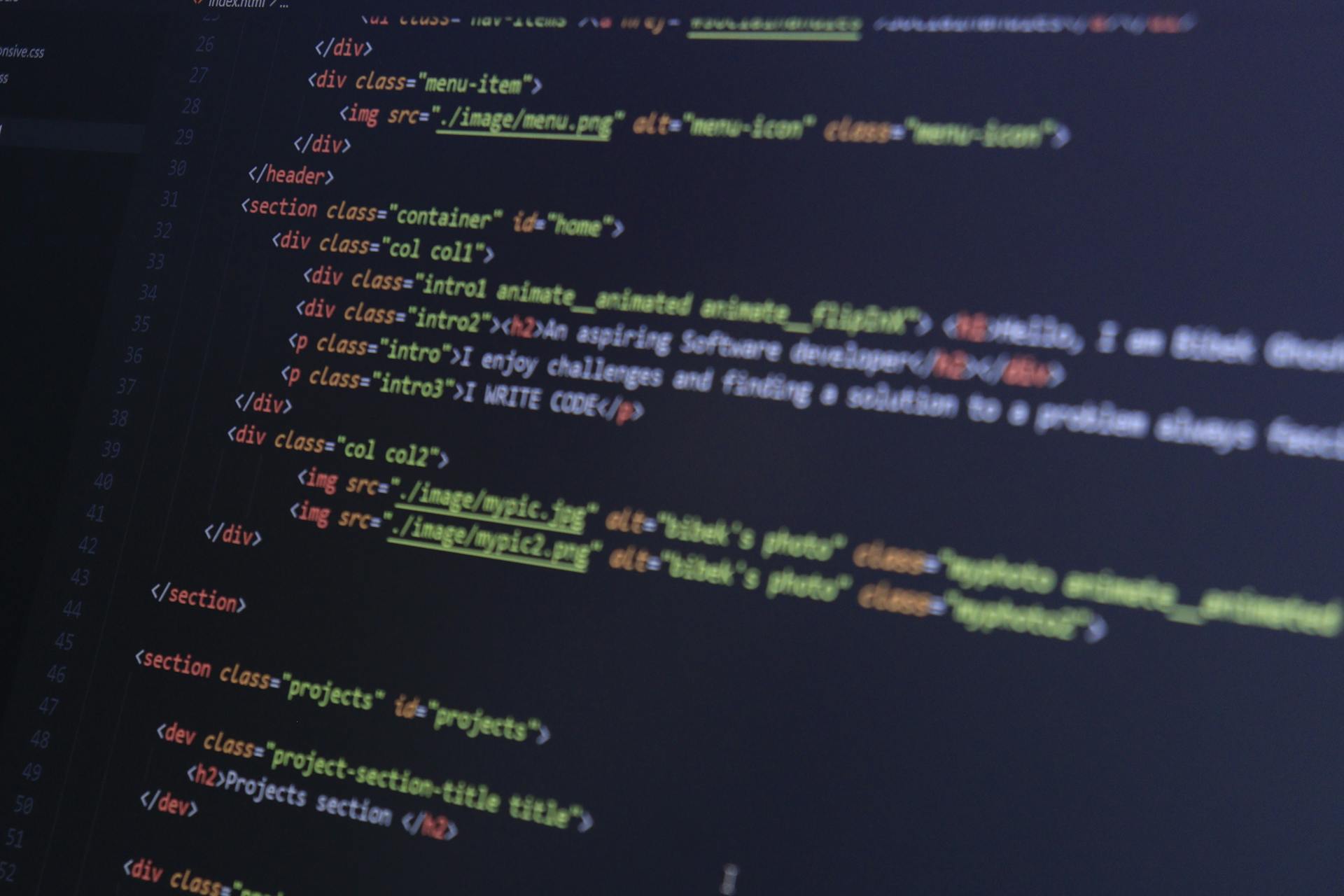
A CSS selector generator is a tool that helps you create specific selectors to target HTML elements on a web page. It's a game-changer for developers who want to improve their CSS skills and write more efficient code.
Using a CSS selector generator can save you a lot of time and effort, especially when working on complex projects. With it, you can generate selectors for elements based on their attributes, such as class, id, and tag name.
A well-crafted CSS selector can make a big difference in the performance and maintainability of your code. By targeting specific elements, you can avoid unnecessary styling and reduce the risk of conflicts with other CSS rules.
For instance, a CSS selector generator can help you create a selector that targets all paragraph elements with a specific class, like '.main-para'.
Basic Selectors
Basic selectors are the foundation of CSS selectors, and they're used to select elements based on their position in the document tree.
You can use the universal selector (*) to select all elements on the page, which is useful when you want to apply a style to every element.
The type selector (e.g., p, h1, etc.) selects elements based on their tag name, and it's the most common way to select elements.
You can also use the class selector (e.g., .header, .footer, etc.) to select elements with a specific class attribute.
Class Class-2 Descendant
When using multiple classes, you can chain them together to select elements that have all the specified classes. This is known as a class class-2 multiple selector.
For instance, a class class-2 multiple selector might look like this: `.class.class-2`. This selector will target elements that have both the class and class-2 classes.
You can also use a descendant selector to target elements that are descendants of another element. This is done by leaving a space between the selectors, like this: `.class .class-2`.
In a descendant selector, the first part of the selector targets the parent element, and the second part targets the child element.
Element of a Type
The :first-of-type pseudo-class is a powerful tool that targets the first element of its type within its parent.
This means that if you have multiple elements of the same type, like paragraphs, the :first-of-type pseudo-class will only select the very first one.
The :nth-of-type pseudo-class is used to match elements based on their type and position among siblings.
For example, if you want to select the third paragraph in a list of paragraphs, the :nth-of-type pseudo-class can help you do that.
Class Selectors
Class selectors are a fundamental part of CSS, allowing you to target elements with a specific class name. You can select all elements with a given class name using a dot followed by the class name, like this: .class.
This selector is particularly useful when you want to apply styles to multiple elements that share the same class. For example, if you have a button and a link with the same class name, you can use this selector to style both elements at once.
Class
The class selector is a powerful tool in CSS that allows you to target specific elements on a webpage based on their class name.
To use a class selector, you simply need to specify the class name in your CSS code, like this: .class class selector. This will select all elements with the specified class name.
You can have multiple elements on a webpage with the same class name, and the class selector will select all of them. This makes it a great way to apply styles to multiple elements at once.
Class selectors are often used in conjunction with other CSS selectors to create more complex and specific styles.
Class Multiple
Class selectors can be used to select elements based on their class names, and it's also possible to apply the same styles to multiple elements with different class names. You can do this by separating multiple selector declarations using a comma, as shown in the example ".class, .class-2 comma combinator".
To select all elements with a specific class name, you can use the ".class class selector" syntax. This is useful when you want to apply a style to all elements with a certain class. For instance, if you want to change the background color of all elements with the class "header", you can use this syntax.
You can also chain multiple classes or IDs to select elements that have all the specified classes or IDs. For example, ".class.class-2 multiple selectors" will select elements that have both the "class" and "class-2" classes. This is a powerful way to target specific elements on your web page.
Attribute Selectors
Attribute selectors are a powerful tool in CSS, allowing you to target elements based on their attributes. You can select elements that have a specified attribute with a value that starts with a certain string.
For example, if you want to target an element with a class attribute that starts with "header-", you can use the selector [class^=header-]. This is especially useful when you have multiple elements with different classes that start with the same prefix.
You can also target elements that have an attribute with a value that ends with a certain string. For instance, if you want to target an element with an id attribute that ends with "-footer", you can use the selector [id$=-footer].
Id
The ID selector is a powerful tool in your CSS arsenal. It allows you to select a specific element on a webpage by its unique ID.
You can use the #id syntax to select the element with the specified ID. For example, if you have an element with the ID "header", you can select it using the CSS code "#header".
The ID selector is particularly useful when you need to apply styles to a single element on a webpage. It's faster and more efficient than using a class selector, especially when you only need to style one element.
The ID selector is case-sensitive, so make sure to get the ID exactly right. If you have an ID like "header" and you try to select it using "#Header", it won't work.
Attribute & Value
Attribute selectors can be used to select elements based on the presence of a specific attribute. This is a powerful way to target elements on a webpage.
You can select elements that have a specified attribute using the [attr] attribute selector. For example, [class] would select any element with a class attribute.
The [attr$=val] attribute selector is used to select elements that have an attribute value that ends with a specific value. This can be useful for targeting elements with a specific suffix.
The [attr^=val] attribute selector is similar, but it's used to select elements that have an attribute value that starts with a specific value. This can be useful for targeting elements with a specific prefix.
You can also use the [attr*=val] attribute selector to select elements that have an attribute value that includes a specific value anywhere in the string. This can be useful for targeting elements with a specific keyword.
The [attr~=val] attribute selector is used to select elements that have an attribute value that includes a specific value as one of its space-separated values. This can be useful for targeting elements with a specific value among a list of values.
Checked Input Element(s)
The :checked pseudo-class is a powerful tool in CSS that targets specific elements when they're checked or selected. It's particularly useful for styling radio buttons, checkboxes, or options in a select element.
You can use appearance: none to remove the default styling of the checkbox input, allowing you to style it however you want. This is demonstrated in an example where the default checkbox styling is removed.
The :checked pseudo-class can also be used to style the sibling label of a checkbox input. This is shown in an example where the label is styled when the checkbox is checked or focused.
Pseudo-Class Selectors
Pseudo-class selectors are a powerful tool in CSS, allowing you to target specific elements based on their state or characteristics.
You can use the :link, :visited, :hover, and :active pseudo-classes to style links in various states, such as unvisited links, visited links, hovered-over links, and active links.
These pseudo-classes are often used with links, but :active can also be useful for buttons and :hover can be applied to all kinds of elements.
Here's a quick rundown of these pseudo-classes:
- :link - Targets unvisited links
- :visited - Targets visited links
- :hover - Targets hovered-over elements
- :active - Targets active elements
More pseudo-classes are available in CSS, including :root, :is(), and :where(), which can be used to target specific elements based on their characteristics.
Link Pseudo-Class
Link pseudo-classes are a powerful tool in CSS, allowing you to style elements in different states. They're most commonly used with links, but can also be applied to other interactive elements like buttons.
The four most basic link pseudo-classes are :link, :visited, :hover, and :active. :link targets unvisited links, while :visited targets links that have already been clicked on.
Here's a quick rundown of each:
- :link - targets unvisited links
- :visited - targets visited links
- :hover - targets elements when hovered over
- :active - targets elements when clicked
These pseudo-classes can be used to create a wide range of visual effects, from simple color changes to complex animations.
Focused Input Element(s)
Focused input element(s) can be targeted using the :focus pseudo-class, which is triggered when a user clicks on an input field or navigates to it using the keyboard.
The :focus pseudo-class is a powerful tool for styling and interacting with focused input elements.
A user's interaction with an input field, such as clicking or navigating to it, is what triggers the :focus pseudo-class.
First Child Element
The first child element is a great place to start when working with pseudo-class selectors. The :first-child pseudo-class targets the first child element within its parent.
To use this selector, you'll need to specify the parent element and the child element you want to target. For example, if you have a list item as the first child of an unordered list, you can use the :first-child pseudo-class to target it.
The :first-child pseudo-class is a simple but powerful tool for targeting specific elements on a webpage. It's often used in combination with other pseudo-class selectors to create more complex selections.
Here are some common use cases for the :first-child pseudo-class:
- Targeting the first item in a list
- Highlighting the first paragraph in an article
- Styling the first element in a container
By using the :first-child pseudo-class, you can add a touch of elegance and sophistication to your web design. It's a great way to draw attention to important elements on a webpage.
First Element of a Type
The :first-of-type pseudo-class is a powerful tool in your CSS toolkit. It targets the first element of its type within its parent.
This means that if you have multiple elements with the same class or tag name, :first-of-type will only select the very first one.
For example, if you have three paragraphs, :first-of-type will select the first paragraph, not the second or third.
Not() Negation Pseudo-Class
The :not() negation pseudo-class is a game-changer for filtering out unwanted elements. It allows you to target elements that don't match a specified selector or condition, essentially acting as an exclusion filter.
This pseudo-class is a functional one, meaning it's not just a simple negation, but a full-fledged selector that can be used in combination with other selectors to achieve complex filtering.
Sources
- https://fffuel.co/css-selectors/
- https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_selectors/Selectors_and_combinators
- https://codebeautify.org/css-selector-cheat-sheet
- https://stackoverflow.com/questions/8588301/how-to-generate-unique-css-selector-for-dom-element
- https://docs.wppopupmaker.com/article/147-getting-css-selectors
Featured Images: pexels.com