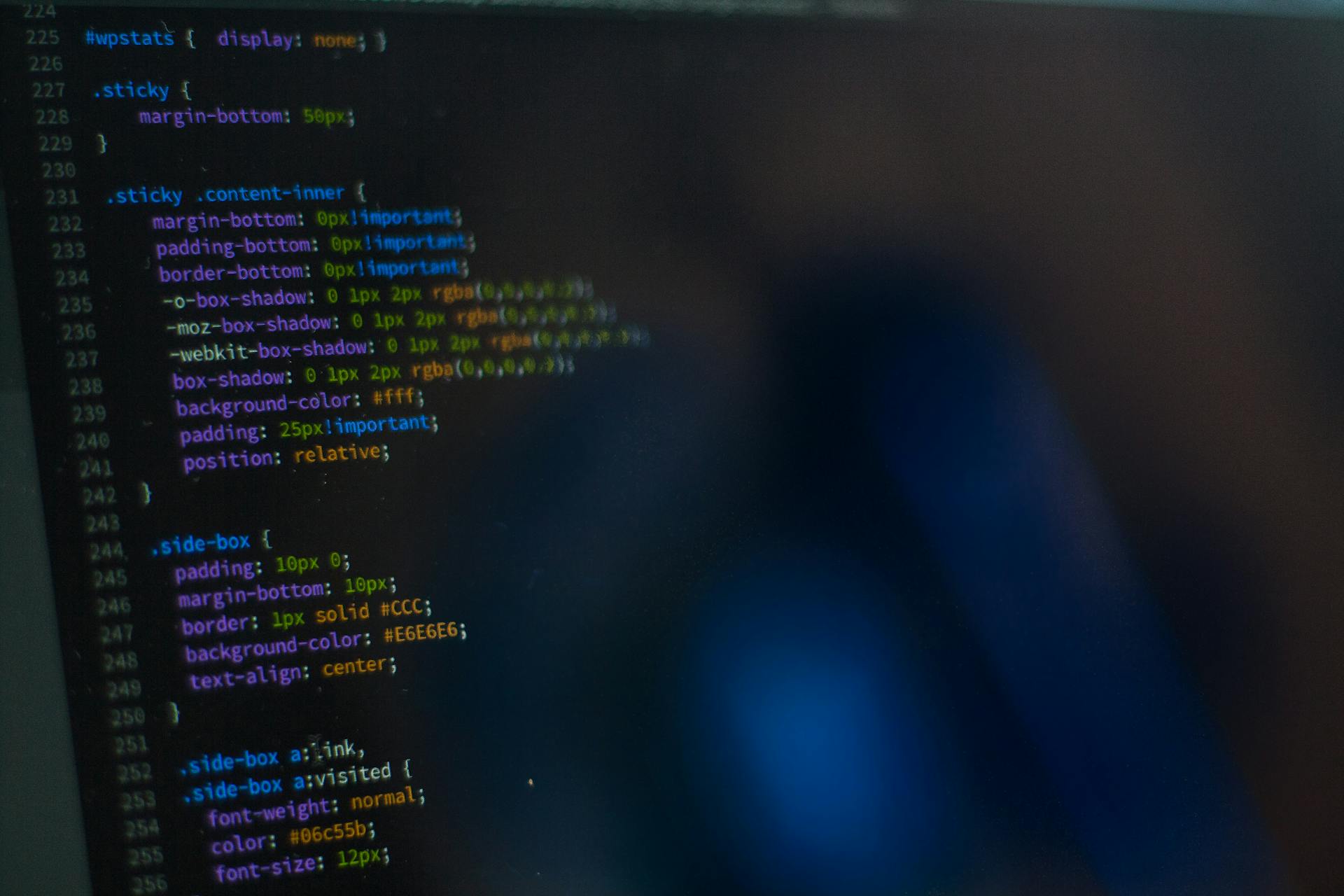
CSS regex selectors are a powerful tool for selecting elements on a webpage based on their attribute values. They use a pattern-matching syntax to filter elements that match specific criteria.
One key aspect of CSS regex selectors is that they use a special syntax to match attribute values, which can include characters like dots, commas, and parentheses. This syntax is different from the syntax used in JavaScript regex.
In CSS regex selectors, the `^` character matches the start of a string, while the `$` character matches the end of a string. For example, the pattern `^hello` would match only the string "hello", not "hello world".
Selecting Elements
Selecting elements is a crucial part of CSS regex selectors, and there are several ways to do it.
You can use XPath syntax to get the value of an attribute, and it's a standard feature that can be used in other parts of an XPath expression.
Related reading: Css Selector vs Xpath
The ::attr() CSS extension is another way to get attribute values, and it's a convenient option if you prefer to use CSS selectors.
The .attrib property of a Selector is also available, and it returns a dictionary with attributes of a first matching element.
This property is particularly useful when a selector is expected to give a single result, such as selecting by element ID.
The .attrib property of an empty SelectorList is empty, so be sure to check for that case.
Attribute Starts With Selector [name^=”value”] selects elements that have the specified attribute with a value beginning exactly with a given string.
This selector is useful for selecting all elements that are enabled.
Additional reading: How to Find Css Selector of an Element
Regex in CSS Selectors
Regex in CSS Selectors can be a bit tricky, but once you get the hang of it, it's incredibly powerful. You can use it to select elements based on specific attribute values.
For instance, the Attribute Contains Prefix Selector allows you to select elements that have an attribute value starting with a given string, followed by a hyphen. This is useful for selecting checkboxes, which typically have a name attribute starting with "checkbox".
Here's an interesting read: Css Select Data Attribute in Pseudo Selector
The Attribute Starts With Selector is similar, but it selects elements that have an attribute value beginning exactly with a given string. This is useful for selecting all elements that are enabled, which typically have a disabled attribute starting with "disabled".
These selectors can be super useful for targeting specific elements on a webpage. For example, you could use the Attribute Contains Prefix Selector to select all checkboxes on a form.
Curious to learn more? Check out: Css Selector Disabled
Selector Syntax
Selector syntax is a crucial part of CSS regex selectors. It allows you to target specific elements on a webpage.
The syntax is based on a pattern language, which is a set of rules used to match strings. This pattern language is made up of several elements, including character classes, quantifiers, and groups.
To start writing a CSS regex selector, you need to specify the selector type, which can be either a tag, class, or ID selector. For example, the selector `div` targets all div elements on the webpage.
Expand your knowledge: Div Class Css
Using Selectors
You can get a value of an attribute using XPath syntax, which has a few advantages, including being a standard XPath feature and allowing attributes to be used in other parts of an XPath expression.
Scrapy provides an extension to CSS selectors (::attr(...)) for getting attribute values. This is another way to get attribute values.
The .attrib property of Selector is also available for looking up attributes in Python code. It's convenient to use when a selector is expected to give a single result.
The .attrib property of an empty SelectorList is empty.
Selector has a .re() method for extracting data using regular expressions. It returns a list of strings.
You can use the .re_first() helper to extract just the first matching string.
There's also Selector.getall(), which returns a list.
Selector can be used to select elements that have a specified attribute with a value ending exactly with a given string. This is done using the Attribute Ends With Selector syntax [name$="value"].
Selector can also be used to select all elements that are visible.
Take a look at this: Css Selector for Data Attribute
Beware of //node[1] vs (//node)[1]
When working with selectors, it's easy to get tripped up by the syntax. Specifically, the difference between //node[1] and (//node)[1] can be a common source of confusion.
//node[1] selects all nodes occurring first under their respective parents. This means if you have multiple elements under different elements, this selector will grab all of them.
On the other hand, (//node)[1] selects all nodes in the document and then gets only the first of them. This means it doesn't matter what parent the node has, it will only grab the first one.
Here's a simple table to illustrate the difference:
For example, if you have a document with multiple elements under different elements, //node[1] will grab all the first elements, while (//node)[1] will only grab the first element in the entire document.
Expand your knowledge: Css Selector the Last 2 Child Elements
Using Exslt Extensions
Scrapy selectors are built atop lxml and support some EXSLT extensions. This means you can use EXSLT extensions in your XPath expressions.
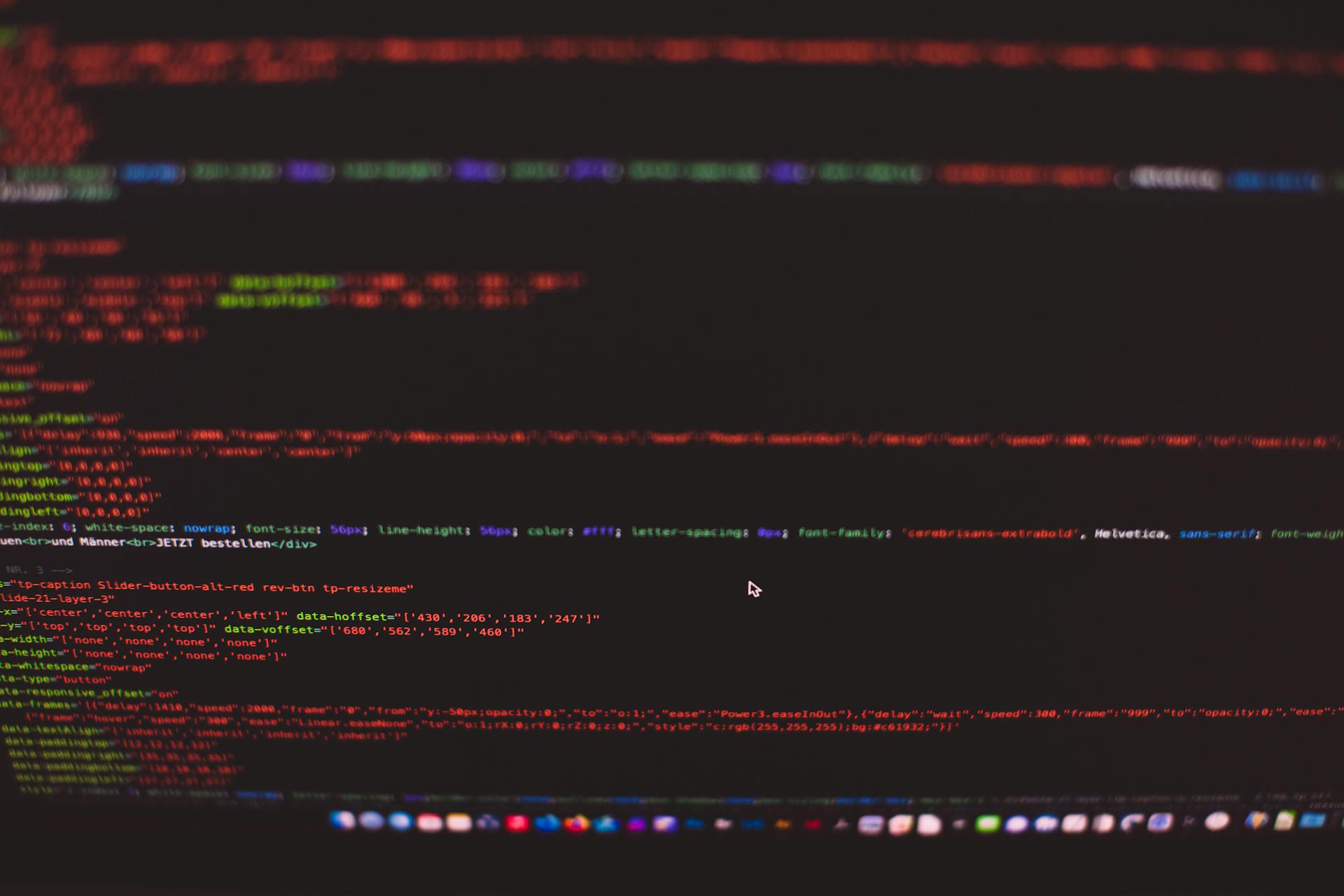
One of the supported extensions is regular expressions, which can be used with the re namespace. The re namespace is registered with the prefix 're' and the namespace 'http://exslt.org/regular-expressions'.
You can use regular expressions to apply a regex and return a list of strings with the matches. By default, character entity references are replaced by their corresponding character, except for & and <. However, you can switch off these replacements by passing replace_entities as False.
Here's a table summarizing the pre-registered namespaces for EXSLT extensions:
Prefix Selector Syntax
Prefix selectors are a powerful tool in the world of CSS. They allow you to select elements based on the presence of a certain attribute, and its value.
The syntax for a prefix selector is [name|="value"], where "name" is the attribute you're looking for, and "value" is the value of that attribute.
For example, if you have an attribute called "type" with a value of "checkbox", the prefix selector [type|="checkbox"] would select all elements of that type.
This can be useful for selecting elements that have a specific attribute, but where the value of that attribute is not fixed.
A unique perspective: Css Selector First of Type
Contains Selector [Name*=\"Value\"]
The Contains Selector [Name*="Value"] is a powerful tool in your CSS toolkit. It selects elements that have the specified attribute with a value containing a given substring.
This selector is particularly useful when you need to target elements based on a partial match of their attribute value. For instance, if you have a checkbox input with a name attribute containing the word "checkbox", this selector will select it.
The Contains Selector [Name*="Value"] is also useful for selecting the last matched element. This is because it will stop matching as soon as it finds the first element that meets the condition, and then return that element.
You can use this selector to select elements that have a value containing a specific substring, such as selecting all elements that have a name attribute containing the word "checkbox".
Check this out: Css Selector Contains Text
Selector Patterns
Selector patterns are a powerful tool in CSS regex selectors, allowing you to match specific elements on a webpage.
In a CSS regex selector, you can use the `^` symbol to match the start of a string, as seen in the example `^#header`, which matches the element with the id "header" exactly at the start of the string.
You can also use the `*` symbol to match any characters, including none, as shown in the example `#header*`, which matches any element with an id that starts with "header".
Selector patterns can be combined using the `|` symbol to match either of two patterns, as demonstrated in the example `#header|footer`, which matches either the element with the id "header" or the element with the id "footer".
You might enjoy: Selector Id Css
Selector Values
Selector values are a crucial part of CSS regex selectors, allowing you to precisely target elements based on their attributes.
To match an attribute with a specific name, you can use the "name" operator. For example, "name" will select any element that has an attribute with that name.
A unique perspective: Css Name Selector
Matching attributes with a specific name and value is done using "name=value". This operator ensures the element has both the attribute name and the exact value specified.
Attribute values can also be matched using the "name^=value" operator, which checks if the attribute value starts with the specified value.
Another option is the "name$=value" operator, which verifies if the attribute value ends with the specified value.
The "name*=value" operator is used to check if the attribute value contains the specified value.
You can also use the "name~=word" operator to match attribute values that contain a specific word, separated by spaces.
Lastly, the "name|=word" operator checks if the attribute value starts with a specified word.
Readers also liked: Css Selector That Targets a Specific Style Declaration
Sources
Featured Images: pexels.com