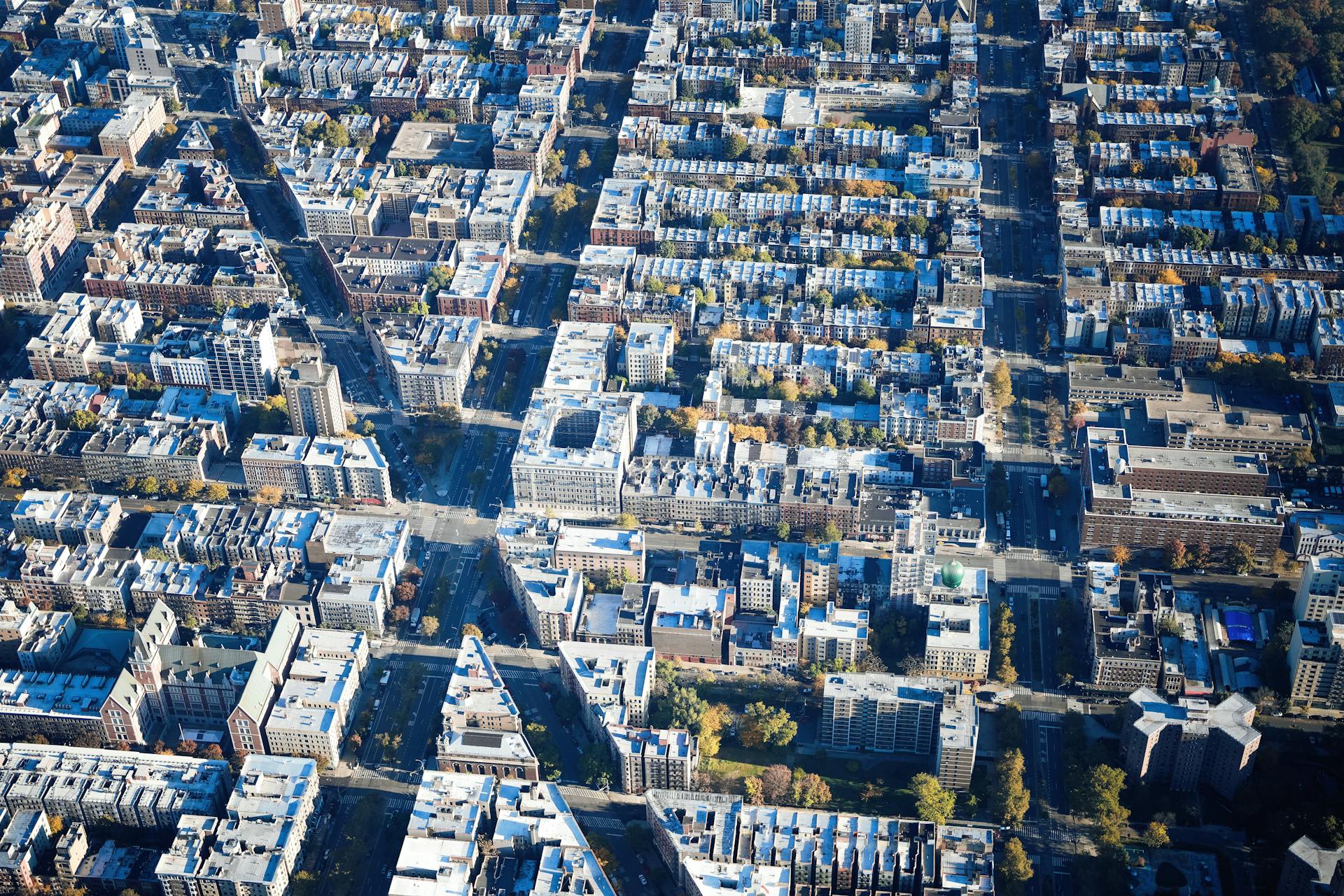
CSS Grid is a powerful tool for creating responsive and dynamic layouts. It allows you to create complex grid systems with ease, making it a game-changer for web developers.
The grid container is the foundation of a CSS Grid layout, and it's defined using the display property. To create a grid container, you simply set the display property to grid or inline-grid.
Grid templates are used to define the structure of the grid, and they're defined using the grid-template-columns and grid-template-rows properties. These properties allow you to specify the number and size of grid tracks, which are the rows and columns of the grid.
Grid tracks can be specified using a track list, which is a list of track sizes separated by space or a comma. For example, grid-template-columns: 100px 1fr 2fr; creates a grid with three tracks, where the first track is 100 pixels wide, and the second and third tracks take up the remaining space in a 2:1 ratio.
Grid Container
The grid container is the element on which display: grid is applied, making it the direct parent of all the grid items. It's the foundation of your grid layout.
A grid container can be any element, as long as display: grid is applied to it. This makes it super easy to create a grid layout in your HTML document.
The grid container is where you'll define the column and row sizes with grid-template-columns and grid-template-rows, and then place its child elements into the grid with grid-column and grid-row. This is where the magic of CSS Grid happens.
Here are some properties you can use on the grid container:
- display
- grid-template-columns
- grid-template-rows
- grid-template-areas
- grid-template
- grid-column-gap
- grid-row-gap
- grid-gap
- justify-items
- align-items
- place-items
- justify-content
- align-content
- place-content
- grid-auto-columns
- grid-auto-rows
- grid-auto-flow
- grid
Basics
Most browsers now support CSS Grid natively, with Chrome, Firefox, Safari, and Opera all having unprefixed support as of March 2017.
To get started with CSS Grid, you need to define a container element as a grid with display: grid.
Setting the column and row sizes is done with grid-template-columns and grid-template-rows, respectively.
Grid items can be placed in any order, which makes it easy to rearrange the grid with media queries.
Grid is one of the most powerful CSS modules ever introduced, making it a game-changer for building layouts that adapt to different screen widths.
Container
The container element is where it all starts. It's the grid container, the direct parent of all the grid items.
To define a grid container, you need to apply the display: grid property to it. This sets the stage for your grid layout.
The grid container is the foundation of your grid layout, and it's where you'll place all your grid items.
Grid Template
Grid Template is a shorthand for setting grid-template-rows, grid-template-columns, and grid-template-areas in a single declaration. It's a convenient way to define the structure of a grid, but it doesn't reset the implicit grid properties.
You can use grid-template to set the number of columns and rows, as well as the widths of each column. The number of width values determines the number of columns. For example, if you set three width values, you'll get three columns.
The grid-template-columns property is used to define the number and width of columns. You can individually set the width of each column or set a uniform width for all columns using the repeat() function.
The grid-template-rows property is used to define the number and height of rows. You can individually set the height of each row or set a uniform height for all rows using the repeat() function.
Grid-template-areas defines a grid template by referencing the names of the grid areas specified with the grid-area property. You can use any number of adjacent periods to declare a single empty cell.
Here's a breakdown of how to use grid-template-areas:
- grid-area-name: the name of a grid area specified with grid-area
- . : a period signifies an empty grid cell
- none: no grid areas are defined
For example:
This will create a grid that's four columns wide by three rows tall.
Note that you're not naming lines with this syntax, just areas. When you use this syntax, the lines on either end of the areas are automatically named.
Grid Items
Grid items are the building blocks of a CSS grid, and understanding how they work is crucial to creating effective grid layouts. Grid items are the direct descendants of the grid container.
Grid items can be aligned along the inline (row) axis using the justify-items property, which applies to all grid items inside the container. This property has four values: start, end, center, and stretch.
Here are the possible values for the justify-items property:
- start – aligns items to be flush with the start edge of their cell
- end – aligns items to be flush with the end edge of their cell
- center – aligns items in the center of their cell
- stretch – fills the whole width of the cell (this is the default)
Grid items can also be aligned along the block (column) axis using the align-items property, which also applies to all grid items inside the container. This property has six values: stretch, start, end, center, baseline, and modifiers like first baseline and last baseline.
Note that grid items can also have their own individual alignment properties, such as justify-self and align-self, which can override the container's properties.
Items
Items are the direct descendants of the grid container, and they're what make up the grid layout. They can be thought of as the individual elements that are arranged within the grid.
The align-items property is used on a grid container to determine how the grid items are spread out along the column. It sets the default align-self property for all child grid items, and its value can be start, end, center, or stretch.
You can also use the align-items property to align grid items along the block (column) axis. The default value is stretch, which fills the whole height of the cell.
The justify-items property, on the other hand, aligns grid items along the inline (row) axis. Its default value is stretch, which fills the whole width of the cell.
Here's a summary of the values for both align-items and justify-items:
The place-items property sets both the align-items and justify-items properties in a single declaration. This can be very useful for quick multi-directional centering.
You can also use the grid-column and grid-row properties to set a grid item's position within the grid. This is done by referencing the grid columns and rows using the grid-column-start and grid-column-end, and grid-row-start and grid-row-end properties.
Note that some properties, such as float, display: inline-block, and vertical-align, have no effect on a grid item.
Content
Content is a crucial aspect of grid items, and understanding how to manage it is essential for creating effective grids.
The align-content property can be used to position the entire grid along the column axis of the grid container.
If the total size of the grid items is smaller than the grid container, the grid can be centered vertically in the container using the value center.
The value stretch stretches the grid items to increase the size of the grid to expand vertically across the container.
The max-content keyword represents the smallest size required for an element to fit all of its content without being wrapped or overflowed.
A grid track with a size value of max-content will grow until its content can fit into a single line.
There are several options for aligning the grid, including start, end, center, stretch, space-around, space-between, and space-evenly.
Here are the options for aligning the grid:
Flex
Flex allows you to create flexible grid tracks by specifying the size of a track as a fraction of the available space in the grid container.
A non-negative value expressed in fr units makes this possible, enabling responsive grids that adapt to changing widths.
The remaining available space is whatever is leftover after the first column's fixed width is taken into account.
In a three-column grid, the second two columns divvy up the remaining space according to how many fractions they get.
One fraction is 200px, so the second column is also 200px wide.
A grid takes any gaps between grid tracks into account when figuring out how much available space there is to distribute to the fractional columns.
With a 15px gap between each column, we have 30px worth of gaps to factor into the available space.
This makes CSS grid awesome because it takes care of the math for us, so we don't have to do manual calculations.
Forms Elements
Forms Elements can be a real challenge to style with CSS. CSS Grid makes it easy to align form elements by telling the left column to display at max-content.
You can use CSS Grid to create a form with stacked text inputs where the input label is on the left and the input is on the right. This is achieved by having the left column display at max-content and the right column take up the rest of the space.
Block Level
To set an HTML element into a block-level grid container, use the display: grid property/value.
A block-level grid container is created when you use the display: grid property/value, making it a fundamental aspect of CSS Grid.
The nested elements inside a block-level grid container are called grid items.
Grid items can be arranged in a grid layout with rows and columns, making it a powerful tool for web design.
To create a block-level grid container, simply add the display: grid property/value to the HTML element you want to use as a grid container.
CSS Grid is a two-dimensional CSS layout system that allows for flexible and responsive grid layouts.
Grid Layout
Grid Layout is a powerful tool in CSS that allows you to create flexible and responsive layouts with ease. You can start out using the basics of CSS Grid to create a layout using a variety of measurement types, such as grid-template-columns, which creates a new column in the grid with the assigned width.
To define an element as a grid container, you can use the display property and set it to either grid or inline-grid, depending on whether you want a block-level or inline-level grid. This will establish a new grid formatting context for its contents.
To create a fluid layout, you can use the grid-template-columns property and set it to a fractional unit, such as 1fr, which will divide the screen into equal parts. For example, dividing the screen into 12 equal parts, with one box occupying one part and the remaining eight parts empty.
Here are the possible values for the display property:
- grid – generates a block-level grid
- inline-grid – generates an inline-level grid
Display
To create a grid container, you simply need to use the display property and set its value to grid. This will establish a new grid formatting context for its contents.
The display property can be set to either grid or inline-grid to create different types of grid containers. Here are the options:
- grid – generates a block-level grid
- inline-grid – generates an inline-level grid
Using display: grid will make the element a block-level grid container, while using display: inline-grid will make it an inline-level grid container.
Gap
The gap property is a crucial aspect of grid layout. It determines the size of the space between grid lines, often referred to as gutters.
You can set the gap size using the column-gap, row-gap, grid-column-gap, or grid-row-gap properties. These properties take a length value, such as a number followed by a unit like px or em.
The gutters are only created between grid lines, not on the outer edges of the grid. This means that you'll have space between columns and rows, but not around the entire grid.
The column-gap property is used to place a gap between columns inside the grid. It's a simple and effective way to create some breathing room between your grid items.
If you want to place a gap between rows, you'll need to use the row-gap property. This property works in conjunction with grid-template-rows to create the desired spacing between rows.
CSS also offers a shorthand property called gap, which combines the row-gap and column-gap properties into one. This can make it easier to set the gap size for both rows and columns at the same time.
Here's a quick summary of the gap properties:
- column-gap: sets the gap between columns
- row-gap: sets the gap between rows
- grid-column-gap: sets the gap between columns (deprecated)
- grid-row-gap: sets the gap between rows (deprecated)
- gap: shorthand for row-gap and column-gap
Keep in mind that the unprefixed properties (column-gap, row-gap, and gap) are already supported in modern browsers like Chrome, Safari, and Opera.
Column Row
You can determine a grid item's location within the grid by referring to specific grid lines using grid-column-start, grid-column-end, grid-row-start, and grid-row-end.
These properties can accept values like a number to refer to a numbered grid line or a name to refer to a named grid line.
You can also use the span keyword to specify how many grid tracks an item will span. For example, span 3 means the item will take up three grid tracks.
If no grid-column-end or grid-row-end is declared, the item will span 1 track by default.
Items can overlap each other, and you can use z-index to control their stacking order.
You can also use the shorthand properties grid-column and grid-row, which are equivalent to grid-column-start + grid-column-end and grid-row-start + grid-row-end, respectively.
The grid-column property can be used to join multiple columns together, and it's a shorthand for grid-column-start and grid-column-end.
To set the grid column widths, you can use the grid-template-columns property, like in the example where the screen is divided into 12 equal parts.
Here's a summary of how to specify grid item positions using grid-row and grid-column:
Justify Items
Justify items is used on a grid container to determine how grid items are spread out along a row by setting the default justify-self property for all child boxes.
The value start aligns grid items to the left side of the grid area. This is the default behavior, but you can also use the justify-items property to override this default.
The value end aligns grid items to the right side of the grid area. This can be useful when you want to create a layout where items are aligned to the right edge of the grid container.
The value center aligns grid items to the center of the grid area. This can be useful when you want to create a layout where items are centered horizontally in the grid container.
You can use the justify-items property to position grid-items (children) inside grid containers along the X-Axis (Main Axis) using the values start, end, center, or stretch.
Place Content
Place Content is a shorthand property that sets both the align-content and justify-content properties in a single declaration. It's a convenient way to align your grid items both horizontally and vertically.
The syntax for place-content is straightforward: you list the value for align-content followed by the value for justify-content. If you only provide one value, it will be assigned to both properties.
Here are the possible values for place-content, which are the same as the values for align-content:
- start
- end
- center
- stretch
- space-around
- space-between
- space-evenly
These values determine how the grid items will be aligned within the grid container.
Auto-columns
Auto-columns are a powerful feature of CSS Grid Layout that allows you to automatically generate grid tracks for grid items that don't have an explicit position. According to Example 10, you can use the grid-auto-columns property to specify the size of these auto-generated grid tracks.
You can set the size of auto-generated grid tracks to a length, percentage, or fraction of the free space in the grid using the fr unit. For example, if you want to create a 2 x 2 grid, you can set grid-auto-columns to 1fr 1fr, which will automatically generate two columns with equal width.
Implicit tracks get created when there are more grid items than cells in the grid or when a grid item is placed outside of the explicit grid. This is demonstrated in Example 10, where setting grid-auto-columns to 1fr 1fr creates two columns with equal width.
Here's a summary of the possible values for grid-auto-columns:
- Length (e.g. 100px)
- Percentage (e.g. 50%)
- Fraction of the free space in the grid (using the fr unit)
By using grid-auto-columns, you can create flexible and responsive grid layouts that adapt to different screen sizes and devices.
Inline Level
Inline Level Grids are a great way to create flexible layouts. You can use display: inline-grid to set an HTML element into an inline-level grid container.
The nested elements inside this element are called grid items. To set alignment for all the items in a grid, you can use the justify-items property.
You can use the grid-column property to join multiple columns together. This is a shorthand of two properties: grid-column-start and grid-column-end.
To create a grid with 12 equal parts, you can use the fr unit, which stands for fraction. For example, 1fr means one fraction, or one part of the grid.
Here's a breakdown of the grid-column property:
- grid-column: start/end
- grid-column-start
- grid-column-end
These properties allow you to specify the start and end points of a column, making it easy to create complex grid layouts.
Frequently Asked Questions
How to set grid system in CSS?
To set a grid system in CSS, define a container element with display: grid and specify column and row sizes with grid-template-columns and grid-template-rows. This sets the foundation for placing child elements into the grid with grid-column and grid-row.
How to specify columns in a grid?
To specify columns in a grid, use the CSS property grid-template-columns on the grid container and list the desired column widths in pixels (px) or percentages (%). The number of width values determines the number of columns.
Sources
- https://css-tricks.com/snippets/css/complete-guide-grid/
- https://css-tricks.com/almanac/properties/g/grid-template-columns/
- https://www.codecademy.com/learn/premium-intermediate-css-css-grid/modules/premium-learn-css-grid/cheatsheet
- https://brandonbrule.com/css-grid.html
- https://www.freecodecamp.org/news/css-grid-tutorial-with-cheatsheet/
Featured Images: pexels.com