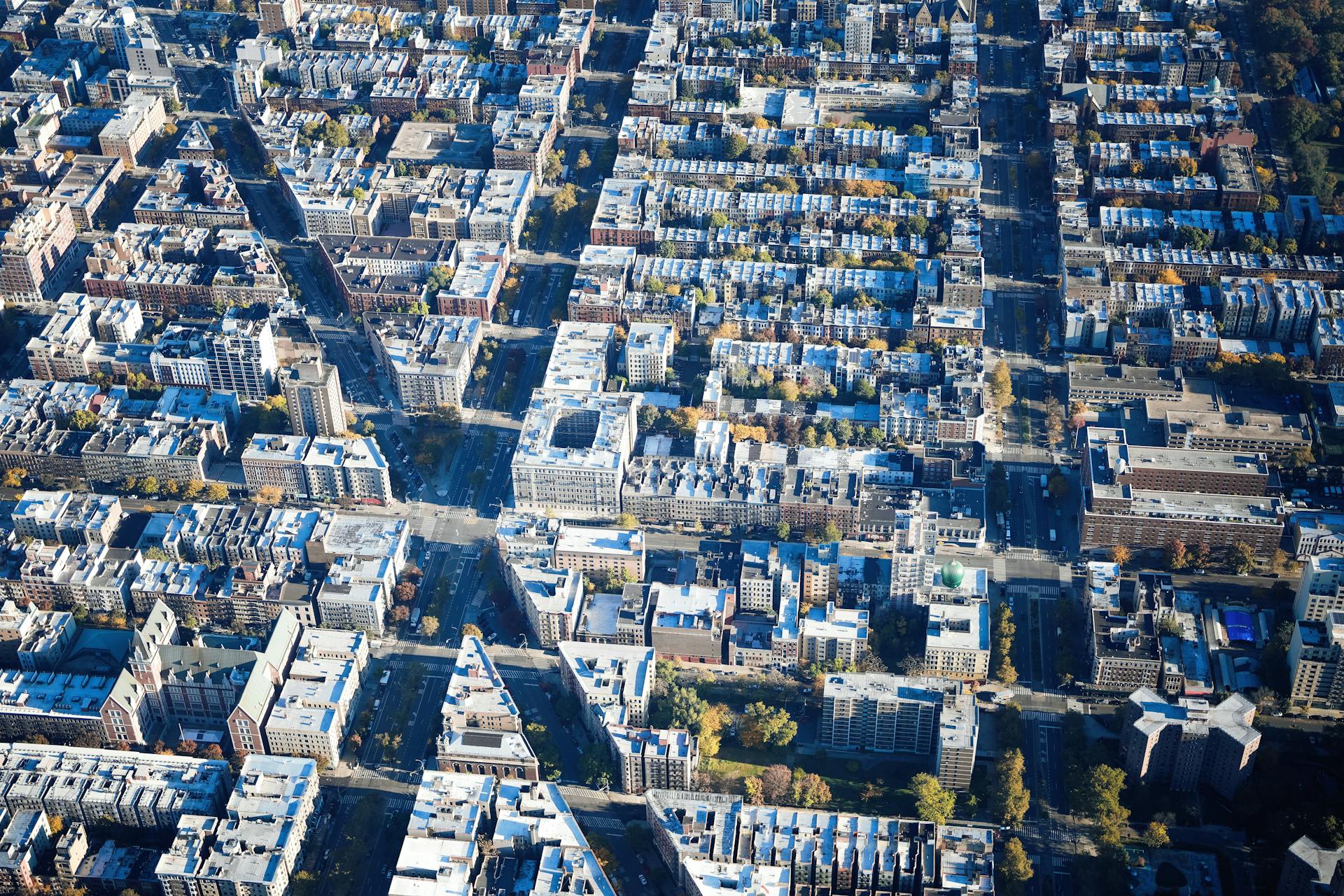
Using CSS Grid to create a table can be a powerful way to layout content, especially when you need to display complex data.
Grid templates are a great way to define the structure of your grid, and they can be used to create a table-like layout.
To create a grid template, you can use the grid-template-columns and grid-template-rows properties. For example, in the "Basic Grid" example, we used grid-template-columns: repeat(3, 1fr) to create a grid with three equal-width columns.
This approach can be particularly useful for creating responsive layouts that adapt to different screen sizes.
Check this out: Css Grid Templates
CSS Grid Basics
Most browsers shipped native support for CSS Grid by March 2017, including Chrome, Firefox, Safari, and Opera.
You can start building with grid now that most browsers have native support. Internet Explorer 10 and 11 do support it, but it's an old implementation with an outdated syntax.
To get started with CSS Grid, you need to define a container element as a grid with display: grid.
Grid items can be placed in any order, which makes it super easy to rearrange your grid with media queries.
Grid Container
The grid container is the backbone of a CSS grid table. It's the element on which display: grid is applied.
In this example, container is the grid container. The grid container is the direct parent of all the grid items.
Grid containers are essential for creating a grid table structure. They allow you to define the grid layout and position grid items within it.
The grid container is where you apply display: grid, which enables the grid layout. This is what makes the grid items appear as a grid table.
Discover more: Css Grid Align Items
Grid Layout
The CSS Grid Layout is a two-dimensional grid-based layout system with rows and columns. It's useful in creating more complex and organized layouts.
To define a grid container, you'll need to pass a display: grid property to your element. This is a simple yet effective way to create a grid layout.
The "Holy Grail" layout is a great example of how CSS Grid can be used to create a complex layout. It involves defining a grid template with specific areas for the header, footer, and content.
Here's a breakdown of the grid template areas:
Fluid columns are also possible with CSS Grid, and they can break into more or less columns as space is available. No media queries are needed to achieve this effect.
Column\nRow
CSS Grid Layout is all about creating complex and organized layouts, and one of the key features is the grid system itself.
To define a grid container, you simply need to pass a display: grid property to your element. This is the foundation of building a grid layout.
Grid columns and rows are defined by the grid-column and grid-row properties, which are shorthand for grid-column-start + grid-column-end and grid-row-start + grid-row-end, respectively.
These properties accept all the same values as the longhand versions, including span, which allows you to specify how many tracks an item will span.
If no end line value is declared, the item will span 1 track by default.
Implicit tracks can be created when you reference lines that don't exist, but you can use grid-auto-columns and grid-auto-rows to specify the widths of these tracks.
These properties can take a track-size value, which can be a length, a percentage, or a fraction of the free space in the grid using the fr unit.
Here are some examples of track-size values:
- Length: 10px, 20%
- Percentage: 50%, 75%
- Fraction: 1fr, 2fr
Grid Properties
Grid Properties are what make the magic happen. The grid properties listed for grid items include grid-column-start, grid-column-end, grid-row-start, grid-row-end, grid-column, grid-row, grid-area, justify-self, align-self, and place-self.
These properties can be used to define the layout of grid items, but it's worth noting that other properties like float, display: inline-block, display: table-cell, vertical-align, and column-* have no effect on a grid item.
To create a grid container, you'll need to use the display property, which defines the element as a grid container and establishes a new grid formatting context for its contents. You can choose between grid, which generates a block-level grid, or inline-grid, which generates an inline-level grid.
For more insights, see: Grid Row Css
Important Terminology
Grid terminology can be confusing, but don't worry, there aren't many terms to memorize. The Grid specification defines a few key terms that are essential to understanding Grid concepts.
The Grid specification defines a few key terms that are essential to understanding Grid concepts. These terms include grid-column-start, grid-column-end, grid-row-start, and grid-row-end.
If this caught your attention, see: Css Grid Two Columns
Grid items can span multiple grid tracks, and the grid-column-start and grid-row-start properties determine the line where the item begins. The grid-column-end and grid-row-end properties determine the line where the item ends.
Here's a summary of the grid properties that determine a grid item's location:
Items can overlap each other, and you can use the z-index property to control their stacking order.
Parent Properties
When setting up a grid container, you'll want to consider the properties that define its structure. The display property should be set to 'grid' to enable grid layout.
The grid-template-columns, grid-template-rows, and grid-template-areas properties allow you to define the grid's structure by specifying the number and arrangement of columns, rows, and areas. You can also use the grid-template shorthand property to combine these properties.
The grid-column-gap, grid-row-gap, and grid-gap properties control the spacing between grid tracks. Setting a gap will add space between the items in your grid.
Explore further: Css Grid Template Areas
The justify-items, align-items, and place-items properties determine how items are aligned within their grid cells. You can use these properties to center, stretch, or baseline-align items.
The justify-content and align-content properties control how the grid's content is distributed within the grid container. You can use these properties to justify or align the content in various ways.
The place-content property is a shorthand for the justify-content and align-content properties, allowing you to set both properties at once.
The grid-auto-columns, grid-auto-rows, and grid-auto-flow properties define the implicit grid structure when no explicit grid is defined. The grid property is a shorthand for all the grid properties, making it a convenient way to set multiple properties at once.
Here are the parent properties listed out for easy reference:
- display
- grid-template-columns
- grid-template-rows
- grid-template-areas
- grid-template
- grid-column-gap
- grid-row-gap
- grid-gap
- justify-items
- align-items
- place-items
- justify-content
- align-content
- place-content
- grid-auto-columns
- grid-auto-rows
- grid-auto-flow
- grid
Repeat() Function
The repeat() function is a game-changer for grid properties. It can save some typing by repeating a value for a specified number of times.
You can get creative with repeat() by combining it with keywords. For example, using auto-fill will fit as many possible columns as possible on a row, even if they are empty.
Broaden your view: Css Grid Repeat
If you want to fit whatever columns there are into the space, auto-fit is the way to go. It prefers expanding columns to fill space rather than empty columns.
Here's a quick rundown of the options:
- auto-fill: Fit as many possible columns as possible on a row, even if they are empty.
- auto-fit: Fit whatever columns there are into the space. Prefer expanding columns to fill space rather than empty columns.
Grid Units and Functions
Fractional units in CSS Grid, like 1fr, mean "portion of the remaining space" and are very flexible.
You'll often use them in combination with other units, making them much friendlier to work with.
The min() and max() functions can be used with fractional units, which is really powerful.
This combination is behind one of the most famous snippets in all of CSS Grid and is considered an all-time great CSS trick.
Grid Alignment and Justification
Grid alignment and justification are crucial aspects of CSS Grid. The `justify-items` property aligns grid items along the inline (row) axis, and its values include `start`, `end`, `center`, and `stretch`.
You can use `justify-items` to set alignment for all grid items inside a container. The `justify-self` property, on the other hand, aligns a grid item inside a single cell along the inline (row) axis.
Here are the values for `justify-self`: start – aligns the grid item to be flush with the start edge of the cellend – aligns the grid item to be flush with the end edge of the cellcenter – aligns the grid item in the center of the cellstretch – fills the whole width of the cell (this is the default)
On a similar theme: Css Grid Justify Content
Justify-Content
Justify-content is a property that aligns the grid along the inline (row) axis, and it's used when the total size of the grid is less than the size of its grid container. This can happen when all grid items are sized with non-flexible units like pixels.
To align the grid, you can use the following values: start, end, center, stretch, space-around, space-between, or space-evenly. The default value is stretch, which resizes the grid items to allow the grid to fill the full width of the grid container.
If you want to align the grid to the start edge of the grid container, use the start value. Similarly, if you want to align the grid to the end edge, use the end value. For a centered alignment, use the center value.
Here are the options for justify-content in a concise table:
Justify-content is a powerful property that helps you manage the alignment of your grid, and by understanding its options, you can create more visually appealing and functional layouts.
Align-Items
Align-items is a crucial property in grid alignment, and it's used to align grid items along the block (column) axis. This means it's the opposite of justify-items, which aligns grid items along the inline (row) axis.
The default value for align-items is stretch, which fills the whole height of the cell. However, you can also use start, end, center, or baseline to achieve different alignments.
For example, if you want to align items to be flush with the start edge of their cell, you can use align-items: start. Similarly, if you want to align items to be flush with the end edge of their cell, you can use align-items: end.
Here's a summary of the different values you can use for align-items:
This property can also be set on individual grid items via the align-self property, which aligns a grid item inside a cell along the block (column) axis.
Sources
- https://www.freecodecamp.org/news/https-medium-com-nakayama-shingo-creating-responsive-tables-with-pure-css-using-the-grid-layout-module-8e0ea8f03e83/
- https://matthewjamestaylor.com/3-column-layouts
- https://www.simplilearn.com/tutorials/css-tutorial/css-grid-vs-flexbox
- https://en.wikipedia.org/wiki/CSS_grid_layout
- https://css-tricks.com/snippets/css/complete-guide-grid/
Featured Images: pexels.com