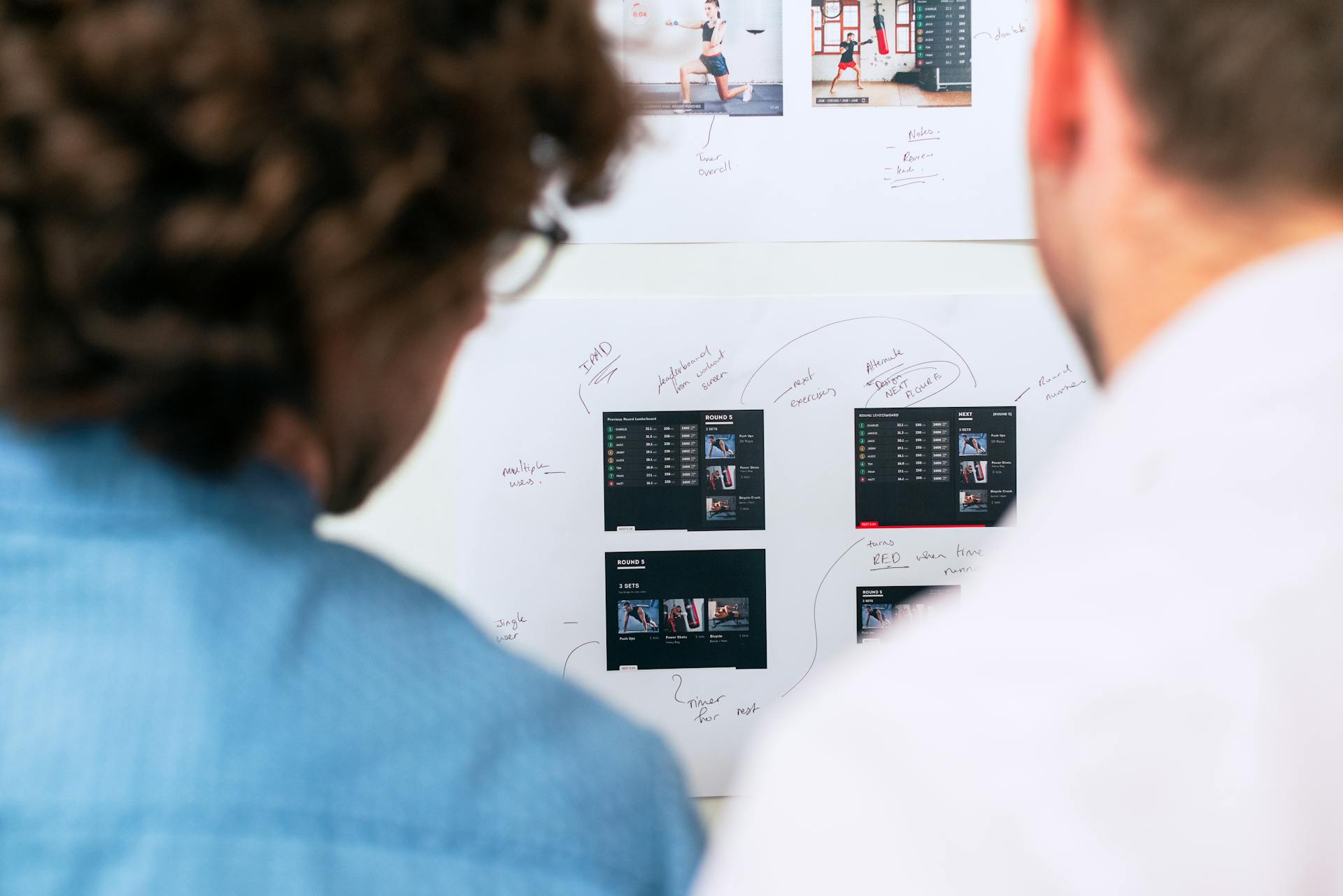
In Next.js, dependency injection helps manage complexity by decoupling components from their dependencies. This allows for easier testing and maintenance of code.
By using a container to hold and manage dependencies, Next.js developers can write more modular and reusable code.
For instance, in the example of a blog post component, dependency injection is used to inject a data service that fetches blog post data from an API. This makes the component more flexible and easier to test.
Using a dependency injection library like Inversify, Next.js developers can easily add and remove dependencies from their components.
For another approach, see: Hire Next Js Developer
What is Dependency Injection?
Dependency injection is a design pattern that helps reduce coupling between modules. It's like building a house with bricks, where each brick depends on the foundation rather than the one below it.
The Dependency Inversion Principle (DIP) states that high-level modules should not depend on low-level modules, but both should depend on abstractions.
This principle helps in decoupling modules, making them more reusable and easier to test. By depending on abstractions, you can replace individual components without affecting the entire system.
A fresh viewpoint: Azure Functions Dependency Injection
For example, in a house built with bricks, if the foundation is strong, the house remains stable even if individual bricks are replaced.
In Next.js, dependency injection can be implemented using React's context API or dependency injection. The StoreProvider component provides the Redux store to the rest of the application, decoupling the creation of the store from the components that use it.
Dependency injection promotes easier testing and reusability by reducing coupling between modules.
Implementing Dependency Injection in Next.js
To implement Dependency Injection in Next.js, you'll want to use a library like InversifyJS, which is a powerful and lightweight inversion of control container for JavaScript & Node.js apps powered by TypeScript. It has a good set of friendly APIs and can encourage the usage of the best OOP and IoC practices.
Inversify requires a modern JavaScript engine with support for Reflect metadata, Map, Promise, and Proxy. This is especially important if you're using provider injection or activation handlers.
To get started, you'll need to install the required dependencies, including Inversify and reflect-metadata. You can do this using npm or yarn.
Inversify required for implementing IoC in Typescript, and reflect-metadata required for Decorator and Decorator Metadata support for Typescript.
To set up Inversify, you'll need to modify your tsconfig.json file to include the necessary settings. This will allow the use of decorators in TypeScript.
Here are the settings you'll need to add:
- experimentalDecorators: true
- emitDecoratorMetadata: true
- types: true
- lib: true
Once you've set up Inversify, you can create a container and register your class instances. This is the final step to take for a properly working IoC.
You can specify the scope for the class instance using the following methods:
- inTransientScope: ensures a fresh instance for each dependency request, promoting lightweight and disposable dependencies.
- inSingletonScope: creates a central point of access for a dependency, ensuring a single instance is used everywhere, promoting efficiency and state management.
- inRequestScope: provides a performance optimization by avoiding redundant object creation within a single resolution process, but it doesn’t guarantee a single instance per HTTP request.
Typically, you only need the method for accessing the service, because it's the one who manages your data via repository. You can add a method to access your repository also if you need to.
InversifyJS
InversifyJS is a powerful and lightweight inversion of control container for JavaScript & Node.js apps powered by TypeScript. It has a good set of friendly APIs and can encourage the usage of the best OOP and IoC practices.
Inversify requires a modern Javascript engine with support for Reflect metadata, Map, and Promise (only required if using provider injection). This makes it a stable choice for production use.
Inversify is as lightweight as 4 KB in size, making it a great option for Next.js applications. Its simplicity and stability are major advantages over other IoC and Dependency Injection libraries.
Here are the system requirements for using Inversify:
- Reflect metadata
- Map
- Promise (only required if using provider injection)
- Proxy (only required if using activation handlers)
Security and Middleware
We use Nextjs middleware to check for a valid session for authenticated routes. This ensures that only authorized users can access certain parts of our application.
To do this, we create a service that wraps NextRequest from Nextjs. We use Effect.provideService to provide a valid implementation of NextRequest.
This approach makes it easy to compose services using Effect's services and layers. We can extract the token from the request using the cookie function, which is derived from NextRequest.
We then use the token to authorize requests to our database. This makes it easier to add more features, test, and refactor our code.
Explore further: Nextrequest Nextjs
Here are the methods derived from NextRequest that we use to extract relevant information from the request:
- cookie: Extracts the cookie value from the request
- pathname: Extracts the pathname from the request
- token: Uses the cookie function to extract the token from the Config
By using middleware and services, we can improve the security and maintainability of our Nextjs application.
Frequently Asked Questions
Is dependency injection still relevant?
Yes, dependency injection remains a valuable technique for managing dependencies in software development, regardless of the language or year. However, the use of dependency injection tools, such as containers, is a matter of debate.
Sources
- https://himynameistim.com/blog/dependency-injection-with-nextjs-and-typescript
- https://iqbalpa.medium.com/mastering-next-js-best-practices-for-clean-scalable-and-type-safe-development-2ee5693e73a9
- https://javascript.plainenglish.io/next-101-ioc-implementation-with-inversify-29ce548aad3b
- https://mmarinescu.hashnode.dev/oop-and-dependency-injection-in-nextjs
- https://www.sandromaglione.com/articles/next-js-authentication-with-effect-and-react-19
Featured Images: pexels.com