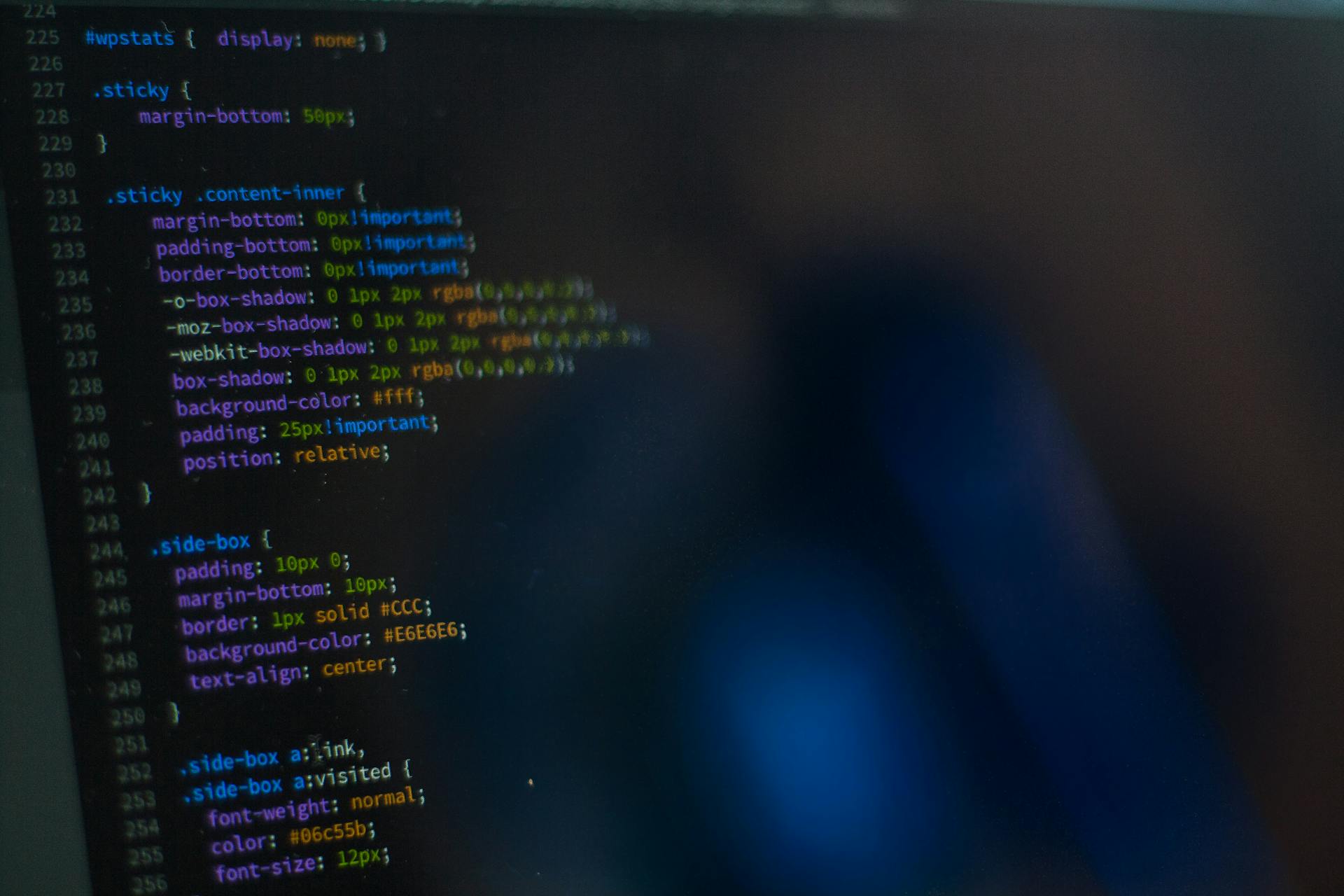
As a web developer, you're likely no stranger to CSS selectors, but even the most seasoned pros can get tripped up by the nuances of different selector types.
The universal selector (*) is the most general selector, selecting all elements on the page.
For more targeted selections, you can use the tag selector to choose specific HTML elements, like headings or paragraphs.
The class selector, denoted by a dot (.), allows you to select elements based on their class attribute, making it perfect for styling groups of elements with shared characteristics.
Additional reading: Css Selector the Last 2 Child Elements
Basic Selectors
Basic Selectors are the foundation of CSS, and they're used to target specific parts of your document for styling. Simple selectors directly select one or more elements, and they're often the ones you'll use the most.
There are four types of simple selectors: the universal selector (*), element selectors, class selectors, and ID selectors. Element selectors target elements based on their name or type, while class selectors target elements based on their class value.
You can use simple selectors to locate HTML elements for web scraping, and many web scraping and automation tools allow you to use CSS selectors to identify HTML elements.
Simple
Simple selectors are the building blocks of CSS, and understanding them is crucial for writing effective styles.
You can use the universal selector, *, to select one or more elements.
Simple selectors directly select one or more elements based on their name or type.
You can also select elements based on their class value or ID value.
Here are the four ways to use simple selectors:
- Universal selector: *
- Name/type of the element
- Class value of the element
- ID value of the element
Simple selectors will be the ones you use the most, especially if you have some experience writing CSS code.
Element
An element in CSS is a basic unit of a document that can be styled and manipulated. It can be an HTML element such as a paragraph, image, or link.
To target an element, you can use a simple selector like the universal selector, *., which selects all elements. You can also use a selector based on the name/type of the element, like p or img.
You can also select an element based on its class value using a dot notation, like .class-name. This is useful when you want to apply a style to multiple elements that share the same class.
Explore further: Css Name Selector
Another way to select an element is by its ID value using a hash notation, like #id-name. This is useful when you want to apply a style to a specific element that has a unique ID.
For example, you can use the pseudo-element selector ::first-letter to select the first letter of a block of text. This is useful when you want to style the first letter of a paragraph or a heading.
Here are some common pseudo-element selectors:
- ::first-line: Selects the first line of a block of text.
- ::first-letter: Selects the first letter of a block of text.
- ::before: Inserts content before the content of an element.
- ::after: Inserts content after the content of an element.
These pseudo-element selectors can be used to add a unique touch to your web page, such as adding a quote mark before a paragraph or an image before a heading.
=
The "=" selector is a powerful tool in CSS that allows you to target elements based on their attributes and values. This selector is used in the CSS [Attribute = Value] Selector, which narrows down the selection of elements.
The syntax for the "=" selector is as follows: element[attribute="value"] { // styling }. This code can be used to target specific elements, such as paragraphs written in a particular language.
For example, the code p[lang = "hi"] {color:red;font-size:20px;} targets only the paragraphs with the attribute "lang" and value "hi". The specified styling is then applied to these elements.
Here are some key points to remember about the "=" selector:
- The "=" selector is used to target elements based on their attributes and values.
- The syntax for the "=" selector is element[attribute="value"] { // styling }.
- This selector can be used to target specific elements, such as paragraphs written in a particular language.
By using the "=" selector, you can create more precise and targeted styles for your web pages.
First
The first-letter pseudo-element is a great way to style the first letter of a paragraph. It's commonly used in article writing to make the first letter bigger and bolder.
You can apply the first-letter pseudo-element to block-level elements, and it can be used with various properties such as font, color, background, margin, padding, border, text-decoration, vertical-align, text-transform, line-height, float, and clear.
The first-line pseudo-element is similar, but it styles the first line of an element instead of just the first letter. It's also applicable only to block-level elements.
Here's a comparison of the first-letter and first-line pseudo-elements:
The :first-of-type pseudo selector selects the first element of a given type among a group of sibling elements. It's similar to :first-child, but it considers only siblings of the same node type.
The :first-child pseudo-class represents the first element among a group of sibling elements, and it's identified by the term "first-child" along with the ":" symbol.
Here's an interesting read: Type Text Css
Cheatsheet
The cheatsheet for basic selectors is a must-have for any web scraping project. It's a quick reference guide that helps you navigate the world of selectors.
To select a direct child, use the '>' symbol. This is a simple but essential selector.
For more complex selections, you can use the space character to select any descendant. This is a powerful tool for selecting elements that are nested deeply within a page.
You can also use the ~ symbol to select the following sibling, and the + symbol to select the direct following sibling. These selectors are useful for selecting elements that are adjacent to each other.
To join multiple selectors, use the comma (,) character. This is a handy way to combine multiple selectors into a single expression.
Here's a quick reference guide to some of the most common selectors:
These selectors are the foundation of any web scraping project, and mastering them will make your life much easier.
Grouping and Combining
Grouping and Combining CSS selectors can be a game-changer for web developers. You can target multiple elements at once using a comma to group and separate different elements.
To group multiple elements, simply use a comma to separate them, as shown in the example where div, p, and span elements are targeted and styled together.
Combinators can also be used to combine multiple selectors and create a more specific selection scope. The descendant combinator (space) selects elements that are descendants of a specified element, while the child combinator (>) selects elements that are direct children of a specified element.
The adjacent sibling combinator (+) selects elements that are immediately following a certain element and share the same parent, while the general sibling combinator (~) selects elements that are siblings of a certain element.
Here are some examples of combinators in action:
Grouping selectors can also be used to select multiple elements at once, such as h1, h2, and h3 elements. By separating the selectors with a comma, you can apply the same styles to multiple elements.
For another approach, see: Css Multiple Selector
Pseudo-classes can be combined with CSS classes to narrow down selections and make selectors work less. This can be achieved by assigning a class to an HTML tag and then using a pseudo-class selector to select the class.
Pseudo-elements can also be combined with CSS classes to give more control to developers and add flexibility to designs. By combining a pseudo-element with a CSS class, you can create unique and creative designs on web pages.
Intriguing read: Css Select Data Attribute in Pseudo Selector
Attribute Selectors
Attribute selectors are a powerful tool in CSS that allow you to select elements based on their attributes. These selectors can be used to target specific elements on a web page, making it easier to style and manipulate them.
The general syntax of using attribute selectors is [attribute ^= “value”], which selects all elements with an attribute that starts with the specified value.
You can also use the |= operator to select attribute values that start with the specified value, but only if the value is a single word or hyphenated. For example, the selector p[title |= "shirt"] will select elements with a title attribute that starts with "shirt", such as "shirt-blue" or "shirt-red", but not "shirt blue".
A fresh viewpoint: Css Selector Select Child of Parent
Here are some common attribute selectors and their uses:
Universal
The universal selector is a powerful tool in CSS that allows you to select every single element on a web page.
You can use the universal selector by adding an asterisk, *, to your CSS code. This will apply the styles to every element on the page.
The universal selector is useful for resetting browser defaults, such as padding and margin, to zero at the top of your CSS file.
However, be careful not to overuse the universal selector, as it can lead to repetitive code and make your CSS more difficult to maintain.
Here are some examples of how to use the universal selector:
- To color every element on the page red, use the following code: `* {color:red;}`
- To reset browser defaults, use the following code: `* {padding:0; margin:0;}`
As shown in the example code, the universal selector can be used to apply styles to every element on the page, making it a useful tool for CSS developers.
Attribute
Attribute selectors in CSS are a powerful tool for selecting HTML elements based on their attributes. They help developers provide specific instructions to web elements and give more control to handle these elements through JavaScript or CSS.
Attributes can be used with CSS selectors to target specific elements, and the general syntax of using CSS attribute selectors is [attribute |="value"]. This syntax selects all those attribute values that start with the word "value".
The CSS [attribute |= “value”] selector is useful when the attribute value is a single word or is hyphenated. However, it will not select values with white space in between. For example, if you have an attribute value like "shirt blue", only the paragraph with the attribute value "shirt-blue" will be selected.
The CSS [attribute ^= “value”] selector is similar to the previous one but does not require the attribute value to be a whole word. Any value that starts with the word "value" will be selected for styling.
Here are the different types of attribute selectors:
By using the i suffix, any attribute matcher can be made case-insensitive. This means that the attribute value can be matched regardless of the case used. For example, the span and div elements can be selected as they match the data-item attribute value case-insensitively.
By Ending With
The $= selector is a powerful tool for selecting elements based on attribute values that end with a specific string exactly. This is particularly useful when working with URLs or file paths.
You can use the $= selector to match attribute values that end with a specific string, such as a file extension. For example, if you want to select all elements with a src attribute that ends with .jpg, you can use the $= selector.
The Attribute Ends With Selector is another way to achieve the same result. According to MDN, this selector selects all elements with the attribute attr for which the value ends with val.
Take a look at this: Css Selector That Targets a Specific Property Declaration
Only of
Only of attribute selectors are a powerful tool in CSS, allowing you to target elements based on the presence or absence of a specific attribute.
You can use the only of selector to select elements that have a certain attribute, but only if that attribute is present. For example, if you have an element with a class attribute, you can use the only of selector to select it.
The only of selector is useful for selecting elements that have a specific attribute, but not for selecting elements that don't have that attribute.
Sources
- https://www.freecodecamp.org/news/css-selectors-cheat-sheet-for-beginners/
- https://scrapfly.io/blog/css-selector-cheatsheet/
- https://www.roborabbit.com/blog/css-selector-cheat-sheet-a-practical-guide/
- https://www.lambdatest.com/blog/css-selectors-cheat-sheet/
- https://medium.com/design-code-repository/css-selectors-cheatsheet-details-9593bc204e3f
Featured Images: pexels.com