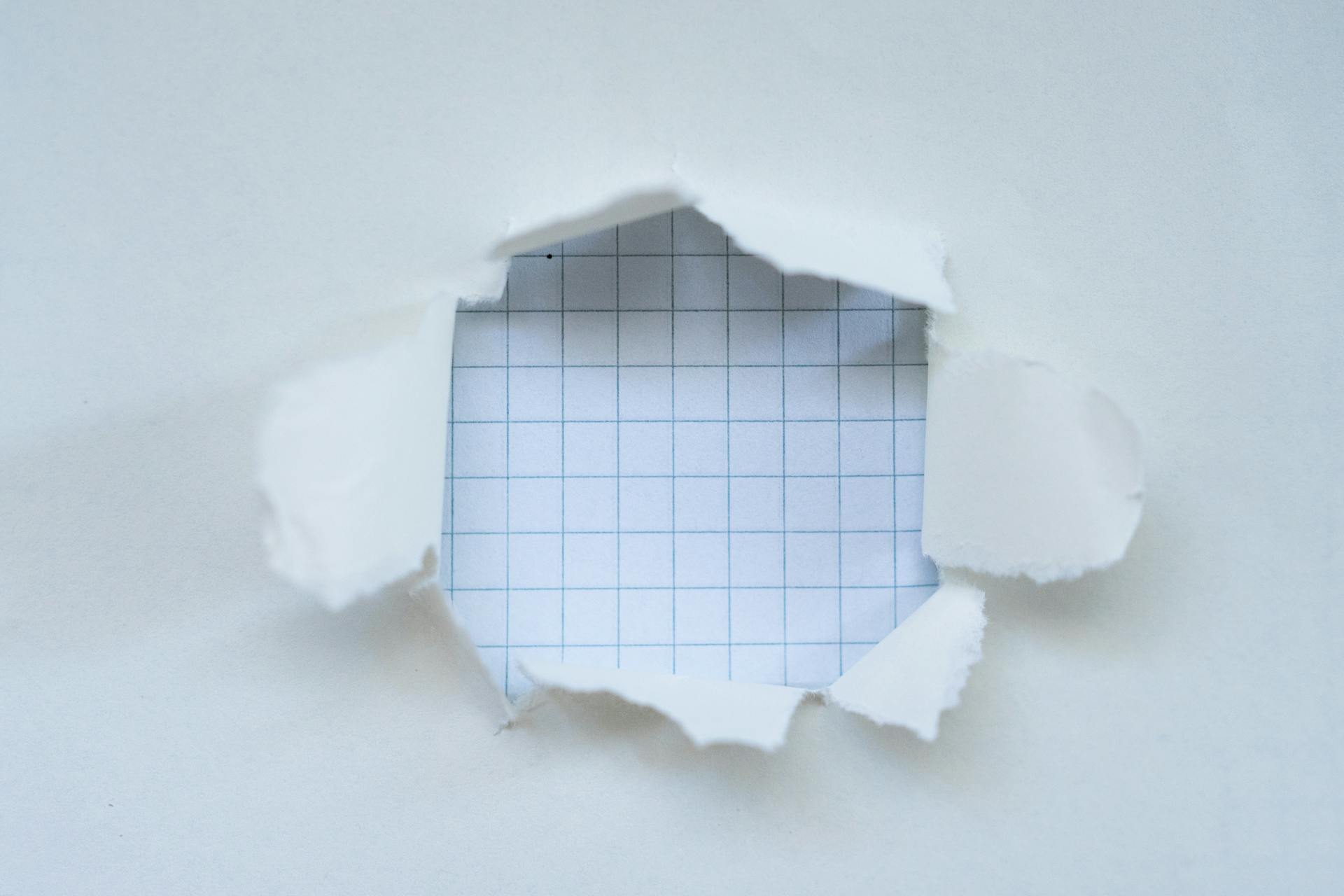
CSS Grid Template Areas are a powerful tool for creating complex layouts. A grid container can have multiple grid template areas, which are essentially named regions within the grid.
Grid template areas are defined using the grid-template-areas property, which takes a string of names separated by spaces. Each name represents a grid cell.
To create a grid with multiple template areas, you need to define the grid-template-areas property in the grid container. The example below shows how to create a grid with two template areas: a header and a footer.
The example code defines the grid-template-areas property with two names: "header" and "footer". This creates two separate regions within the grid.
A unique perspective: Grid Container Css
Parent Properties
Parent properties are essential in CSS Grid Template Areas, and one of the key properties is grid-template-areas.
Grid-template-areas is a shorthand property that allows you to define the layout of your grid by specifying the areas where content should be placed.
On a similar theme: Css Grid Properties
You can define multiple areas in a grid-template-areas property, separated by a space. For example, "header sidebar main" defines three areas in a grid.
The grid-template-columns and grid-template-rows properties can also be used in combination with grid-template-areas to create a more complex grid layout.
Grid-template-columns defines the number of columns in the grid, and grid-template-rows defines the number of rows.
By using grid-template-columns and grid-template-rows with grid-template-areas, you can create a grid layout with specific areas for content.
The grid-template-areas property is a powerful tool for creating complex grid layouts, and it's a key part of CSS Grid Template Areas.
Explore further: Css Grid Auto Rows
Template Layout
The grid-template-areas property is a powerful tool for describing a layout with CSS Grid.
You can use it to create a grid with multiple areas, each spanning multiple rows and columns.
Each string in the grid-template-areas property represents a row of your grid, and you can use repeating names to connect cells and create a rectangular area.
For example, if you want to create a grid with four areas, each spanning two column tracks and two row tracks, you can use the following property and value: grid-template-areas: "one one one one" "one one one one" "one one one one" "one one one one";
Items are placed into the layout by being named with an ident using the grid-area property.
You can see this in action in the CodePen example shown in the article, where four items are assigned to the relevant grid area using the grid-area property and display on the grid in the correct boxes.
To create a more complex layout, you can use the grid-template-areas property in combination with other CSS Grid properties, such as grid-template-rows and grid-template-columns.
For example, you can create a grid with a header across the top, a footer across the bottom, and the main content in the middle row, as shown in the Responsive Holy Grail layout example.
To span rows or columns in the grid-template-areas property, you can use repeating names to connect cells.
For example, if you want to create a named area called feature and for it to take up two columns in the top row, you can use the following declaration: grid-template-areas: "feature feature feature feature" "main main main main" "footer footer footer footer";
A different take: Grid Area Css
You can also span cells and rows in the vertical direction by starting each row with the same named area.
For example, if you want to create a grid with a feature area that spans two columns and two rows, you can use the following declaration: grid-template-areas: "feature feature" "feature feature" "main main" "footer footer";
Curious to learn more? Check out: Css Text Area
Grid Tracks and Gaps
Grid tracks and gaps are essential components of a CSS grid layout. The gap property is a shortcut for setting both row-gap and column-gap.
You can use a single value for gap, which applies to both row-gap and column-gap. Alternatively, you can specify two values, where the first value sets the row-gap and the second value sets the column-gap.
The gap property creates gaps between grid cells or flex items, but it doesn't add gutters on the outer edges.
Here's a breakdown of the properties used to specify the size of grid lines:
- column-gap: specifies the size of the grid lines between columns
- row-gap: specifies the size of the grid lines between rows
- grid-column-gap: an older property that will be removed in favor of column-gap
- grid-row-gap: an older property that will be removed in favor of row-gap
These properties take a length value, which sets the width of the gutters between the columns/rows.
Grid Alignment
Grid Alignment is a crucial aspect of CSS Grid Template Areas. You can use the align-items property to align grid items along the block (column) axis.
The align-items property has several values, including stretch, start, end, center, and baseline. The default value is stretch, which fills the whole height of the cell. You can also use modifiers like first baseline and last baseline for multi-line text.
To align items along the inline (row) axis, you can use the justify-items property, which is not discussed here. Instead, we'll focus on align-items and its modifiers. The safe and unsafe keywords can be used to fine-tune the alignment, but that's a topic for another time.
Here's a quick rundown of the align-items values:
- stretch – fills the whole height of the cell
- start – aligns items to be flush with the start edge of their cell
- end – aligns items to be flush with the end edge of their cell
- center – aligns items in the center of their cell
- baseline – align items along text baseline
Align-Items
Align-items is a crucial property in grid alignment that helps you control how grid items are arranged along the block axis. It's the default value for all grid items inside a container.
You might like: Css Grid Align Items
You can choose from five main values for align-items: stretch, start, end, center, and baseline. The stretch value fills the whole height of the cell, which is the default behavior.
The start value aligns items to be flush with the start edge of their cell, while the end value does the opposite, aligning items to the end edge. The center value, as you might expect, aligns items in the center of their cell.
The baseline value aligns items along the text baseline, but there are modifiers for this value too - first baseline and last baseline. These modifiers use the baseline from the first or last line in the case of multi-line text.
Here are the main align-items values in a quick reference list:
- stretch – fills the whole height of the cell
- start – aligns items to be flush with the start edge of their cell
- end – aligns items to be flush with the end edge of their cell
- center – aligns items in the center of their cell
- baseline – aligns items along text baseline
- first baseline – uses the baseline from the first line in the case of multi-line text
- last baseline – uses the baseline from the last line in the case of multi-line text
You can also use the safe and unsafe keywords with align-items. The safe keyword means "try to align like this, but not if it means aligning an item such that it moves into inaccessible overflow area". The unsafe keyword, on the other hand, will allow moving content into inaccessible areas.
For your interest: Css Grid Vertical Align
Justify-Content
Justify-Content is a property that aligns the grid along the inline (row) axis, which is different from align-content that aligns the grid along the block (column) axis.
This property is useful when the total size of your grid is less than the size of its grid container, which can happen if all of your grid items are sized with non-flexible units like px.
The justify-content property can be set to one of several values, including start, end, center, and stretch.
Here are the possible values for justify-content:
Grid Auto-Flow
Grid auto-flow is a crucial property in CSS grid template areas that determines how items are automatically placed on the grid if not explicitly defined. It's controlled by the grid-auto-flow property.
The default value of grid-auto-flow is row, which means the auto-placement algorithm will fill in each row in turn, adding new rows as necessary. This is the most straightforward way to use grid auto-flow.
Here's an interesting read: Css Grid Row
You can also set grid-auto-flow to column, which tells the auto-placement algorithm to fill in each column in turn, adding new columns as necessary. This will change the layout of your grid, but keep in mind that it might cause items to appear out of order, which is bad for accessibility.
If you want to get a bit fancier, you can use the dense value, which tells the auto-placement algorithm to attempt to fill in holes earlier in the grid if smaller items come up later. This can create a more visually appealing layout, but it's not recommended for accessibility reasons.
Here are the possible values for grid-auto-flow, along with a brief description of each:
- row - fills in each row in turn, adding new rows as necessary (default)
- column - fills in each column in turn, adding new columns as necessary
- dense - attempts to fill in holes earlier in the grid if smaller items come up later
Properties for Children
Grid items have their own set of properties that control their behavior within a grid container. These properties include grid-column-start, grid-column-end, grid-row-start, grid-row-end, grid-column, grid-row, and grid-area.
These properties are used to define the size and position of a grid item within the grid. Grid items can also have their alignment and justification controlled using the justify-self, align-self, and place-self properties.
Here are the properties for children in a grid container:
- grid-column-start
- grid-column-end
- grid-row-start
- grid-row-end
- grid-column
- grid-row
- grid-area
- justify-self
- align-self
- place-self
Some properties have no effect on a grid item, including float, display: inline-block, display: table-cell, vertical-align, and column-* properties.
Grid Units and Functions
The repeat() function can save some typing, making it a convenient tool for working with CSS Grid template areas.
One notable feature of the repeat() function is its ability to be combined with keywords, which can significantly enhance its functionality.
The two main keywords to know are auto-fill and auto-fit.
Here's a brief rundown of what each keyword does:
These keywords can make a big difference in how your grid layout looks and functions, especially when combined with the repeat() function.
Grid Layout and Rules
Grid layout can be tricky, but there are some rules to keep in mind to make it work.
You must describe a complete grid, which means every cell must be filled. If you want to leave a cell empty, use a dot (.) or a series of dots with no space between them.
Leaving cells empty can sometimes cause issues, like item three only displaying in the last row of the grid.
You can only define each area once, so be careful not to duplicate an area, as this will make the entire property invalid.
Non-rectangular areas are also a no-go, so you can't create an 'L' or 'T' shaped area with grid template areas.
For more insights, see: Css No Class
Grid Layering and Positioning
Grid layering and positioning is a powerful feature of CSS grid template areas. You can add additional items to the grid after defining your main layout.
Only one name can occupy each cell when using grid-template-areas, but you can still use line numbers as usual. This is useful for adding extra items to your grid.
You can use line names defined when creating your usual columns or rows, which is a convenient way to position items. Even better, you'll have some line names created by the formation of the areas.
You get four line names with the name of the area, plus start and end edge lines with -start and -end appended. For example, the area named "one" has start edge lines named "one-start" and end edge lines named "one-end".
These implicit line names can be used to place an item on the grid, which is useful if you're redefining the grid at different breakpoints. This way, the item will always come after a certain line name.
Discover more: Css Grid Lines
Responsive Design
Using grid-template-areas can be very helpful in responsive design, especially when building up components in a component library.
It's straightforward to redefine a component at different breakpoints by redefining the value of grid-template-areas, sometimes in addition to changing the number of available column tracks.
You can create a single column layout for a component, then redefine the number of columns and grid-template-areas at a minimum width of 600px to create a layout with two columns.
The nice thing about this approach is that anyone looking at the CSS can see how the layout works.
The Holy Grail layout is a great example of using grid-template-areas in responsive design. It's a layout that was incredibly difficult to accomplish back in the days of using floats.
You can set up the named grid template areas in CSS and span rows where needed to create the layout.
Assigning each element to a named grid area is also a crucial step in creating the Holy Grail layout.
You can take this one step further by stacking the grid areas vertically by default, then building the Holy Grail on larger screens where it looks much better.
You might like: Css Grid Two Columns
Sources
- https://css-tricks.com/snippets/css/complete-guide-grid/
- https://www.smashingmagazine.com/2020/02/understanding-css-grid-template-areas/
- https://www.codecademy.com/learn/learn-intermediate-css/modules/learn-css-grid/cheatsheet
- https://css-tricks.com/almanac/properties/g/grid-template-areas/
- https://tutorial.techaltum.com/css-grids.html
Featured Images: pexels.com