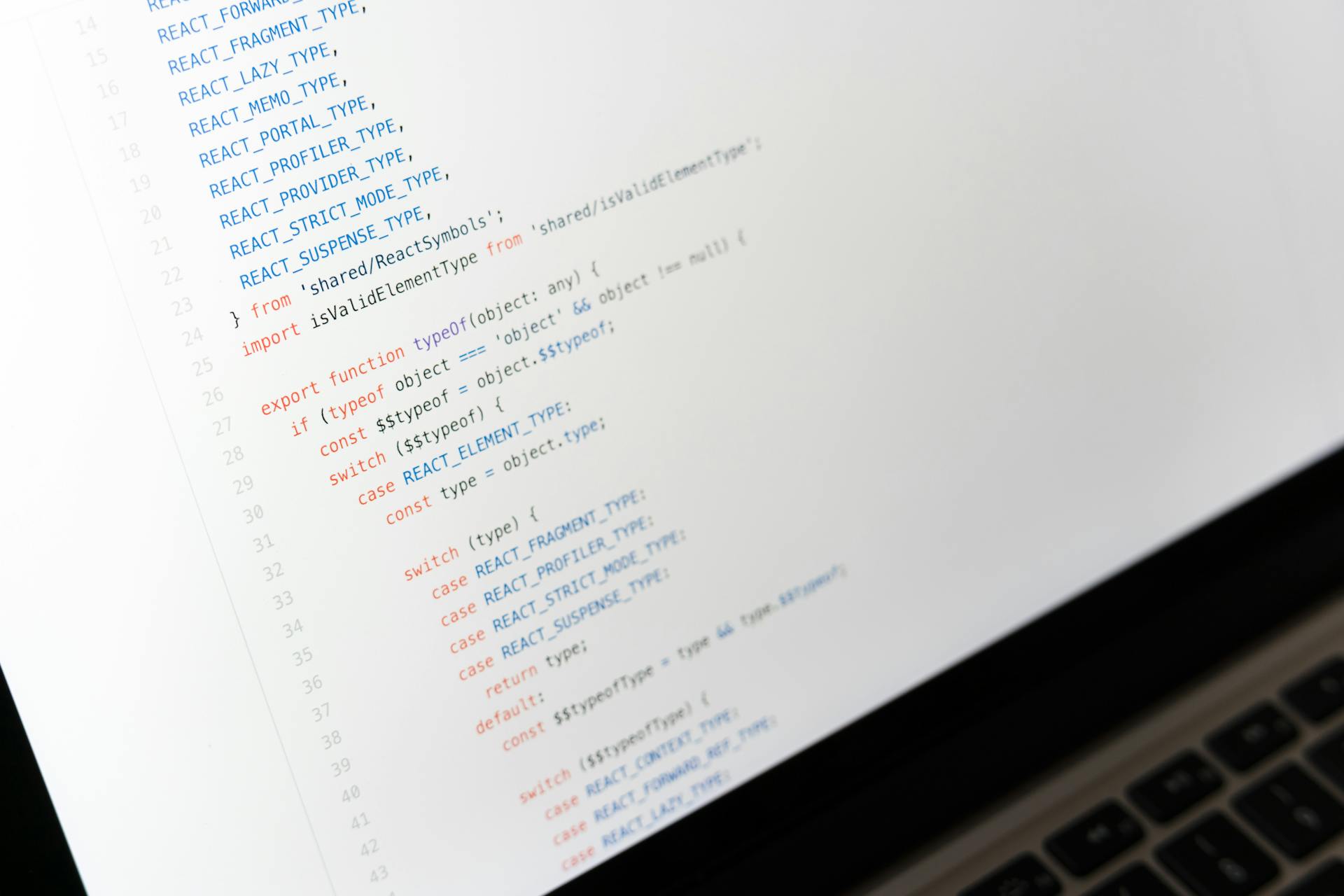
Next.js is an excellent choice for building scalable multi-tenant apps, thanks to its robust features and flexibility.
Next.js allows for easy API routing, making it simple to manage multiple tenants and their respective data.
With Next.js, you can create a centralized API that handles requests from all tenants, while also providing a scalable and efficient architecture.
By using Next.js, you can build a multi-tenant app that can handle a large number of users and tenants, making it perfect for large-scale applications.
Configuration File
The configuration file is a crucial part of setting up NextRequest Next.js, and it's now located in the root of your repository, specifically in a file named auth.ts.
This file should export the necessary functions you can use everywhere else in your application, similar to your previous route-based Auth.js configuration.
The configuration file has undergone some changes, and it's now much shorter compared to the old configuration file.
Here are some key things to note about the new configuration:
- This file can be named anything, but it's recommended to keep it short since you'll be importing the exported methods from here across your app.
- You no longer need to install @auth/core to import the provider definitions from, as they come from NextAuth itself.
- The configuration object passed to the NextAuth() function is the same as before.
- The returned methods exported from the NextAuth() function call are new and will be required elsewhere in your application.
Authentication
Authentication in Next.js has been simplified thanks to the investment in using standard Web APIs. This means you can now authenticate server-side with a single auth() function call in most cases.
The auth() function call is a game-changer, especially when working with Next.js components, which are server-first by default. This simplifies the authentication process and reduces the complexity of your code.
To give you a better idea of the changes, here's a summary of the authentication methods:
With these changes, you can now authenticate in various environments and contexts, including server components, middleware, client components, route handlers, and API routes.
Stripe Integration
Stripe Integration is a breeze with Next.js. You can get started with Next.js, TypeScript, and Stripe Checkout by following a simple guide.
To integrate Stripe with Next.js, you'll need to install the Stripe library and set up a Stripe account. This is a straightforward process that can be completed in just a few minutes.
Next.js provides a built-in integration with Stripe Checkout, which allows you to create a seamless checkout experience for your users. With Stripe Checkout, you can create a secure and PCI-compliant payment form that's easy to use.
To use Stripe Checkout with Next.js, you'll need to create a Stripe Checkout session and pass the session ID to your Next.js page. This can be done using the Stripe library's createCheckoutSession function.
Next.js and Stripe Checkout work together to create a fast and reliable checkout experience. With Next.js, you can create a custom checkout form that's tailored to your brand's look and feel.
Related reading: Next Js Strike Checkout
Contentful Integration
You can use Next.js with Contentful as your headless CMS. Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized websites.
Contentful is a cloud-based headless CMS that allows you to manage your content in a flexible and scalable way. It's a great choice for Next.js users who need a robust content management system.
On a similar theme: Headless Woocommerce Nextjs
To integrate Contentful with Next.js, you'll need to set up a Contentful space and create a Content Model to define the structure of your content. This will allow you to manage your content in a structured way and make it easily accessible to your Next.js application.
With Contentful, you can manage all your content in one place, including text, images, and other media. This makes it easy to keep your content up-to-date and consistent across all your Next.js pages.
By integrating Contentful with Next.js, you can create a seamless and efficient content management workflow that saves you time and effort in the long run.
For another approach, see: Create Next Js App
Ecommerce Integration
Next.js ecommerce integration is a breeze with Shopify. Learn how to build an ecommerce site with Next.js and Shopify.
You can use Next.js ecommerce with Shopify to create a seamless shopping experience for your customers. Next.js ecommerce with Shopify is a powerful combination that allows you to build a scalable and fast ecommerce site.
With Next.js ecommerce integration, you can easily connect your Shopify store to your Next.js application, allowing you to manage your products, inventory, and orders in one place.
Explore further: Nextjs Ecommerce
Stripe Checkout with TypeScript
Integrating Stripe Checkout with your ecommerce platform is a great way to streamline payments and improve the user experience. Next.js with Stripe Checkout and TypeScript is a popular combination for building scalable and secure ecommerce applications.
Using Stripe Checkout with TypeScript allows you to build a seamless payment experience for your customers. This is achieved by leveraging the Stripe Checkout API with TypeScript's type safety features.
With Next.js, you can easily integrate Stripe Checkout using the Stripe library. This library provides a simple and intuitive API for handling payments.
For another approach, see: Next Js 14 Ecommerce
Ecommerce with Shopify
Building an ecommerce site with Shopify is a great way to get started. You can learn how to do it with Next.js.
Shopify is a popular ecommerce platform that offers a lot of flexibility and customization options. It's a great choice for beginners and experienced developers alike.
Next.js is a React-based framework that makes it easy to build fast and scalable web applications. You can use it to build an ecommerce site with Shopify.
With Shopify, you can create a professional-looking online store in no time. It's user-friendly and has a lot of built-in features that make it easy to manage your products and orders.
Next.js ecommerce with Shopify is a great combination for building a fast and scalable ecommerce site.
Explore further: Next Js Static Site Generation
Deploying and Hosting
To deploy and host your Next.js app, you can start by using Vercel, a platform that makes it easy to deploy and host your app.
You can deploy a locally built Next.js app to Vercel, which is a great option if you're new to hosting or want a hassle-free experience.
Worth a look: Nextjs by Vercel Meaning
Middleware and Hooks
You can use a Next.js Middleware with NextAuth.js to protect your site. This allows you to run logic before accessing any page, even when they are static.
Middleware is a way to run logic before accessing any page, even when they are static. On platforms like Vercel, Middleware is run at the Edge.
You can get the withAuth middleware function from next-auth/middleware either as a default or a named import. This function can be reused and is a subset of the options available for NextAuth.js.
Here are the ways to create tRPC hooks:
* use the createTRPCNext function to create a set of strongly-typed hooks from your API's type signature
If this caught your attention, see: Nextjs Middleware Firebase Auth
Middleware
Middleware is a game-changer for Next.js developers. It allows you to run logic before accessing any page, even when they are static, and it's especially useful for protecting your site with NextAuth.js.
You can get the withAuth middleware function from next-auth/middleware either as a default or a named import. This function is a crucial part of setting up authentication for your Next.js site.
Middleware is run at the Edge on platforms like Vercel, which means it's fast and efficient. You can extract common configuration options to a reusable object, making it easier to manage your middleware setup.
Here are some key features of Next.js Middleware:
- Runs logic before accessing any page, even static pages
- Can be used with NextAuth.js to protect your site
- Runs at the Edge on platforms like Vercel
- Can reuse common configuration options
By using Middleware, you can create a more secure and efficient Next.js site. It's a powerful tool that's definitely worth exploring further.
Create Trpc Hooks
Create tRPC hooks using the createTRPCNext function to create a set of strongly-typed hooks from your API's type signature. This is a crucial step in setting up your tRPC API.
For another approach, see: Next Js Typescript Hooks
The createTRPCNext function is used to create a set of strongly-typed hooks from your API's type signature. This allows you to use the React hooks you have just created to invoke your API.
You can use the React hooks you have just created to invoke your API. For more detail, see the React Query Integration.
Here's a quick rundown of the steps to create tRPC hooks:
- Use the createTRPCNext function to create a set of strongly-typed hooks from your API's type signature.
- The createTRPCNext function is used to create strongly-typed hooks from your API's type signature.
- This allows you to use the React hooks you have just created to invoke your API.
Featured Images: pexels.com