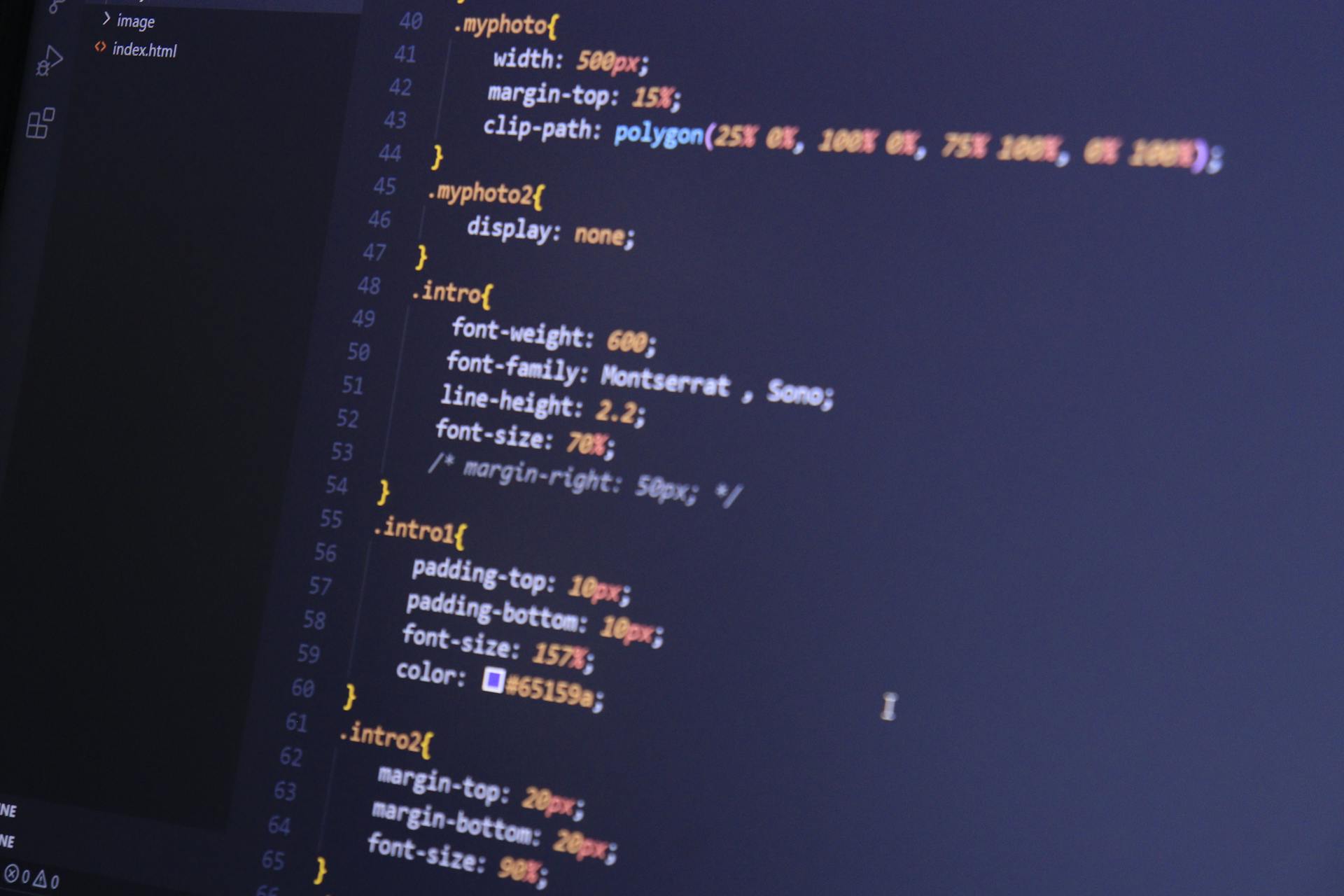
Extending your CSS classes can be a game-changer for your coding workflow. Here are some of the key benefits you can expect.
Applying a single class is faster than typing out a long list of chained classes. This means you can save time on repetitive tasks.
Less verbose HTML is a major advantage of extending your CSS classes. By reducing the number of chained classes, your HTML becomes easier to read and understand.
Reducing the number of chained classes can also make your HTML quicker to write. This is because you don't have to type out a long list of classes every time you need to apply a specific style.
- Less verbose HTML
- Quicker to write
Cons of Extending
Extending classes can lead to duplicated styles in your generated CSS, bloating your stylesheets. This can make your code harder to maintain and slower to load.
Less control is another con of extending classes. The @extend directive can be tricky to control, leading to unintended style inheritance.
See what others are reading: Css Class Inheritance
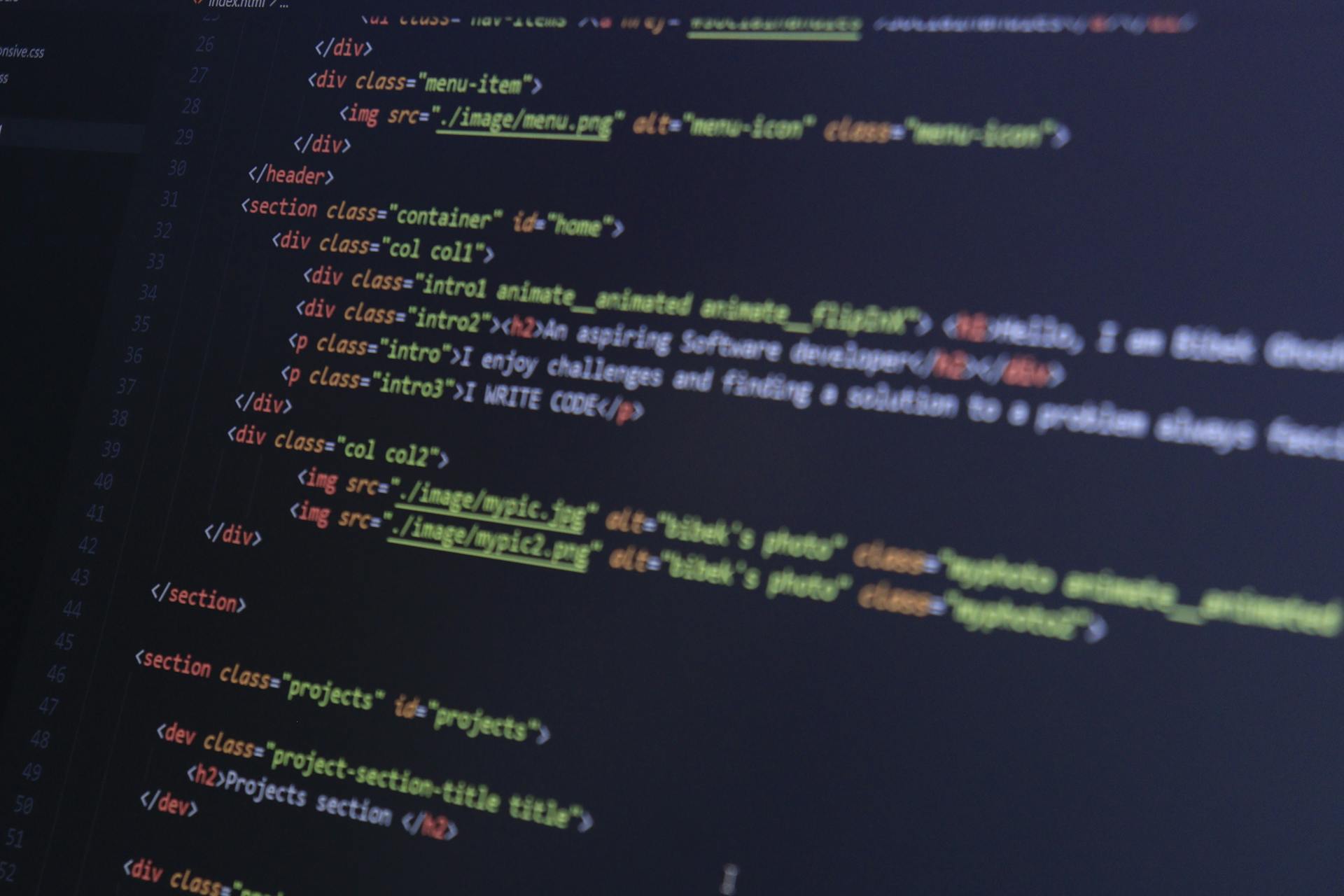
If you need to apply multiple modifiers to an element, you'll need to create new classes in your CSS instead of simply chaining existing ones. This can be a hassle, especially if you have many modifiers.
Here are some specific issues to watch out for:
- Heavier CSS
- Less control
- Inflexible
Your uncompressed file size will increase when the selector using @extend is longer than the properties it avoids repeating. This is because of the extra code generated by the @extend directive.
Selector precedence can break when @extend regroups rules, changing source order. This can make it harder to predict how styles will be applied.
Extension Scope
Extension Scope is a crucial consideration when working with @extend rules.
Extensions only affect style rules written in upstream modules, which are loaded by the stylesheet using the @use rule or the @forward rule, and their dependencies.
This predictable behavior helps ensure that @extend rules have the desired impact.
Extensions aren't scoped at all if you're using the @import rule, affecting every stylesheet you import and their dependencies.
This can lead to unintended consequences, making @use a safer choice.
See what others are reading: Css Stylesheet Import
Mandatory and Optional
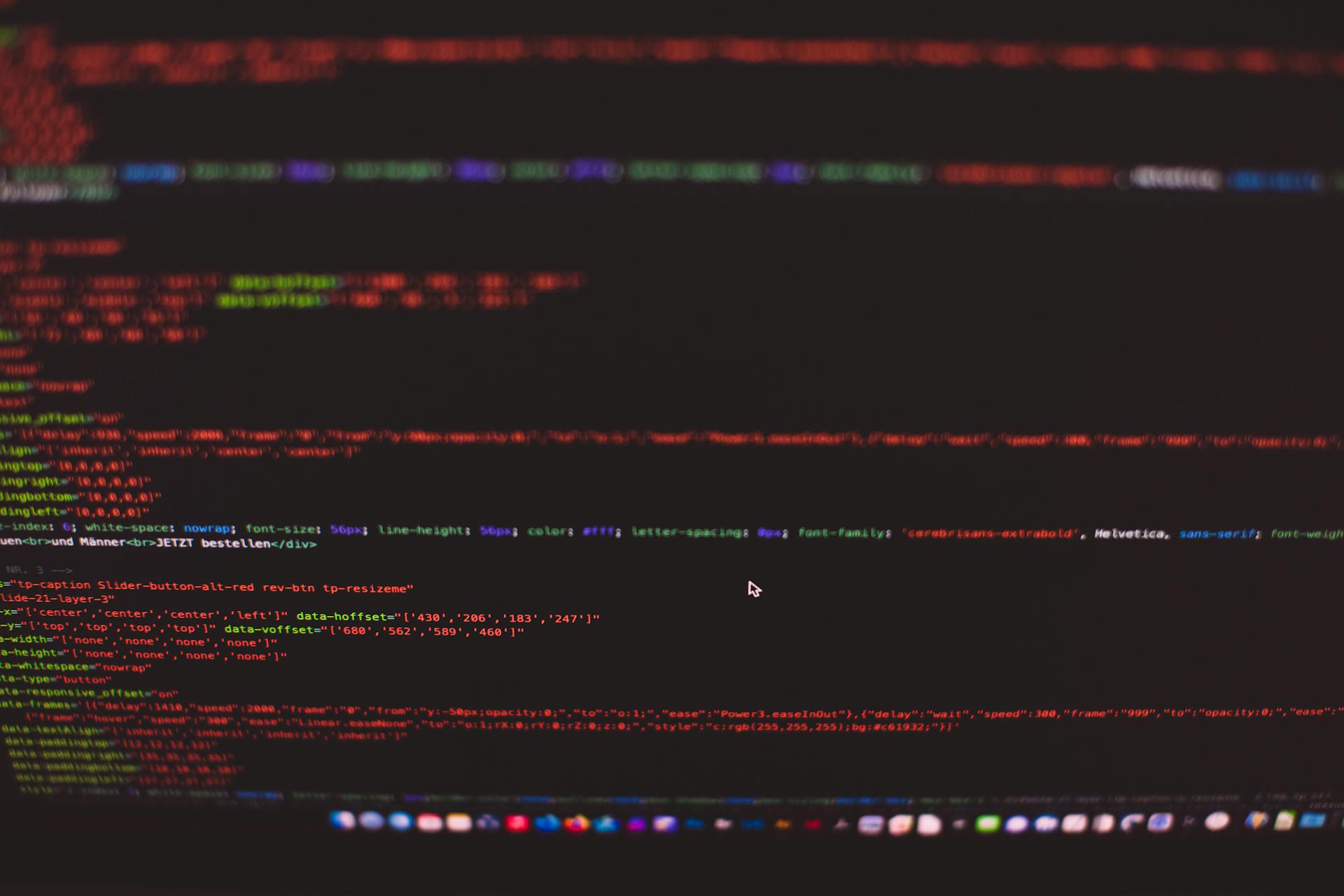
Sass will produce an error if an @extend doesn't match any selectors in the stylesheet.
This is because mandatory @extends require the extended selector to exist, helping protect from typos or renaming a selector without renaming the selectors that inherit from it.
If you want the @extend to do nothing if the extended selector doesn’t exist, add !optional to the end of the @extend directive.
Mandatory @extends help ensure you don't accidentally remove a selector without updating the @extends that rely on it.
How it Works
Sass does intelligent unification when extending selectors, which means it never generates selectors that can't possibly match any elements.
It ensures that complex selectors are interleaved so that they work no matter which order the HTML elements are nested.
Sass trims redundant selectors as much as possible, while still ensuring that the specificity is greater than or equal to that of the extender.
Here are the benefits of Sass's intelligent unification:
- It never generates selectors like #main#footer that can’t possibly match any elements.
- It ensures that complex selectors are interleaved so that they work no matter which order the HTML elements are nested.
- It trims redundant selectors as much as possible, while still ensuring that the specificity is greater than or equal to that of the extender.
- It knows when one selector matches everything another does, and can combine them together.
- It intelligently handles combinators, universal selectors, and pseudo-classes that contain selectors.
Sass Syntax and Usage
You can extend CSS classes using a preprocessor like Sass, which allows you to inherit styles from one class to another.
With Sass, you create a base class with common styles, then use @extend to inherit those styles in modifier classes. This approach is an alternative to chaining, which can result in a lot of repeated HTML code.
By using @extend, you can inherit styles from the base class, so you only need to apply a single class in your HTML, like .btn-primary in the button example.
If this caught your attention, see: Html Css Grid
Sass Syntax
Sass Syntax allows selectors to be used on their own in style rules, and it knows to extend everywhere the selector is used.
This ensures that your elements are styled exactly as if they matched the extended selector. The extended selector can be used throughout your code, and Sass will take care of the rest.
Selectors are a fundamental part of Sass syntax, and understanding how they work is key to writing efficient and effective code. Sass extends selectors everywhere they are used, making it easy to apply styles to multiple elements at once.
By using Sass syntax, you can write more concise and readable code, which can save you time and reduce errors.
Placeholder Selectors
Placeholder selectors are a special type of class selector that starts with a percent sign instead of a dot.
These selectors look like they're meant to be used as classes, but they don't get included in the CSS output unless they're extended by another selector.
You can use placeholder selectors to write style rules that are meant to be extended later, and any selectors that include placeholders won't be included in the CSS output.
This means you can keep your code organized and focused on the rules that are actually being applied, without cluttering up the output with unnecessary selectors.
Extends or Mixins?
Extends and mixins are both powerful tools in Sass, but they serve different purposes. Extends are best used when expressing a relationship between semantic classes, like when an element with class .error--serious is an error and should extend the .error class.
A good rule of thumb is to choose the feature that makes the most sense for your use-case, not the one that generates the least CSS. Mixins may produce more CSS than extends, but most web servers compress the CSS they serve, which means the difference is likely to be negligible.
In general, mixins are necessary when you need to configure styles using arguments. However, if you're dealing with non-semantic collections of styles, writing a mixin can avoid cascade headaches and make it easier to configure down the line.
Here are some key differences between extends and mixins:
Whether to use extends or mixins depends on your project's size and complexity, as well as your team's preferences. For large-scale projects, chained classes may be worth the extra HTML, while for small, self-contained components, extended classes can help reduce duplication.
Readers also liked: Tailwind Css Class
Example with @extend
You can use @extend to inherit styles from one class to another in Sass. This makes your code more efficient and easier to maintain.
With @extend, you can create a base class with common styles, then use it to inherit those styles in modifier classes. This approach is an alternative to chaining.
By using @extend, you can apply a single class in your HTML, like .btn, and still get the same result as if you had applied multiple classes.
For example, you can create a base class .btn with common styles, then use @extend to inherit those styles in .btn-primary and .btn-outline classes.
The output CSS will be the same as if you had written separate style rules for each class.
Limitations and Considerations
CSS class extend has its limitations, and it's essential to consider them when deciding whether to use this technique.
The primary limitation of CSS class extend is that it can lead to complex and hard-to-maintain stylesheets, as seen in the example where the `.button` class is extended by multiple other classes.
Another consideration is that CSS class extend can make it difficult to override styles, as demonstrated in the example where the `.button` class is overridden by a more specific selector.
This can result in unexpected behavior and make debugging more challenging.
Disallowed Selectors Permalink
You can't @extend compound selectors like .message.info or .main .info, as it's not clear what elements would be styled. This behavior is now deprecated and will be removed in future versions.
Only simple selectors like .info or a can be extended. This is because extending compound selectors would be the same as matching both individual selectors.
You can't @extend an outer selector from within @media, or group selectors with and without media queries. This means you can't @extend a selector that's inside a media query from outside the query.
Here are some examples of disallowed selectors:
- .message.info
- .main .info
- @extend .message from within @media
These selectors are disallowed because they don't follow the definition of @extend, which is to style elements as though they matched the extended selector.
11. @extend Unpredictable
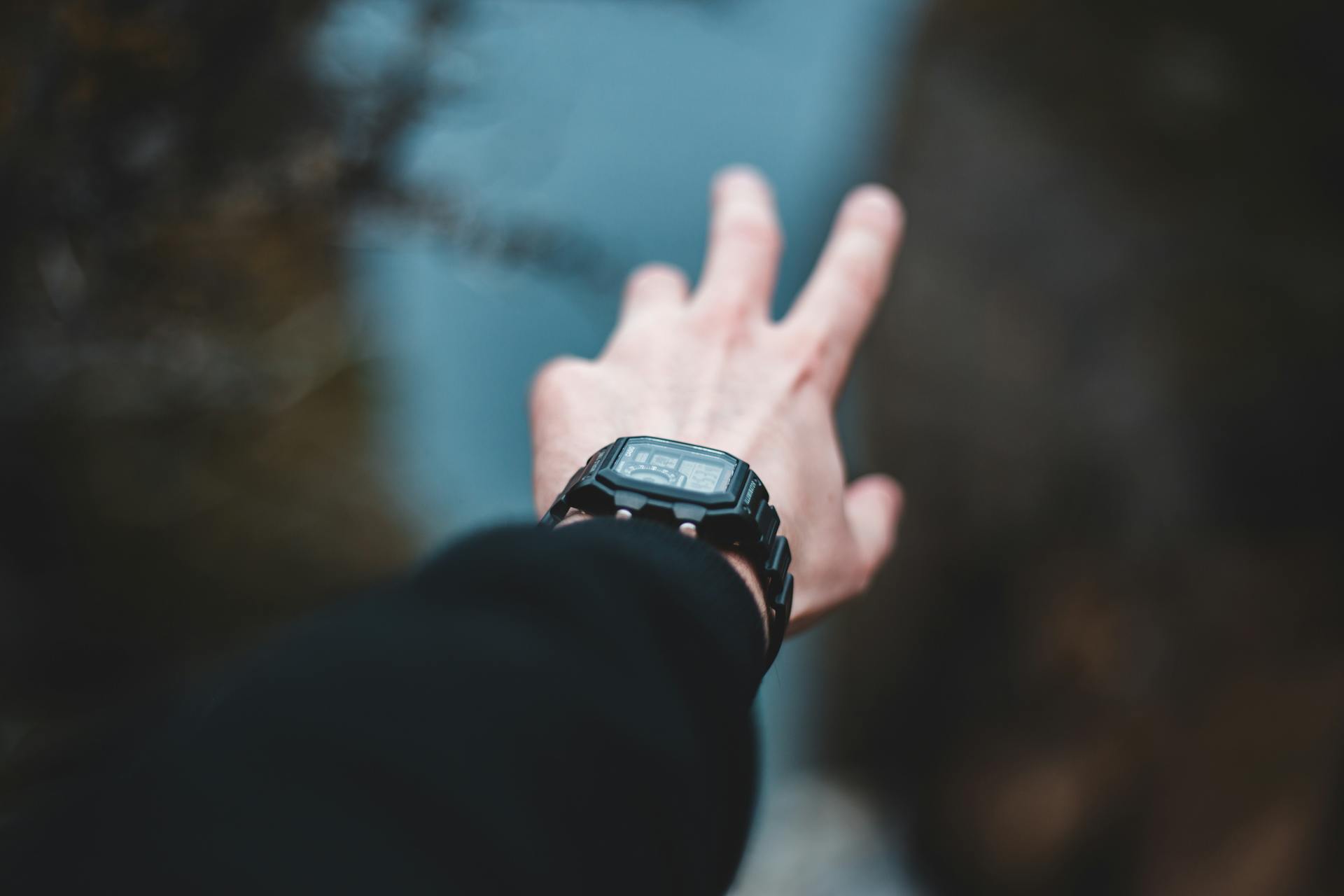
@extend can be unpredictable, as seen in the example where it extends every instance of the selector it matches, resulting in unexpected CSS output.
Harry Roberts' example illustrates this issue clearly, showing that @extend will extend every instance of the selector, even if you don't want it to.
The CSS output can get complicated, especially with more complex @extend usage, making it difficult to notice the issue unless you inspect the output carefully.
You might expect a specific CSS output, but @extend can surprise you with its behavior, as demonstrated in the example where the .footer .bar selector is unexpectedly added.
7. Weakens Gzip Compression
Using @extend can weaken gzip compression. This is because gzip compresses each unique grouped selector poorly.
Gzip compression works well with repeated properties, which is exactly what @mixin provides. @mixin makes properties appear many times, and selectors appear once.
In contrast, @extend makes properties appear once, and selectors appear many times. This can lead to inefficient compression.
Sources
- https://stackoverflow.com/questions/41635618/how-to-extend-css-class-with-another-style
- https://frontstuff.io/should-you-chain-or-extend-css-classes
- https://sass-lang.com/documentation/at-rules/extend/
- https://www.bomberbot.com/css/how-to-decide-whether-you-should-chain-or-extend-css-classes/
- https://manuals.gravitydept.com/code/css/extends
Featured Images: pexels.com